Learn Python practically and Get Certified .

Popular Tutorials
Popular examples, reference materials, learn python interactively, python introduction.
- How to Get Started With Python?
- Python Comments
- Python Variables, Constants and Literals
- Python Type Conversion
- Python Basic Input and Output
- Python Operators
- Precedence and Associativity of Operators in Python (programiz.com)
Python Flow Control
- Python if...else Statement
- Python for Loop
- Python while Loop
- Python break and continue
- Python pass Statement
Python Data types
- Python Numbers, Type Conversion and Mathematics
Python List
Python Tuple
- Python Sets
- Python Dictionary
- Python String
- Python Functions
- Python Function Arguments
- Python Variable Scope
- Python Global Keyword
- Python Recursion
- Python Modules
- Python Package
- Python Main function (programiz.com)
Python Files
- Python Directory and Files Management
- Python CSV: Read and Write CSV files (programiz.com)
- Reading CSV files in Python (programiz.com)
- Writing CSV files in Python (programiz.com)
- Python Exception Handling
- Python Exceptions
- Python Custom Exceptions
Python Object & Class
- Python Objects and Classes
- Python Inheritance
- Python Multiple Inheritance
- Polymorphism in Python(with Examples) (programiz.com)
- Python Operator Overloading
Python Advanced Topics
- List comprehension
- Python Lambda/Anonymous Function
- Python Iterators
- Python Generators
- Python Namespace and Scope
- Python Closures
- Python Decorators
- Python @property decorator
- Python RegEx
Python Date and Time
- Python datetime
- Python strftime()
- Python strptime()
- How to get current date and time in Python?
- Python Get Current Time
- Python timestamp to datetime and vice-versa
- Python time Module
- Python sleep()
Additional Topic
- Python Keywords and Identifiers
- Python Asserts
- Python Json
- Python *args and **kwargs (With Examples) (programiz.com)
Python Tutorials
Python List pop()
Python List insert()
Python List extend()
- Python List index()
- Python List append()
- Python List remove()
Python lists are one of the most commonly used and versatile built-in types. They allow us to store multiple items in a single variable.
- Create a Python List
We create a list by placing elements inside square brackets [] , separated by commas. For example,
Here, the ages list has three items.
In Python, lists can store data of different data types.
We can use the built-in list() function to convert other iterables (strings, dictionaries, tuples, etc.) to a list.
List Characteristics
- Ordered - They maintain the order of elements.
- Mutable - Items can be changed after creation.
- Allow duplicates - They can contain duplicate values.
- Access List Elements
Each element in a list is associated with a number, known as a index .
The index always starts from 0 . The first element of a list is at index 0 , the second element is at index 1 , and so on.

Access Elements Using Index
We use index numbers to access list elements. For example,

More on Accessing List Elements
Python also supports negative indexing. The index of the last element is -1 , the second-last element is -2 , and so on.
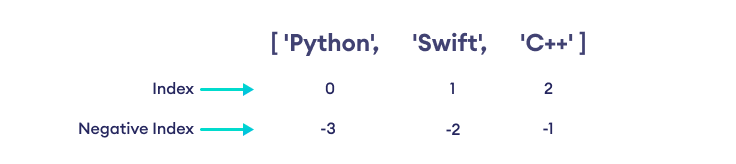
Negative indexing makes it easy to access list items from last.
Let's see an example,
In Python, it is possible to access a section of items from the list using the slicing operator : . For example,
To learn more about slicing, visit Python program to slice lists .
Note : If the specified index does not exist in a list, Python throws the IndexError exception.
- Add Elements to a Python List
We use the append() method to add an element to the end of a Python list. For example,
The insert() method adds an element at the specified index. For example,
We use the extend() method to add elements to a list from other iterables. For example,
- Change List Items
We can change the items of a list by assigning new values using the = operator. For example,
Here, we have replaced the element at index 2: 'Green' with 'Blue' .
- Remove an Item From a List
We can remove an item from a list using the remove() method. For example,
The del statement removes one or more items from a list. For example,
Note : We can also use the del statement to delete the entire list. For example,
- Python List Length
We can use the built-in len() function to find the number of elements in a list. For example,
- Iterating Through a List
We can use a for loop to iterate over the elements of a list. For example,
- Python List Methods
Python has many useful list methods that make it really easy to work with lists.
More on Python Lists
List Comprehension is a concise and elegant way to create a list. For example,
To learn more, visit Python List Comprehension .
We use the in keyword to check if an item exists in the list. For example,
- orange is not present in fruits , so, 'orange' in fruits evaluates to False .
- cherry is present in fruits , so, 'cherry' in fruits evaluates to True .
Note: Lists are similar to arrays in other programming languages. When people refer to arrays in Python, they often mean lists, even though there is a numeric array type in Python.
- Python list()
Table of Contents
- Introduction
Video: Python Lists and Tuples
Sorry about that.
Related Tutorials
Python Library
Python Tutorial
Never forget a class or assignment again.
Unlock your potential and manage your classes, tasks and exams with mystudylife- the world's #1 student planner and school organizer app..
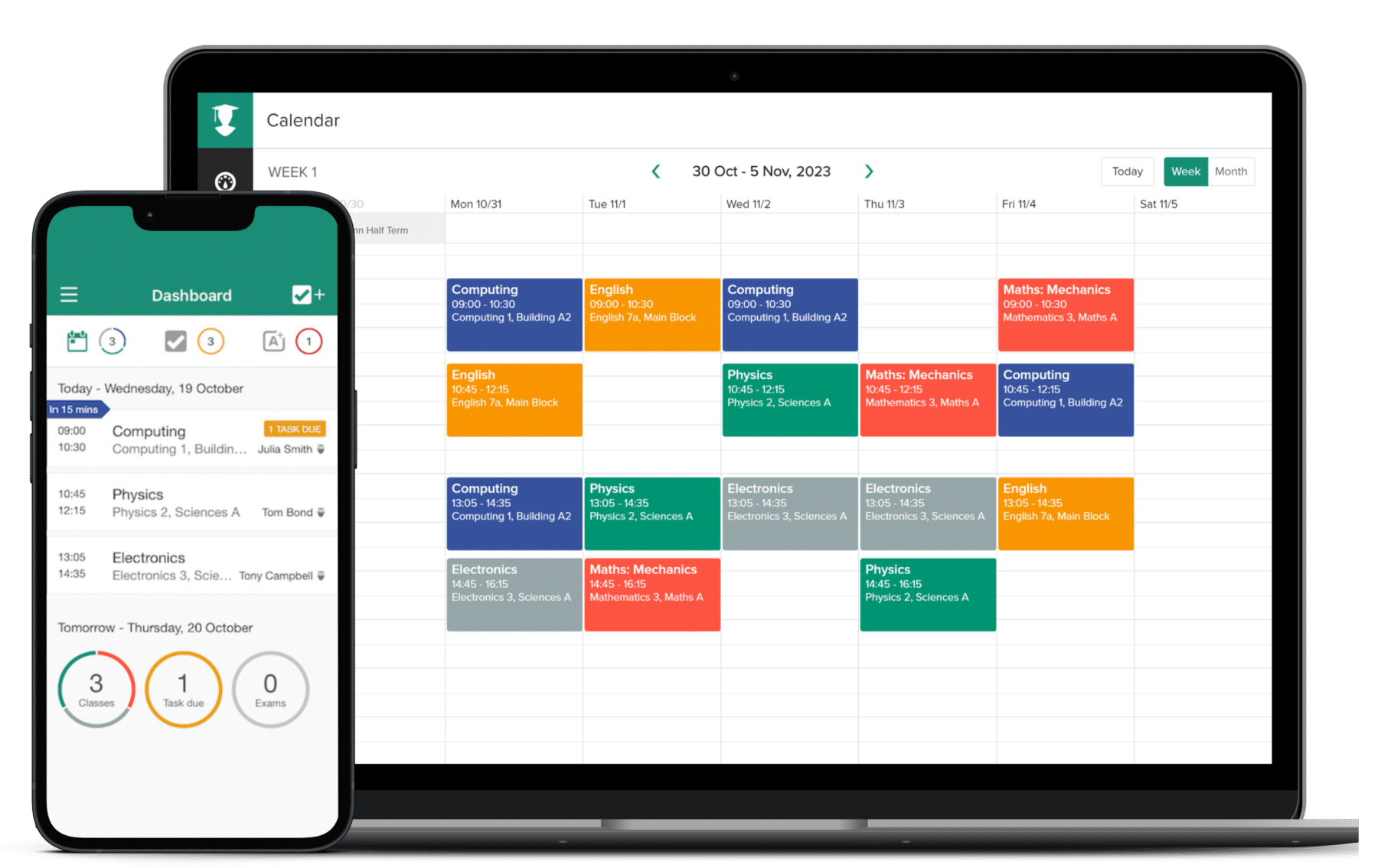
School planner and organizer
The MyStudyLife planner app supports rotation schedules, as well as traditional weekly schedules. MSL allows you to enter your school subjects, organize your workload, and enter information about your classes – all so you can effortlessly keep on track of your school calendar.
Homework planner and task tracker
Become a master of task management by tracking every single task with our online planner – no matter how big or small.
Stay on top of your workload by receiving notifications of upcoming classes, assignments or exams, as well as incomplete tasks, on all your devices.

“Featuring a clean interface, MyStudyLife offers a comprehensive palette of schedules, timetables and personalized notifications that sync across multiple devices.”
” My Study Life is a calendar app designed specifically for students. As well as showing you your weekly timetable– with support for rotations – you can add exams, essay deadlines and reminders, and keep a list of all the tasks you need to complete. It also works on the web, so you can log in and check your schedule from any device.”
“MyStudyLife is a great study planner app that makes it simple for students to add assignments, classes, and tests to a standard weekly schedule.”
“I cannot recommend this platform enough. My Study Life is the perfect online planner to keep track of your classes and assignments. I like to use both the website and the mobile app so I can use it on my phone and computer! I do not go a single day without using this platform–go check it out!!”
“Staying organized is a critical part of being a disciplined student, and the MyStudyLife app is an excellent organizer.”

The ultimate study app
The MyStudyLife student planner helps you keep track of all your classes, tasks, assignments and exams – anywhere, on any device.
Whether you’re in middle school, high school or college MyStudyLife’s online school agenda will organize your school life for you for less stress, more productivity, and ultimately, better grades.

Take control of your day with MyStudyLife

Stay on top of your studies. Organize tasks, set reminders, and get better grades, one day at a time.

We get it- student life can be busy. Start each day with the confidence that nothing important will be forgotten, so that you can stay focused and get more done.

Track your class schedule on your phone or computer, online or offline, so that you always know where you’re meant to be.

Shift your focus back to your goals, knowing that MyStudyLife has your back with timely reminders that make success the main event of your day

Say goodbye to last minute stress with MyStudyLife’s homework planner to make procrastination a thing of the past.

Coming soon!
MyStudyLife has lots of exciting changes and features in the works. Stay tuned!
Stay on track on all of your devices.
All your tasks are automatically synced across all your devices, instantly.
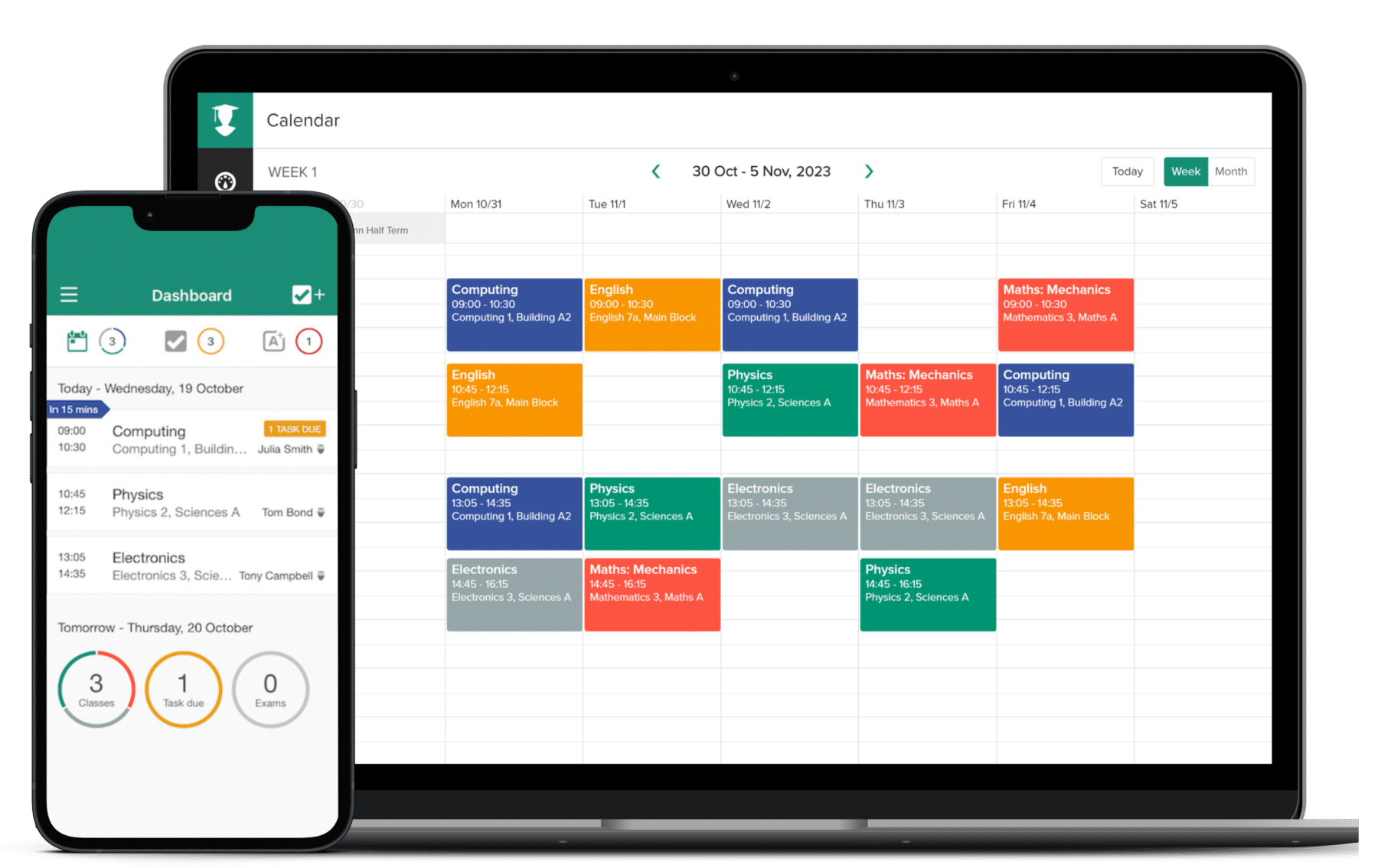
Trusted by millions of students around the world.

School can be hard. MyStudyLife makes it easier.
Our easy-to-use online study planner app is available on the App Store, the Google Play Store and can be used on desktop. This means that you can use MyStudyLife anywhere and on any device.
Discover more on the MyStudyLife blog
See how MyStudyLife can help organize your life.

JEE Main 2024: Best Tips, Study Plan & Timetable
Las 10 mejores apps gratis para estudiar mejor en 2024 , filter by category.
- Career Planning
- High School Tips and Tricks
- Productivity
- Spanish/Español
- Student News
- University Advice
- Using MyStudyLife
Hit enter to search or ESC to close

Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python lists.
Lists are used to store multiple items in a single variable.
Lists are one of 4 built-in data types in Python used to store collections of data, the other 3 are Tuple , Set , and Dictionary , all with different qualities and usage.
Lists are created using square brackets:
Create a List:
List items are ordered, changeable, and allow duplicate values.
List items are indexed, the first item has index [0] , the second item has index [1] etc.
When we say that lists are ordered, it means that the items have a defined order, and that order will not change.
If you add new items to a list, the new items will be placed at the end of the list.
Note: There are some list methods that will change the order, but in general: the order of the items will not change.
The list is changeable, meaning that we can change, add, and remove items in a list after it has been created.
Allow Duplicates
Since lists are indexed, lists can have items with the same value:
Lists allow duplicate values:
Advertisement
List Length
To determine how many items a list has, use the len() function:
Print the number of items in the list:
List Items - Data Types
List items can be of any data type:
String, int and boolean data types:
A list can contain different data types:
A list with strings, integers and boolean values:
From Python's perspective, lists are defined as objects with the data type 'list':
What is the data type of a list?
The list() Constructor
It is also possible to use the list() constructor when creating a new list.
Using the list() constructor to make a List:
Python Collections (Arrays)
There are four collection data types in the Python programming language:
- List is a collection which is ordered and changeable. Allows duplicate members.
- Tuple is a collection which is ordered and unchangeable. Allows duplicate members.
- Set is a collection which is unordered, unchangeable*, and unindexed. No duplicate members.
- Dictionary is a collection which is ordered** and changeable. No duplicate members.
*Set items are unchangeable, but you can remove and/or add items whenever you like.
**As of Python version 3.7, dictionaries are ordered . In Python 3.6 and earlier, dictionaries are unordered .
When choosing a collection type, it is useful to understand the properties of that type. Choosing the right type for a particular data set could mean retention of meaning, and, it could mean an increase in efficiency or security.

