C Functions
C structures, c exercises.
You can test your C skills with W3Schools' Exercises.
We have gathered a variety of C exercises (with answers) for each C Chapter.
Try to solve an exercise by editing some code, or show the answer to see what you've done wrong.

Count Your Score
You will get 1 point for each correct answer. Your score and total score will always be displayed.
Start C Exercises
Start C Exercises ❯
If you don't know C, we suggest that you read our C Tutorial from scratch.

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.
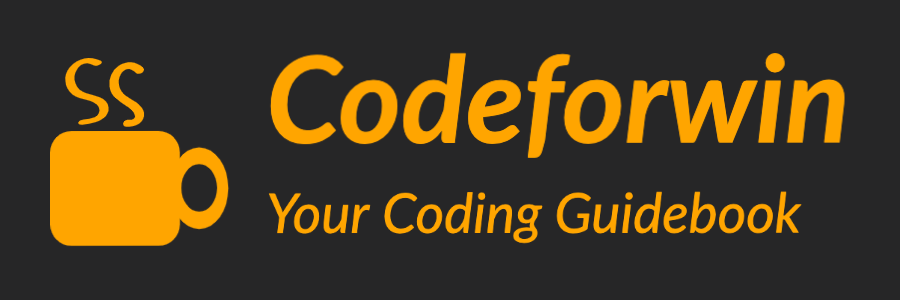
Function, recursion programming exercises and solutions in C
A function is a collection of statements grouped together to do some specific task. In series of learning C programming, we already used many functions unknowingly. Functions such as – printf() , scanf() , sqrt() , pow() or the most important the main() function. Every C program has at least one function i.e. the main() function.
Function provides modularity to our program. Dividing a program in different modules makes it easy to maintain, debug and understand the code.
Functions in C programming is classified in two categories i.e. library functions and user defined functions . In this exercise we will focus on user defined functions and learn to write our own functions.
Feel free to drop your queries and suggestions below in the comments section . I would try my best to help asap.
Required knowledge
Basic C programming , Functions , Returning value from function , Recursion
List of function and recursion programming exercises
- Write a C program to find cube of any number using function .
- Write a C program to find diameter, circumference and area of circle using functions .
- Write a C program to find maximum and minimum between two numbers using functions .
- Write a C program to check whether a number is even or odd using functions .
- Write a C program to check whether a number is prime, Armstrong or perfect number using functions .
- Write a C program to find all prime numbers between given interval using functions .
- Write a C program to print all strong numbers between given interval using functions .
- Write a C program to print all Armstrong numbers between given interval using functions .
- Write a C program to print all perfect numbers between given interval using functions .
- Write a C program to find power of any number using recursion .
- Write a C program to print all natural numbers between 1 to n using recursion .
- Write a C program to print all even or odd numbers in given range using recursion .
- Write a C program to find sum of all natural numbers between 1 to n using recursion .
- Write a C program to find sum of all even or odd numbers in given range using recursion .
- Write a C program to find reverse of any number using recursion .
- Write a C program to check whether a number is palindrome or not using recursion .
- Write a C program to find sum of digits of a given number using recursion .
- Write a C program to find factorial of any number using recursion .
- Write a C program to generate nth Fibonacci term using recursion .
- Write a C program to find GCD (HCF) of two numbers using recursion .
- Write a C program to find LCM of two numbers using recursion .
- Write a C program to display all array elements using recursion .
- Write a C program to find sum of elements of array using recursion .
- Write a C program to find maximum and minimum elements in array using recursion .
C Programming Questions and Answers PDF | C Language
Here is the list of the top 500 C Programming Questions and Answers. Download C Programming Questions PDF free with Solutions. All solutions are in C language. All the solutions have 4 basic parts programming problems, logic & explanation of code, programming solutions code, and the output of the program.
To summarize our programming questions list does not contain only answers In addition, it also contains all the aspects of the problem, if any programming questions have multiple solutions then we try to add all possible solutions to the problem programming problem.
We also divide all the c programming questions into multiple levels. Levels are nothing but the complexity and toughness of programming questions. We have 5 levels, Newbie, Easy, Medium, Master, and Legendary . Therefore all the c programming questions are also separated into 14 categories. Download C Programming Questions and Answers PDF.
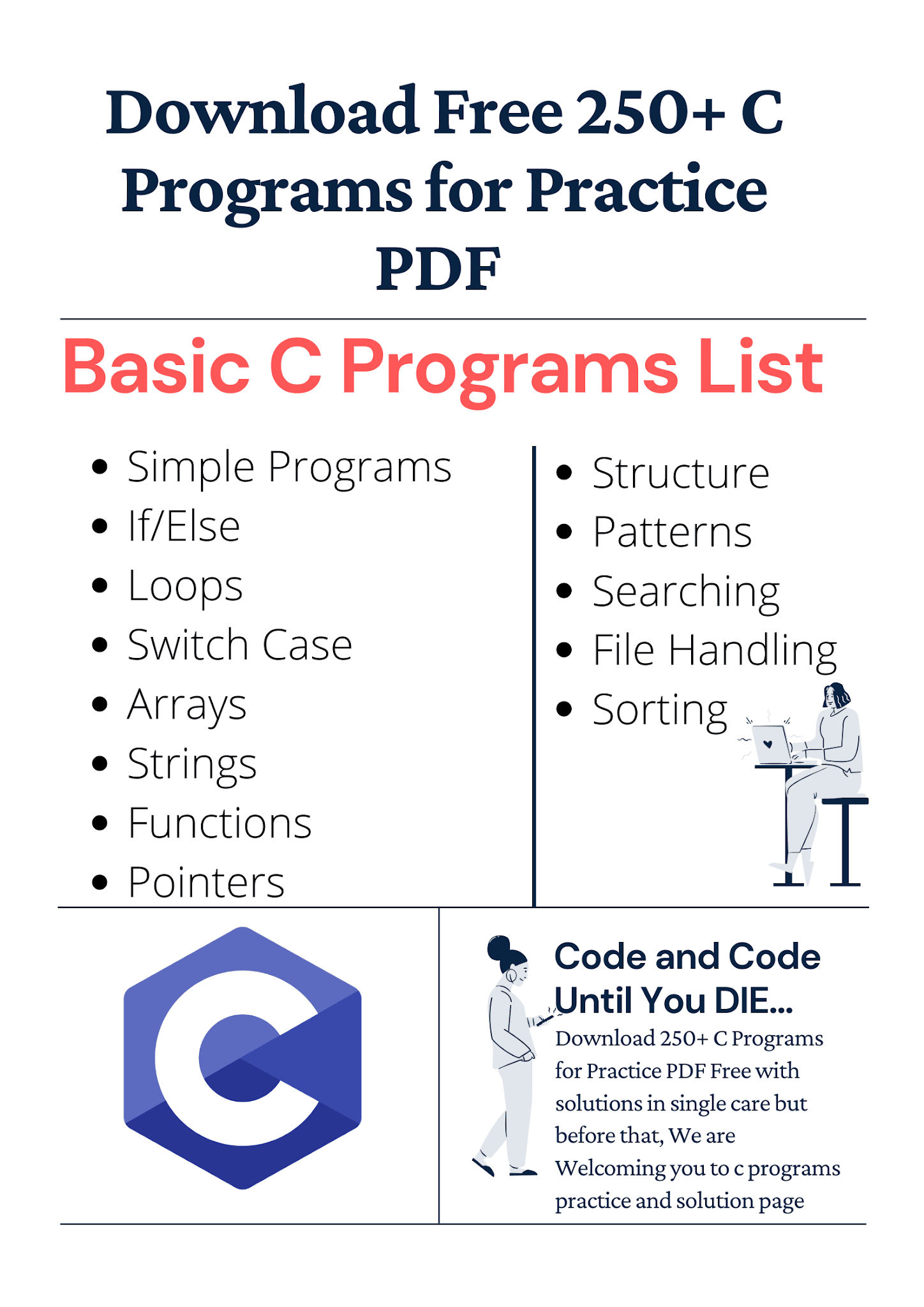
Table of Contents
Below is the List of C Programming Questions for Practice.
List of C Programming Questions and Answers by Categories.
- If / Else Statement
- While loop or While-Do loop
- Do-While loop
- Switch Case
- File Handling
- Bubble Sort
- Bucket or Radix Sort
- Selection Sort
- Insertion Sort
- Binary Search
- Linear Search
- Recursive Binary Search
- Number Series
- StartPattern Printing
Simple C Programming Questions and Answers List
- Area and Circumference of a Circle
- Print Ascii Value of the Character
- Area of Triangle
- Convert a Person’s Name into an Abbreviated
- Simple Interest
- Gross Salary of an Employee
- Percentage of 5 Subjects
- Converting Temperature Celsius into Fahrenheit
- The Display Size of the Different Datatype
- Factorial of a Given Number
- Read Integer (N) and Print the First Three Powers (N^1, N^2, N^3)
- Area of a Circle
- LCM of Two Numbers
- GCD of Two Numbers
If / Else Statement Questions and Answers
- The Greatest Number Among the Given Three Numbers
- The Number Is Positive or Negative
- Character Is Vowel or Consonant
- A Character Is an Alphabet or Not
- Uppercase, Lowercase, Special Character, or Digit
- The Number Is Even or Odd
- Greatest of Two Numbers
- Greatest Among Three Numbers
- The Date Is Correct or Not
- Voting Eligibility Checker
- Find the maximum between two numbers.
- Find the maximum between the three numbers.
- Check whether a number is negative, positive or zero.
- Check whether a number is divisible by 5 and 11 or not.
- Find whether a number is even or odd.
- Check whether a year is a leap year or not.
- Check whether a character is an alphabet or not.
- Input any alphabet and check whether it is a vowel or consonant.
- Input any character and check whether it is the alphabet, digit or special character.
- Check whether a character is an uppercase or lowercase alphabet.
- Input the week number and print the weekday.
- Input the month number and print the number of days in that month.
- Count the total number of notes in a given amount.
- Input the angles of a triangle and check whether the triangle is valid or not.
- Input all sides of a triangle and check whether the triangle is valid or not.
- Check whether the triangle is equilateral, isosceles or scalene.
- Find all roots of a quadratic equation.
- Calculate profit or loss.
Loops- List of C Programming Questions and Answers
While loop or while-do loop questions list.
- Reverse A given Number
- Find Number Is Armstrong Or Not
- Calculate the Sum of Natural Numbers
- Display Fibonacci Series
- Find the LCM of two Numbers
- Reverse a Number
- Check Whether a Number is A Palindrome or Not
- Count the Number of Digits of an Integer
- Find A Generic Root Of the Number
- Print A Calendar Taking Input From User Using Loop
- Number Is Divisible By 11 Using (VEDIC MATH)
- Denomination of an Amount
Do-While Loop Questions List
For loop questions list.
- Generate IP (Internet Protocol) Addresses Using
- Print Multiplication Table Using
- Sort A Float Array In Ascending And Descending Order Using
- Find the GCD of two Numbers Using
Switch Case- C Programming Questions and Answers List
- Temperature Conversion Celcius To Fahrenheit And Vice Versa
- Find The Day
- Find A Grade Of Given Marks or ( Find a Grade of Given Marks Using Switch Case )
- Find the Radius, Circumference and Volume of the Cylinder
- Remove All Vowels From A String
- Print the day of the week name using a switch case.
- Print the total number of days in a month using a switch case.
- Check whether an alphabet is a vowel or consonant using a switch case.
- Find the maximum between two numbers using a switch case.
- Check whether a number is even or odd using a switch case.
- Check whether a number is positive, negative or zero using a switch case.
- Find the roots of a quadratic equation using a switch case.
- Create a Simple Calculator using a switch case.