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Integrations
- Getting Started
- Help Center
- Productivity Methods + Quiz
- Inspiration Hub
Organize your work and life, finally.
Become focused, organized, and calm with Todoist. The world’s #1 task manager and to-do list app.
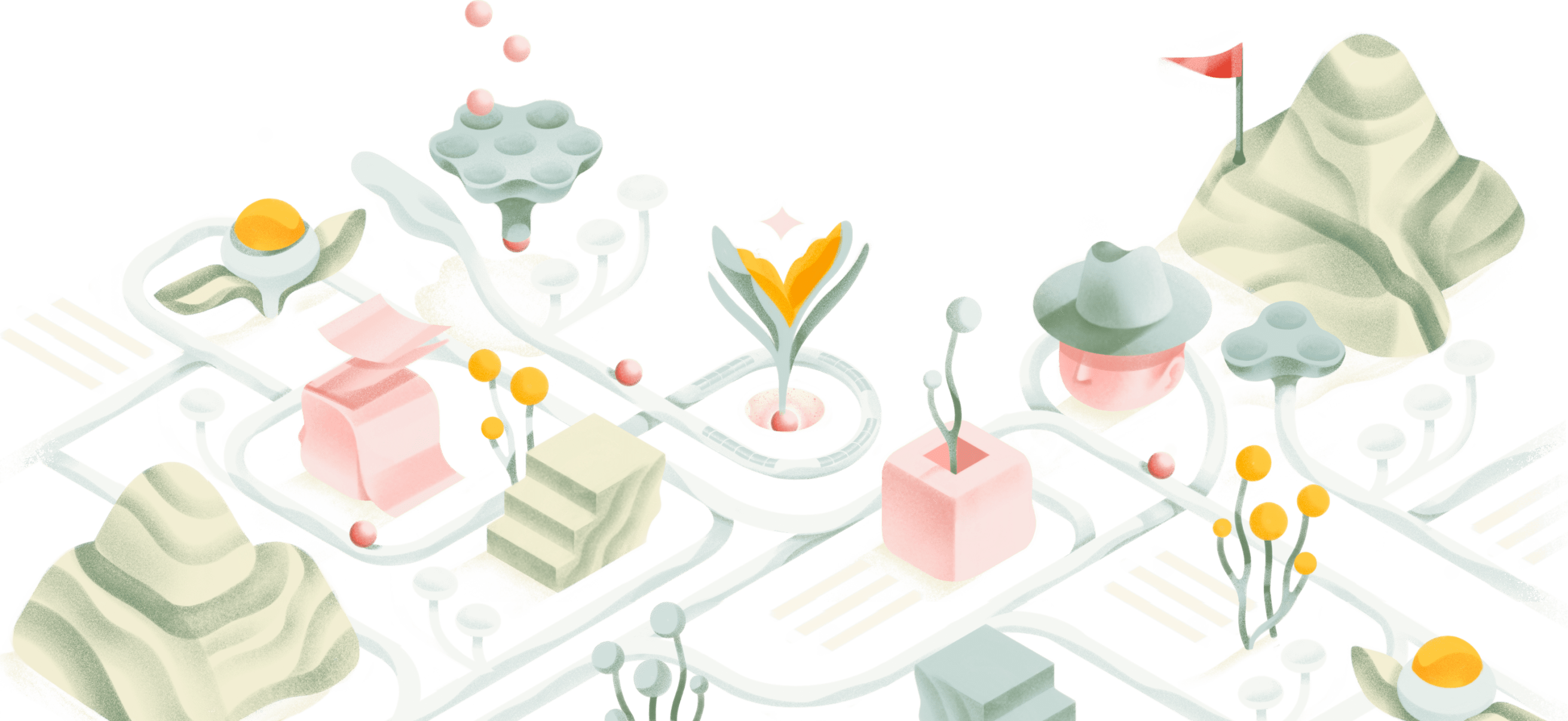
42+ million people and teams trust their sanity and productivity to Todoist
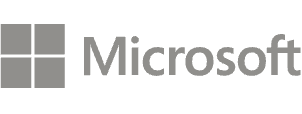
Clear your mind
The fastest way to get tasks out of your head.
Type just about anything into the task field and Todoist’s one-of-its-kind natural language recognition will instantly fill your to-do list.
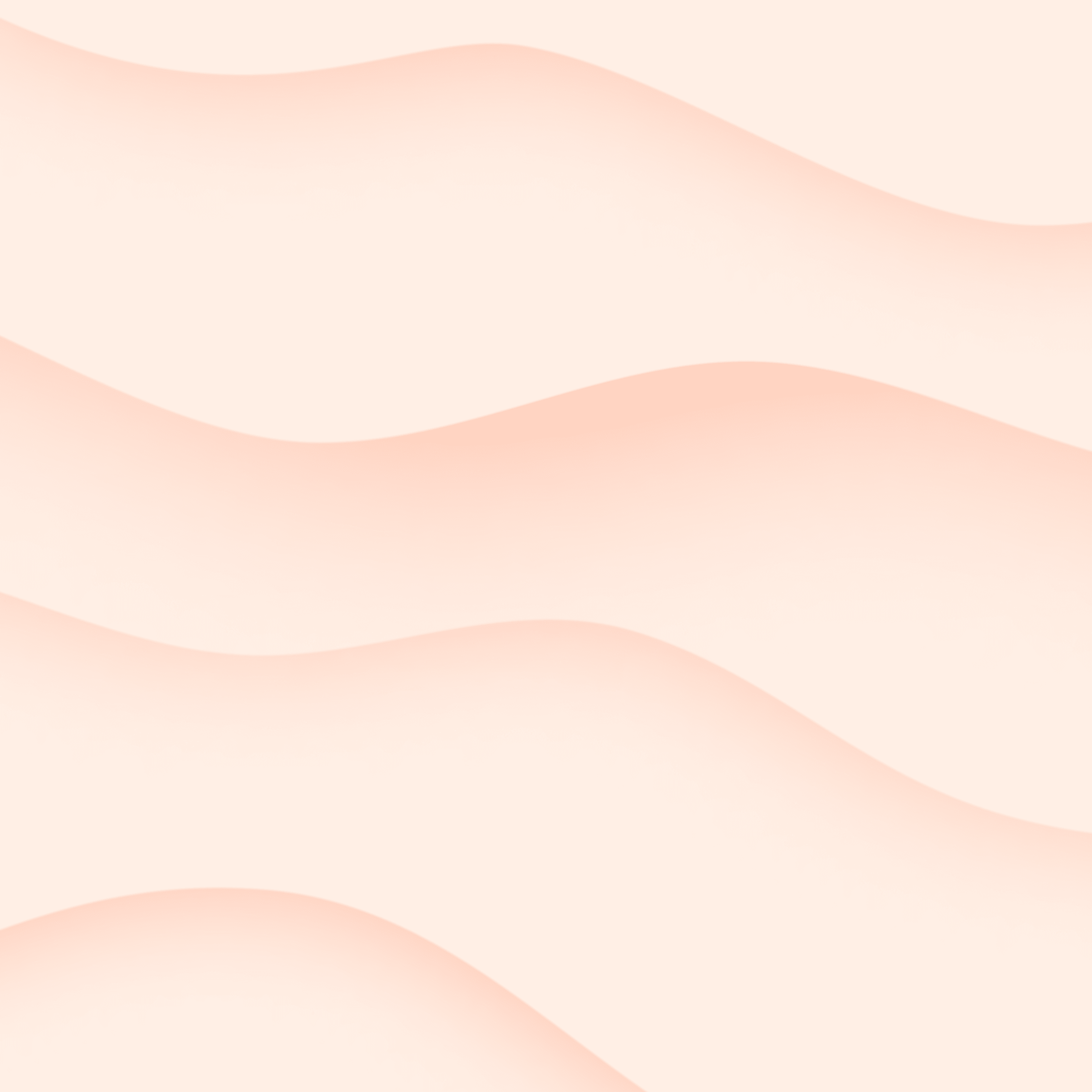
Focus on what’s important
Reach that mental clarity you’ve been longing for.
Your tasks are automatically sorted into Today, Upcoming, and custom Filter views to help you prioritize your most important work.
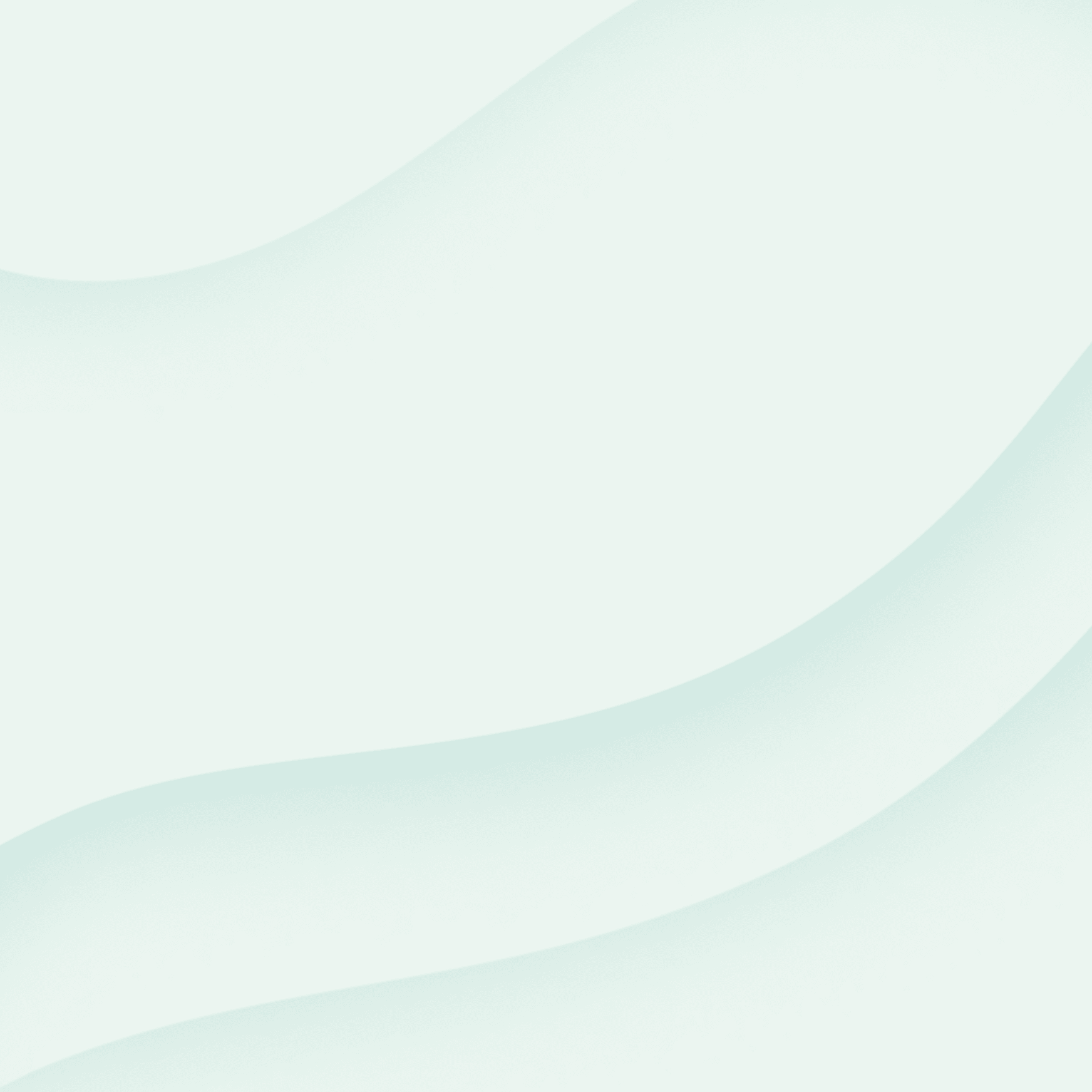
You reached #TodoistZero!
Organize your teamwork, too
Where all your tasks can finally coexist.
Give your team a shared space to collaborate and stay on top of it all – alongside but separate from your personal tasks and projects.
My Projects
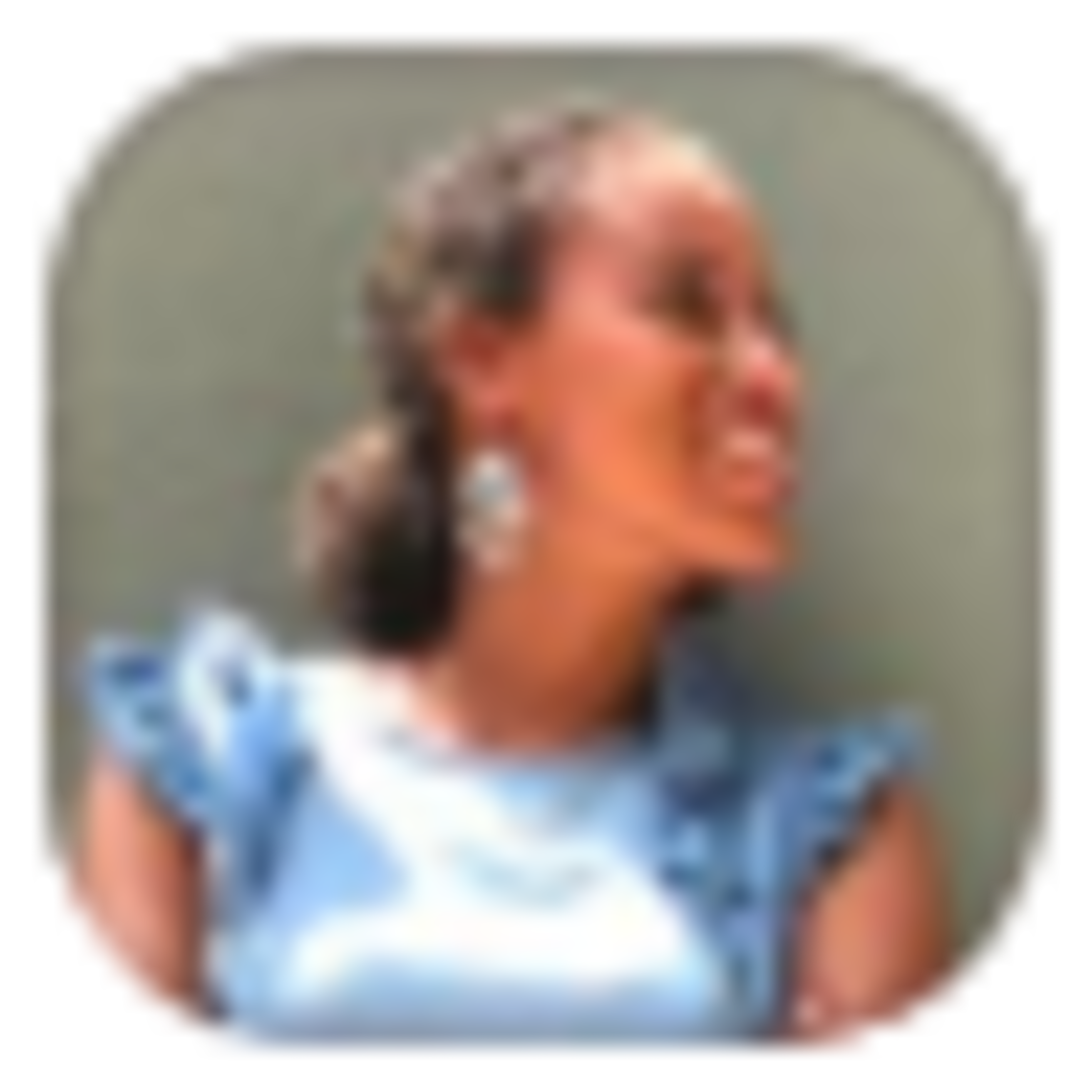
Appointments
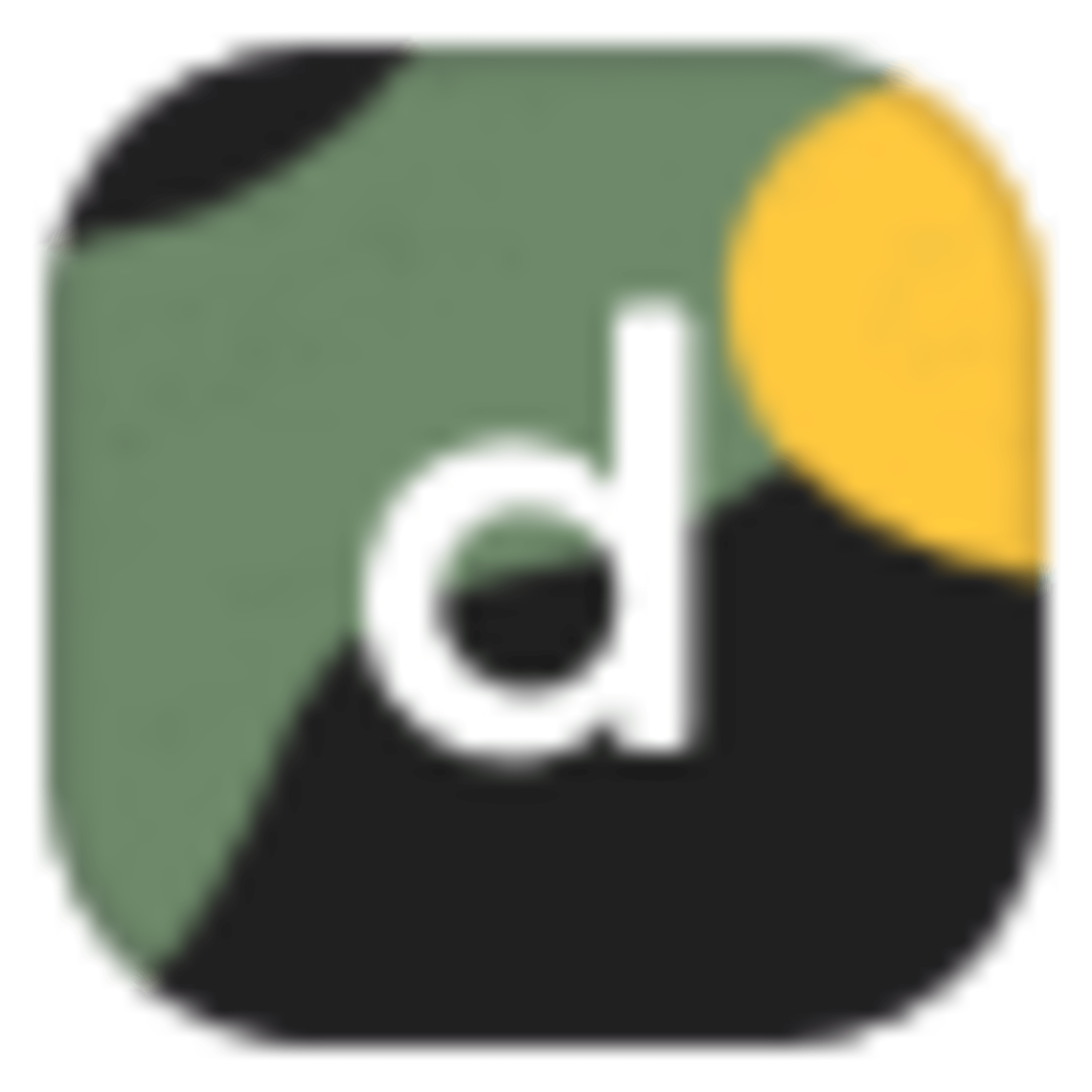
Website Update
Product Roadmap
Meeting Agenda
“Todoist makes it easy to go as simple or as complex as you want”
– The Verge
Explore all Todoist has to offer
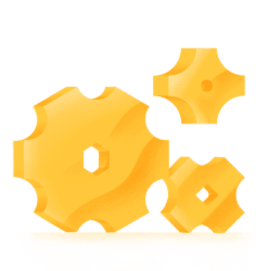
Template gallery
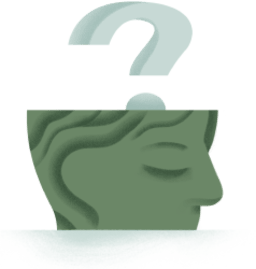
Productivity quiz
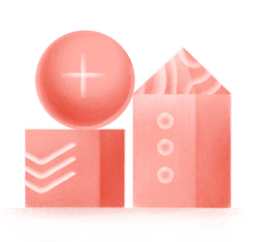
Extension gallery
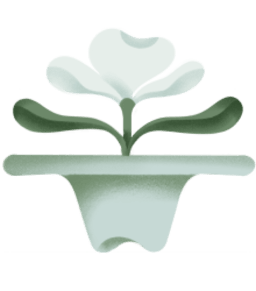
Inspiration hub
15 Checklist, Schedule, and Planner Templates for Students
Planning templates for students can help keep track of classes and homework, making preparations for the school year a breeze.
Templates are extremely useful for business documents, but for students they can be lifesavers.
You have enough to think about during the school year, so using a template can save a ton of time. Put your mind on your classes and use these helpful checklist and planning templates for the rest.
1. Homework Checklist
For a plain and simple homework checklist, this template from TeacherVision is great for younger students, but can work for any age. Each subject is in its own spot with days of the week and check boxes to mark off as you complete assignments.
2. Printable Homework Planner
This next homework planner from TidyForm lets you easily plan your assignments for each day of the week and even the weekend. Instead of listing out the subjects, you can enter them yourself for the day and include details with due dates for each.
Note: you will need a PDF editor to make changes to the template on your computer.
3. Homework Schedule
Another planner from TidyForm breaks down your days into time blocks. Each hour slot is along the left side of the sheet with the seven days of the week across the top. This one is great for assignments, but you could use it for class schedules or work shifts to plan your entire week ahead of time.
It is a basic template, but a useful one.
4. Class Schedule and Planner
If you need a more detailed planner, this schedule is intended for classes. However, it can also be used for more. It uses time blocks like the TidyForm planner, but breaks them down into increments that you choose. Adjust the start time and interval minutes and the sheet automatically updates. You can add your classes, pop in your homework time, and add shifts for work all in one place.
5. Assignment Schedule
This template from Vertex42 is another with time blocks in 30-minute increments. And, this one has even more detail. On one side of the template, you can list out classes with assignments, dates, and times.
On the other side, you can add your class schedule or plan your homework and projects. The workbook also includes a Homeschool tab for parents homeschooling their children. Overall, it's a good dual-purpose option.
6. Multiple-Task Planner
If you are a OneNote user, check out this option from OneNoteGem. You can quickly fill out subjects and assignments for five days of the week. This is ideal for classes that have many tasks on the same day.
For example, you may need to work on a group project, research a paper, and finish an assignment in one day. The template has a good amount of room for those to-dos.
7. Student Notebook
Also, for OneNote you can download an entire student notebook template. Just scroll further down on the OneNoteGem templates page for this option.
What's nice about this template is that the notebook includes sections for planners, five classes, and research along with note-taking tips.
8. Class Schedule
For a neat and flexible class schedule template, this one is available for Excel, OpenOffice, and Google Sheets. It is basic with time slots broken into 15-minute increments on one tab and 30-minute increments on another. Plus, it includes seven days of the week, unlike many others. For college students, this is a terrific class schedule template.
9. Student Planner
With a student planner that lists your subjects by week, you can stay on track every single day. Vertex42 has two templates to pick from that offer different layouts.
One option has the subjects down the left side with days of the week across the top. The other template is the reverse of that. Each has spots for to-dos and notes and is available for either Excel or as a PDF.
10. All-in-One Schedule and Budget
For an all-in-one workbook for college, this Excel template has sheets for classes per term, course credits, a college budget, and textbooks. You can keep everything in one place. You can also track your overall progress and your current GPA.
11. Student Calendar
Another planner from Microsoft Office is this 12-month student calendar. There is a tab for each month, spots for a weekly schedule, and a section for assignments. The year cell is editable making it reusable for your entire college career.
This template makes planning study time and homework a breeze.
12. Dorm Room Checklist
If you are heading to a dorm room for college, there is no better way to make sure you have everything than with this checklist template. You can add box numbers for packing and checks when you pack the items.
The template gives you sections such as kitchen supplies, electronics, computer equipment, safety items, and more.
13. Back to School Checklist
For parents with kids in elementary or middle school, this checklist is perfect for back-to-school time. One column has tasks to take care of like verifying immunizations and obtaining a school supply list. The second column has items to purchase from clothes and a backpack to school supplies.
If you have a youngster getting ready for a new school year, this is the template for you.
14. College Budget
When you need to keep an eye on your college budget, this template is just for it. The top section is for your funding and income with the bottom for your expenses. The most common types of college-related items are included, making this a convenient template for college students.
15. Monthly College Budget
This monthly budget tracker from Microsoft Office gives you a simple way to view your cash flow. You can glance at the pie charts at the top to get an overview of your income and expenses by month. Change the values below to add your items and the charts change automatically.
It's one simple sheet with everything you need to budget each month.
Time for Class!
For classes, assignments, budgeting, supplies, course credits, and all that goes with these things, make sure you are prepared when the bell rings or classroom door closes. Now that you have these 15 awesome template options, you are on your way to starting the school year off right.
You might also check out these essential Windows apps for students to help with school.
Image Credits: Rawpixel.com/Shutterstock

Understanding Assignments
What this handout is about.
The first step in any successful college writing venture is reading the assignment. While this sounds like a simple task, it can be a tough one. This handout will help you unravel your assignment and begin to craft an effective response. Much of the following advice will involve translating typical assignment terms and practices into meaningful clues to the type of writing your instructor expects. See our short video for more tips.
Basic beginnings
Regardless of the assignment, department, or instructor, adopting these two habits will serve you well :
- Read the assignment carefully as soon as you receive it. Do not put this task off—reading the assignment at the beginning will save you time, stress, and problems later. An assignment can look pretty straightforward at first, particularly if the instructor has provided lots of information. That does not mean it will not take time and effort to complete; you may even have to learn a new skill to complete the assignment.
- Ask the instructor about anything you do not understand. Do not hesitate to approach your instructor. Instructors would prefer to set you straight before you hand the paper in. That’s also when you will find their feedback most useful.
Assignment formats
Many assignments follow a basic format. Assignments often begin with an overview of the topic, include a central verb or verbs that describe the task, and offer some additional suggestions, questions, or prompts to get you started.
An Overview of Some Kind
The instructor might set the stage with some general discussion of the subject of the assignment, introduce the topic, or remind you of something pertinent that you have discussed in class. For example:
“Throughout history, gerbils have played a key role in politics,” or “In the last few weeks of class, we have focused on the evening wear of the housefly …”
The Task of the Assignment
Pay attention; this part tells you what to do when you write the paper. Look for the key verb or verbs in the sentence. Words like analyze, summarize, or compare direct you to think about your topic in a certain way. Also pay attention to words such as how, what, when, where, and why; these words guide your attention toward specific information. (See the section in this handout titled “Key Terms” for more information.)
“Analyze the effect that gerbils had on the Russian Revolution”, or “Suggest an interpretation of housefly undergarments that differs from Darwin’s.”
Additional Material to Think about
Here you will find some questions to use as springboards as you begin to think about the topic. Instructors usually include these questions as suggestions rather than requirements. Do not feel compelled to answer every question unless the instructor asks you to do so. Pay attention to the order of the questions. Sometimes they suggest the thinking process your instructor imagines you will need to follow to begin thinking about the topic.
“You may wish to consider the differing views held by Communist gerbils vs. Monarchist gerbils, or Can there be such a thing as ‘the housefly garment industry’ or is it just a home-based craft?”
These are the instructor’s comments about writing expectations:
“Be concise”, “Write effectively”, or “Argue furiously.”
Technical Details
These instructions usually indicate format rules or guidelines.
“Your paper must be typed in Palatino font on gray paper and must not exceed 600 pages. It is due on the anniversary of Mao Tse-tung’s death.”
The assignment’s parts may not appear in exactly this order, and each part may be very long or really short. Nonetheless, being aware of this standard pattern can help you understand what your instructor wants you to do.
Interpreting the assignment
Ask yourself a few basic questions as you read and jot down the answers on the assignment sheet:
Why did your instructor ask you to do this particular task?
Who is your audience.
- What kind of evidence do you need to support your ideas?
What kind of writing style is acceptable?
- What are the absolute rules of the paper?
Try to look at the question from the point of view of the instructor. Recognize that your instructor has a reason for giving you this assignment and for giving it to you at a particular point in the semester. In every assignment, the instructor has a challenge for you. This challenge could be anything from demonstrating an ability to think clearly to demonstrating an ability to use the library. See the assignment not as a vague suggestion of what to do but as an opportunity to show that you can handle the course material as directed. Paper assignments give you more than a topic to discuss—they ask you to do something with the topic. Keep reminding yourself of that. Be careful to avoid the other extreme as well: do not read more into the assignment than what is there.
Of course, your instructor has given you an assignment so that he or she will be able to assess your understanding of the course material and give you an appropriate grade. But there is more to it than that. Your instructor has tried to design a learning experience of some kind. Your instructor wants you to think about something in a particular way for a particular reason. If you read the course description at the beginning of your syllabus, review the assigned readings, and consider the assignment itself, you may begin to see the plan, purpose, or approach to the subject matter that your instructor has created for you. If you still aren’t sure of the assignment’s goals, try asking the instructor. For help with this, see our handout on getting feedback .
Given your instructor’s efforts, it helps to answer the question: What is my purpose in completing this assignment? Is it to gather research from a variety of outside sources and present a coherent picture? Is it to take material I have been learning in class and apply it to a new situation? Is it to prove a point one way or another? Key words from the assignment can help you figure this out. Look for key terms in the form of active verbs that tell you what to do.
Key Terms: Finding Those Active Verbs
Here are some common key words and definitions to help you think about assignment terms:
Information words Ask you to demonstrate what you know about the subject, such as who, what, when, where, how, and why.
- define —give the subject’s meaning (according to someone or something). Sometimes you have to give more than one view on the subject’s meaning
- describe —provide details about the subject by answering question words (such as who, what, when, where, how, and why); you might also give details related to the five senses (what you see, hear, feel, taste, and smell)
- explain —give reasons why or examples of how something happened
- illustrate —give descriptive examples of the subject and show how each is connected with the subject
- summarize —briefly list the important ideas you learned about the subject
- trace —outline how something has changed or developed from an earlier time to its current form
- research —gather material from outside sources about the subject, often with the implication or requirement that you will analyze what you have found
Relation words Ask you to demonstrate how things are connected.
- compare —show how two or more things are similar (and, sometimes, different)
- contrast —show how two or more things are dissimilar
- apply—use details that you’ve been given to demonstrate how an idea, theory, or concept works in a particular situation
- cause —show how one event or series of events made something else happen
- relate —show or describe the connections between things
Interpretation words Ask you to defend ideas of your own about the subject. Do not see these words as requesting opinion alone (unless the assignment specifically says so), but as requiring opinion that is supported by concrete evidence. Remember examples, principles, definitions, or concepts from class or research and use them in your interpretation.
- assess —summarize your opinion of the subject and measure it against something
- prove, justify —give reasons or examples to demonstrate how or why something is the truth
- evaluate, respond —state your opinion of the subject as good, bad, or some combination of the two, with examples and reasons
- support —give reasons or evidence for something you believe (be sure to state clearly what it is that you believe)
- synthesize —put two or more things together that have not been put together in class or in your readings before; do not just summarize one and then the other and say that they are similar or different—you must provide a reason for putting them together that runs all the way through the paper
- analyze —determine how individual parts create or relate to the whole, figure out how something works, what it might mean, or why it is important
- argue —take a side and defend it with evidence against the other side
More Clues to Your Purpose As you read the assignment, think about what the teacher does in class:
- What kinds of textbooks or coursepack did your instructor choose for the course—ones that provide background information, explain theories or perspectives, or argue a point of view?
- In lecture, does your instructor ask your opinion, try to prove her point of view, or use keywords that show up again in the assignment?
- What kinds of assignments are typical in this discipline? Social science classes often expect more research. Humanities classes thrive on interpretation and analysis.
- How do the assignments, readings, and lectures work together in the course? Instructors spend time designing courses, sometimes even arguing with their peers about the most effective course materials. Figuring out the overall design to the course will help you understand what each assignment is meant to achieve.
Now, what about your reader? Most undergraduates think of their audience as the instructor. True, your instructor is a good person to keep in mind as you write. But for the purposes of a good paper, think of your audience as someone like your roommate: smart enough to understand a clear, logical argument, but not someone who already knows exactly what is going on in your particular paper. Remember, even if the instructor knows everything there is to know about your paper topic, he or she still has to read your paper and assess your understanding. In other words, teach the material to your reader.
Aiming a paper at your audience happens in two ways: you make decisions about the tone and the level of information you want to convey.
- Tone means the “voice” of your paper. Should you be chatty, formal, or objective? Usually you will find some happy medium—you do not want to alienate your reader by sounding condescending or superior, but you do not want to, um, like, totally wig on the man, you know? Eschew ostentatious erudition: some students think the way to sound academic is to use big words. Be careful—you can sound ridiculous, especially if you use the wrong big words.
- The level of information you use depends on who you think your audience is. If you imagine your audience as your instructor and she already knows everything you have to say, you may find yourself leaving out key information that can cause your argument to be unconvincing and illogical. But you do not have to explain every single word or issue. If you are telling your roommate what happened on your favorite science fiction TV show last night, you do not say, “First a dark-haired white man of average height, wearing a suit and carrying a flashlight, walked into the room. Then a purple alien with fifteen arms and at least three eyes turned around. Then the man smiled slightly. In the background, you could hear a clock ticking. The room was fairly dark and had at least two windows that I saw.” You also do not say, “This guy found some aliens. The end.” Find some balance of useful details that support your main point.
You’ll find a much more detailed discussion of these concepts in our handout on audience .
The Grim Truth
With a few exceptions (including some lab and ethnography reports), you are probably being asked to make an argument. You must convince your audience. It is easy to forget this aim when you are researching and writing; as you become involved in your subject matter, you may become enmeshed in the details and focus on learning or simply telling the information you have found. You need to do more than just repeat what you have read. Your writing should have a point, and you should be able to say it in a sentence. Sometimes instructors call this sentence a “thesis” or a “claim.”
So, if your instructor tells you to write about some aspect of oral hygiene, you do not want to just list: “First, you brush your teeth with a soft brush and some peanut butter. Then, you floss with unwaxed, bologna-flavored string. Finally, gargle with bourbon.” Instead, you could say, “Of all the oral cleaning methods, sandblasting removes the most plaque. Therefore it should be recommended by the American Dental Association.” Or, “From an aesthetic perspective, moldy teeth can be quite charming. However, their joys are short-lived.”
Convincing the reader of your argument is the goal of academic writing. It doesn’t have to say “argument” anywhere in the assignment for you to need one. Look at the assignment and think about what kind of argument you could make about it instead of just seeing it as a checklist of information you have to present. For help with understanding the role of argument in academic writing, see our handout on argument .
What kind of evidence do you need?
There are many kinds of evidence, and what type of evidence will work for your assignment can depend on several factors–the discipline, the parameters of the assignment, and your instructor’s preference. Should you use statistics? Historical examples? Do you need to conduct your own experiment? Can you rely on personal experience? See our handout on evidence for suggestions on how to use evidence appropriately.
Make sure you are clear about this part of the assignment, because your use of evidence will be crucial in writing a successful paper. You are not just learning how to argue; you are learning how to argue with specific types of materials and ideas. Ask your instructor what counts as acceptable evidence. You can also ask a librarian for help. No matter what kind of evidence you use, be sure to cite it correctly—see the UNC Libraries citation tutorial .
You cannot always tell from the assignment just what sort of writing style your instructor expects. The instructor may be really laid back in class but still expect you to sound formal in writing. Or the instructor may be fairly formal in class and ask you to write a reflection paper where you need to use “I” and speak from your own experience.
Try to avoid false associations of a particular field with a style (“art historians like wacky creativity,” or “political scientists are boring and just give facts”) and look instead to the types of readings you have been given in class. No one expects you to write like Plato—just use the readings as a guide for what is standard or preferable to your instructor. When in doubt, ask your instructor about the level of formality she or he expects.
No matter what field you are writing for or what facts you are including, if you do not write so that your reader can understand your main idea, you have wasted your time. So make clarity your main goal. For specific help with style, see our handout on style .
Technical details about the assignment
The technical information you are given in an assignment always seems like the easy part. This section can actually give you lots of little hints about approaching the task. Find out if elements such as page length and citation format (see the UNC Libraries citation tutorial ) are negotiable. Some professors do not have strong preferences as long as you are consistent and fully answer the assignment. Some professors are very specific and will deduct big points for deviations.
Usually, the page length tells you something important: The instructor thinks the size of the paper is appropriate to the assignment’s parameters. In plain English, your instructor is telling you how many pages it should take for you to answer the question as fully as you are expected to. So if an assignment is two pages long, you cannot pad your paper with examples or reword your main idea several times. Hit your one point early, defend it with the clearest example, and finish quickly. If an assignment is ten pages long, you can be more complex in your main points and examples—and if you can only produce five pages for that assignment, you need to see someone for help—as soon as possible.
Tricks that don’t work
Your instructors are not fooled when you:
- spend more time on the cover page than the essay —graphics, cool binders, and cute titles are no replacement for a well-written paper.
- use huge fonts, wide margins, or extra spacing to pad the page length —these tricks are immediately obvious to the eye. Most instructors use the same word processor you do. They know what’s possible. Such tactics are especially damning when the instructor has a stack of 60 papers to grade and yours is the only one that low-flying airplane pilots could read.
- use a paper from another class that covered “sort of similar” material . Again, the instructor has a particular task for you to fulfill in the assignment that usually relates to course material and lectures. Your other paper may not cover this material, and turning in the same paper for more than one course may constitute an Honor Code violation . Ask the instructor—it can’t hurt.
- get all wacky and “creative” before you answer the question . Showing that you are able to think beyond the boundaries of a simple assignment can be good, but you must do what the assignment calls for first. Again, check with your instructor. A humorous tone can be refreshing for someone grading a stack of papers, but it will not get you a good grade if you have not fulfilled the task.
Critical reading of assignments leads to skills in other types of reading and writing. If you get good at figuring out what the real goals of assignments are, you are going to be better at understanding the goals of all of your classes and fields of study.
You may reproduce it for non-commercial use if you use the entire handout and attribute the source: The Writing Center, University of North Carolina at Chapel Hill
Make a Gift

How to Write a List Correctly: Colons, Commas, and Semicolons
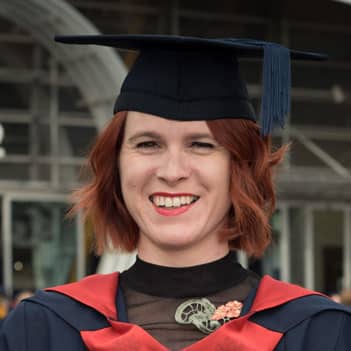
If you want to write a list but aren’t sure about the correct punctuation, look no further. In this article, you’ll learn how to appropriately use colons, commas, and semicolons when making lists.
- Colons are sometimes used to introduce a list.
- Commas separate items in a simple list.
- Semicolons are used to separate items in a complex list.
How to Write a List Correctly
For writers, list-making is a handy tool to illustrate your ideas or to make your text more readable by breaking it up.
There are two types of lists: horizontal and vertical. Each type uses colons, commas, and/or semicolons.
A Punctuation Review
Before we dig in, let’s review what colons, commas, and semicolons are.
Colons look like this:
Commas look like this:
Semicolons look like this:
Horizontal Lists
Horizontal lists help you give examples or specify your argument by having ideas laid out next to each other.
Colons, commas, and semicolons come in handy when it comes to laying out your list and making it look neat. But what are the standard guidelines?
Using Colons in a List
First of all, the colon. It can be used to introduce lists but isn’t necessary. Your list can be a simple continuation of your sentence.
For instance:
The available colors are blue, gray , and white.
You should use a colon, though, if you use an apposition (e.g., “the following”).
The available colors are the following: blue, gray, and white.
You should also use a colon to introduce a list if semicolons separate the items in the list:
The available colors are: blue and gray; black and white; and red and pink.
Later I’ll explain whether to choose commas or semicolons to separate the items in your list.
Using Commas in a List
Use commas to separate items in a simple list - that is, if each item comprises a single word.
The following sentence illustrates this:
For lunch, you can have a toastie, salad, or fries.
Using Semicolons in a List
You can use semicolons to separate items in complex lists - that is, if each item comprises several words or contains the conjunction ‘and.’
If you use semicolons to separate the items, you must also introduce the list with a colon.
I’ll show you what I mean.
For lunch, you can have: a cheese and ham toastie; a caesar salad; or french fries with ketchup .
Because each separate item contains several words, and sometimes the word ‘and’ it could be confusing to the reader if they were only separated with commas.
It’s by no means necessary to do this and perfectly acceptable to use commas still, but it’s just a way to make your list easier on the eye.
Vertical Lists
Vertical lists are a great way to make items stand out or to break up your text by making it more visually appealing. They are usually made with bullet points, numbers, or letters.
A common problem with vertical lists is deciding which punctuation to use at the end of each item.
Here are some easy-to-follow guidelines:
- Put a comma at the end if the items are unpunctuated single words or phrases.
- Use a semicolon at the end if the items are punctuated but aren’t complete sentences.
- If the items are complete sentences, use a full stop at the end as you usually would when writing a regular sentence.
The last item in your bulleted list needs a full stop. You can look at the bulleted list above as an example of a vertical list that uses full sentences.
Here’s an example of a vertical list with unpunctuated single words or phrases:
The top three things we look for in a Masters Student candidate are
- motivation,
And here’s an example of a vertical list with punctuated clauses or phrases:
The plan for this evening is to go:
- to the restaurant for dinner;
- dancing with friends;
- have the happiest birthday .
To introduce a list, use a colon if the items are complete sentences that stand alone. If it’s just a clause or phrase, use no punctuation, and imagine the bulleted list as being a continuation of the sentence.
Top Tip! If you’re writing some kind of brochure or creative document, you can take more freedom with the punctuation since your goal is to make it look as appealing and readable as possible.
Concluding Thoughts on How to Write a List Correctly
I hope this article has helped you feel more confident about using punctuation when writing lists. Let’s summarize what we’ve learned:
- Use commas to separate items in a simple list.
- Use semicolons to separate items in a complex list.
- Use colons to introduce a list after an apposition or semicolons to separate the list items.
If you found this article helpful, check out our blog archive on navigating complex grammar rules.
Learn More:
- 'Dos and Don'ts': How to Write Them With Proper Grammar
- How to Write a Movie Title in an Essay or Article
- How to Write Comedy: Tips and Examples to Make People Laugh
- ‘Spicket’ or ‘Spigot’: How to Spell It Correctly
- How to Write Height Correctly - Writing Feet and Inches
- Assertive Sentence Examples: What is an Assertive Sentence?
- Optative Sentence Example and Definition: What Is an Optative Sentence?
- Grammar Book: Learn Basic English Grammar
- How to Write a Monologue: Tips and Examples
- ‘Writing’ or ‘Writting’: How to Spell It Correctly
- How to Correctly Apply 'In Which', 'Of Which', 'At Which', Etc.
- ‘Goodmorning’ or ‘Good Morning’: How to Spell ‘Good Morning’ Correctly
- ‘Realy’ or ‘Really’: How to Spell ‘Really’ Correctly
- ‘Eachother’ or ‘Each Other’: How to Spell ‘Each Other’ Correctly
- 'Do' or 'Does': How to Use Them Correctly
We encourage you to share this article on Twitter and Facebook . Just click those two links - you'll see why.
It's important to share the news to spread the truth. Most people won't.
Add new comment Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Post Comment