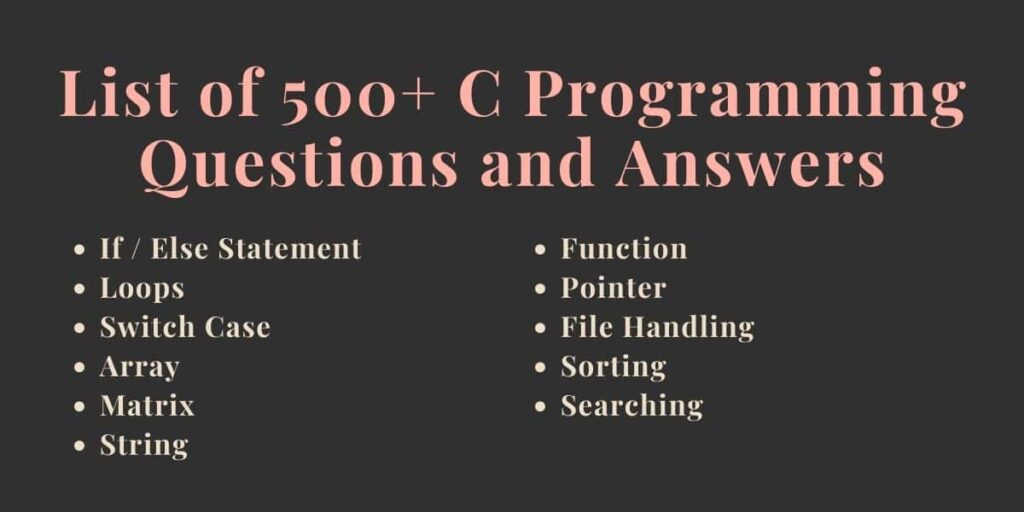
Array Questions List
- Insert An Element Desired or Specific Position In An Array
- Remove Duplicates Items In An Array
- Delete Element From Array At Desired Or Specific Position
- Print “I AM IDIOT” Instead Of Your Name Using Array
- Check String Is Palindrome Or Not Using For Loop
- Convert All Input String Simultaneously Into Asterisk ( * )
- Read and print elements of the array. – using recursion.
- Print all negative elements in an array.
- The sum of all array elements. – using recursion.
- Find a maximum and minimum element in an array. – using recursion.
- Get the second largest element in an array.
- Count the total number of even and odd elements in an array.
- Count the total number of negative elements in an array.
- Copy all elements from an array to another array.
- Insert an element in an array.
- Delete an element from an array at the specified position.
- Count the frequency of each element in an array.
- Print all unique elements in the array.
- Count the total number of duplicate elements in an array.
- Delete all duplicate elements from an array.
- Merge two arrays to the third array.
- Find the reverse of an array.
- Put even and odd elements of an array in two separate arrays.
- Search an element in an array.
- Sort array elements in ascending or descending order.
- Sort even and odd elements of the array separately.
- Left rotate an array.
- Right-rotate an array.
Matrix Questions- Download C Programming Questions and Answers
- Add two matrices.
- Subtract two matrices.
- Perform scalar matrix multiplication.
- Multiply two matrices.
- Check whether the two matrices are equal or not.
- SUM of the main diagonal elements of a matrix.
- Find the sum of minor diagonal elements of a matrix.
- Find the sum of each row and column of a matrix.
- Interchange diagonals of a matrix.
- The upper triangular matrix.
- Find a lower triangular matrix.
- SUM of the upper triangular matrix.
- Find the sum of a lower triangular matrix.
- The transpose of a matrix.
- Find the determinant of a matrix.
- Identity matrix in C.
- Check the sparse matrix.
- Check the symmetric matrix.
- Recommended posts
String Questions List
String C Programming Questions and Answers.
- String Char-Case Change
- A String is Palindrome or Not
- A String Is an Anagram or Not
- Find the length of a string.
- Copy one string to another string.
- Concatenate two strings.
- Compare two strings.
- Convert lowercase string to uppercase.
- Convert uppercase string to lowercase.
- Toggle the case of each character of a string.
- Find the total number of alphabets, digits or special characters in a string.
- Count the total number of vowels and consonants in a string.
- Count the total number of words in a string.
- Find the reverse of a string.
- Check whether a string is a palindrome or not.
- Reverse the order of words in a given string.
String Questions List: Level Up
- Find the first occurrence of a character in a given string.
- Find the last occurrence of a character in a given string.
- Search all occurrences of a character in a given string.
- Count occurrences of a character in a given string.
- Find the highest frequency character in a string.
- Find the lowest frequency character in a string.
- Count the frequency of each character in a string.
- Remove the first occurrence of a character from a string.
- Remove the last occurrence of a character from a string.
- Delete all occurrences of a character from a string.
- Remove all repeated characters from a given string.
- Replace the first occurrence of a character with another in a string.
- Replace the last occurrence of a character with another in a string.
- Put all occurrences of a character with another in a string.
- Find the first occurrence of a word in a given string.
- Find the last occurrence of a word in a given string.
- Search all occurrences of a word in a given string.
- Count occurrences of a word in a given string.
- Remove the first occurrence of a word from a string.
- Remove the last occurrence of a word in a given string.
- Delete all occurrences of a word in a given string.
- A Trim leading white space characters from a given string.
- Trim trailing white space characters from a given string.
- Trim both leading and trailing white space characters from a given string.
- Remove all extra blank spaces from the given string.
Function Questions List
- Cube of any number using the function.
- Find the diameter, circumference and area of a circle using functions.
- Maximum and minimum between two numbers using functions.
- Check whether a number is even or odd using functions.
- Check whether a number is a prime, Armstrong or perfect number using functions.
- Find all prime numbers between the given interval using functions.
- Print all strong numbers between the given interval using functions.
- Armstrong numbers between the given interval using functions.
- Print all perfect numbers between the given interval using functions.
- Find the power of any number using recursion.
- Print all natural numbers between 1 to n using recursion.
- Print all even or odd numbers in a given range using recursion.
- The sum of all natural numbers between 1 to n using recursion.
- Find the sum of all even or odd numbers in a given range using recursion.
- Find the reverse of any number using recursion.
- Check whether a number is a palindrome or not using recursion.
- Find the sum of digits of a given number using recursion.
- Find the factorial of any number using recursion.
- Generate nth Fibonacci term using recursion.
- Find the GCD (HCF) of two numbers using recursion.
- Find the LCM of two numbers using recursion.
- Display all array elements using recursion.
- Find the sum of elements of the array using recursion.
- Find maximum and minimum elements in an array using recursion.
- Stricmp() Function (Case In-Sensitive Compare)
- strncat() Function (String Concatenate)
- Strstr() Function (Sub-String)
- Strlwr() Function (To Lower-Case)
- Strupr() Function (To Upper-Case)
- Strncmp() Compare & Chars
- strncpy() Copy N Chars
- Strrev() String Reverse
- Strlen() String Length
- Strcat() String Concatenate
- Strcmp() String Compare
- Strcpy() Copy the String
Pointer Questions List in C Language
- Add two numbers using pointers.
- Swap two numbers using pointers.
- Input and print array elements using a pointer.
- Copy one array to another using pointer.
- Swap two arrays using pointers.
- Reverse an array using pointers.
- Search an element in an array using pointers.
- Access two-dimensional array using pointers.
- Add two matrices using pointers.
- Multiply two matrices using pointers.
- Find the length of the string using pointers.
- In short How to Copy one string to another using pointer.
- Concatenate two strings using pointers.
- Compare two strings using pointers.
- Find the reverse of a string using pointers.
- Sort array using pointers.
- Return multiple values from a function using pointers.
File Handling- Programming Questions and Answers in C Language
- Create a file and write contents, save and close the file.
- Read file contents and display them on the console.
- Read numbers from a file and write even, odd and prime numbers to separate files.
- Append content to a file.
- Compare two files.
- How to Copy contents from one file to another file.
- Merge two files into the third file.
- Count characters, words and lines in a text file.
- Delete a word from a text file.
- Remove the specific line from a text file.
- Remove empty lines from a text file.
- Find the occurrence of a word in a text file.
- Count occurrences of a word in a text file.
- Count occurrences of all words in a text file.
- Find and replace a word in a text file.
- Replace a specific line in a text file.
- Print the source code of the same program.
- Convert uppercase to the lowercase character and vice versa in a text file.
- Find properties of a file using the stat() function.
- Check if a file or directory exists.
- Rename a file using rename() function.
- List all files and sub-directories recursively.
Sorting in C Language
- Bubble Sort in C
- Bucket or Radix Sort in C
- Shell Sort in C
- Merge Sort in C
- Heap Sort in C
- Selection Sort in C
- Insertion Sort in C
Searching in C Language
- Binary Search in C
- Linear Search in C
- Recursive Binary Search in C
Tricky Questions for Expert Only | Legendary level
This is a high-level section for legendary programmers or thinkers, this section can help you to become a pro programmer. In this section, two categories are the number pattern and the start pattern. I just keep the pattern programming and number programming separate. All the tricky questions or we can say that number programming or start pattern programming solutions of c programming questions and answers are below.
- Number Series- Questions and Answers
- Start Pattern Printing- C Programming Questions and Answers
- Puzzles Questions
Puzzles Questions list
- Print numbers from 1 to N without using a semicolon.
- The sum of two numbers without using any operator
- How to show the memory representation of c variables?
- Condition to print “HelloWorld”
- Modify/add only one character and print ‘*’ exactly 20 times
- Sum the digits of a given number in a single statement.
Hacker Rank 30 Days of Code Solution.
99+ C Programming Questions and Answers PDF Check Here .

- Data Structure
- Coding Problems
- C Interview Programs
- C++ Aptitude
- Java Aptitude
- C# Aptitude
- PHP Aptitude
- Linux Aptitude
- DBMS Aptitude
- Networking Aptitude
- AI Aptitude
- MIS Executive
- Web Technologie MCQs
- CS Subjects MCQs
- Databases MCQs
- Programming MCQs
- Testing Software MCQs
- Digital Mktg Subjects MCQs
- Cloud Computing S/W MCQs
- Engineering Subjects MCQs
- Commerce MCQs
- More MCQs...
- Machine Learning/AI
- Operating System
- Computer Network
- Software Engineering
- Discrete Mathematics
- Digital Electronics
- Data Mining
- Embedded Systems
- Cryptography
- CS Fundamental
- More Tutorials...
- Tech Articles
- Code Examples
- Programmer's Calculator
- XML Sitemap Generator
- Tools & Generators
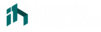
Home » C programming language
C Programs with Solutions
This section contains popular C programs with solution. Learn and practice these programs to test and enhance your C skills. Last updated : April 01, 2023
The best way to learn C programming is by practicing and solving the C programs (C problems). We have 1000+ C programs with solutions which are categorized below. Practice these C programs to learn and enhance your C problem-solving skills.
List of C programs
Practice the C programs based on the categories, library functions, advanced, top searched, and latest.
C programs by categories
- C Basic and Conditional Programs 90
- C switch case programs 06
- C 'goto' programs 10
- Bitwise related Programs 32
- Looping (for, while, do while) Programs 18
- C String Manipulation programs 10
- C String programs 50
- String User Define Functions Programs 11
- Recursion Programs 13
- Number (Digits Manipulation) Programs 10
- Number System Conversion Programs 15
- Star/Pyramid Programs 17
- Sum of Series Programs (set 1) 05
- Sum of Series Programs (set 2) 13
- Pattern printing programs 01
- User Define Function Programs (1) 05
- User Define Function Programs (2) 13
- One Dimensional Array Programs 58
- Two Dimensional Array (Matrix) Programs 21
- File Handling Programs 32
- Structure & Union Programs 12
- Pointer Programs 13
- Dynamic Memory Allocation Programs 05
- Command Line Arguments Programs 06
- Common C program Errors 22
- C scanf() programs 11
- C preprocessor programs 24
- C typedef programs 03
- C SQLite programs 11
- C MySQL programs 09
- Misc Problems & Solutions 05
C programs on standard library functions
- ctype.h Library Functions (Set 1)
- ctype.h Library Functions (Set 2)
- string.h Library Functions
- conio.h Library Functions
- dos.h Library Functions
- math.h Library Functions
- graphics.h Library Functions
- assert.h Library Functions
- stdio.h Library Functions
Advance C programs
- C program to create your own header file/ Create your your own header file in C
- gotoxy(),clrscr(),getch(),getche() for GCC, Linux.
- fork() function explanation and examples in Linux C
- C program to print character without using format specifiers.
- C program to find Binary Addition and Binary Subtraction.
- C program to print weekday of given date.
- C program to format/extract ip address octets
- C program to check given string is a valid IPv4 address or not.
- C program to extract bytes from an integer (Hexadecimal) value
- C program to store date in an integer variable
Top searched C programs
Here is the list of most important/useful programs searched on the web .
Top visited programs on IncludeHelp
- Pattern Programs in C
- C program to design calculator with basic operations using switch
- C program to find factorial of a number
- C program to check whether number is Perfect Square or not
- C program to find SUM and AVERAGE of two numbers
- C program to convert temperature from Fahrenheit to Celsius and Celsius to Fahrenheit
- C program to read and print an employee's detail using structure
- Dynamic Memory Allocation programs
- C program to convert number from Decimal to Binary
- C program to check whether number is Palindrome or not
Top searched programs on the web
- First C program to print "Hello World".
- C program to find factorial of a number.
- C program to swap two numbers without using third variable.
- C program to check whether a number if Armstrong or not.
- C program to check whether a number if Even or Odd.
- C program to print all leap years from 1 to N.
- C program to calculate employee gross salary.
- C Program to print tables of numbers from 1 to 20.
- C program to print star/pyramid series.
- C program to convert temperature from Celsius to Fahrenheit and vice versa.
- C program to convert number from Decimal to Binary.
- C program to convert number from Binary to Decimal.
- C program to print ASCII Table.
- C program to get and set current system date and time.
- C program to run dos command.
Latest C programs
- C program to generate random numbers within a range
- C program to compare strings using strcmp() function
- Interchange the two adjacent nodes in a given circular linked list | C program
- Find the largest element in a doubly linked list | C program
- Convert a given singly linked list to a circular list | C program
- Implement Circular Doubly Linked List | C program
- Print the Alternate Nodes in a Linked List without using Recursion
- Print the Alternate Nodes in a Linked List using Recursion
- Find the length of a linked list without using recursion
- Find the length of a linked list using recursion
- Count the number of occurrences of an element in a linked list without using recursion
- Count the number of occurrences of an element in a linked list using recursion
- C program to convert a Binary Tree into a Singly Linked List by Traversing Level by Level
- C program to Check if nth Bit in a 32-bit Integer is set or not
- C program to swap two Integers using Bitwise Operators
- C program to replace bit in an integer at a specified position from another integer
- C program to find odd or even number using bitmasking
- C program to check whether a given number is palindrome or not using Bitwise Operator
- C program to count number of bits set to 1 in an Integer
- C program to check if all the bits of a given integer is one (1)
- C program to find the Highest Bit Set for any given Integer
- C program to Count the Number of Trailing Zeroes in an Integer
- C Program to find the Biggest Number in an Array of Numbers using Recursion
- C program to accept Sorted Array and do Search using Binary Search
- C Program to Cyclically Permute the Elements of an Array
- C program to find two smallest elements in a one dimensional array
- Write your own memset() function in C
- memset() function in C with Example
- Write your own memcpy() function in C
- memcpy() function in C with Example
Comments and Discussions!
Load comments ↻
- Marketing MCQs
- Blockchain MCQs
- Artificial Intelligence MCQs
- Data Analytics & Visualization MCQs
- Python MCQs
- C++ Programs
- Python Programs
- Java Programs
- D.S. Programs
- Golang Programs
- C# Programs
- JavaScript Examples
- jQuery Examples
- CSS Examples
- C++ Tutorial
- Python Tutorial
- ML/AI Tutorial
- MIS Tutorial
- Software Engineering Tutorial
- Scala Tutorial
- Privacy policy
- Certificates
- Content Writers of the Month
Copyright © 2024 www.includehelp.com. All rights reserved.
C++ Solved programs, problems/Examples with solutions
C++ solved programs, problems with solutions.
C++ Solved programs —-> C++ is a powerful general-purpose programming language. It is fast, portable and available in all platforms.
This page contains the C++ solved programs/examples with solutions, here we are providing most important programs on each topic . These C examples cover a wide range of programming areas in Computer Science.
Every example program includes the description of the program, C++ code as well as output of the program. All examples are compiled and tested on a Windows system.
These examples can be as simple and basic as “Hello World” program to extremely tough and advanced C++ programs. Here is the List of C++ solved programs/examples with solutions (category wise) and detailed explanation.
C++ Solved Programs by categories…….
C++ basic solved programs.
- C++ Program to Print Number (Entered by the User)
- C++ Program to Addition of Two Numbers
- C++ Program to Find Quotient and Remainder
- C++ Program to Swap Two Numbers Without Using third variable
- C++ Program to Find Size of int, float, double and char
- C++ Program to Multiply two Numbers
- C++ Program to Find ASCII Value of a Character
- C++ program to generate random numbers
- C++ Program to calculate sum and average of three numbers
- C++ Program to convert inches into yard, feet and inches
- C++ Program to raise any number X to power N
- C++ Program to Add Two Numbers
- C++ Program to Convert Days Into Years, Weeks and Days
- C++ Program to find Square Root of a number
- C++ Program to find Compound Interest
- C++ program to find cube of number using macros
- C++ Program to Check Number is Odd or Even
- C++ Program to Check Character is Uppercase, Lowercase, Digit or Special Character
- C++ Program to Check whether year is Leap year or not
- C++ Program to Find All Roots of a Quadratic Equation
- C++ Program to Check Whether a character is Vowel or Consonant
- C++ Program to Check given number is Even or Odd
- C++ Program to Check given number is Prime number or not
- C++ Program to find Factorial of a Number
- C++ program to Print Table of any Number
- C++ Program to Reverse a Number
- C++ Program to Find number of Digits in any number
- C++ Program to find Fibonacci Series
- C++ Program to Check given number is Armstrong or Not
- C++ program to Find Largest Number among three numbers
- C++ program to Check Number is Palindrome or not
- C++ Program to Find HCF of two numbers
- C++ program to find LCM of two numbers
- C++ program to find Square Root of a Number
- C++ Program to find Cube Root of Number
- C++ program to find sum of digits of a number
- C++ Program to Find Power of Number
- C++ Program to find average of numbers
- C++ Program for Pascal Triangle
C++ Number Solved Programs
- C++ Program to Check Number is Unique Number or Not
- C++ Program to Find Sum of First n Natural Numbers
- C++ Program to Find Divisors of a Number
- C++ Program to Find Sum of Square of n Natural Numbers
- C++ Program to Convert Binary to Decimal
- C++ Program to Convert Decimal to Binary
- C++ Program to Display Prime Numbers Between Two Intervals
- C++ Program to Convert Binary Number to Octal
- C++ Program to Convert Octal to Binary
- C++ Program to Convert Octal Number to Decimal
- C++ Program to Convert Decimal Number to Octal
- C++ Program to Make a Simple Calculator
- C++ program to find area of circle
- C++ Program to find Area and Perimeter of Rectangle
- C++ Program to find Perfect Number
- C++ Program to Calculate Grade of Student
- C++ Program to Calculate Arithmetic Mean
- C++ Program to Calculate Average Percentage Marks
C++ String Solved Programs
- C++ Program to Print entered String
- C++ Program to Find Frequency of Characters in String
- C++ program to Find Length of String
- C++ Program to Compare Two Strings
- C++ Program to Reverse String
- C++ program to concatenate two string
- C++ Program to Copy String into Another String
- C++ Program to Find Number of Vowels, Consonants, Digits, Spaces in String
- C++ Program to Remove Characters in String Except Alphabets
- C++ Program to Sort Elements in Lexicographical Order
- C++ Program to Swap Two Strings
- C++ Program to Convert Lowercase to Uppercase
- C++ Program to Convert Uppercase Character to Lowercase
- C++ Program to Convert Uppercase String to Lowercase
- C++ Program to Remove Spaces from String
- C++ Program to Count Words in String
- C++ Program to Delete Words from String
- C++ Program to Delete Vowels from String
- C++ Program to Find Substring in String
- C++ Program for Permutation of String
- C++ Program to reverse all strings stored in an array
- C++ Program to Convert first alphabet from lowercase to uppercase
- C++ Program to check whether String is Palindrome or not
- C++ Program to convert first letter of each word to uppercase and other to lowercase
C++ Arrays Solved Programs
- C++ Program to find Maximum or Largest number in array
- C++ Program to enter 5 numbers & display first and last only
- C++ Program To reverse an array elements entered by user
- C++ Program to Sort an Array Elements in Ascending Order
- C++ Program to Sort an Array Elements in Descending Order
- C++ Program for Addition of two matrix
- C++ Program to Find Duplicate Elements in Array
- C++ Program to Find Sum of Elements of an Array
- C++ Program to Reverse elements of an Array
- C++ Program to Find Even & Odd Elements in Array
- C++ Program to Delete element from Array
- C++ program to Insert element at specific position in array
- C++ Program to Pass Array in Function
- C++ Program for Three Dimensional (3D) Array
- C++ Program for Two Dimensional Array
- C++ Program to Delete Element from Array
- C++ Program to Find Smallest Element in Array
- C++ Program to Find Largest Element in Array
- C++Program for One Dimensional (1-D) Array
- C++ Program to Calculate Average of elements in an Arrays
- C++ Program to Access Elements of an Array Using Pointer
- C++ Program to Multiply Two Matrix Using Multi-dimensional Arrays
- C++ Program to Find Transpose of a Matrix
- C++ Program to Subtract Two Matrices
- C++ Program for Quick Sort using arrays
- C++ Program for Shell Sort using Arrays
- C++ Program for Union of Two Sorted Arrays
- C++ Program for Union of Two Unsorted Arrays
- C++ Program for Insertion Sort using Array
- C++ Program for Selection Sort using Arrays
- C++ Program to Find Largest and Smallest Element of a Matrix
- C++ Program for Binary Search in Arrays
- C++ Program for Linear Search in Arrays
- C++ Program to Find Sum of Diagonals of Matrix
- C++ Program to Print Lowerhalf and Upperhalf of Triangle Matrix
- C++ Program to Find Sum Above and Below of Main Diagonal Matrix
- C++ Menu Driven Program for Queue Operations using Arrays
- C++ Menu Driven Program for Stack Operations Using Arrays
- C++ Program to implement Merge Sort using Divide and Conquer Algorithm
C++ Functions Solved Programs
- C++ Program for Linear search using recursion
- C++ Program to Find Factorial of Number Using Recursion
- C++ program to Find Sum of Natural Numbers using Recursion
- C++ program to Check Number can Express as Sum of Prime Numbers
- C++ Program to Check Prime Number
- C++ Program to Find GCD Using Recursion
- C++ Program to Find Power Using Recursion
- C++ program to Reverse String Using Recursion
- C++Program to Convert Octal to Binary
- C++ Program to Convert Binary Number to Decimal
- C++ Program to convert decimal number to binary
- C++ Program for Fibonacci Series Using Recursion
- C++ program to swap values using pass by reference
- C++ Program to print series using function: x + x^3/3! + x^5/5! +…….+ x^n/n!