Free Task List and Checklist Templates
By Kate Eby | July 19, 2016
- Share on Facebook
- Share on LinkedIn
Link copied
In this article, we’ve gathered the most comprehensive list of downloadable task and checklist templates to keep your personal and professional endeavors on track.
Included on this page, you’ll find a variety of free templates in Word, Excel, and PDF formats, such as a weekly task list template , project task template , event to-do list template , and more.
Team Task List Template
Use this template to keep your team organized and cut wasted time in status meetings. Create a centralized view of all team member's responsibilities across multiple projects.
We’ve also included pre-built templates from Smartsheet, a work execution platform that empowers you to better manage checklists and deadlines with real-time collaboration and project visibility.
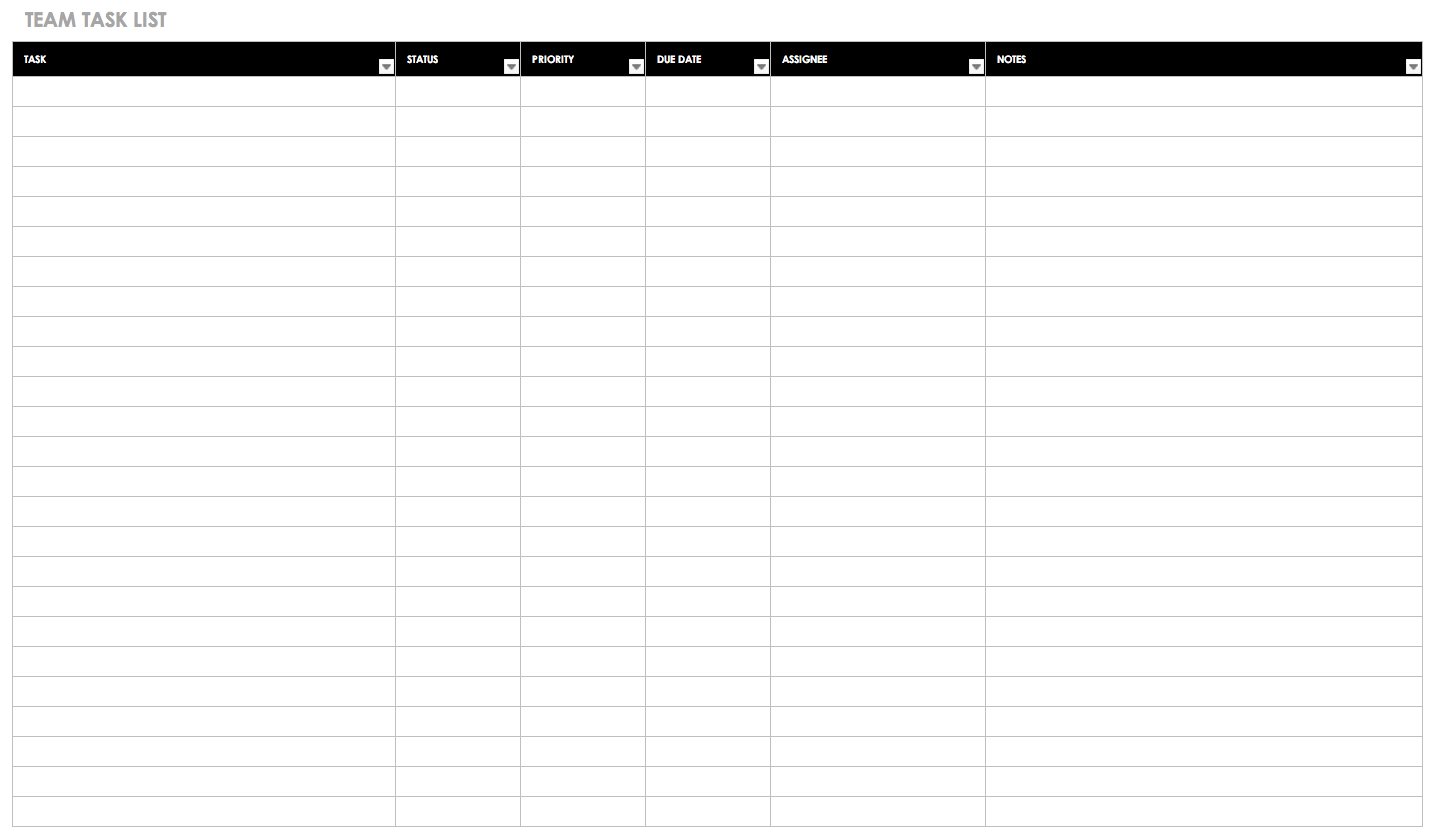
Download Excel Template Try Smartsheet Template
Gantt Chart Task List Template
Get a visual picture of your scheduled tasks with this Gantt chart template. Often used in project management, a Gantt chart shows the duration of each task as a horizontal bar that spans start and end dates. Thus, it’s easy to see the different phases of a project, identify dependencies, and prioritize tasks. A Gantt chart can be useful for managing any task list that spans a set period of time.
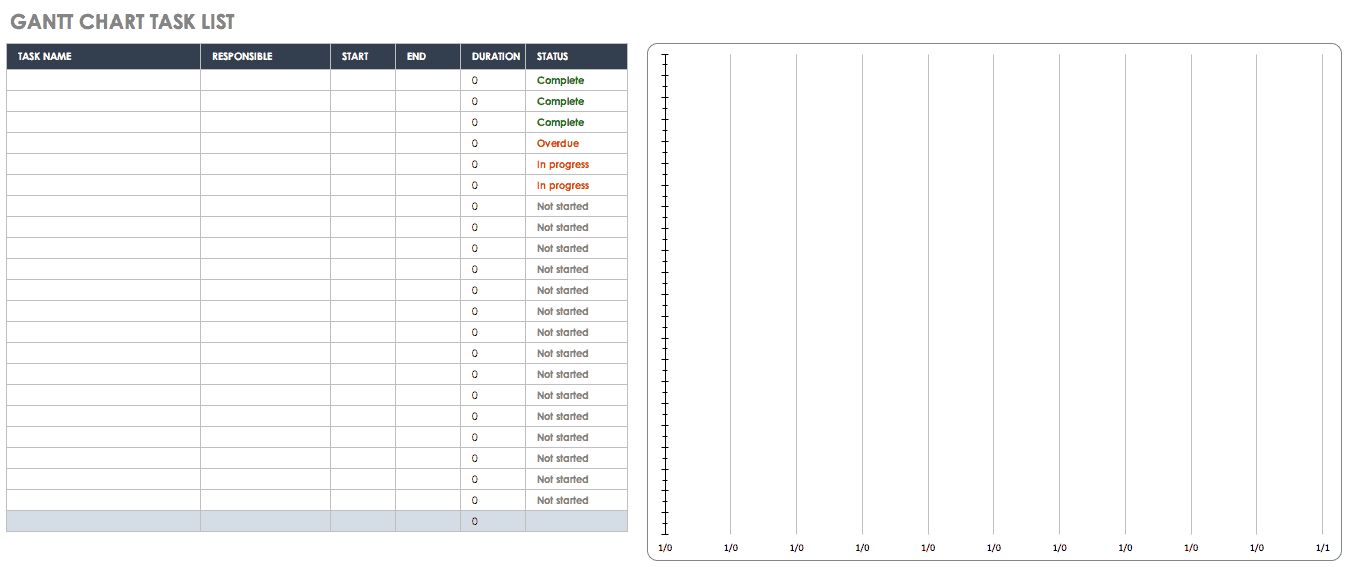
Task Tracker Template
This task tracking template documents the progress of each item on your list, so it’s easy to assess the status of individual tasks or an entire project. There is space to list start and end dates, as well as to mark completed tasks. This template keeps things simple by providing only essential tracking information, but there is also space for notes in case you need to include further details or instructions.
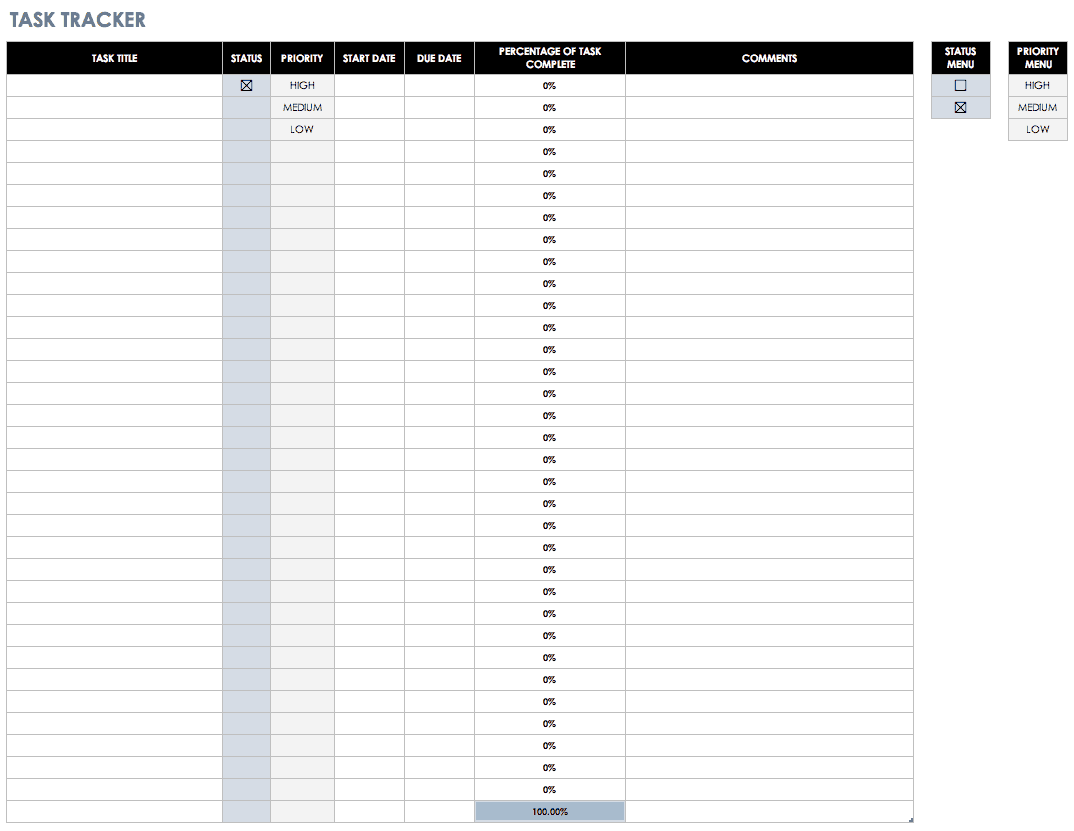
Project Task Template
This template is designed for project management, and it can be used in both a business or personal setting. The template provides sections for project tasks, priority, deadlines, assignees, and deliverables, and it allows you to track project costs and compare estimated to actual hours. This is a detailed template that can easily be edited to match the exact needs of your project.
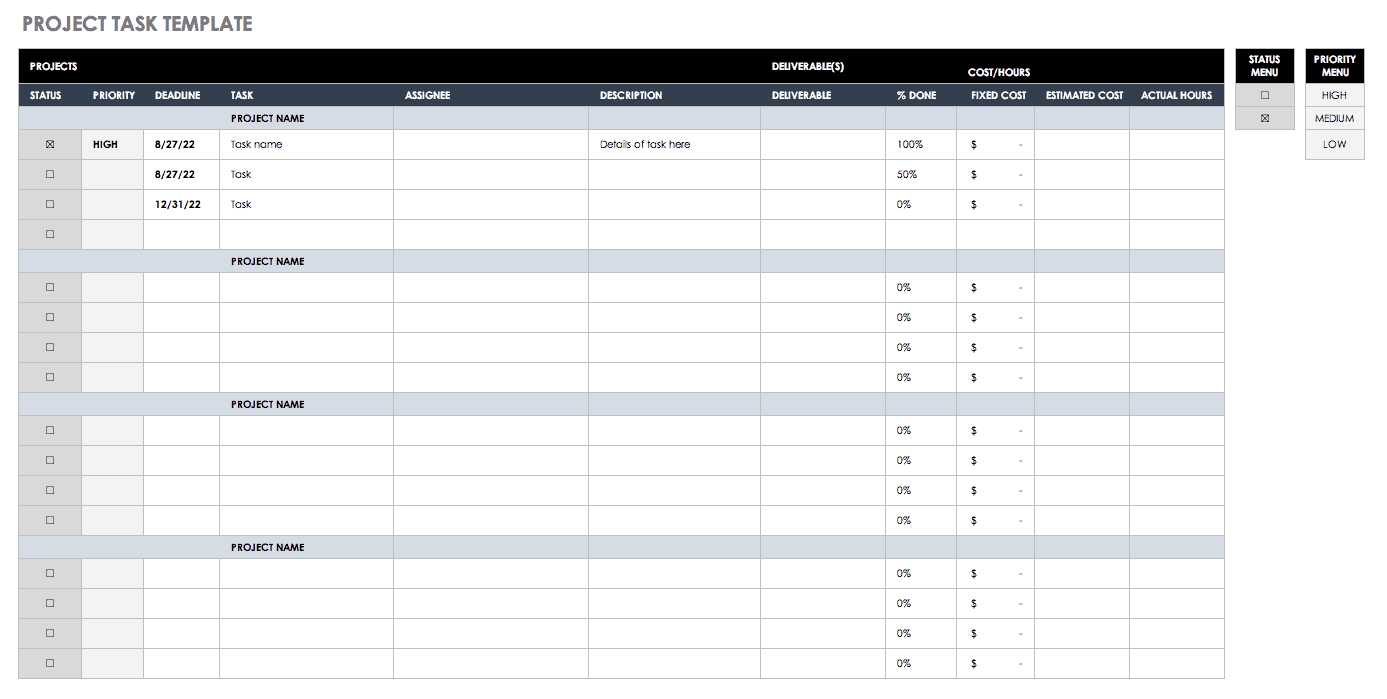
Weekly Task List Template
This weekly task list schedule includes columns for assigning a category to each item, along with deadlines and completion status. The default weekly calendar runs Sunday to Saturday, but you can also choose the starting date for the week. If you need a combined calendar and task list, this template offers an easy solution.
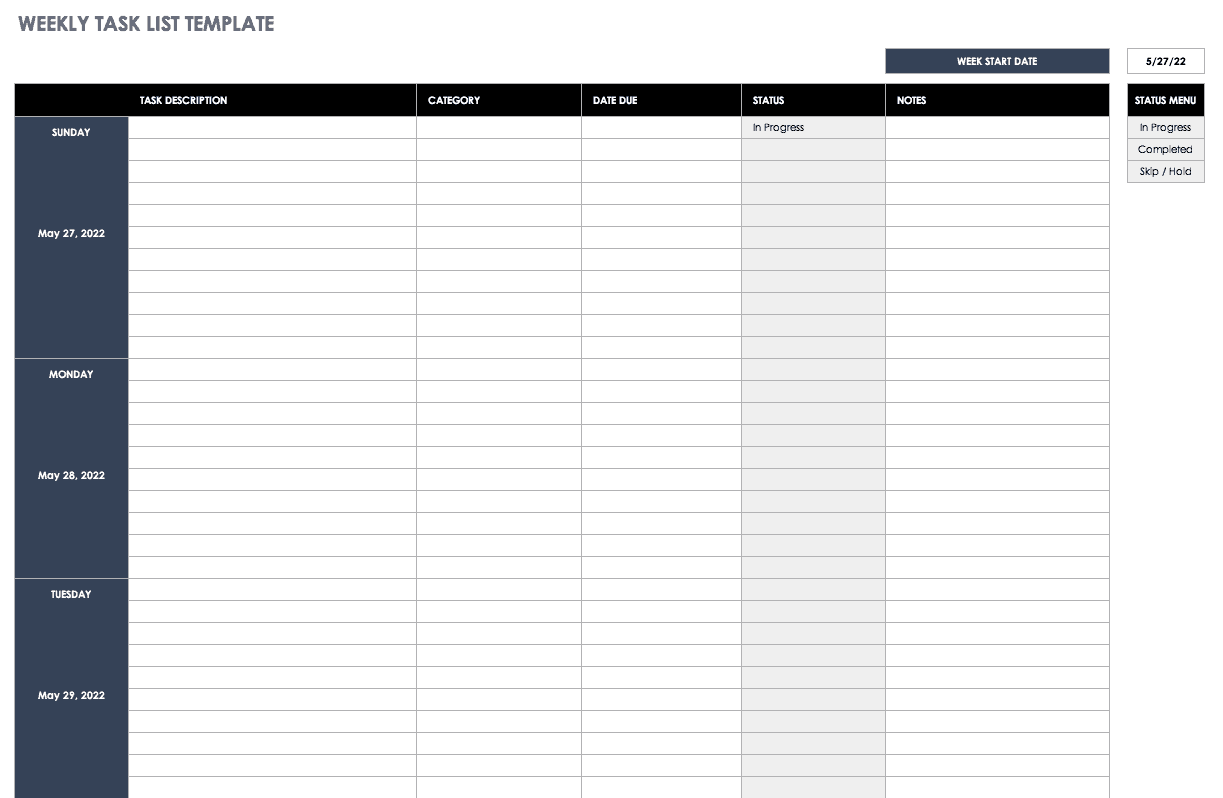
Daily Task List Template
You can use this daily task list template to schedule tasks throughout the day while also planning ahead for an entire week. Choose the starting time for each day, as well as the starting date for your weekly calendar view. You can also adjust the time interval of each task, which allows you to break down each hour into incremental tasks, if needed.
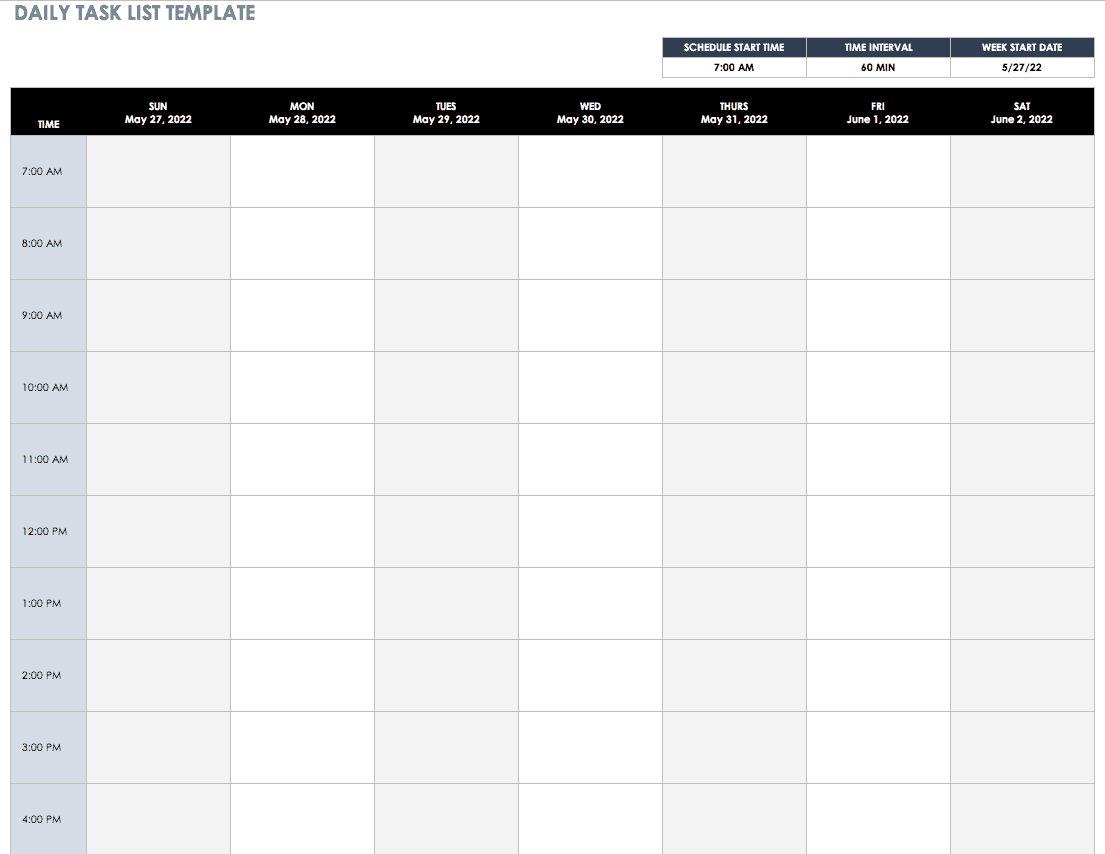
To-Do List with Drop-Down Menus Template
This simple to-do list template includes drop-down menus for indicating priority and status on each item. When a task is marked as complete, the row changes color; this enables you to quickly spot which tasks are still in progress or have yet to be started. This task template could be used for a broad range of applications, from organizing homework assignments to planning an event or tracking work projects.
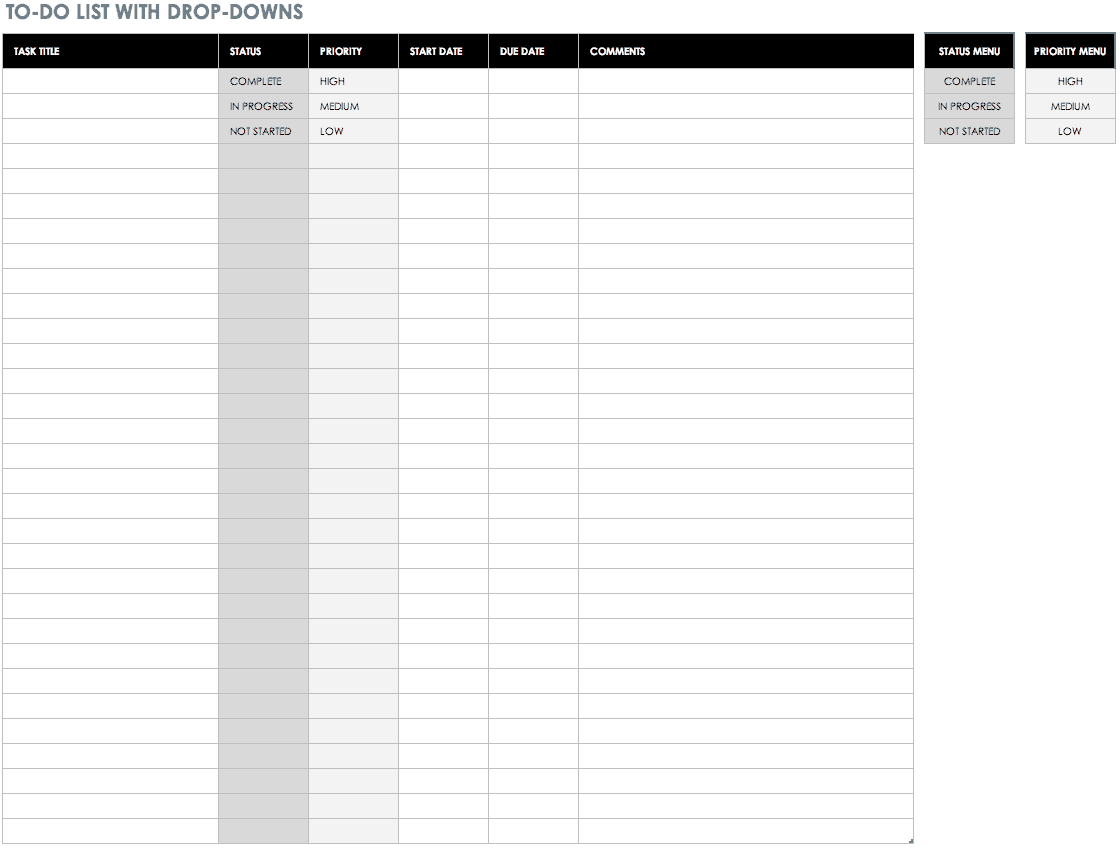

Prioritized Task List Template
This template is a step up from a basic to-do list, as it allows you to rank your tasks with the highest-priority items first. There is also a section for notes where you can elaborate on your tasks or add reminders. This template offers a simple way to stay organized while ensuring that you don’t overlook high-priority tasks.
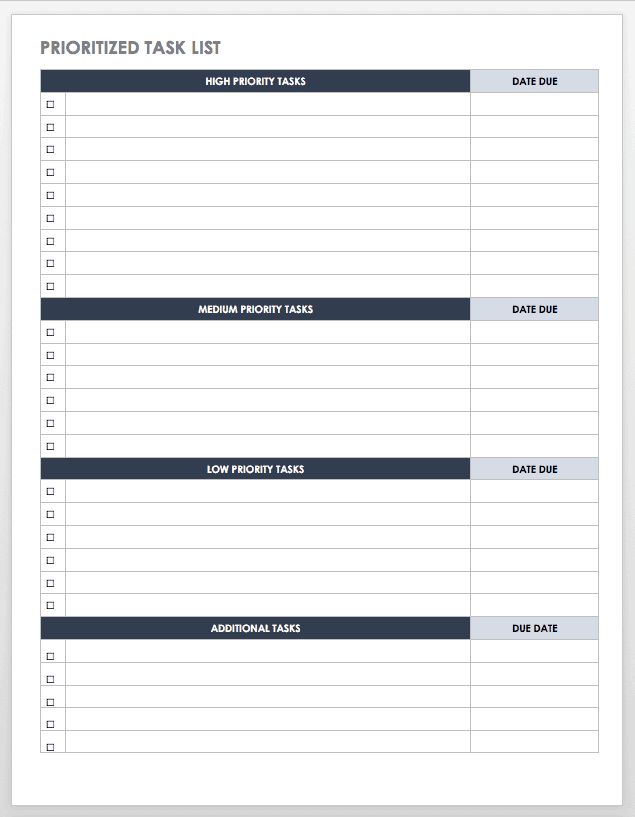
Download Word Template Try Smartsheet Template
Yearly Calendar Template (2024)
Use this 12-month calendar for a high-level look at your schedule and upcoming tasks. The template displays a standard January-to-December calendar, so you can view your long-term projects and tasks at a glance.
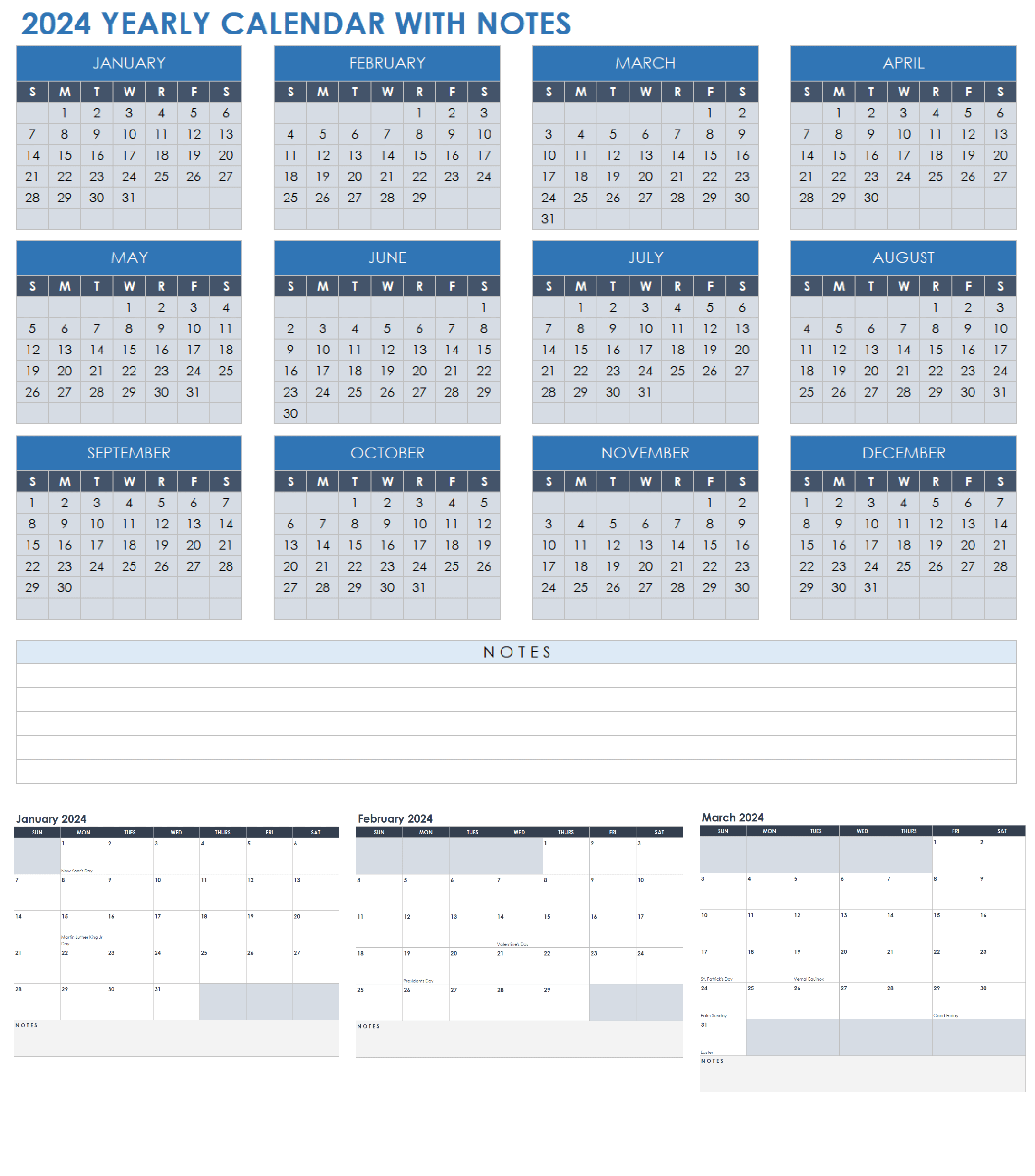
Download Excel Template
Basic Task Checklist Template
This task checklist offers a basic outline with checkboxes for each item on your to-do list. It also has sections to note the due date and status of each task to assist with planning. After downloading this template, save a blank version so that you have a master copy ready to print and use any time you need to create a new task checklist.
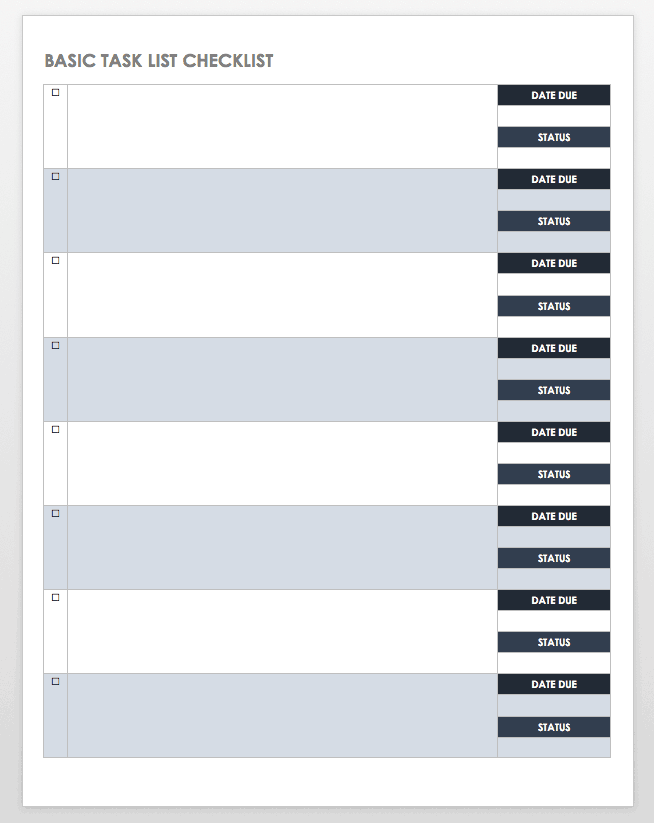
Download Word Template
Task Analysis Template
Use this task analysis template to determine the resources and time required to successfully complete a given task. The template includes sections for delineating the steps involved in a task, the resources (human, mechanical, or monetary) required for each step, and the time spent on each item. This provides a detailed analysis of a task, which can be useful for training purposes or for any situation where you need to evaluate a process.
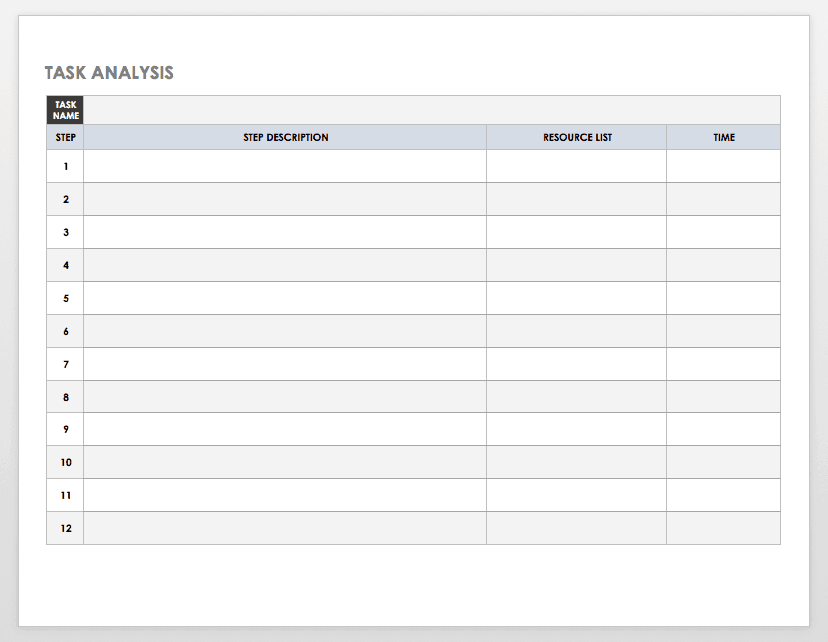
Action Item List Template
Help your team stay organized and on task with this action item list for Word. The template includes a tracking number for each action item, start and due dates, the assigned owner for each task, and a notes section for adding updates or resolving issues. By defining and scheduling tasks as actionable items, you can track individual and team progress — and in the process, create a useful communication tool for all team members.
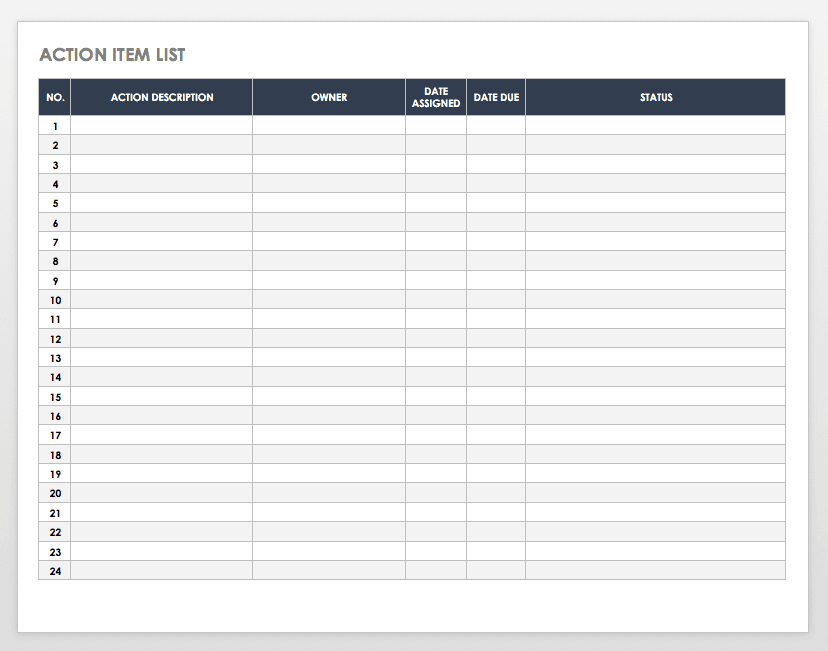
Download Template in Word Try Smartsheet Template
Password Log Template
This template is designed to help you keep track of the passwords to all of your various accounts. List each account, and then fill in the columns for website URL, email address, username and password, security questions and answers, and any additional notes. Save the form to your computer or print a hard copy — remember, though, to store the information in a safe place and and not to share your passwords with anyone.
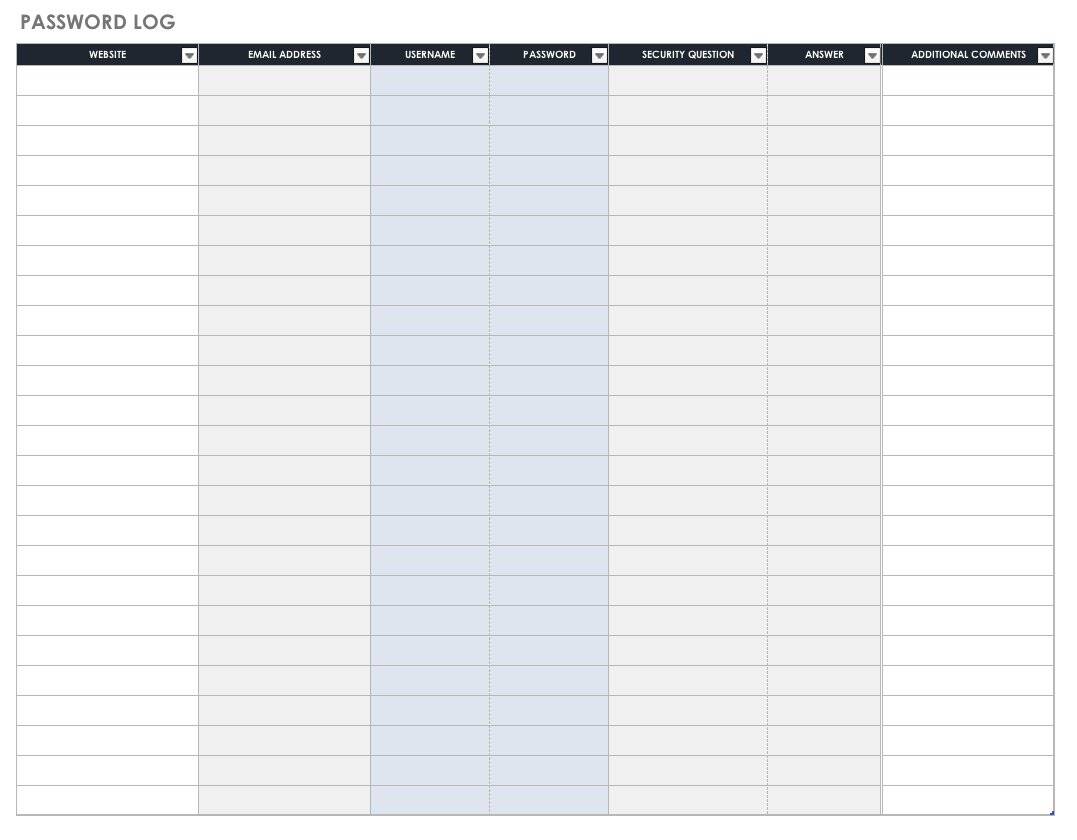
Download Password Log Template:
Excel | Word | PDF
Event To-Do List
This template can help you stay organized when planning an event, such as a dinner party, a baby or bridal shower, a wedding, or a birthday party. Simply list each task and the person responsible, and assign a priority level to the task so you and your team can prioritize the many moving pieces. Additionally, note the due date and date completed — any discrepancy between the two dates will help you more accurately plan the timeline for your next event.
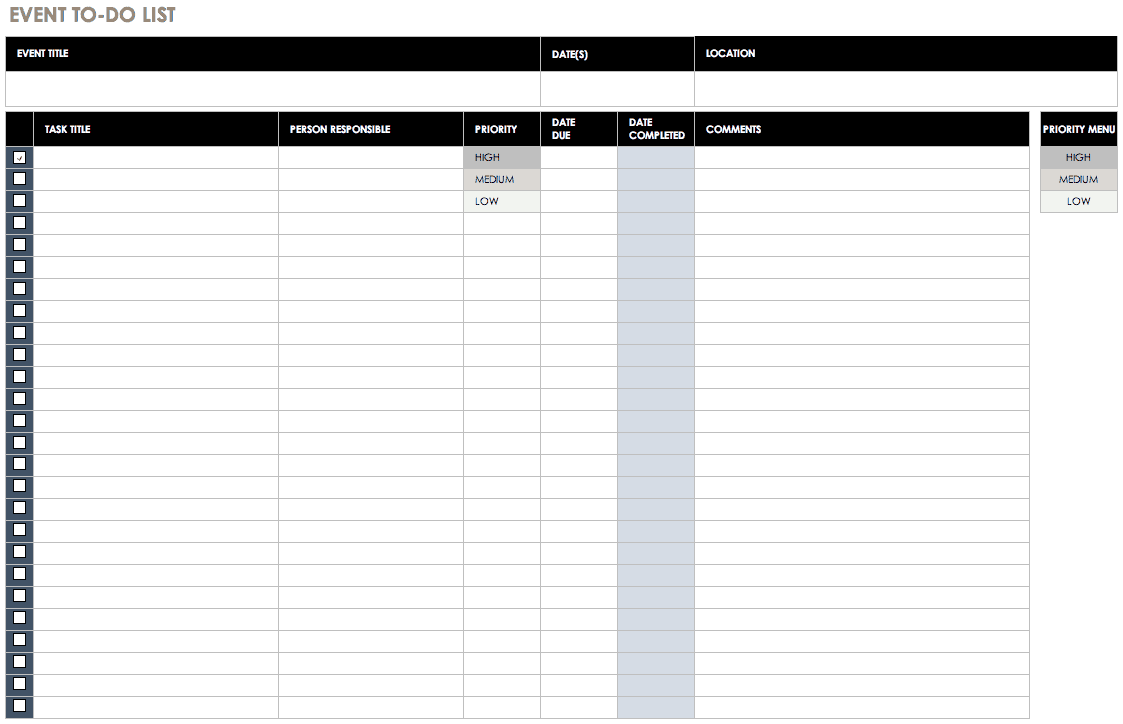
Family To-Do List
This template, available in Word and PDF, is ideal for families who want a central resource to organize family tasks. The template provides separate tables for each family member to list their individual tasks, and then register due dates and notes, along with a column to mark completion. Of course, you can add or delete tables as needed.
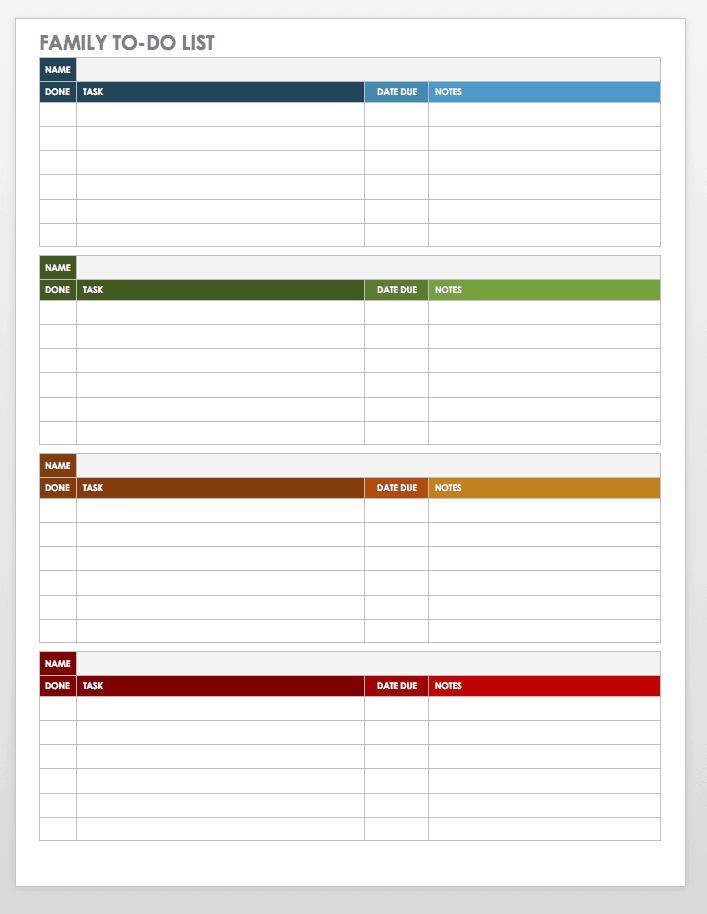
Download Family To-Do List:
Household Chores Task List Template
This chore list template allows you to create a weekly schedule for all of your household tasks. Create a list of tasks and assign each item to an individual for any day of the week. With a simple format, this template is easy to use, so you can streamline the planning process and start organizing your home. Additionally, you always have the option of saving the template as a PDF and printing a copy to share with others.
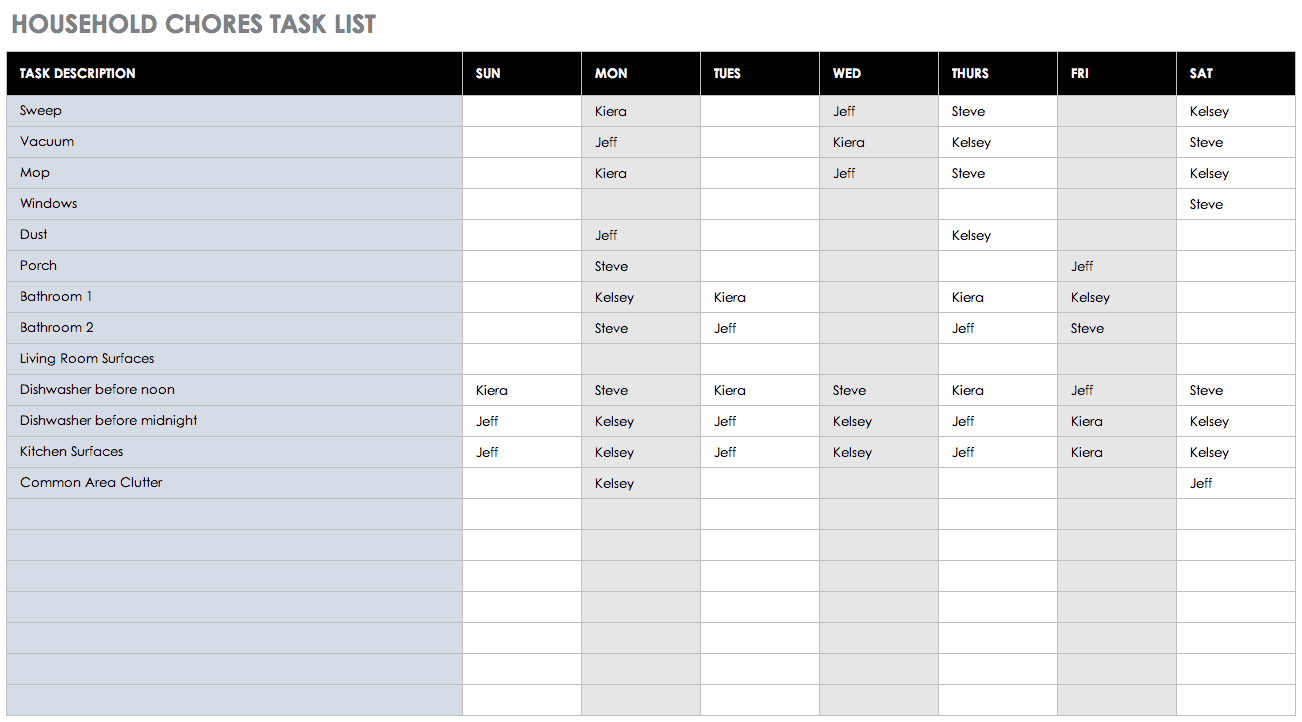
Project Punch List Form
In the construction industry, a punch list is a document that you complete toward the end of a project. In it, the contractor or property owner lists any work that has been done incorrectly or does not meet the specifications outlined in the original contract. The construction team then uses this punch list as a reference to complete or repair the work items before the project is officially closed. This punch list form provides a separate table to list each work item that needs repair or attention. Save the PDF form, print it, and fill it out by hand for easy use.
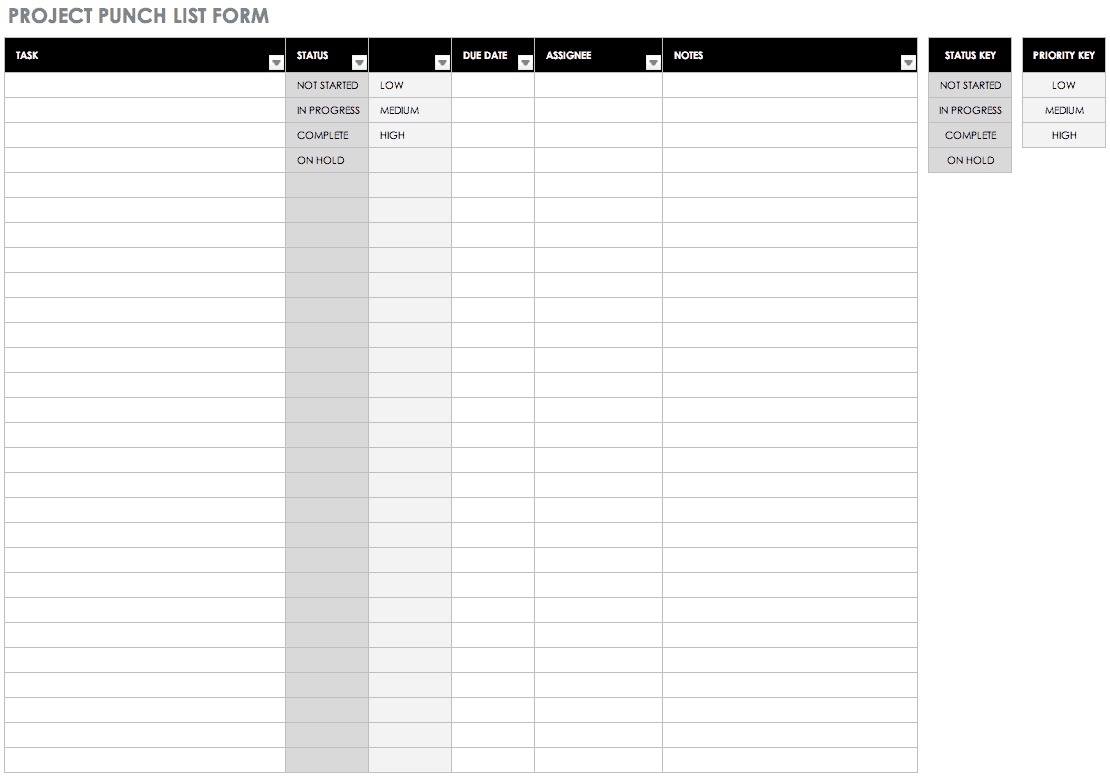
Project Closeout Template
Use this checklist to ensure you have completed all necessary tasks in a project. This template is designed with construction projects in mind, but you can edit the categories to reflect the needs of any multidimensional project, whether it pertains to building, real estate, or business — or even as a termination checklist if you are ending an association, an agreement, or a hiring arrangement. Simply list each task, the quantity requested (if applicable), specific requirements, and any notes. This easy-to-use form serves as a final check so you don’t overlook any requirements as you prepare to officially close a project.
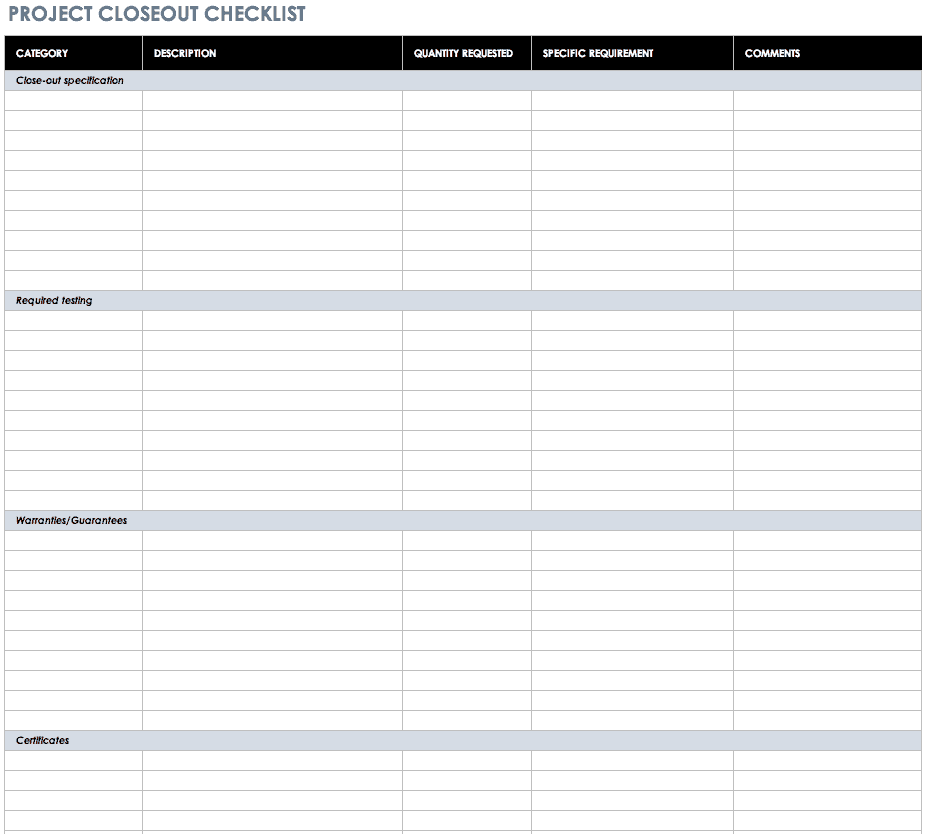
Risk Assessment Matrix
Before you embark on a project, you should perform a risk assessment. While you can’t control or prevent every possible risk, taking the time to assess the possible threats to your project will help you plan for and mitigate some hazards. This matrix allows you to perform a qualitative risk assessment, gauge the probability, and predict how each could affect your project budget, scope, and timeline. The template also provides space for you to list events that could trigger each risk, designate a respondee, and make notes for a response plan.
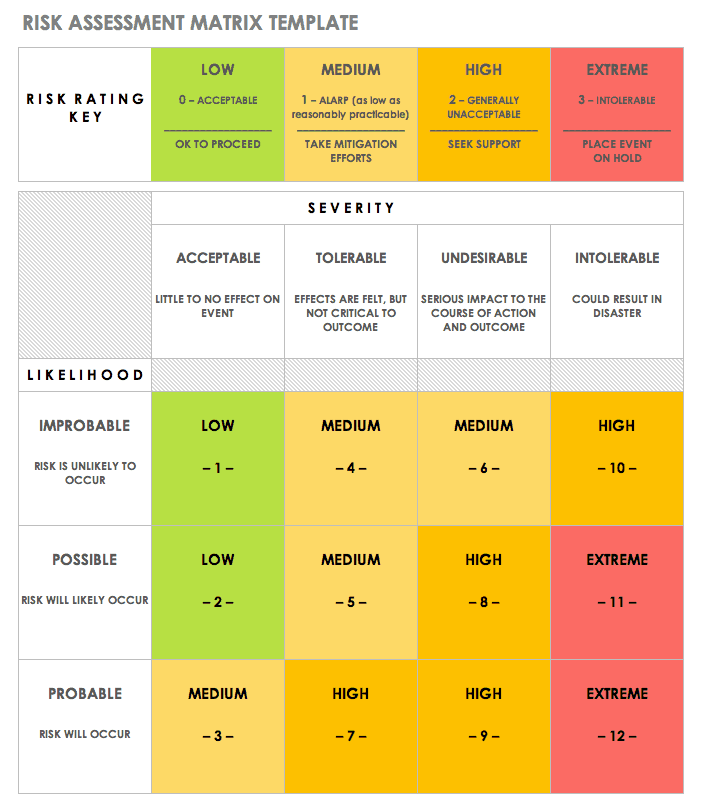
Download Risk Assessment Matrix:
Excel | Word | PDF | Smartsheet
Customer List
This simple form allows you to list contact details for each customer or client. This list includes columns for the company; the name, title, email, and phone number of your contact; and any comments. You can or remove columns as necessary, and highlight or flag certain customers who you need to follow up with.
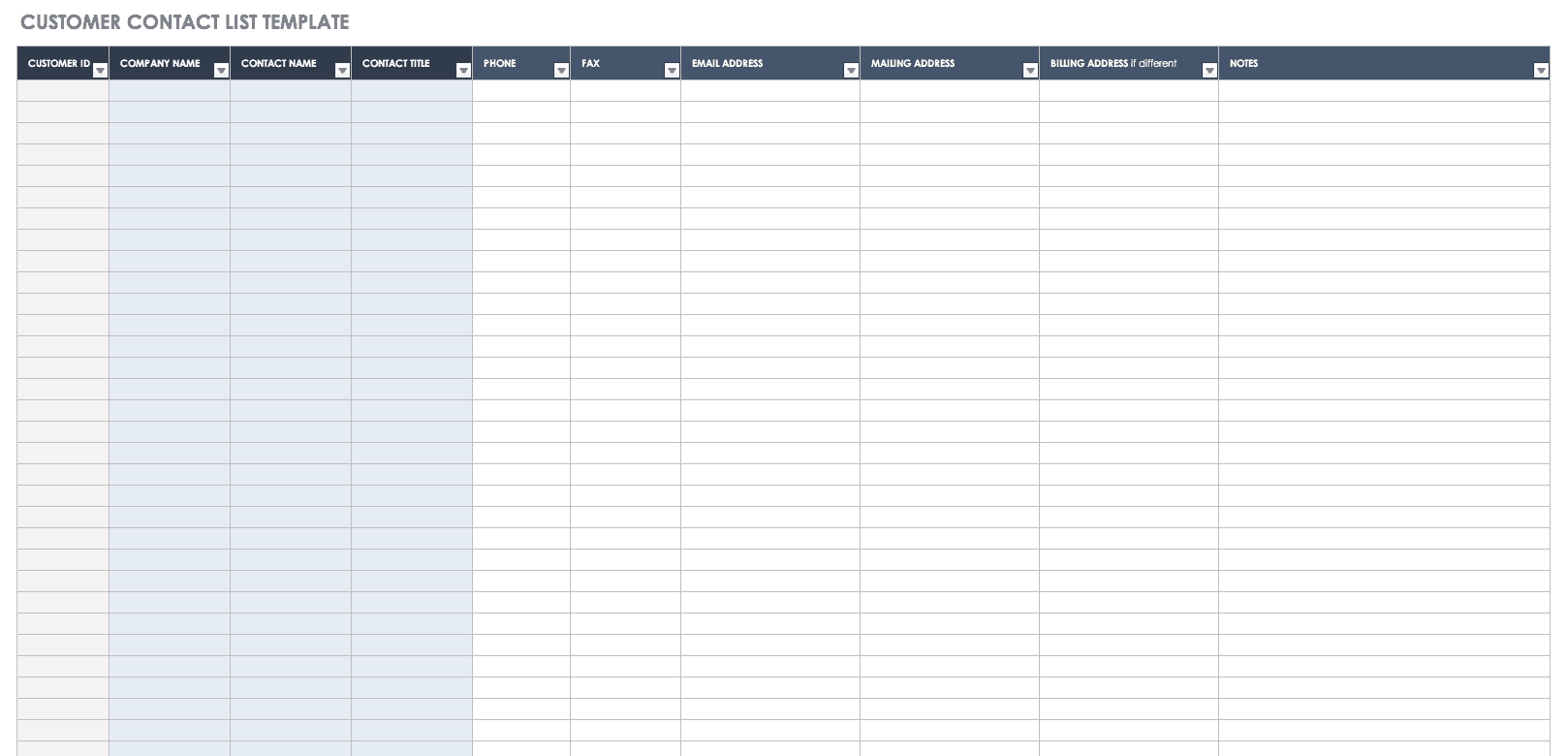
Inventory and Equipment Checklist
Use this template to keep track of inventory or equipment you either need or have newly acquired for a project. List each item number and name, followed by a description, date of purchase, cost, and other notes. Additionally, you can track initial value, loan details, and depreciation amounts for each piece of equipment in your inventory.
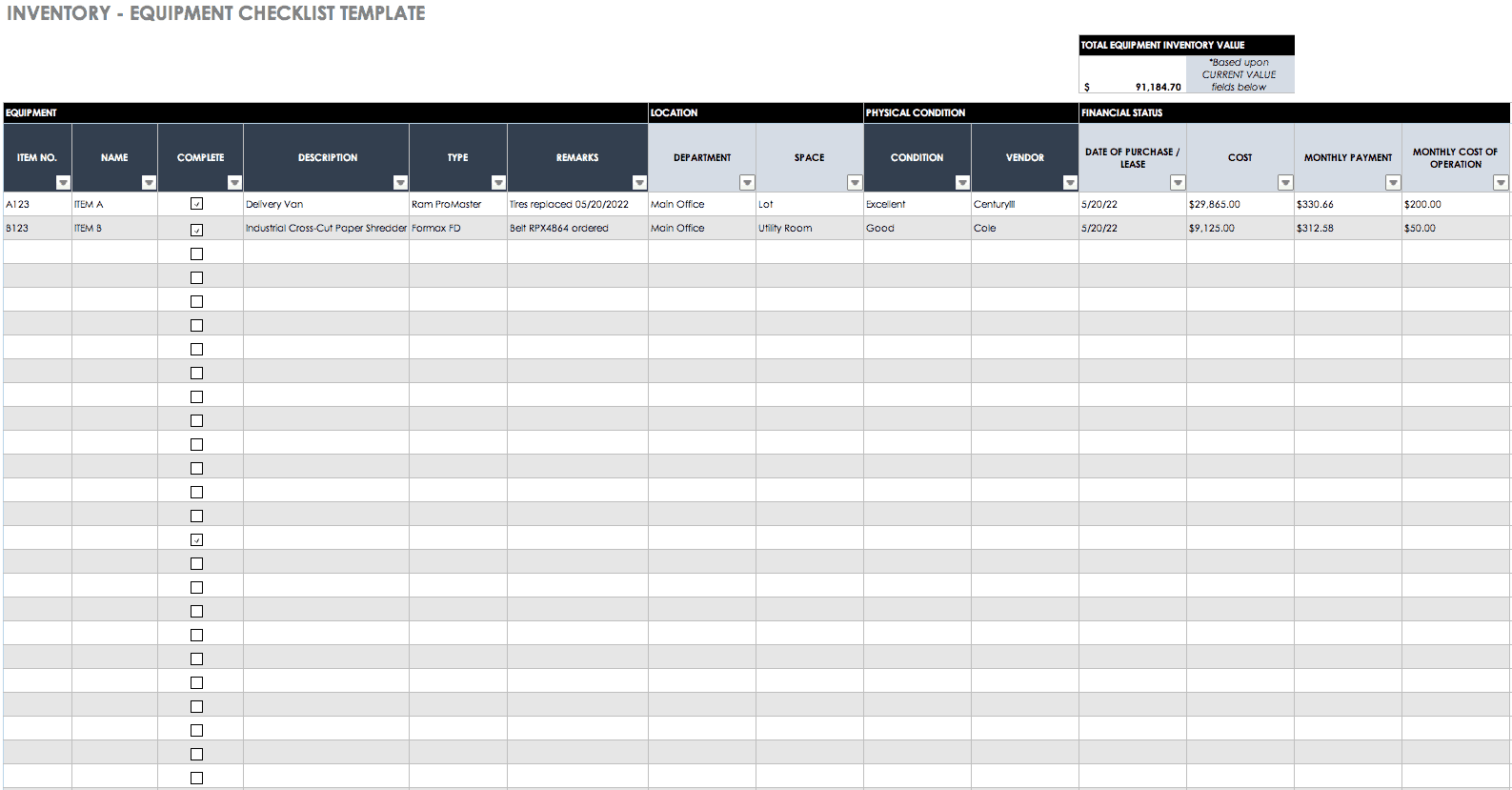
New Hire Checklist
This template is designed for human resources to ensure that a new employee (and other internal teams) complete all necessary onboarding tasks. The Excel spreadsheet includes columns for completing new hire paperwork and sending it to the appropriate parties, but you can edit the form to include any tasks that your organization requires of new hires. In addition, there is space to assign tasks to employees, to set due dates, and to list contact information.
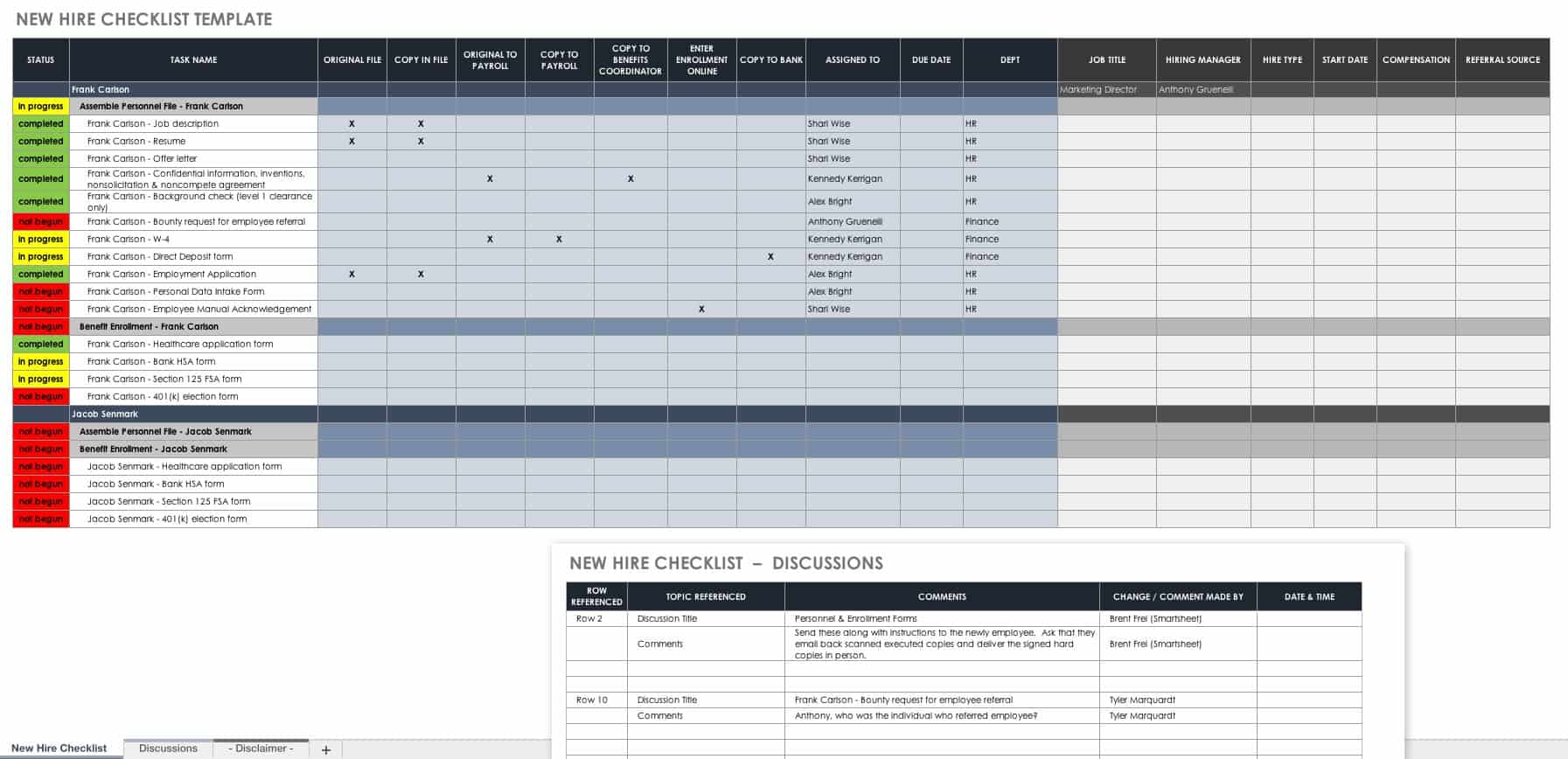
Grocery List
Use this basic template to make a shopping list; you can fill it out on your computer or print and complete it by hand. In addition to providing an easy-to-read table, the template includes a checkbox column where you can mark off each item as you obtain it, as well as a column for individual items and total cost. This template is created with grocery shopping in mind, but you can edit it for retail, gifts, and other consumer needs.
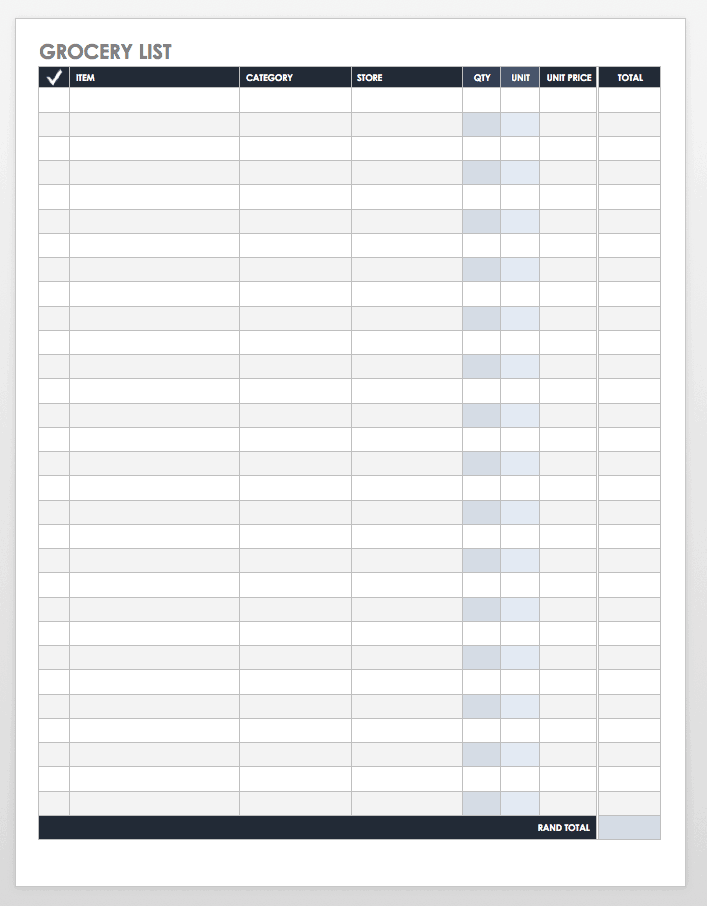
Download Grocery List:
Weekly Staff Meeting Template
Use this template to prepare for a recurring meeting. Note your agenda, attendees, action items, and whether or not the associated tasks have been completed. The template is designed with weekly meetings in mind, but you can edit the dates for a monthly, quarterly, or annual gathering.
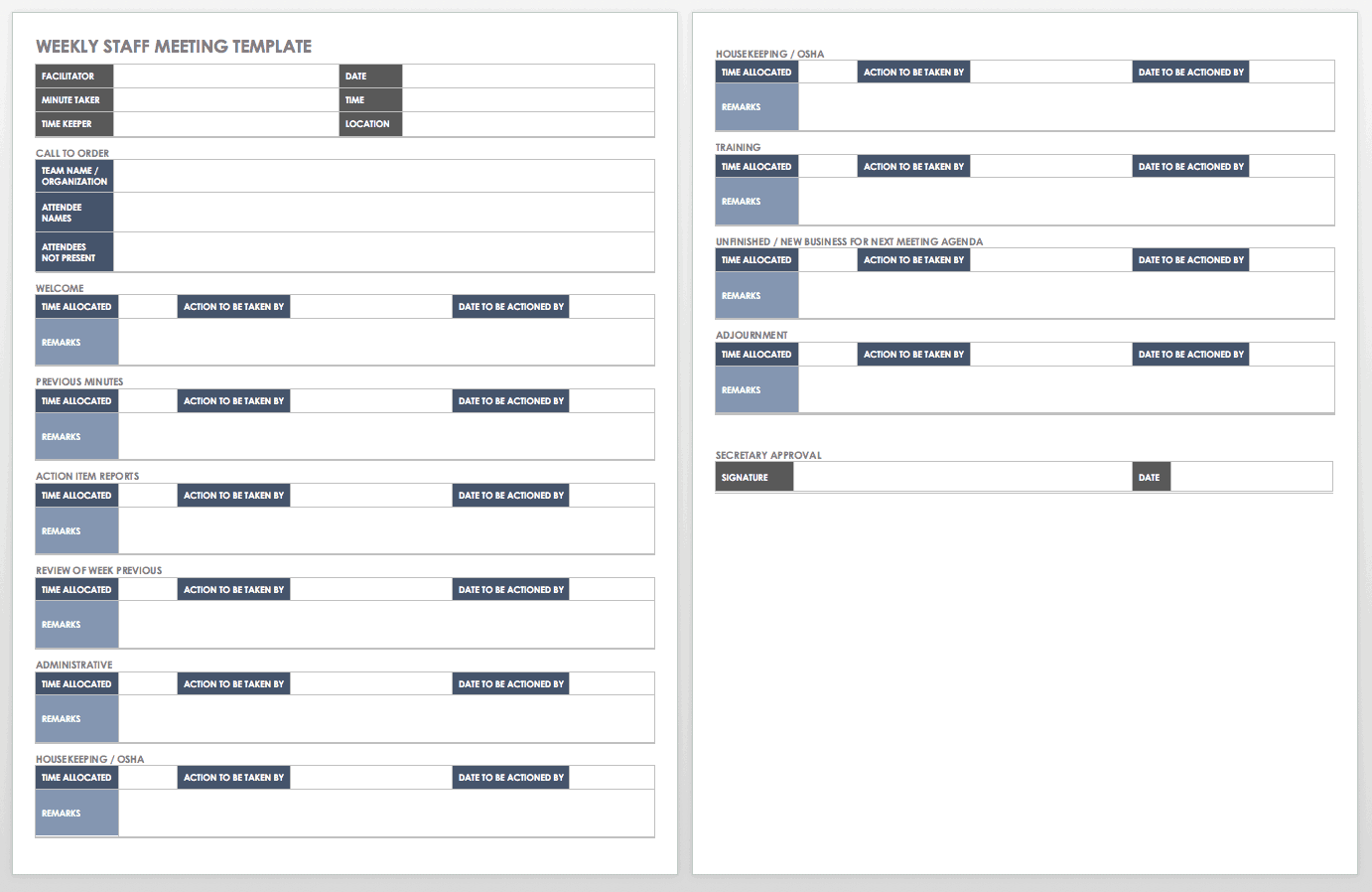
Business Trip Checklist Template
Preparing for a business trip may include managing staff adjustments at work, making schedule changes with family members or caregivers at home, and taking on extra work to prepare for the trip — plus, packing everything you’ll need on the road. Use this business trip checklist template to help make the process more manageable and reduce the likelihood of forgetting a vital task or travel item. Edit the template to reflect your agenda, and then mark each item off your checklist as it’s completed. For personal use, you can also edit this template with relevant details for solo, family, or group travel.
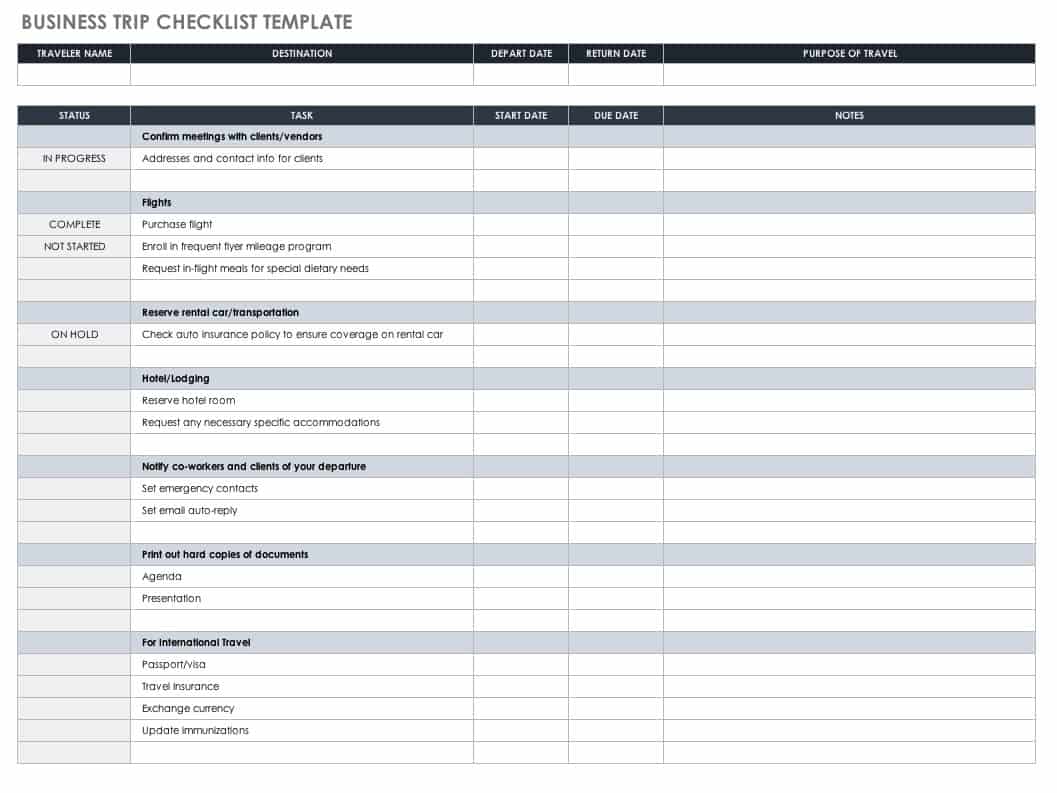
Download Excel Template Try Smartsheet Template
Student Planner Template
Students can plan for the week by using this free template to keep track of classes and assignments. The template includes columns for listing the due date and status of each assignment, which helps students stay organized, prioritize their workload, and meet deadlines with less stress.
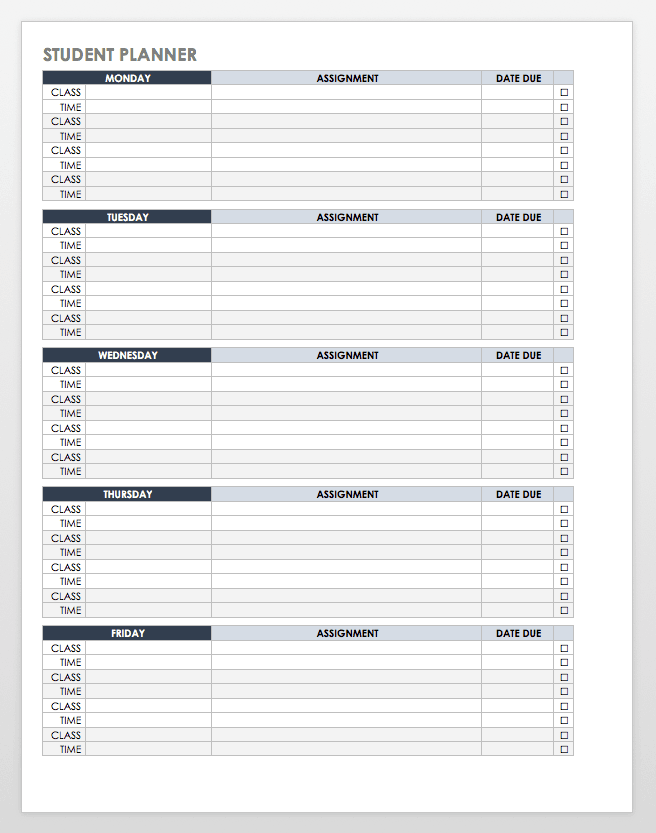
Day Planner Template
This detailed day planner template provides multiple sections for planning various aspects of your day, from shopping needs to meals and appointments. There is also room for a general task list and a section to add items to be accomplished on another day. Customize the template by applying new labels to sections to match your daily routines.
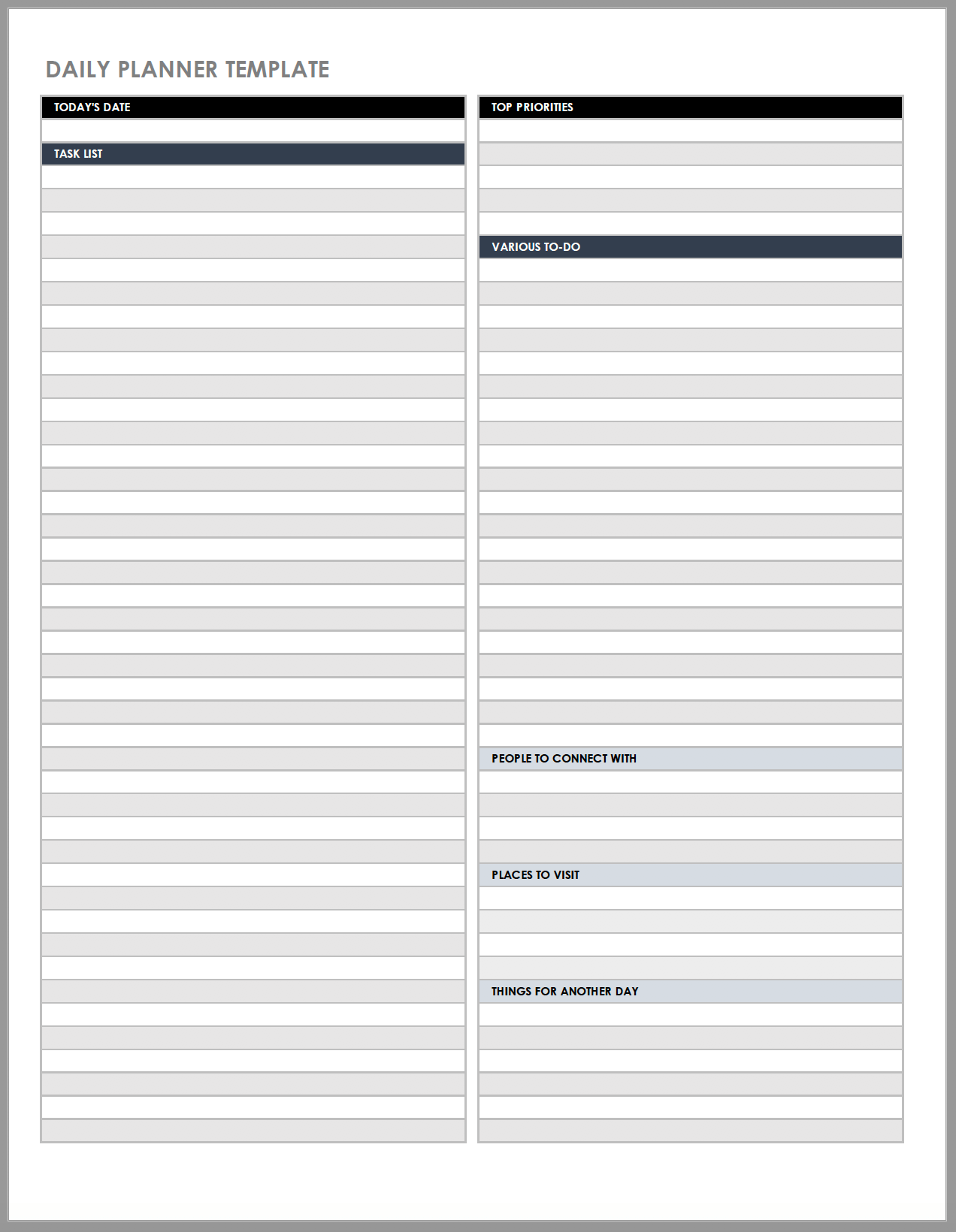
Business Plan Checklist
Use this simple business plan template to organize project tasks. The template is divided into phases so you can list tasks chronologically; it also includes columns for start and end dates and duration. Plus, a simple checkbox allows you to clearly mark which steps have been completed so you know your exact progress.
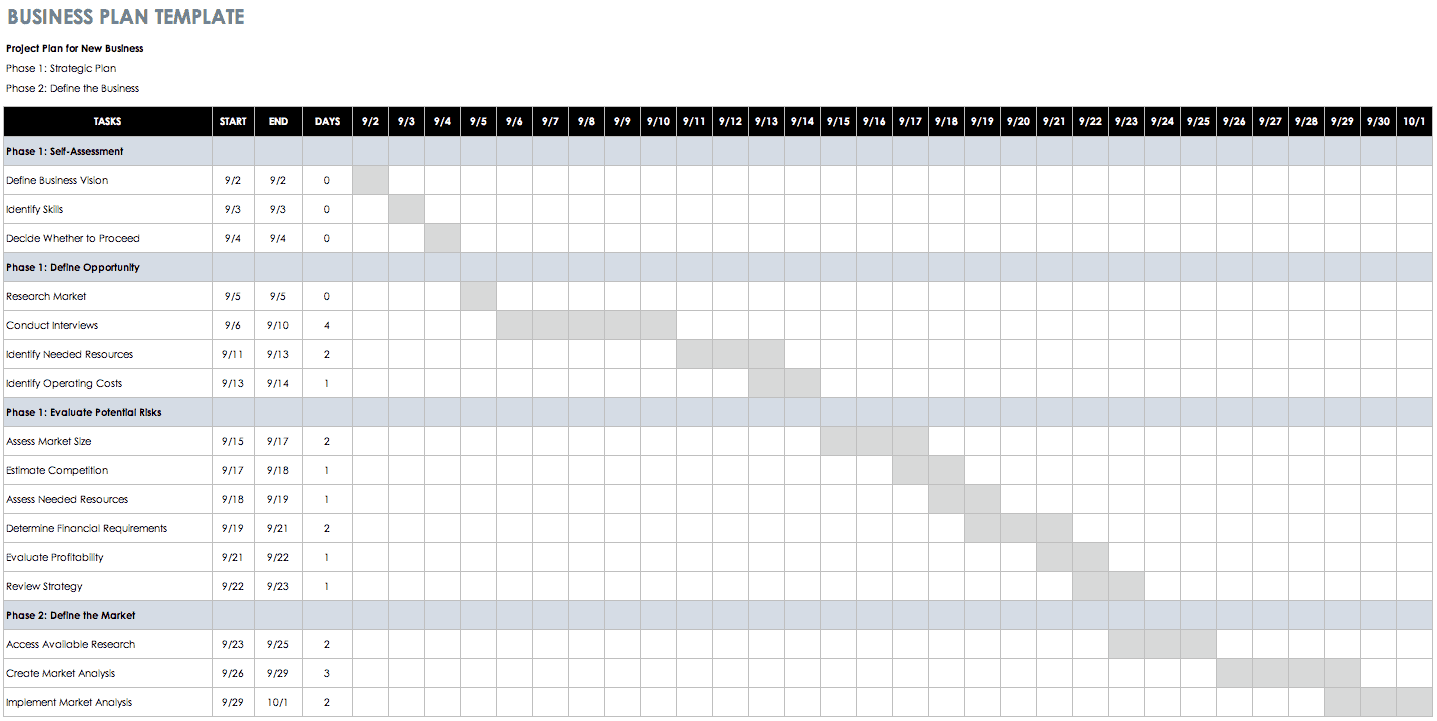
Group Project Task List
This template is ideal for organizing the basics of a group project or any assignment that involves multiple people. Simply list each task, a description, an assignee, a due date, and any notes in the columns provided, and add more columns if needed. This template does not have project management capabilities, but will give you an overview of every individual task and who is responsible for it. For more full-scale project management templates, visit “ Top Project Management Excel Templates .”
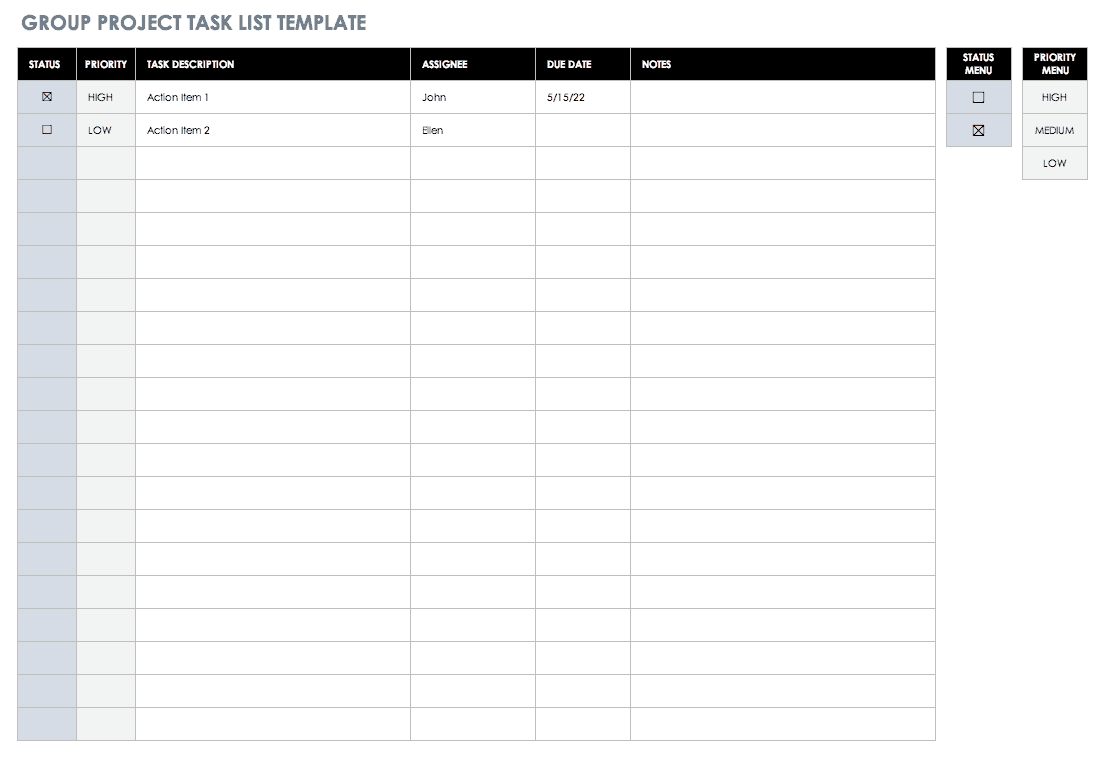
Job Task Analysis Template
A job task analysis can be used to determine which actions are critical for a certain job. Identifying tasks in this manner can help determine the scope of a job, appraise employee performance, inform training methods, and improve work processes. On this template, you can list the tasks that are required to complete a job, then rate the importance of each task, along with how frequently the actions are performed. You can also document your source of information for each task.
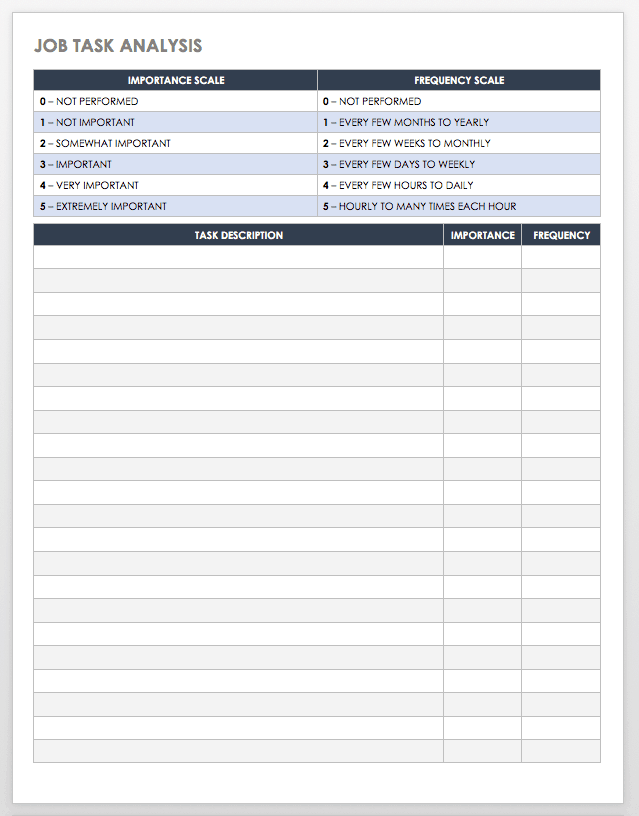
Download Word Template
Brainstorm and Collaboration Worksheet
Use this form to record information from a brainstorm or collaboration session. The template includes space to list ideas, their pros and cons, and the originator. In addition, there is a column for each team member to rank each idea. While this template doesn’t assist with idea generation, it enables you to keep all brainstorm information in one place so you can more easily compare ideas when it’s time to make a decision.
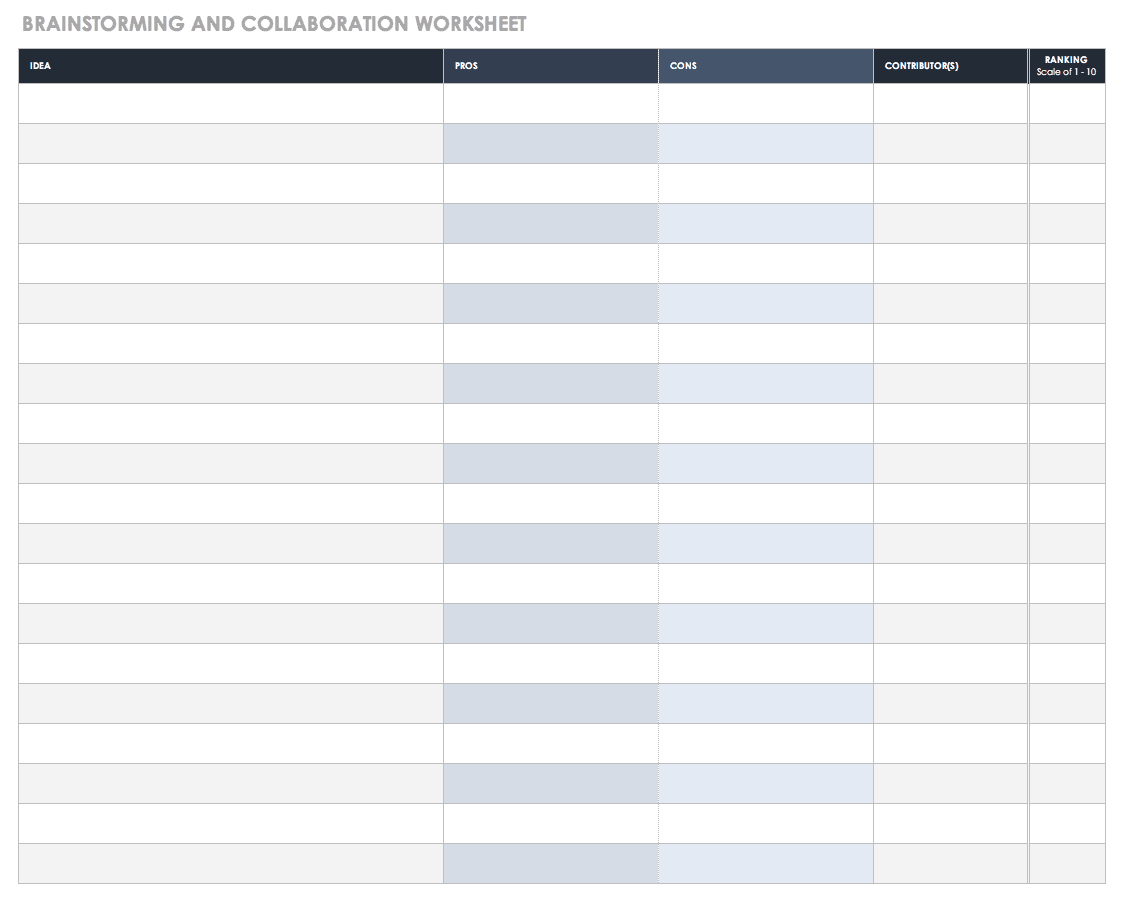
Download Brainstorm and Collaboration Worksheet:
Internal Audit Checklist
Use this template to prepare for a financial audit. The simple template includes rows for every required document in a standard audit (general ledger, balance and financial statements, tax reports, etc.) and a checkbox to note if an item has been reviewed and is attached. Add or subtract rows to include every document that your audit requires. To learn more about how best to prepare for a financial audit, read this article .
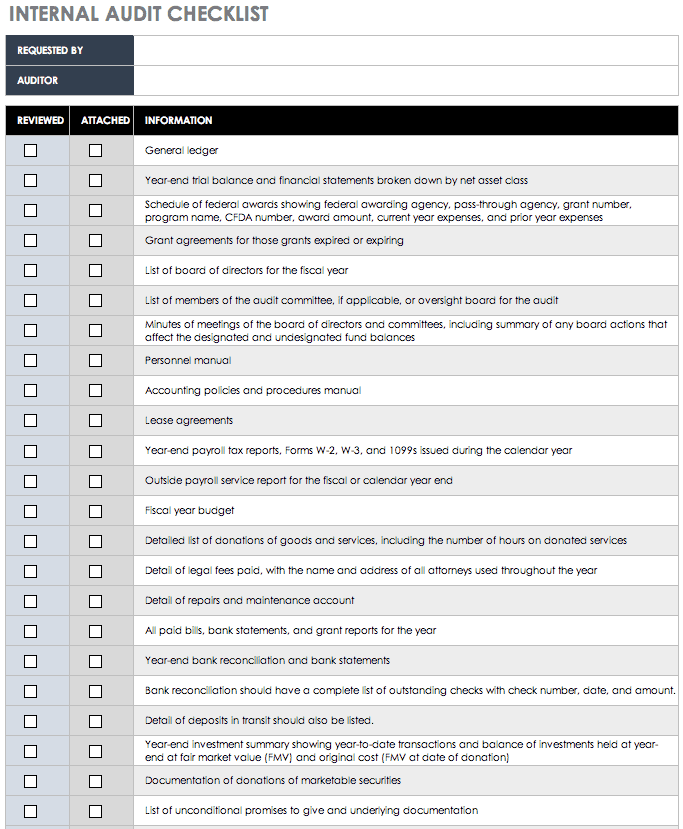
Contact List
This Excel template can be used as a contact list for a variety of personal use cases, including for classes, group memberships, event attendees, or emergency communications. The template includes columns for phone, email, and address, as well as to note the preferred mode of contact. The form is fully customizable, so add or subtract sections as needed.
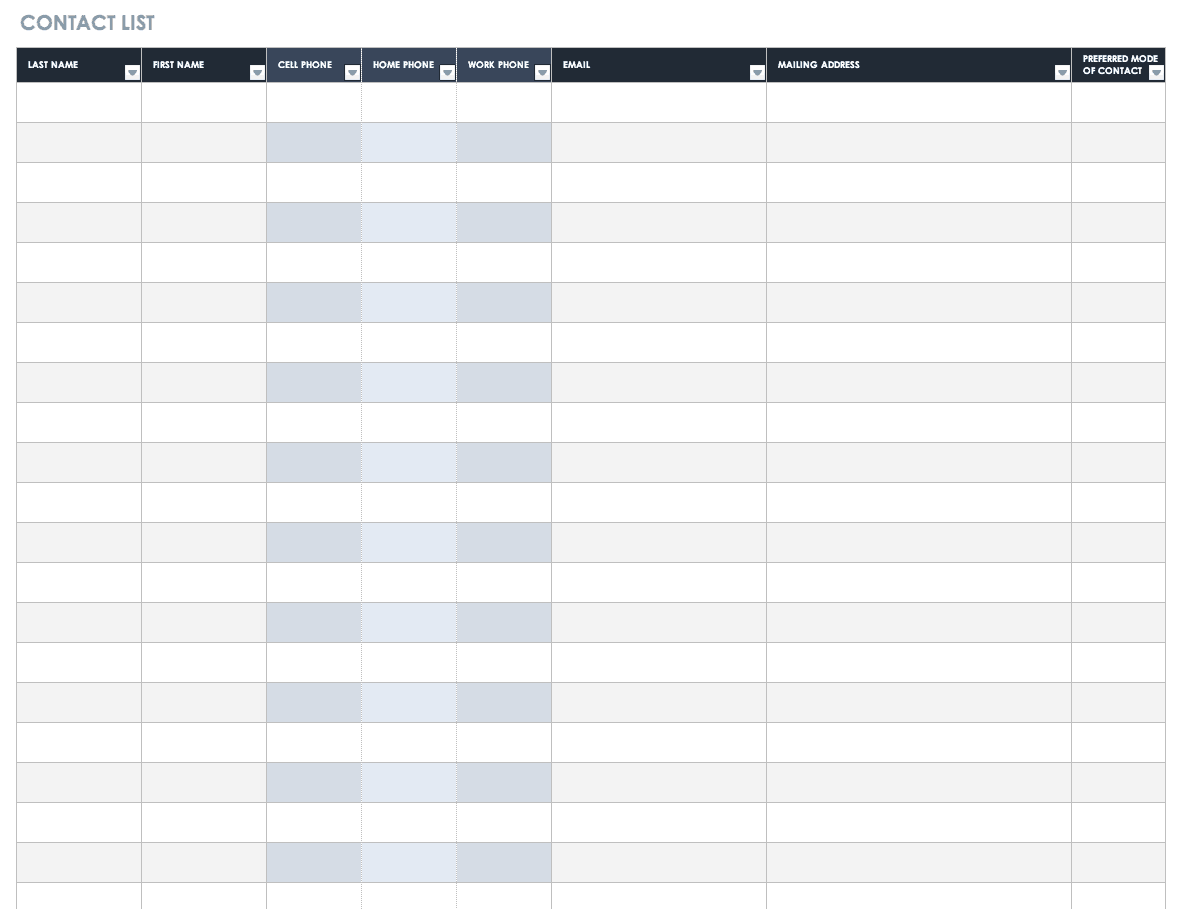
Download Contact List:
Increase Accountability with Real-Time Task Management in Smartsheet
Empower your people to go above and beyond with a flexible platform designed to match the needs of your team — and adapt as those needs change.
The Smartsheet platform makes it easy to plan, capture, manage, and report on work from anywhere, helping your team be more effective and get more done. Report on key metrics and get real-time visibility into work as it happens with roll-up reports, dashboards, and automated workflows built to keep your team connected and informed.
When teams have clarity into the work getting done, there’s no telling how much more they can accomplish in the same amount of time. Try Smartsheet for free, today.
Our Privacy Notice describes how we process your personal data.
Discover why over 90% of Fortune 100 companies trust Smartsheet to get work done.
- Link to facebook
- Link to linkedin
- Link to twitter
- Link to youtube
- Writing Tips
Assignment Tracker Template For Students (Google Sheets)
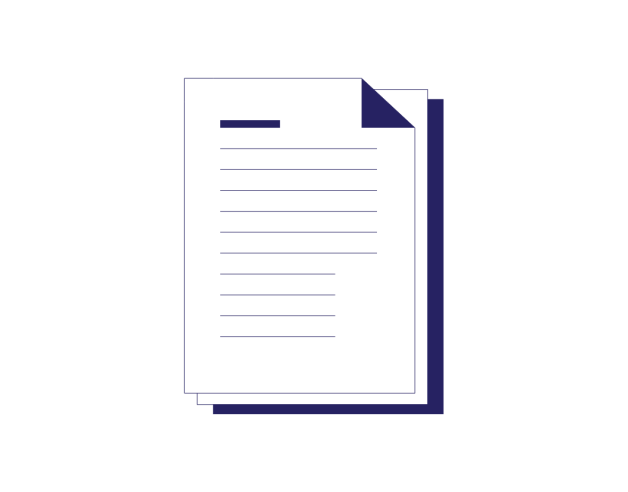
- 6-minute read
- 18th May 2023
If you’re a student searching for a way to keep your assignments organized, congratulate yourself for taking the time to set yourself up for success. Tracking your assignments is one of the most important steps you can take to help you stay on top of your schoolwork .
In this Writing Tips blog post, we’ll discuss why keeping an inventory of your assignments is important, go over a few popular ways to do so, and introduce you to our student assignment tracker, which is free for you to use.
Why Tracking Is Important
Keeping your assignments organized is essential for many reasons. First off, tracking your assignments enables you to keep abreast of deadlines. In addition to risking late submission penalties that may result in low grades, meeting deadlines can help develop your work ethic and increase productivity. Staying ahead of your deadlines also helps lower stress levels and promote a healthy study-life balance.
Second, keeping track of your assignments assists with time management by helping prioritize the order you complete your projects.
Third, keeping a list of your completed projects can help you stay motivated by recording your progress and seeing how far you’ve come.
Different Ways to Organize Your Assignments
There are many ways to organize your assignment, each with its pros and cons. Here are a few tried and true methods:
- Sticky notes
Whether they are online or in real life , sticky notes are one of the most popular ways to bring attention to an important reminder. Sticky notes are a quick, easy, and effective tool to highlight time-sensitive reminders. However, they work best when used temporarily and sparingly and, therefore, are likely better used for the occasional can’t-miss deadline rather than for comprehensive assignment organization.
- Phone calendar reminders
The use of cell phone calendar reminders is also a useful approach to alert you to an upcoming deadline. An advantage to this method is that reminders on your mobile device have a good chance of grabbing your attention no matter what activity you’re involved with.
On the downside, depending on how many assignments you’re juggling, too many notifications might be overwhelming and there won’t be as much space to log the details of the assignment (e.g., related textbook pages, length requirements) as you would have in a dedicated assignment tracking system.
- Planners/apps
There are a multitude of physical planners and organization apps for students to help manage assignments and deadlines. Although some vow that physical planners reign superior and even increase focus and concentration , there is almost always a financial cost involved and the added necessity to carry around a sometimes weighty object (as well as remembering to bring it along with you).
Mobile organization apps come with a variety of features, including notifications sent to your phone, but may also require a financial investment (at least for the premium features) and generally will not provide substantial space to add details about your assignments.
- Spreadsheets
With spreadsheets, what you lose in bells and whistles, you gain in straightforwardness and customizability – and they’re often free! Spreadsheets are easy to access from your laptop or phone and can provide you with enough space to include whatever information you need to complete your assignments.
There are templates available online for several different spreadsheet programs, or you can use our student assignment tracker for Google Sheets . We’ll show you how to use it in the next section.
How to Use Our Free Writing Tips Student Assignment Tracker
Follow this step-by-step guide to use our student assignment tracker for Google Sheets :
- Click on this link to the student assignment tracker . After the prompt “Would you like to make a copy of Assignment Tracker Template ?”, click Make a copy .
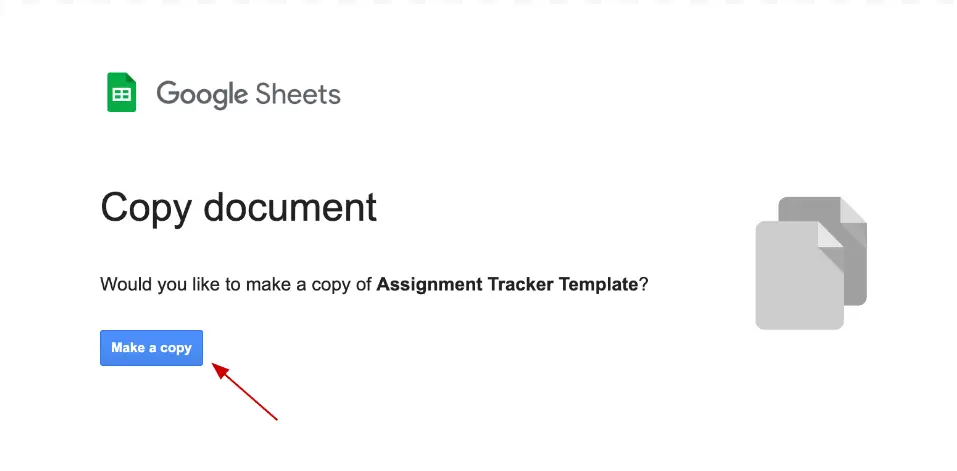
Screenshot of the “Copy document” screen
Find this useful?
Subscribe to our newsletter and get writing tips from our editors straight to your inbox.
2. The first tab in the spreadsheet will display several premade assignment trackers for individual subjects with the name of the subject in the header (e.g., Subject 1, Subject 2). In each header, fill in the title of the subjects you would like to track assignments for. Copy and paste additional assignment tracker boxes for any other subjects you’d like to track, and color code the labels.
Screenshot of the blank assignment template
3. Under each subject header, there are columns labeled for each assignment (e.g., Assignment A, Assignment B). Fill in the title of each of your assignments in one of these columns, and add additional columns if need be. Directly under the assignment title is a cell for you to fill in the due date for the assignment. Below the due date, fill in each task that needs to be accomplished to complete the assignment. In the final row of the tracker, you should select whether the status of your assignment is Not Started , In Progress , or Complete . Please see the example of a template that has been filled in (which is also available for viewing in the Example tab of the spreadsheet):
Example of completed assignment tracker
4. Finally, for an overview of all the assignments you have for each subject throughout the semester, fill out the assignment tracker in the Study Schedule tab. In this tracker, list the title of the assignment for each subject under the Assignment column, and then color code the weeks you plan to be working on each one. Add any additional columns or rows that you need. This overview is particularly helpful for time management throughout the semester.
There you have it.
To help you take full advantage of this student assignment tracker let’s recap the steps:
1. Make a copy of the student assignment tracker .
2. Fill in the title of the subjects you would like to track assignments for in each header row in the Assignments tab.
3. Fill in the title of each of your assignments and all the required tasks underneath each assignment.
4. List the title of the assignment for each subject and color code the week that the assignment is due in the Study Schedule .
Now that your assignments are organized, you can rest easy . Happy studying! And remember, if you need help from a subject-matter expert to proofread your work before submission, we’ll happily proofread it for free .
Share this article:
Post A New Comment
Got content that needs a quick turnaround? Let us polish your work. Explore our editorial business services.
2-minute read
How to Cite the CDC in APA
If you’re writing about health issues, you might need to reference the Centers for Disease...
5-minute read
Six Product Description Generator Tools for Your Product Copy
Introduction If you’re involved with ecommerce, you’re likely familiar with the often painstaking process of...
3-minute read
What Is a Content Editor?
Are you interested in learning more about the role of a content editor and the...
4-minute read
The Benefits of Using an Online Proofreading Service
Proofreading is important to ensure your writing is clear and concise for your readers. Whether...
6 Online AI Presentation Maker Tools
Creating presentations can be time-consuming and frustrating. Trying to construct a visually appealing and informative...
What Is Market Research?
No matter your industry, conducting market research helps you keep up to date with shifting...
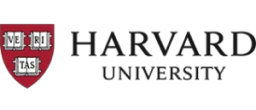
Make sure your writing is the best it can be with our expert English proofreading and editing.
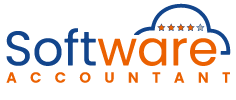
5 Free Assignment Tracking Templates for Google Sheets
Posted on Last updated: November 18, 2023
It’s that time of year again—assignments are piling up and it feels impossible to stay on top of everything. As a student, keeping track of all your assignments, due dates, and grades can be overwhelmingly stressful. That’s why using a Google Sheet as an assignment tracker can be a total game-changer.
With customizable assignment tracking templates for Google Sheets, you can easily create a centralized place to organize all your academic responsibilities. The best part? These templates are completely free.
In this article, we’ll explore the benefits of using assignment tracking templates for Google Sheets and provide links to some excellent templates that any student can use to get organized and take control of their workload.
The Benefits of Using Assignment Tracking Templates for Google Sheets
Assignment tracking templates for Google Sheets offer several advantages that can help students stay on top of their work. Here are some of the key benefits:
- Centralized tracking: Rather than having assignments scattered across syllabi, emails, and other documents, an assignment tracking spreadsheet consolidates everything in one place. By leveraging assignment tracking templates for Google Sheets, you can kiss goodbye to hunting for due dates or double-checking requirements.
- Customizable organization: Students can add or remove columns in the template to fit their needs. Thanks to this, they can effectively track due dates, point values, grades, and other helpful details. They can also color code by class or status for visual organization.
- Easy access: Google Sheets are accessible from any device with an internet connection. With this, you can easily view, update, or add assignments whether you are on your laptop, phone, or tablet.
- Shareable with others: For group assignments or projects, assignment tracking templates for Google Sheets make collaboration seamless as you can share the sheet with a study group or entire class to coordinate.
- Helps prioritization: Sort assignments by due date or point value to always know what needs your attention first. With prioritization added to assignment tracking templates for Google Sheets, you can stay on top of bigger projects and assignments.
- Reduces stress: There’s no better feeling than looking at your assignment tracker and knowing everything is organized and under control. Saves time spent scrambling, too.
Picking the Perfect Assignment Tracking Templates Google Sheets
When choosing assignment tracking templates for Google Sheets, you’ll want one with specific fields and features that make it easy to stay on top of your work. Here’s what to look for in a homework organizer template:
- Assignment Details: A column for writing down each assignment’s name, instructions, and notes will help you remember exactly what you need to do.
- Due Dates: Columns for listing the due dates of assignments, tests, and projects allow you to see what’s coming up and schedule your time wisely.
- Status Tracker: A place to mark assignments as “Not Started,” “In Progress,” or “Completed” lets you check on what still needs your attention.
- Subject and Type: Categories or labels for sorting assignments by subject or type (essay, presentation, etc) keep your spreadsheet tidy.
- Big Picture View: Some templates include a calendar view or semester schedule to help you plan assignments week-by-week or month-by-month.
The right spreadsheet has the fields you need to fully describe your homework and organize it in a way that works for you. With the perfect template, staying on top of assignments is easy
Top Assignment Tracking Templates
Now that you know the benefits and what to look for in an assignment spreadsheet, we have compiled a list of top assignment tracking templates for Google Sheets that will help you seamlessly track your assignments.
And guess what? You don’t need robust experience with Google Sheets to maximize these templates, as they are easy to use.
Convenient Homework Planner Template
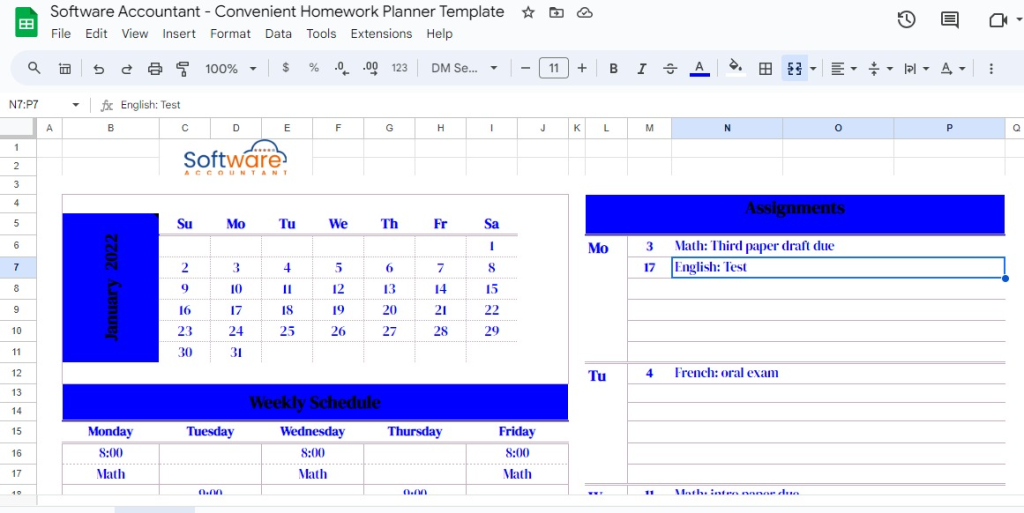
The Convenient Homework Planner Template is one of the most comprehensive and user-friendly assignment tracking templates for Google Sheets. It’s an excellent fit for students seeking an all-in-one solution to organize their work.
This template includes separate tabs for an overview calendar, assignment list, and weekly schedule. The calendar view lets you see all assignments, tests, and projects for the month at a glance. You can quickly identify busy weeks and plan accordingly.
On the assignment list tab, you can enter details like the assignment name, class, due date, and status.
The weekly schedule tab provides a simple agenda-style layout to record daily assignments, activities, and reminders. This helps you allocate time and schedule focused work sessions for tasks.
Key Features
- Monthly calendar view for big-picture planning
- Assignment list with details like class, due date, and status
- Weekly schedule with time slots to map out days
- Due date alerts to never miss a deadline
With its intuitive layout, useful visual features, and thorough assignment tracking, the Convenient Homework Planner has all you need to master organization and time management as a student. By leveraging this template, you’ll spend less time shuffling papers and focusing more on your academics.
Ready to explore this assignment tracking template? Click the link below to get started.
The Homework Hero Template
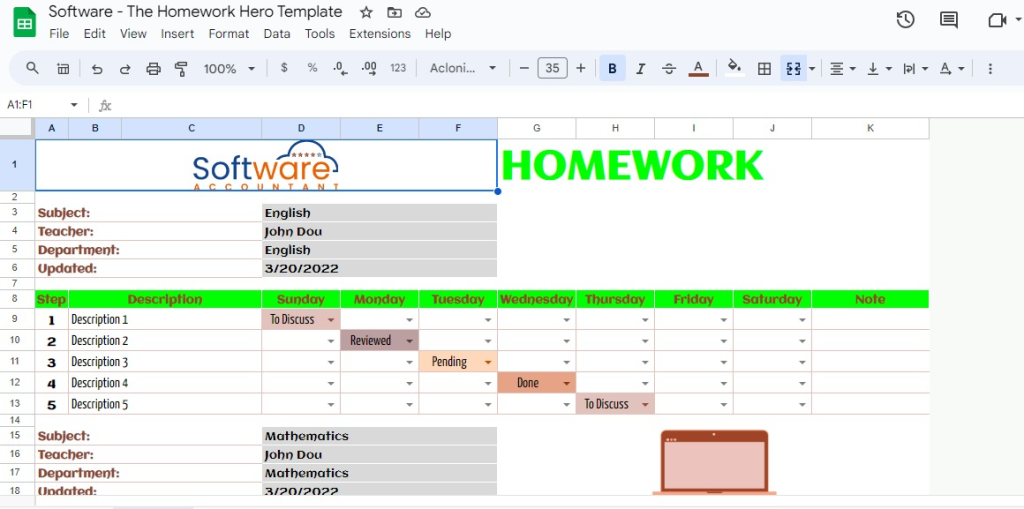
The Homework Hero is an excellent assignment-tracking template tailored to help students conquer their academic workload. This easy-to-use Google Sheet template has dedicated sections to log critical details for each class.
The Subject Overview area allows you to record the teacher’s name, subject, department, and timeline for each course. This provides helpful context and reminds you of important class details.
The main homework tracking area includes columns for each day of the week. Here, you can enter the specific assignments, readings, and tasks to be completed for every class on a given day. No more guessing what work needs to get done.
At the extreme end of this sheet is a section for additional notes. Use this to jot down reminders about upcoming projects, tests, or other priorities.
Key features
- Subject Overview section for every class
- Columns to record daily homework tasks
- Extra space for notes and reminders
- An intuitive layout to map out the weekly workload
- Easy to customize with additional subjects
The Homework Hero assignment tracking template empowers students to feel in control of their assignments. No more frantic scrambling each day to figure out what’s due. With this template, you can approach schoolwork with confidence.
Click the link below to get started with this template.
The A+ Student Planner Template
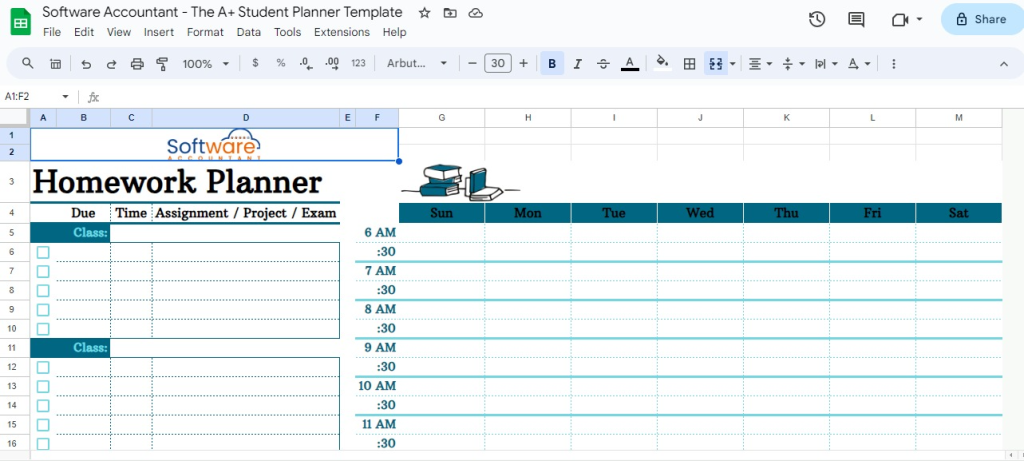
The A+ Student Planner is the perfect template for students seeking an organized system to manage assignments across all their courses. This Google Sheet template has useful sections to input key details for flawless homework tracking.
The Weekly Overview calendar makes it easy to see your full workload at a glance from Sunday to Saturday. You can note assignments, projects, tests, and other school events in the daily boxes.
The Class Information section contains columns to list your class, teacher, room number, and times. This ensures you have all the essential details in one place for each course.
The main Assignment Tracking area provides space to log the name, description, due date, and status of each homework task, project, exam, or paper. No more scrambling to remember what needs to get done.
- Weekly calendar view to map out school events and tasks
- Class information organizer for easy reference
- Robust assignment tracking with all critical details
- An intuitive layout to input assignments across courses
- Great for visual learners
With a structured format and helpful organization tools, The A+ Student Planner provides next-level assignment tracking to ensure academic success. Staying on top of homework has never been easier.
Ready to get started with this assignment tracking template? Access it for free via this link below.
The Complete Student Organizer Template