- C++ Program for Addition,subtraction and multiplication using function
- C++ Program to find cube of number using function
- C++ program to find greatest between two numbers using inline function
- C++ program to find factorial by defining functions outside the class
- C++ program to find greatest b/w 3 nos. by defining the functions inside class
- C++ program to swap two characters and integers by call by value
- C++ program to find reverse of number by defining functions outside class
- C++ program to swap 2 characaters and integers by call by address
- C++ program to find sum and product of 5 numbers using inline function
- C++ program to add two time by Call by reference
- C++ program to add two time by Call by address
- C++ program to find how many times function called by objects
- C++ program to find square of float and integer using inline function
- C++ program to add two complex number passing objects as arguments
- C++ program to find factorial of number using functions
- C++ program to check number is palindrome or not using Function
- C++ Program to Swap two numbers using call by value
- C++ Program to Swap two numbers by call by address
- C++ Program to Swap two numbers by call by reference
C++ Classes and Objects Solved Programs
- C++ Program to display entered Date
- C++ program to display Student details using class
- C++ Program to find Largest among 3 numbers using classes
- C++ Program to find Sum of odd numbers between 1 and 100 using class
- C++ program to display Entered Time using class
- C++ program to find Largest among 2 numbers using class
- C++ program to Swap two numbers using class
- C++ program for various Mathematical Operations using Switch case
- C++ Program to Compare Two Strings using overloading
- C++ Program to enter student marks and roll no. using Virtual Class
- C++ Program to Print Numbers From 1 to n using class
- C++ Program to calculate Volume of Cube using constructor and destructor
- C++ Program to Perform Complex Operations using Overloading
- C++ Program to determine the Area of Rectangle using constructors
- C++ Program to show Constructor & Destructor Example
- C++ Program to Show Counter using Constructors
- C++ Program to Display Date using Constructors
- C++ program to Display Student Details using constructor and destructor
- C++ Program to enter student details by Passing parameters to constructors
- C++ Program to Show Overload Constructor Example
- C++ Program to show Example of Default copy constructor
- C++ Program to demonstrate Constructor Overloading
- C++ Program To calculate Volume of Box using Constructor
- C++ Program To Calculate Simple Interest using class
- C++ Program To Calculate Electricity Bill Of Person using Class
- C++ program to multiply every member by 10 using class
- C++ program for Simple Queue using Class
- C++ Program to implement B- Trees using Linked Lists
C++ Constructor and Destructor Solved Programs
- C++ Program to Show an Example of Destructor
- C++ Program to Show an Example demonstrating Order of constructor invocation
- C++ Program For Constructor with Two Parameters
- C++ Program to illustrates the use of Constructors in single inheritance
- C++ Program to illustrates the use of Constructors in multilevel inheritance
C++ Inheritance Solved Programs
- C++ Program to access protected data member using Inheritance
- C++ Program for Inheritance Beyond Single Level
- C++ Program for enter Patient details using Inheritance
- C++ program to demonstrate an example of Single Inheritance
- C++ Program to demonstrate an Example of Multiple Inheritance
- C++ Program to demonstrate an Example of Hybrid Inheritance
- C++ program display student marksheet using multiple inheritance
- C++ Program to find Area of Rectangle using inheritance
- C++ Program to show access to private,public and protected using Inheritance
- C++ Program to Overriding the member functions using Inheritance
- C++ Program to enter Student details of different Stream using Hierarchical Inheritance
- C++ Program to find area and volume using multiple inheritance
- C++ Program to demonstrate an Example of Multilevel Inheritance
- C++ Program to illustrates the use of Constructors in multiple inheritance
- C++ Program of templated class derived from non-templated class
- C++ Program of non-templated class derived from templated base class
- C++ Program of templated class derived from another templated class
C++ Overloading Solved Programs
- C++ program to Swap variables using function overloading
- C++ program for show Counter using Overloading unary operator ++
- C++ program to perform operations on complex numbers
- C++ class Program to perform rational number arithmetic
- C++ class Program to perform Complex Arithmetic using operator overloading
- C++ Program to Find the Area of shapes using function overloading
- C++ Program to check Palindrome using function overloading
- C++ program to find area of square,rectangle,circle and triangle
- C++ program to find volume of cube, cylinder, sphere by function overloading
C++ Polymorphism Solved Programs
- C++ Program to demonstrate Run time polymorphism
- C++ Program to illustrate the use of pure virtual function in Polymorphism
- C++ Program to define an Abstract Class in Polymorphism
- C++ Program to illustrates the use of Virtual base class
- C++ Program to show an Example of Pointers to base class
- C++ Program to illustrate Abstract Base Class
- C++ Program to illustrate an example of Pure Virtual functions
- C++ Program to maintain employee database using virtual class
C++ File Handling Solved Programs
- C++ Program to Maintain Book Record using File Handling
- C++ Program to Maintain House Records using File Handling
- C++ Program for Registration(Signup) process using File Handling
- C++ Program to Read and Write File Operation in File Handling
- C++ Menu Driven Program to perform Add,Modify,Append and Display
- C++ Program to Store or Enter Data to file using File Handling
- C++ Program to Retrieve information from the file using File Handling
- C++ Program to Read and Display file using File Handling
- C++ Program to Merge Two Files to Third File using File Handling
- C++ Program to Encrypt Files using File Handling
- C++ Program to Decrypt Files using File Handling
- C++ program to read and write values through object using File Handling
- C++ Program to Count Digits, Alphabates & Spaces using File Handling
- C++ Program to Count Words, Lines and Total Size using File Handling
- C++ Program to read text file and write in another text file in File Handling
- C++ Program to Count Occurrence of Word using File Handling
- C++ Program to Read Write Student Details using File Handling
- C++ Program of Manipulation of file pointers in File Handling
C++ Template Solved Programs
- C++ Program to find Largest among two numbers using function template
- C++ Program to Swap data using function template
- C++ Program to build Simple calculator using Class template
- C++ Program to demonstrate an Example of Template specialization
- C++ Program to demonstrate an Example of Non-type parameters for templates
- C++ Program to implement Generic methods on Stack using Class Template
- C++ Program to implement push & pop methods from stack using template
- C++ Program to Add two numbers using function template
- C++ program to find Sum of numbers using Overload template function
- C++ Program to Perform Simple Addition Function Using Templates
- C++ Program to find sum of Array using function template
- C++ Program to find Square function of each data-type using single template
- C++ Program of square function using template specialization
- C++ Program to show Example of Static member variable of template class
- C++ Menu Driven Program for Stack using Templates
- C++ program to implement Hash Table using Template Class
If you found any error or any queries related to the above programs or any questions or reviews , you wanna to ask from us ,you may Contact Us through our contact Page or you can also comment below in the comment section.We will try our best to reach up to you in short interval.
Thanks for reading the post….
No related posts.
You are tasked by Skontiri Delivery Company, to develop a system to calculate the delivery cost for their clients. Clients are charged per pounds in weight based on the delivery area code. A Provincial delivery code is 1, a National delivery code is 2 and an International delivery code is 3. The fee for the delivery is as follows: For each delivery, enter the delivery number (use as sentinel), weight and specify if the delivery is insured or not. Before you calculate the Price confirm if a valid area code is entered. Price is calculated per pounds based on the … Read more »
Hi please i am help me soultion plz
#include <iostream> #include <string> double calculatePrice(int areaCode, double weight) { double feePerPound; switch (areaCode) { case 1: feePerPound = 1.5; break; case 2: feePerPound = 2.5; break; case 3: feePerPound = 4.0; break; default: std::cout << “Invalid area code” << std::endl; return 0.0; } return feePerPound * weight; } double calculateTax(double price) { const double taxRate = 0.1; return price * taxRate; } double calculateInsurance(double price, bool insured) { const double insuranceRate = 0.05; if (insured) { return price * insuranceRate; } return 0.0; } int main() { std::string areaName; int deliveryNumber, areaCode; double weight, price, tax, insurance, total, grandTotal = 0.0; … Read more »
cout <<“Machine is working: “<< machineisworking;
#include <iostream> #include <string> #include<math.h> using namespace std; int main() { int price=10,total,tax=1.088,weight,area_code,deliveryno; cout<<“\n DELIVERY CALCULATOR:”; cout<<“\n enter the delivery number”; cin>>deliveryno; cout<<“\n enter the weight of the package”; cin>>weight; cout<<“\n Enter the area code from below:\n Provincial delivery code is 1\n a National delivery code is 2\n and an International delivery code is 3.\n”; cin>>area_code; switch(area_code) { case 1: total=weight*price*tax*1; break; case 2: total=weight*price*tax*2; break; case 3: total=weight*price*tax*3; break; } cout<<“\n the delivery fee is:”<<total; return 0; }
# include < iostream > //#include <string> //#include<math.h> using namespace std ; int main () { int price = 10 ; int tax = 2 ; int total , weight , area_code , deliveryno ; cout << “ DELIVERY CALCULATOR: “; cout << “ enter the delivery number “ <<endl ; cin >> deliveryno ; cout << “ enter the weight of the package “ <<endl ; cin >> weight ; cout << “ Enter the area code from below: Provincial delivery code is 1 a National delivery code is 2 and an International delivery code is 3. “ <<endl ; cin >> area_code ; switch ( area_code ) { case 1 : total = weight * price * tax * deliveryno ; break ; case 2 : total = weight * price * tax * deliveryno ; break ; case 3 : total = weight * price * tax * deliveryno ; break ; } cout << “ the delivery fee is: “ << total ; return 0 ; }
Write a program that prompts the user to enter two integers.
The program outputs how many numbers are multiples of 3 and how many numbers are multiples of 5 between the two integers (inclusive)
#include using namespace std; int main() { int a,b,i,count1=0,count2=0;
cout< first integer): “<>a>>b;
for(i=a;i<=b;i++){ if(i%3==0){ ++count1; } }
for(i=a;i<=b;i++){ if(i%5==0){ ++count2; } }
cout<<"No. of multiples of 3: "<<count1<<endl; cout<<"No. of multiples of 5: "<<count2;
These programs are wonderful! But, the most important thing is missing is the comments! I am not saying that there should be comment for every line of code but where ever it need so. I am sure without comments, it’s not useful for students nor for anyone novice to programming! That’s why this site has less viewers. Still, it is something that can be revised anytime.
marks in maths >= 70 marks in physics >= 65 marks in chemistry >= 60 Total marks in all three subjects >= 180
Make a function bool Is_Digit (char n) which returns true,..if the character is between character 0 to 9 else, it returns false using inline function. plz help
Write a program that creates and then reads a matrix of 4 rows and 4 columns of type int. while reading; the program should not accept values greater than 100. For any entered value greater than 100, the program should ask for input repeatedly. After reading all numbers, the system should find the largest number in the matrix and its location or index values. The program should print the largest number and its location (row and column).
write a programme which stores different tax record of a company
There is a garage where the access road can accommodate any number of trucks at one time. The garage is build such a way that only the last truck entered can be moved out. Each of the trucks is identified by a positive integer (a truck_id). Write a program to handle truck moves, allowing for the following commands: a) On_road (truck_id) b) Enter_garage (truck_ id) c) Exit_garage (truck_id) d) Show_trucks (garage ) e) Show_trucks(road) f) user menu for function calling
Good day everyone, please i would like to know how to write a nested if program following a step by step process; payment of fees admission letter online registration identification card an allocated hall of residence Please i will need all the help i can get, i am a computer science student and i am having little problems with this… please can i get the information sent to my provided gmail account. THANK YOU SO MUCH FOR YOUR FURTHER ASSISTANCE IT NEEDS TO BE SUBMITTED IN LESS THAN 12 HOURS ALL THE HELP I WILL GET WOULD BE NEEDED,
You are given a sequence a1, a2, …, aN . Find the smallest possible value of ai + aj , where 1 ≤ i < j ≤ N . Input The first line of each description consists of a single integer N . The second line of each description contains N space separated integers – a1, a2, …, aN respectively. Output A single line containing a single integer (the smallest possible sum). Sample Input1: Sample Input2: 4 5 1 3 4 10 9 2 7 4 5 6 3 8 1 10 Sample Output2: Sample Output2: 4 3
Write a C++ program to calculate the tuition fee for a student at a University. The program should prompt for and accept the idnumber and the total number of credits for which he/she has enrolled. The bill outputted should contain the idnumber and tuition fee. Calculate the tuition fee as follows: • Total credits of 15 or more indicates that the student is full-time. Full-time students pay a flat rate of $35,000 for tuition. • Total credits of less than 15 indicate that the student is part-time. Part-time students pay $850 per credit for tuition. After printing the tuition fee, … Read more »
#include<iostream> using namespace std; class Student{ public: string regNo; int noOfcredits; double tBill; string status1 = “Full-time”; string status2 = “Part-time”; string answer; }; isBool(string answer){ if(answer == “yes”){ return true; }else if(answer == “no”){ return false; } } int main() { Student stud1,stud2; stud1.regNo; cout << “Enter registration number: ” ; getline(cin, stud1.regNo); stud1.noOfcredits; cout << “Enter number of credits: ” ; cin >> stud1.noOfcredits; isBool(stud1.answer); stud1.tBill; if(stud1.noOfcredits > 15){ cout << “Status: “<< stud1.status1 ; cout << “\nTution fee: $35,000 “; cout << “\nCalculate tution fee for another student? “; cin >> stud1.answer; }else{ cout << “Status: “<< stud1.status2 … Read more »
Write a program to display only months, but with a forward/backward button to move bw the months from January to December
Welcome A recently launched attraction at the “Events Square” entertainment fair is the “Carnival of Terror” which is an interactive fun zone featuring scary, horror and Halloween stories. The Entry tickets for the show is to be printed with a Welcome message along with an additional message for Children stating they should be accompanied by an adult. Given the age of the person visiting the scary house, the ticket should carry the additional message only for Children whose age is less than 15 years. The show organizers wanted your help to accomplish this task. Write a program that … Read more »
#include<iostream>
using namespace std; class Child{ public: string name; int age; };
int main() { Child child1; cout << “Enter child’s first name: “; cin >> child1.name; cout << “Enter age: “; cin >> child1.age; if(child1.age > 15){ cout << child1.name << ” welcome to Carnival of Terror..”<< endl; }else{ cout << child1.name << ” welcome to Carnival of Terror..”<< endl; cout << “Please note that you should be accompanied by an adult..”<< endl; } return 0; }
galat program hai
. include new functions of -print a single node -print entire list search a node by, name, id, cnic, dob (function overload…one function per filter) insert at head -insert at tail
The management of Lifetime Company Limited decided to pay a bonus of 4.3% of total sales to every salesperson of the company who made total sales of GHS 5000 or more in six (6) months. Write a C++ program to accept the monthly sales of each salesperson and display whether the person deserve a bonus or not.
hello programmer
Find Second Maximum Number(using operators &&, >, <) Write a program which takes 3 input integers and tell the 2nd maximum. Sample Input : 90 5 60 Sample Output
In this program the user has to insert two integer numbers: number1 which must be between 1 and 50 inclusive (i.e. 1<= number1 <=50) and number2 which must be between 51 and 100 inclusive (i.e. 51 <= number2 <= 100). A wrong inserted value for any number causes the output message “Invalid” to be displayed on the console and the program returns. When valid numbers are entred, they are used to in a loop that applies the following equation in each iteration: k = i * i + 2 * j. In this loop, “i” represnts a series of consecutive ascending even numbers starting with … Read more »
can you provide some more programs of oop for better pratice and for concept clarification ?
This website is very helpful . I’m in love with it .
Supermarket is a retail facility with wide range of products under one roof, including fullgroceries and general merchandise. They satisfy the customers in all their routine shopping needs in one trip. Arun owns a supermarket in Chennai and he wanted to create a software that contains the purchase details of all the customers made on that day and at the end of the day, he wants to calculate the total amount received and the total number of items purchased by all the customers in a database. Help him to do this by writing a C++ program. Strictly adhere to the Object Oriented Specifications … Read more »
write c++ program to add two integer vectors together and store them in a third vector. the first two vectors should accepts user input for five elements each.
The representation of a complex number as a sum of a real and imaginary number, z = x + i y, is called its Cartesian representation. We can therefore express any complex number z = x + i y into , this representation is known polar representation of any complex number z. where and You need to create a class having name, Polar Representation with data members, magnitude and angle. Make sure you provide a default constructor too. Overload following operators of the class; 1. + operator (to add two objects of class) 2. – operator (to subtract two objects of class) 3. * operator (to multiply … Read more »
please help me

. Write a “Garage” class that has a “Car” that is having troubles with its “Motor”. Use a function-level try block in the “Garage” class constructor to catch an exception (thrown from the “Motor” class) when its “Car” object is initialized. Throw a different exception from the body of the “Garage” constructor s handler and catch it in main( ).
Write a program that asks the user to enter the number of courses he/she is taking and then enter the course code and its number of credits. The program should sum the total number of credits and display it.
Create publisher class that represents a book publisher, publisher has two properties/attributes name and a code which should be a non-negative integer. Class must have a constructor that should take parameters to set the both properties, get functions for both attributes, a set method to change name. and a print function to print/display the publisher. now create a main function make and object of publisher, print the publisher then change the publisher code and again print the publisher. your program should run like this the old publisher the new publisher
Define a class named ‘Train’ representing following members: Data members :- – Train Number – Train Name – Source – Destination – Journey Date – Capacity Member functions: – Initialise members – Input Train data – Display data Write a C++ program to test the train class.
Develop a geometry calculator that will do the following things: 1. It should give the user many options to choose between (user should right 1 if he/she wants to calculate something related to circle, 2 for rectangle and so on). Like 1. Circle 1. Area 2. Circumference 2. Rectangle 1. area 3. Triangle 1. Area 2. base 4. Parallelogram 1. Area 5. Trapezoid 1. Area
2. Each of these operations must be handled by a function 3. You should use call by value, call by reference with pointers in any of these functions
Your task is to create a program that examines the distribution grades on an exam. Ask the user to input how many students does he have followed by the grades of those students. Then, group the grades depending on the range and then display the summary in a bar graph format. The ranges are as follows: 0 – 9, 10 – 19, 20 – 29, 30 – 39, 40 – 49, 50 – 59, 60 – 69, 70 – 79, 80 – 89, 90 – 99, and 100.
A company has three types of employee: 1. Managers: receive a fixed monthly salary 2. Commission Workers: receive $1000 plus 5% of their gross monthly sales 3. Piece Workers: receive a fixed amount of money per item for each of the items they produce; each pieceworker in this company works on only one type of item. Write a program to compute the monthly pay for each employee. P.S: You do not know the number of employees in advance. Each type of employee has its own pay code: Managers have code 1 Commission Workers have code 2 Pieceworkers … Read more »
write a program 1) Declare all variables and constants paying attention to the types 2) Calculate the area of the circle given the radius 3) Divide the area of the circle by the number of segments, but only if this number is different from zero 4) Print all partial results
Overload the ‘subscript’ operator for the class ‘String’ so that it takes a character as a parameter and returns the position of its first occurrence. The output of the following code should be two. String s1(“abcd”); cout<<s1[‘c’]<<endl; Also, overload ‘+’ operator to merge two strings. Create an menu-driven program. 1. Find 2. Merge 3. Exit
plz help me
Write a program to process the quote Four Score and Seven Years Ago. Copy the quotation into a string. Create three character arrays – one for vowels, one for consonants and one for reverse order. The program will copy the vowels into the vowels array, the consonants into the consonants array and the reverse order quote into the reverse array. For example, if the string were “hello world”: • The vowels array would equal ‘e’, ‘o’, ‘o’ • The consonants array would equal ‘h’, ‘l’, ‘l’, ‘w’, ‘r’, ‘l’, ‘d’ • The reverse array would equal ‘d’, ‘l’, ‘r’, ‘o’, … Read more »
1) Prompts the user to enter from the keyboard car price and profit percentage. (Both are float). 2) Calculates the total price using car price and profit percentage. For example, if the car price is 100 BD and profit percentage is 25%, then the total price is 125 BD. 3) Display the value of car price, profit percentage, total price. Each in separate line formatted in two decimal places. Sample Input/output (Input in red color)
whats my problem

#include<iostream> using namespace std ; int main() { cout<<“Fahadvai” ; return 0 : }
help problem?
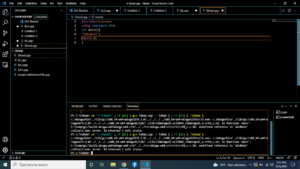
Write a program to display your name 500 times
Write a program to accept any number and check whether the number is even or odd
Using switch case statement accept any two numbers and display sum, product or difference according to the user’s choice. An appropriate message should be displayed for the wrong choice
Write a program to accept any positive integer and display its square. i.e. if the input is 5 then the output should be 25. Program will exit only if user enters less than or equals to 0
#include<iostream> using namespace std; int main() { /*Write a program to accept any positive integer and display its square. i.e. if the input is 5 then the output should be 25. Program will exit only if user enters less than or equals to 0 */
int num; cout<< “Enter any number :”; cin>>num; if( num<0) exit(0);
else cout<<num<< ” it’s square is “<<num*num<<endl;
return 0; }
Write a program to accept any number and display the sum of its factors. *
The programme below in C++ is given to you. Complete the Binnary Search function so as to work properly with a head and tail pointer and return whether value x is included in the table or not. Assume that the table already has all its elements in ascending order. // Example program #include #include using namespace std; void filltable(int *p){ for (int i=0; i<5; i++){ cout <> *p; p++; } } void printtable(int *pr){ for (int i=0; i<5; i++){ cout << *pr <<" "; pr++; } } void findx(int *p, int x){ … Read more »
Write a c++ program to perform following operations: Create a class with following Data Members and Member Functions: • Data Members : JavaMarks and CPPMarks • Member Functions: ◦ Constructor() – Create a constructor to initalize the data members ◦ Overload the binary + operator using Friend Function ◦ Display() – Display the data ◦ Call the Destructor at the end of the code
- A string (X).
- A letter (L1) .
- A letter (L2) .
Output: – The same string (X) , but modified as the following: o Find some word that start with (L1) , and end with the letter (L2) . o Copy the founded word to the last of the array but in reverse manner. Example: L1 = ‘S’ L2 = ‘L’
h i s S S C H O O L L y e s
c o m e ‘\0’
x[]= we his school yes come loohcs ‘\0’
how can i solve it
write a C++ program to find number of similar words between 5 text files and 1 source file one by one, and common phrases among the these files
i like that you have variety of questions.
hi I want code of that program
s= y/(x+1)! +y/(x+2)! +y/(x+3)! +…. y/(x+n)!

Top 100 C Programming Interview Questions and Answers (PDF)
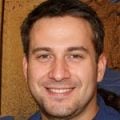
Here are C Programming interview questions and answers for fresher as well as experienced candidates to get their dream job.
Basic C Programming Interview Questions and Answers for Freshers
1) how do you construct an increment statement or decrement statement in c.
There are actually two ways you can do this. One is to use the increment operator ++ and decrement operator –. For example, the statement “x++” means to increment the value of x by 1. Likewise, the statement “x –” means to decrement the value of x by 1. Another way of writing increment statements is to use the conventional + plus sign or – minus sign. In the case of “x++”, another way to write it is “x = x +1”.
👉 Free PDF Download: C Programming Interview Questions & Answers >>
2) What is the difference between Call by Value and Call by Reference?
When using Call by Value, you are sending the value of a variable as parameter to a function, whereas Call by Reference sends the address of the variable. Also, under Call by Value, the value in the parameter is not affected by whatever operation that takes place, while in the case of Call by Reference, values can be affected by the process within the function.
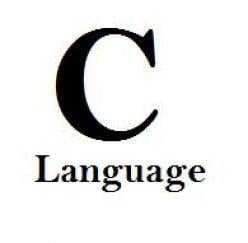
3) Some coders debug their programs by placing comment symbols on some codes instead of deleting it. How does this aid in debugging?
Placing comment symbols /* */ around a code, also referred to as “commenting out”, is a way of isolating some codes that you think maybe causing errors in the program, without deleting the code. The idea is that if the code is in fact correct, you simply remove the comment symbols and continue on. It also saves you time and effort on having to retype the codes if you have deleted it in the first place.
4) What is the equivalent code of the following statement in WHILE LOOP format?
5) what is a stack.
A stack is one form of a data structure. Data is stored in stacks using the FILO (First In Last Out) approach. At any particular instance, only the top of the stack is accessible, which means that in order to retrieve data that is stored inside the stack, those on the upper part should be extracted first. Storing data in a stack is also referred to as a PUSH, while data retrieval is referred to as a POP.
6) What is a sequential access file?
When writing programs that will store and retrieve data in a file, it is possible to designate that file into different forms. A sequential access file is such that data are saved in sequential order: one data is placed into the file after another. To access a particular data within the sequential access file, data has to be read one data at a time, until the right one is reached.
7) What is variable initialization and why is it important?
This refers to the process wherein a variable is assigned an initial value before it is used in the program. Without initialization, a variable would have an unknown value, which can lead to unpredictable outputs when used in computations or other operations.
8 What is spaghetti programming?
Spaghetti programming refers to codes that tend to get tangled and overlapped throughout the program. This unstructured approach to coding is usually attributed to lack of experience on the part of the programmer. Spaghetti programing makes a program complex and analyzing the codes difficult, and so must be avoided as much as possible.
9) Differentiate Source Codes from Object Codes
Source codes are codes that were written by the programmer. It is made up of the commands and other English-like keywords that are supposed to instruct the computer what to do. However, computers would not be able to understand source codes. Therefore, source codes are compiled using a compiler. The resulting outputs are object codes, which are in a format that can be understood by the computer processor. In C programming , source codes are saved with the file extension .C, while object codes are saved with the file extension .OBJ
10) In C programming, how do you insert quote characters (‘ and “) into the output screen?
This is a common problem for beginners because quotes are normally part of a printf statement. To insert the quote character as part of the output, use the format specifiers \’ (for single quote), and \” (for double quote).
11) What is the use of a ‘\0’ character?
It is referred to as a terminating null character, and is used primarily to show the end of a string value.
12) What is the difference between the = symbol and == symbol?
The = symbol is often used in mathematical operations. It is used to assign a value to a given variable. On the other hand, the == symbol, also known as “equal to” or “equivalent to”, is a relational operator that is used to compare two values.
13) What is the modulus operator?