The Complete Student Organizer is an excellent minimalist assignment tracking template for focused homework management.
This straightforward Google Sheets assignment template includes columns for the date, total time needed, assignment details, and status. By paring down to just the essentials, it provides a simple system to stay on top of homework.
To use this template, just fill in the date and time required as you get assigned new homework. In the assignment details column, outline what needs to be done. Finally, mark the status as you work through tasks.
- Streamlined columns for date, time, assignment, and status
- Minimalist layout focused only on crucial details
- Easy input to quickly log assignments
- Track time estimates required for assignments
- Update status as you progress through homework
The Complete Student Organizer is the perfect template for students who want a fuss-free way to track their homework. The simplicity of the grid-style layout makes it easy to use without extra complexity. Stay focused and organized with this efficient assignment tracking sheet.
You can get access to this template by visiting the link below.
Assignment Slayer: The Ultimate Planner Template
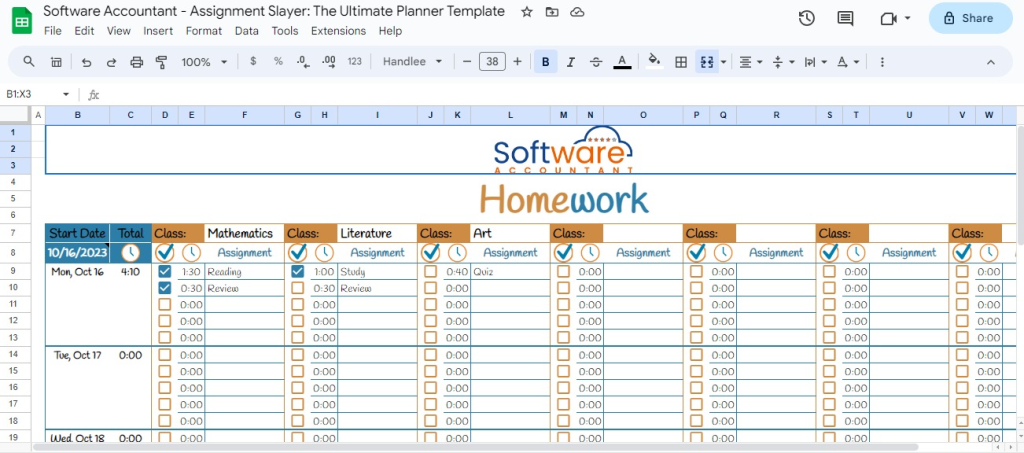
Assignment Slayer is the supreme template for tackling schoolwork with military-level organizations. This comprehensive planner is ideal for students taking multiple classes and juggling a heavy workload.
The template includes separate tabs for each academic subject. Within each tab, you can log critical details, including the assignment name, description, status, due date, and associated readings or tasks. With this assignment tracking template, no assignment will fall through the cracks again.
Plus, it has additional columns that allow you to record scores and grades as they are received throughout the semester. This level of detail helps you better understand your standing in each class.
The Ultimate Planner also contains an overview dashboard with calendars for the month, week, and each day. With this, you can visually map out all upcoming assignments, tests, and projects in one view.
- Individual subject tabs for detailed tracking
- Robust assignment logging with name, description, status, due date, and more
- Columns to record scores and grades when received
- Monthly, weekly, and daily calendar dashboard
- Visual layout ideal for visual learners
Assignment Slayer equips students with military-level organization. Its comprehensive features give you command over academic responsibilities, resulting in stress-free homework mastery.
Want to explore how this template can make your job easy? Click the link below to access this free assignment tracking template now.
Why You Should Take Advantage of These Assignment Tracking Templates For Google Sheets
The assignment tracking templates for Google Sheets we reviewed in today’s guide offer significant advantages that can make managing homework easier. Here are some of the top reasons students love using these digital planners:
Get Organized
The templates allow you to sort all your assignments neatly by subject, type, due date, and status. No more fumbling through papers to find the next thing you need to work on. Plus, the level of organization you get with these templates helps reduce stress.
Manage Time Better
Knowing exactly when assignments are due helps with planning out your week. You can see what needs to get done first and schedule time accordingly. No more last-minute assignment crunches.
Access Anywhere
You can view and update your homework template from any device as long as you have an internet connection. The templates are ready to go as soon as you make a copy – no setup is needed. Easy access keeps you on track.
With useful tools for organization, planning, and accessibility, these assignment tracking templates for Google Sheets make managing homework a total breeze. Boost your productivity and reduce academic stress today by using these templates for your assignment.
Final Thoughts
Today’s guide explored some of the most accessible and useful assignment tracking templates for Google Sheets. These handy templates make it easy for students to stay organized and on top of their workload.
As a busy student, keeping track of your homework, projects, tests, and other responsibilities across all your courses can be daunting. This is where leveraging a spreadsheet template can make a huge difference in simplifying academic organization.
The assignment tracking templates for Google Sheets reviewed today offer intuitive layouts and customizable features to create a centralized homework hub tailored to your needs.
Key benefits include:
- Inputting all assignments in one place for easy reference
- Tracking due dates, status, grades, and other key details
- Customizable columns, colors, and more to fit your study style
- Easy access to update assignments from any device
- Helps prioritize your time and tasks needing attention
- Reduces stress by helping you feel in control
By taking advantage of these assignment tracking templates for Google Sheets, you can reduce time spent shuffling papers and focus your energy where it matters – knocking out quality academic work. Make your life easier and get a digital organizational system in place.