The modulus operator outputs the remainder of a division. It makes use of the percentage (%) symbol. For example: 10 % 3 = 1, meaning when you divide 10 by 3, the remainder is 1.
14) What is a nested loop?
A nested loop is a loop that runs within another loop. Put it in another sense, you have an inner loop that is inside an outer loop. In this scenario, the inner loop is performed a number of times as specified by the outer loop. For each turn on the outer loop, the inner loop is first performed.
15) Which of the following operators is incorrect and why? ( >=, <=, <>, ==)
<> is incorrect. While this operator is correctly interpreted as “not equal to” in writing conditional statements, it is not the proper operator to be used in C programming . Instead, the operator != must be used to indicate “not equal to” condition.
16) Compare and contrast compilers from interpreters.
Compilers and interpreters often deal with how program codes are executed. Interpreters execute program codes one line at a time, while compilers take the program as a whole and convert it into object code, before executing it. The key difference here is that in the case of interpreters, a program may encounter syntax errors in the middle of execution, and will stop from there. On the other hand, compilers check the syntax of the entire program and will only proceed to execution when no syntax errors are found.
17) How do you declare a variable that will hold string values?
The char keyword can only hold 1 character value at a time. By creating an array of characters, you can store string values in it. Example: “char MyName[50]; ” declares a string variable named MyName that can hold a maximum of 50 characters.
18) Can the curly brackets { } be used to enclose a single line of code?
While curly brackets are mainly used to group several lines of codes, it will still work without error if you used it for a single line. Some programmers prefer this method as a way of organizing codes to make it look clearer, especially in conditional statements.
19) What are header files and what are its uses in C programming?
Header files are also known as library files. They contain two essential things: the definitions and prototypes of functions being used in a program. Simply put, commands that you use in C programming are actually functions that are defined from within each header files. Each header file contains a set of functions. For example: stdio.h is a header file that contains definition and prototypes of commands like printf and scanf.
20) What is syntax error?
Syntax errors are associated with mistakes in the use of a programming language. It maybe a command that was misspelled or a command that must was entered in lowercase mode but was instead entered with an upper case character. A misplaced symbol, or lack of symbol, somewhere within a line of code can also lead to syntax error.
21) What are variables and it what way is it different from constants?
Variables and constants may at first look similar in a sense that both are identifiers made up of one character or more characters (letters, numbers and a few allowable symbols). Both will also hold a particular value. Values held by a variable can be altered throughout the program, and can be used in most operations and computations. Constants are given values at one time only, placed at the beginning of a program. This value is not altered in the program. For example, you can assigned a constant named PI and give it a value 3.1415 . You can then use it as PI in the program, instead of having to write 3.1415 each time you need it.
22) How do you access the values within an array?
Arrays contain a number of elements, depending on the size you gave it during variable declaration. Each element is assigned a number from 0 to number of elements-1. To assign or retrieve the value of a particular element, refer to the element number. For example: if you have a declaration that says “intscores[5];”, then you have 5 accessible elements, namely: scores[0], scores[1], scores[2], scores[3] and scores[4].
23) Can I use “int” data type to store the value 32768? Why?
No. “int” data type is capable of storing values from -32768 to 32767. To store 32768, you can use “long int” instead. You can also use “unsigned int”, assuming you don’t intend to store negative values.
24) Can two or more operators such as \n and \t be combined in a single line of program code?
Yes, it’s perfectly valid to combine operators, especially if the need arises. For example: you can have a code like printf (“Hello\n\n\’World\'”) to output the text “Hello” on the first line and “World” enclosed in single quotes to appear on the next two lines.
25) Why is it that not all header files are declared in every C program?
The choice of declaring a header file at the top of each C program would depend on what commands/functions you will be using in that program. Since each header file contains different function definitions and prototype, you would be using only those header files that would contain the functions you will need. Declaring all header files in every program would only increase the overall file size and load of the program, and is not considered a good programming style.
26) When is the “void” keyword used in a function?
When declaring functions, you will decide whether that function would be returning a value or not. If that function will not return a value, such as when the purpose of a function is to display some outputs on the screen, then “void” is to be placed at the leftmost part of the function header. When a return value is expected after the function execution, the data type of the return value is placed instead of “void”.
27) What are compound statements?
Compound statements are made up of two or more program statements that are executed together. This usually occurs while handling conditions wherein a series of statements are executed when a TRUE or FALSE is evaluated. Compound statements can also be executed within a loop. Curly brackets { } are placed before and after compound statements.
28) What is the significance of an algorithm to C programming?
Before a program can be written, an algorithm has to be created first. An algorithm provides a step by step procedure on how a solution can be derived. It also acts as a blueprint on how a program will start and end, including what process and computations are involved.
29) What is the advantage of an array over individual variables?
When storing multiple related data, it is a good idea to use arrays. This is because arrays are named using only 1 word followed by an element number. For example: to store the 10 test results of 1 student, one can use 10 different variable names (grade1, grade2, grade3… grade10). With arrays, only 1 name is used, the rest are accessible through the index name (grade[0], grade[1], grade[2]… grade[9]).

30) Write a loop statement that will show the following output:
C programming interview questions and answers for experienced, 31) what is wrong in this statement scanf(“%d”,whatnumber);.
An ampersand & symbol must be placed before the variable name whatnumber. Placing & means whatever integer value is entered by the user is stored at the “address” of the variable name. This is a common mistake for programmers, often leading to logical errors.
32) How do you generate random numbers in C?
Random numbers are generated in C using the rand() command . For example: anyNum = rand() will generate any integer number beginning from 0, assuming that anyNum is a variable of type integer.
33) What could possibly be the problem if a valid function name such as tolower() is being reported by the C compiler as undefined?
The most probable reason behind this error is that the header file for that function was not indicated at the top of the program. Header files contain the definition and prototype for functions and commands used in a C program. In the case of “tolower()”, the code “#include <ctype.h>” must be present at the beginning of the program.
34) What are comments and how do you insert it in a C program?
35) what is debugging.
Debugging is the process of identifying errors within a program. During program compilation, errors that are found will stop the program from executing completely. At this state, the programmer would look into the possible portions where the error occurred. Debugging ensures the removal of errors, and plays an important role in ensuring that the expected program output is met.
36) What does the && operator do in a program code?
The && is also referred to as AND operator. When using this operator, all conditions specified must be TRUE before the next action can be performed. If you have 10 conditions and all but 1 fails to evaluate as TRUE, the entire condition statement is already evaluated as FALSE
37) In C programming, what command or code can be used to determine if a number of odd or even?
There is no single command or function in C that can check if a number is odd or even. However, this can be accomplished by dividing that number by 2, then checking the remainder. If the remainder is 0, then that number is even, otherwise, it is odd. You can write it in code as:
38) What does the format %10.2 mean when included in a printf statement?
This format is used for two things: to set the number of spaces allotted for the output number and to set the number of decimal places. The number before the decimal point is for the allotted space, in this case it would allot 10 spaces for the output number. If the number of space occupied by the output number is less than 10, addition space characters will be inserted before the actual output number. The number after the decimal point sets the number of decimal places, in this case, it’s 2 decimal spaces.
39) What are logical errors and how does it differ from syntax errors?
Program that contains logical errors tend to pass the compilation process, but the resulting output may not be the expected one. This happens when a wrong formula was inserted into the code, or a wrong sequence of commands was performed. Syntax errors, on the other hand, deal with incorrect commands that are misspelled or not recognized by the compiler.
40) What are the different types of control structures in programming?
There are 3 main control structures in programming: Sequence, Selection and Repetition. Sequential control follows a top to bottom flow in executing a program, such that step 1 is first perform, followed by step 2, all the way until the last step is performed. Selection deals with conditional statements, which mean codes are executed depending on the evaluation of conditions as being TRUE or FALSE. This also means that not all codes may be executed, and there are alternative flows within. Repetitions are also known as loop structures, and will repeat one or two program statements set by a counter.
41) What is || operator and how does it function in a program?
The || is also known as the OR operator in C programming. When using || to evaluate logical conditions, any condition that evaluates to TRUE will render the entire condition statement as TRUE.
42) Can the “if” function be used in comparing strings?
No. “if” command can only be used to compare numerical values and single character values. For comparing string values, there is another function called strcmp that deals specifically with strings.
43) What are preprocessor directives?
Preprocessor directives are placed at the beginning of every C program. This is where library files are specified, which would depend on what functions are to be used in the program. Another use of preprocessor directives is the declaration of constants.Preprocessor directives begin with the # symbol.
44) What will be the outcome of the following conditional statement if the value of variable s is 10?
s >=10 && s < 25 && s!=12
45) Describe the order of precedence with regards to operators in C.
Order of precedence determines which operation must first take place in an operation statement or conditional statement. On the top most level of precedence are the unary operators !, +, – and &. It is followed by the regular mathematical operators (*, / and modulus % first, followed by + and -). Next in line are the relational operators <, <=, >= and >. This is then followed by the two equality operators == and !=. The logical operators && and || are next evaluated. On the last level is the assignment operator =.
46) What is wrong with this statement? myName = “Robin”;
You cannot use the = sign to assign values to a string variable. Instead, use the strcpy function. The correct statement would be: strcpy(myName, “Robin”);
47) How do you determine the length of a string value that was stored in a variable?
To get the length of a string value, use the function strlen(). For example, if you have a variable named FullName, you can get the length of the stored string value by using this statement: I = strlen(FullName); the variable I will now have the character length of the string value.
48) Is it possible to initialize a variable at the time it was declared?
Yes, you don’t have to write a separate assignment statement after the variable declaration, unless you plan to change it later on. For example: char planet[15] = “Earth”; does two things: it declares a string variable named planet, then initializes it with the value “Earth”.
49) Why is C language being considered a middle level language?
This is because C language is rich in features that make it behave like a high level language while at the same time can interact with hardware using low level methods. The use of a well structured approach to programming, coupled with English-like words used in functions, makes it act as a high level language. On the other hand, C can directly access memory structures similar to assembly language routines.
50) What are the different file extensions involved when programming in C?
Source codes in C are saved with .C file extension. Header files or library files have the .H file extension. Every time a program source code is successfully compiled, it creates an .OBJ object file, and an executable .EXE file.
51) What are reserved words?
Reserved words are words that are part of the standard C language library. This means that reserved words have special meaning and therefore cannot be used for purposes other than what it is originally intended for. Examples of reserved words are int, void, and return.
52) What are linked list?
A linked list is composed of nodes that are connected with another. In C programming, linked lists are created using pointers. Using linked lists is one efficient way of utilizing memory for storage.
53) What is FIFO?
In C programming, there is a data structure known as queue. In this structure, data is stored and accessed using FIFO format, or First-In-First-Out. A queue represents a line wherein the first data that was stored will be the first one that is accessible as well.
54) What are binary trees?
Binary trees are actually an extension of the concept of linked lists. A binary tree has two pointers, a left one and a right one. Each side can further branch to form additional nodes, which each node having two pointers as well. Learn more about Binary Tree in Data Structure if you are interested.
55) Not all reserved words are written in lowercase. TRUE or FALSE?
FALSE. All reserved words must be written in lowercase; otherwise the C compiler would interpret this as unidentified and invalid.
56) What is the difference between the expression “++a” and “a++”?
In the first expression, the increment would happen first on variable a, and the resulting value will be the one to be used. This is also known as a prefix increment. In the second expression, the current value of variable a would the one to be used in an operation, before the value of a itself is incremented. This is also known as postfix increment.
57) What would happen to X in this expression: X += 15; (assuming the value of X is 5)
X +=15 is a short method of writing X = X + 15, so if the initial value of X is 5, then 5 + 15 = 20.
58) In C language, the variables NAME, name, and Name are all the same. TRUE or FALSE?
FALSE. C language is a case sensitive language. Therefore, NAME, name and Name are three uniquely different variables.
59) What is an endless loop?
An endless loop can mean two things. One is that it was designed to loop continuously until the condition within the loop is met, after which a break function would cause the program to step out of the loop. Another idea of an endless loop is when an incorrect loop condition was written, causing the loop to run erroneously forever. Endless loops are oftentimes referred to as infinite loops.
60) What is a program flowchart and how does it help in writing a program?
A flowchart provides a visual representation of the step by step procedure towards solving a given problem. Flowcharts are made of symbols, with each symbol in the form of different shapes. Each shape may represent a particular entity within the entire program structure, such as a process, a condition, or even an input/output phase.