You're signed out
Sign in to ask questions, follow content, and engage with the Community
- Student Information Systems (SIS)
- SIS Discussion
Sync to SIS list of assignments - is there a way t...
- Subscribe to RSS Feed
- Mark Topic as New
- Mark Topic as Read
- Float this Topic for Current User
- Printer Friendly Page
Sync to SIS list of assignments - is there a way to limit, filter?
- Mark as New
- All forum topics
- Previous Topic
Canvas does not sync late assignments to Aspen
Syncing grades from one course into multiple infin..., attendance passback to banner, re: changing the teacher of a class without losing..., can i sync all class grades to skyward at once man..., ps category syncing into incorrect classes, sis integration id vs sis source id custom params..., community help, view our top guides and resources:.
To participate in the Instructurer Community, you need to sign up or log in:
Help | Advanced Search
Computer Science > Computer Vision and Pattern Recognition
Title: bridging vision and language spaces with assignment prediction.
Abstract: This paper introduces VLAP, a novel approach that bridges pretrained vision models and large language models (LLMs) to make frozen LLMs understand the visual world. VLAP transforms the embedding space of pretrained vision models into the LLMs' word embedding space using a single linear layer for efficient and general-purpose visual and language understanding. Specifically, we harness well-established word embeddings to bridge two modality embedding spaces. The visual and text representations are simultaneously assigned to a set of word embeddings within pretrained LLMs by formulating the assigning procedure as an optimal transport problem. We predict the assignment of one modality from the representation of another modality data, enforcing consistent assignments for paired multimodal data. This allows vision and language representations to contain the same information, grounding the frozen LLMs' word embedding space in visual data. Moreover, a robust semantic taxonomy of LLMs can be preserved with visual data since the LLMs interpret and reason linguistic information from correlations between word embeddings. Experimental results show that VLAP achieves substantial improvements over the previous linear transformation-based approaches across a range of vision-language tasks, including image captioning, visual question answering, and cross-modal retrieval. We also demonstrate the learned visual representations hold a semantic taxonomy of LLMs, making visual semantic arithmetic possible.
Submission history
Access paper:.
- HTML (experimental)
- Other Formats
References & Citations
- Google Scholar
- Semantic Scholar
BibTeX formatted citation