61) What is wrong with this program statement? void = 10;
The word void is a reserved word in C language. You cannot use reserved words as a user-defined variable.
62) Is this program statement valid? INT = 10.50;
Assuming that INT is a variable of type float, this statement is valid. One may think that INT is a reserved word and must not be used for other purposes. However, recall that reserved words are express in lowercase, so the C compiler will not interpret this as a reserved word.
63) What are actual arguments?
When you create and use functions that need to perform an action on some given values, you need to pass these given values to that function. The values that are being passed into the called function are referred to as actual arguments.
64) What is a newline escape sequence?
A newline escape sequence is represented by the \n character. This is used to insert a new line when displaying data in the output screen. More spaces can be added by inserting more \n characters. For example, \n\n would insert two spaces. A newline escape sequence can be placed before the actual output expression or after.
65) What is output redirection?
It is the process of transferring data to an alternative output source other than the display screen. Output redirection allows a program to have its output saved to a file. For example, if you have a program named COMPUTE, typing this on the command line as COMPUTE >DATA can accept input from the user, perform certain computations, then have the output redirected to a file named DATA, instead of showing it on the screen.
66) What are run-time errors?
These are errors that occur while the program is being executed. One common instance wherein run-time errors can happen is when you are trying to divide a number by zero. When run-time errors occur, program execution will pause, showing which program line caused the error.
67) What is the difference between functions abs() and fabs()?
These 2 functions basically perform the same action, which is to get the absolute value of the given value. Abs() is used for integer values, while fabs() is used for floating type numbers. Also, the prototype for abs() is under <stdlib.h>, while fabs() is under <math.h>.
68) What are formal parameters?
In using functions in a C program, formal parameters contain the values that were passed by the calling function. The values are substituted in these formal parameters and used in whatever operations as indicated within the main body of the called function.
69) What are control structures?
Control structures take charge at which instructions are to be performed in a program. This means that program flow may not necessarily move from one statement to the next one, but rather some alternative portions may need to be pass into or bypassed from, depending on the outcome of the conditional statements.
70) Write a simple code fragment that will check if a number is positive or negative
71) when is a “switch” statement preferable over an “if” statement.
The switch statement is best used when dealing with selections based on a single variable or expression. However, switch statements can only evaluate integer and character data types.
72) What are global variables and how do you declare them?
Global variables are variables that can be accessed and manipulated anywhere in the program. To make a variable global, place the variable declaration on the upper portion of the program, just after the preprocessor directives section.
73) What are enumerated types?
Enumerated types allow the programmer to use more meaningful words as values to a variable. Each item in the enumerated type variable is actually associated with a numeric code. For example, one can create an enumerated type variable named DAYS whose values are Monday, Tuesday… Sunday.
74) What does the function toupper() do?
It is used to convert any letter to its upper case mode. Toupper() function prototype is declared in <ctype.h>. Note that this function will only convert a single character, and not an entire string.
75) Is it possible to have a function as a parameter in another function?
Yes, that is allowed in C programming. You just need to include the entire function prototype into the parameter field of the other function where it is to be used.
76) What are multidimensional arrays?
Multidimensional arrays are capable of storing data in a two or more dimensional structure. For example, you can use a 2 dimensional array to store the current position of pieces in a chess game, or position of players in a tic-tac-toe program.
77) Which function in C can be used to append a string to another string?
The strcat function. It takes two parameters, the source string and the string value to be appended to the source string.
78) What is the difference between functions getch() and getche()?
Both functions will accept a character input value from the user. When using getch(), the key that was pressed will not appear on the screen, and is automatically captured and assigned to a variable. When using getche(), the key that was pressed by the user will appear on the screen, while at the same time being assigned to a variable.
79) Dothese two program statements perform the same output? 1) scanf(“%c”, &letter); 2) letter=getchar()
Yes, they both do the exact same thing, which is to accept the next key pressed by the user and assign it to variable named letter.
80) What are structure types in C?
Structure types are primarily used to store records. A record is made up of related fields. This makes it easier to organize a group of related data.
81) What does the characters “r” and “w” mean when writing programs that will make use of files?
“r” means “read” and will open a file as input wherein data is to be retrieved. “w” means “write”, and will open a file for output. Previous data that was stored on that file will be erased.
82) What is the difference between text files and binary files?
Text files contain data that can easily be understood by humans. It includes letters, numbers and other characters. On the other hand, binary files contain 1s and 0s that only computers can interpret.
83) is it possible to create your own header files?
Yes, it is possible to create a customized header file. Just include in it the function prototypes that you want to use in your program, and use the #include directive followed by the name of your header file.
84) What is dynamic data structure?
Dynamic data structure provides a means for storing data more efficiently into memory. Using Using dynamic memory allocation , your program will access memory spaces as needed. This is in contrast to static data structure, wherein the programmer has to indicate a fix number of memory space to be used in the program.
85) What are the different data types in C?
The basic data types in C are int, char, and float. Int is used to declare variables that will be storing integer values. Float is used to store real numbers. Char can store individual character values.
86) What is the general form of a C program?
A C program begins with the preprocessor directives, in which the programmer would specify which header file and what constants (if any) to be used. This is followed by the main function heading. Within the main function lies the variable declaration and program statement.
87) What is the advantage of a random access file?
If the amount of data stored in a file is fairly large, the use of random access will allow you to search through it quicker. If it had been a sequential access file, you would have to go through one record at a time until you reach the target data. A random access file lets you jump directly to the target address where data is located.
88) In a switch statement, what will happen if a break statement is omitted?
If a break statement was not placed at the end of a particular case portion? It will move on to the next case portion, possibly causing incorrect output.
89) Describe how arrays can be passed to a user defined function
One thing to note is that you cannot pass the entire array to a function. Instead, you pass to it a pointer that will point to the array first element in memory. To do this, you indicate the name of the array without the brackets.
90) What are pointers?
Pointers point to specific areas in the memory. Pointers contain the address of a variable, which in turn may contain a value or even an address to another memory.
91) Can you pass an entire structure to functions?
Yes, it is possible to pass an entire structure to a function in a call by method style. However, some programmers prefer declaring the structure globally, then pass a variable of that structure type to a function. This method helps maintain consistency and uniformity in terms of argument type.
92) What is gets() function?
The gets() function allows a full line data entry from the user. When the user presses the enter key to end the input, the entire line of characters is stored to a string variable. Note that the enter key is not included in the variable, but instead a null terminator \0 is placed after the last character.
93) The % symbol has a special use in a printf statement. How would you place this character as part of the output on the screen?
You can do this by using %% in the printf statement. For example, you can write printf(“10%%”) to have the output appear as 10% on the screen.
94) How do you search data in a data file using random access method?
Use the fseek() function to perform random access input/ouput on a file. After the file was opened by the fopen() function, the fseek would require three parameters to work: a file pointer to the file, the number of bytes to search, and the point of origin in the file.
95) Are comments included during the compilation stage and placed in the EXE file as well?
No, comments that were encountered by the compiler are disregarded. Comments are mostly for the guidance of the programmer only and do not have any other significant use in the program functionality.
96) Is there a built-in function in C that can be used for sorting data?
Yes, use the qsort() function. It is also possible to create user defined functions for sorting, such as those based on the balloon sort and bubble sort algorithm.
97) What are the advantages and disadvantages of a heap?
Storing data on the heap is slower than it would take when using the stack. However, the main advantage of using the heap is its flexibility. That’s because memory in this structure can be allocated and remove in any particular order. Slowness in the heap can be compensated if an algorithm was well designed and implemented.
98) How do you convert strings to numbers in C?
You can write you own functions to do string to number conversions, or instead use C’s built in functions. You can use atof to convert to a floating point value, atoi to convert to an integer value, and atol to convert to a long integer value.
99) Create a simple code fragment that will swap the values of two variables num1 and num2.
100) what is the use of a semicolon (;) at the end of every program statement.
It has to do with the parsing process and compilation of the code. A semicolon acts as a delimiter, so that the compiler knows where each statement ends, and can proceed to divide the statement into smaller elements for syntax checking.
These interview questions will also help in your viva(orals)
- Bitwise Operators in C: AND, OR, XOR, Shift & Complement
- Dynamic Memory Allocation in C using malloc(), calloc() Functions
- Type Casting in C: Type Conversion, Implicit, Explicit with Example
- C Programming Tutorial PDF for Beginners
- 13 BEST C Programming Books for Beginners (2024 Update)
- Difference Between C and Java
- Difference Between Structure and Union in C
- calloc() Function in C Library with Program EXAMPLE
Say "Hello, World!" With C++ Easy C++ (Basic) Max Score: 5 Success Rate: 98.65%
Input and output easy c++ (basic) max score: 5 success rate: 94.00%, basic data types easy c++ (basic) max score: 10 success rate: 80.45%, conditional statements easy c++ (basic) max score: 10 success rate: 96.80%, for loop easy c++ (basic) max score: 10 success rate: 94.75%, functions easy c++ (basic) max score: 10 success rate: 97.44%, pointer easy c++ (basic) max score: 10 success rate: 97.22%, arrays introduction easy c++ (basic) max score: 10 success rate: 97.08%, variable sized arrays easy c++ (basic) max score: 30 success rate: 93.18%, attribute parser medium c++ (basic) max score: 35 success rate: 84.76%, cookie support is required to access hackerrank.
Seems like cookies are disabled on this browser, please enable them to open this website
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
- 30 OOPs Interview Questions and Answers (2024)
- C++ Interview Questions and Answers (2024)
Top 100 C++ Coding Interview Questions and Answers (2024)
- Top 50+ Python Interview Questions and Answers (Latest 2024)
- Java Interview Questions and Answers
- Java Collections Interview Questions and Answers
- Top 20 Java Multithreading Interview Questions & Answers
- Top 100 Data Structure and Algorithms DSA Interview Questions Topic-wise
- Top 50 Array Coding Problems for Interviews
- Most Asked Problems in Data Structures and Algorithms | Beginner DSA Sheet
- Top 10 Algorithms in Interview Questions
- Machine Learning Interview Questions
- Top 50 Problems on Linked List Data Structure asked in SDE Interviews
- Top 50 Problems on Heap Data Structure asked in SDE Interviews
- Data Analyst Interview Questions and Answers
- SQL Query Interview Questions
- Top Linux Interview Questions With Answer
- MySQL Interview Questions
- Top 50 Django Interview Questions and Answers
- Top 50 Networking Interview Questions (2024)
- Software Testing Interview Questions
C++ is one of the most popular languages in the software industry for developing software ranging from operating systems, and DBMS to games. That is why it is also popular to be asked to write C++ Programs in live coding sessions in job placement interviews.
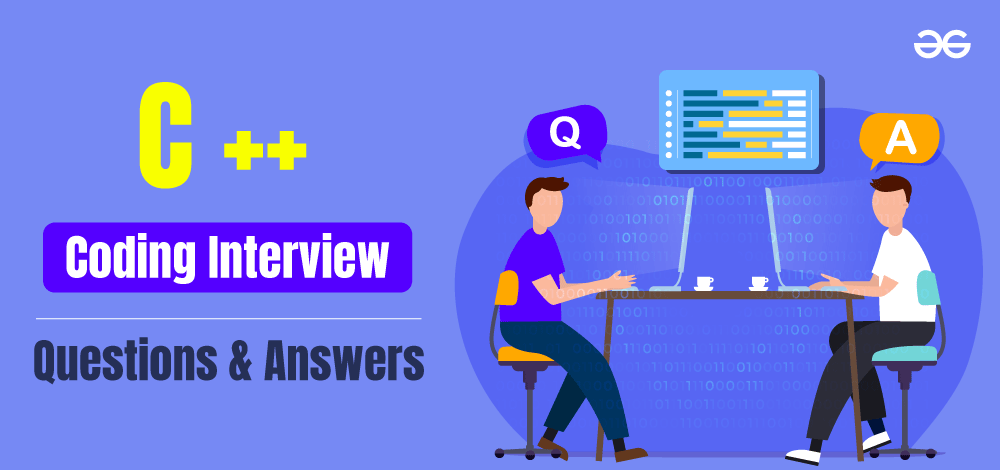
This article provides a list of C++ coding interview questions for beginners as well as experienced professionals. The questions are designed to test candidates’ understanding of the following topics:
- C++ syntax and semantics
- Data structures and algorithms
- Object-oriented programming
- Memory management
List of 100 C++ Coding Interview Questions and Answers
Here is a list of 100 C++ coding interview questions and answers
1. Write a C++ Program to Check Whether a Number is a Positive or Negative Number.
2. write a program to find the greatest of the three numbers., 3. c++ program to check whether number is even or odd.
For more information, refer to the article – C++ Program To Check Whether Number is Even Or Odd
4. Write a Program to Find the ASCII Value of a Character
5. write a program to check whether a character is a vowel or consonant, 6. write a program to print check whether a character is an alphabet or not, 7. write a program to find the length of the string without using strlen() function , 8. write a program to toggle each character in a string , 9. write a program to count the number of vowels , 10. write a program to remove the vowels from a string, 11. write a program to remove all characters from a string except alphabets, 12. write a program to remove spaces from a string, 13. write a program to find the sum of the first n natural numbers, 14. write a program to find the factorial of a number using loops, 15. write a program to find a leap year or not, 16. write a program to check the prime number, 17. write a program to check palindrome, 18. write a program to check whether a number is an armstrong number or not, 19. write a program to find the nth term of the fibonacci series, 20. write a program to calculate the greatest common divisor of two numbers, 21. write a program to calculate the lowest common multiple (lcm) of two numbers, 22. write a program for finding the roots of a quadratic equation, 23. write a program to find the smallest and largest element in an array, 24. write a program to find the second smallest element in an array, 25. write a program to calculate the sum of elements in an array, 26. write a program to check if the given string is palindrome or not, 27. write a program to check if two strings are anagram or not, 28. write a program to print a diamond pattern, 29. write a program to print a pyramid pattern, 30. write a program to print the hourglass pattern, 31. write a program to print the rotated hourglass pattern, 32. write a program to print a simple pyramid pattern, 33. write a program to print an inverted pyramid , 34. write a program to print a triangle star pattern, 35. write a program to print floyd’s triangle, 36. write a program to print the pascal triangle, 37. write a program to print the given string in reverse order , 38. write a c++ program to print the given string in reverse order using recursion, 39. write a program to check if the given string is palindrome or not using recursion, 40. write a program to calculate the length of the string using recursion, 41. write a program to calculate the factorial of a number using recursion, 42. write a program to count the sum of numbers in a string, 43. write a program to print all natural numbers up to n without using a semi-colon, 44. write a program to swap the values of two variables without using any extra variable, 45. write a program to print the maximum value of an unsigned int using one’s complement (~) operator, 46. write a program to check for the equality of two numbers without using arithmetic or comparison operator, 47. write a program to find the maximum and minimum of the two numbers without using the comparison operator, 48. write a program for octal to decimal conversion, 49. write a program for hexadecimal to decimal conversion, 50. write a program for decimal to binary conversion, 51. write a program for decimal octal conversion, 52. write a program for decimal to hexadecimal conversion, 53. write a program for binary to octal conversion , 54. write a program for octal to binary conversion , 55. write a program to implement the use of encapsulation, 56. write a program to implement the concept of abstraction, 57. write a program to implement the concept of compile-time polymorphism or function overloading, 58. write a program to implement the concept of operator overloading, 59. write a program to implement the concept of function overriding or runtime polymorphism, 60. write a program to implement single-level inheritance, 61. write a program to create a class for complex numbers, 62. write a program to implement the inch feet system, 63. write a program to implement bubble sort, 64. write a program to implement insertion sort, 65. write a program to implement selection sort, 66. write a program to implement merge sort, 67. write a program to implement quick sort, 68. write a program to implement linear search, 69. write a program to implement binary search, 70. write a program to find the index of a given element in a vector, 71. write a program to remove duplicate elements in an array using stl, 72. write a program to sort an array in descending order using stl, 73. write a program to calculate the frequency of each word in the given string, 74. write a program to find k maximum elements of an array in the original order, 75. write a program to find all unique subsets of a given set using stl, 76. write a program to iterate over a queue without removing the element, 77. write a program for the implementation of stacks using an array, 78. write a program for the implementation of a queue using an array, 79. write a program implementation of stacks using a queue, 80. write a program to implement a stack using the list in stl, 81. write a program to determine array is a subset of another array or not, 82. write a program for finding the circular rotation of an array by k positions, 83. write a program to sort the first half in ascending order and the second half in descending, 84. write a program to print the given string in reverse order, 85. write a program to print all permutations of a string using recursion, 86. write a program to print all permutations of a given string in lexicographically sorted order, 87. write a program to remove brackets from an algebraic expression, 88. program to perform insert, delete, and print operations singly linked list, 89. program to perform insert, delete, and print operations doubly linked list, 90. program to perform insert, delete, and print operations circular linked list, 91. program for inorder traversal in a binary tree, 92. program to find all its subsets from a set of positive integers, 93. program for preorder traversal in a binary tree, 94. program for postorder traversal in a binary tree, 95. program for level-order traversal in a binary tree, 96. write a program for the top view of a binary tree, 97. write a program to print the bottom view of a binary tree, 98. write a program to print the left view of a binary tree, 99. write a program to print the right view of the binary tree, 100. write a program for the conversion of infix expression to postfix expression.
Coding interviews can be challenging task, but they are also a great opportunity to test your skills and knowledge. By practicing with commonly ask C++ coding interview questions , you can increase your chances of success.
Remember to stay calm, communicate your thought process clearly, and don’t be afraid to ask for clarification if needed. With hard work and preparation, you can ace your interview and land your dream job!
C++ Coding Interview Questions – FAQs
Q: what are the most common c++ coding interview questions.
The most common C++ coding interview questions are designed to test your knowledge of the following topics: C++ syntax and semantics Data structures and algorithms Object-oriented programming Memory management Pointers Templates & More.
Q: What should I do during a C++ coding interview?
When you are asked a coding question in an interview, take a moment to think about the problem before you start coding. Explain your thought process to the interviewer as you are coding. This will help them to understand your approach to solving problems.
Q: What are some tips for writing clean and efficient code?
Here are some tips for writing clean and efficient code: Use meaningful variable names. Use whitespace to make your code readable. Break down complex problems into smaller, more manageable problems. Use comments to explain your code. Test your code thoroughly. Use appropriate data structures and algorithms. Avoid unnecessary duplication of code.
Q: How can I improve my communication skills during C++ coding interviews?
Here are some tips for improving your communication skills during C++ coding interviews: Speak clearly and concisely. Explain your thought process to the interviewer. Ask clarifying questions if needed. Be prepared to answer questions about your code. Be open to feedback. Practicing explaining your code to others is a great way to improve your communication skills. You can also practice by giving yourself mock interviews.
Please Login to comment...
Similar reads.
- interview-questions
- How to Use ChatGPT with Bing for Free?
- 7 Best Movavi Video Editor Alternatives in 2024
- How to Edit Comment on Instagram
- 10 Best AI Grammar Checkers and Rewording Tools
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

IMAGES
VIDEO
COMMENTS
Q2: Write a Program to find the Sum of two numbers entered by the user. In this problem, you have to write a program that adds two numbers and prints their sum on the console screen. For Example, Input: Enter two numbers A and B : 5 2. Output: Sum of A and B is: 7.
C is a general-purpose, imperative computer programming language, supporting structured programming, lexical variable scope and recursion, while a static type system prevents many unintended operations. C was originally developed by Dennis Ritchie between 1969 and 1973 at Bell Labs.
We have gathered a variety of C exercises (with answers) for each C Chapter. Try to solve an exercise by editing some code, or show the answer to see what you've done wrong. Count Your Score. You will get 1 point for each correct answer. Your score and total score will always be displayed.
1. Write a C program to get the indices of two numbers in a given array of integers. This will enable you to get the sum of two numbers equal to a specific target. Expected Output: Original Array: 4 2 1 5 Target Value: 7 Indices of the two numbers whose sum equal to target value: 7 1 3. Click me to see the solution. 2.
Matrix (2D array) Add two matrices. Scalar matrix multiplication. Multiply two matrices. Check if two matrices are equal. Sum of diagonal elements of matrix. Interchange diagonal of matrix. Find upper triangular matrix. Find sum of lower triangular matrix.
Join over 23 million developers in solving code challenges on HackerRank, one of the best ways to prepare for programming interviews. ... For Loop in C. Easy C (Basic) Max Score: 10 Success Rate: 93.64%. Solve Challenge. Sum of Digits of a Five Digit Number. Easy C (Basic) Max Score: 15 Success Rate: 98.66%.
C Programs: Practicing and solving problems is the best way to learn anything. Here, we have provided 100+ C programming examples in different categories like basic C Programs, Fibonacci series in C, String, Array, Base Conversion, Pattern Printing, Pointers, etc. These C programs are the most asked interview questions from basic to advanced level.
1. Why is C called a mid-level programming language? Due to its ability to support both low-level and high-level features, C is considered a middle-level language. It is both an assembly-level language, i.e. a low-level language, and a higher-level language.
List of function and recursion programming exercises. Write a C program to find cube of any number using function. Write a C program to find diameter, circumference and area of circle using functions. Write a C program to find maximum and minimum between two numbers using functions. Write a C program to check whether a number is even or odd ...
Don't stop until you are in the top 1%. Get hands-on experience with Practice C programming practice problem course on CodeChef. Solve a wide range of Practice C coding challenges and boost your confidence in programming.
C Basic Solved Programs. C Program to Print Hello World Program. C Program to calculate a simple interest. C program to convert Total days to year, month and days. C program to find Sum and Average of two numbers. C Program to check whether number is EVEN or ODD. C Program to find largest number among three numbers.
Here is the list of the top 500 C Programming Questions and Answers. Download C Programming Questions PDF free with Solutions. All solutions are in C language. All the solutions have 4 basic parts programming problems, logic & explanation of code, programming solutions code, and the output of the program. To summarize our programming questions ...
gotoxy (),clrscr (),getch (),getche () for GCC, Linux. C program to print character without using format specifiers. C program to find Binary Addition and Binary Subtraction. C program to print weekday of given date. C program to check given string is a valid IPv4 address or not. More advance programs...
C++ Solved programs —-> C++ is a powerful general-purpose programming language. It is fast, portable and available in all platforms. This page contains the C++ solved programs/examples with solutions, here we are providing most important programs on each topic. These C examples cover a wide range of programming areas in Computer Science.
Likewise, the statement "x -" means to decrement the value of x by 1. Another way of writing increment statements is to use the conventional + plus sign or - minus sign. In the case of "x++", another way to write it is "x = x +1". 👉 Free PDF Download: C Programming Interview Questions & Answers >>.
There are 50 questions to complete. Here's a complete roadmap for you to become a developer: Learn DSA -> Master Frontend/Backend/Full Stack -> Build Projects -> Keep Applying to Jobs. And why go anywhere else when our DSA to Development: Coding Guide helps you do this in a single program!
Join over 23 million developers in solving code challenges on HackerRank, one of the best ways to prepare for programming interviews.
Boost your coding interview skills and confidence by practicing real interview questions with LeetCode. Our platform offers a range of essential problems for practice, as well as the latest questions being asked by top-tier companies.
If you would like to learn "C Programming" thoroughly, you should attempt to work on the complete set of 1000+ MCQs - multiple choice questions and answers mentioned above. It will immensely help anyone trying to crack an exam or an interview. Wish you the best in your endeavor to learn and master C Programming!
List of 50 C Coding Interview Questions and Answer. Here is a list of 50 C coding interview questions and answers: 1. Find the largest number among the three numbers. 2. Write a Program to check whether a number is prime or not. 3. Write a C program to calculate Compound Interest. 4.
Coding has become a crucial round of elimination in every company's recruitment process. Most beginners prefer to code in C than other languages like C++, Java, Python, etc.With this in mind, we have exclusively listed 150+ most asked C programming questions/examples based on their difficulty level.. You can practice the below listed basic C programs in the order listed & in case you get stuck ...
Watch the live stream of the Inter-Schools Reading Quiz quarter-final on the Joy Prime channel.
Our C++ exercise sheet is designed to help you at every stage of the learning process. Solving C++ exercises can help you practice different C++ concepts in codes, and understand their workings. Each problem you solve brings you one step closer to your goal of mastering C++. Frequently Asked Questions (FAQs) Q1.
This article provides a list of C++ coding interview questions for beginners as well as experienced professionals. The questions are designed to test candidates' understanding of the following topics: C++ syntax and semantics. Data structures and algorithms. Object-oriented programming.