Bibliographic and Citation Tools
Code, data and media associated with this article, recommenders and search tools.
- Institution
arXivLabs: experimental projects with community collaborators
arXivLabs is a framework that allows collaborators to develop and share new arXiv features directly on our website.
Both individuals and organizations that work with arXivLabs have embraced and accepted our values of openness, community, excellence, and user data privacy. arXiv is committed to these values and only works with partners that adhere to them.
Have an idea for a project that will add value for arXiv's community? Learn more about arXivLabs .
Milwaukee Brewers Boot Former Highly-Regarded Prospect Off 40-Man Roster
In a series of roster moves on Wednesday, the Milwaukee Brewers designated former highly-regarded prospect Vladimir Gutierrez for assignment.
- Author: brady farkas
In this story:
They'll now have a week to trade him, release him or outright him to the minors. He never even pitched a game for the Brewers after being claimed off waivers from the Toronto Blue Jays earlier this year.
Per the team on social media:
RHP Tobias Myers selected from Triple-A Nashville. LHP Jared Koenig optioned to Nashville. RHP Vladimir Gutierrez designated for assignment.
RHP Tobias Myers selected from Triple-A Nashville. LHP Jared Koenig optioned to Nashville. RHP Vladimir Gutierrez designated for assignment. pic.twitter.com/EMyDhKD2mG — Milwaukee Brewers (@Brewers) April 17, 2024
The 28-year-old native of Cuba has spent parts of three years in the big leagues with the Reds and Marlins. He made his debut in 2021 and actually started 22 games for Cincy, pitching to a 4.74 ERA. Unfortuantely, that success didn't translate. He had a 7.61 ERA in 10 appearances in 2022 and missed all of 2023 with injury.
This offseason, he joined up with the Marlins, appearing in one game for Miami. He then landed with Toronto and Milwaukee but didn't appear in a game for either of them.
Given his age and former high prospect status, he's likely to get more opportunities, it's just a question of where. Perhaps he could stay in the Brewers organization as a depth piece.
Milwaukee enters play on Wednesday at 10-6 overall. They'll take on the San Diego Padres on Wednesday at 1:10 p.m. ET.
Michael King (2-0, 4.19 ERA) gets the ball for San Diego (11-9) while Bryse Wilson pitches for Milwaukee. The 26-year-old is 1-0 with a 5.19 ERA thus far.
Follow Fastball on FanNation on social media
Continue to follow our Fastball on FanNation coverage on social media by liking us on Facebook and by following us on Twitter @FastballFN .
Latest News
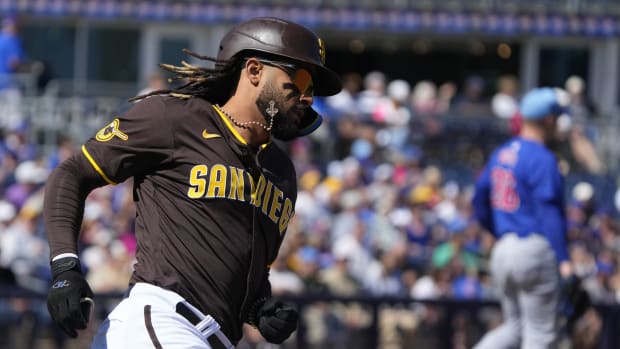
Fernando Tatis Jr. Does Something He's Never Done in His Career on Thursday
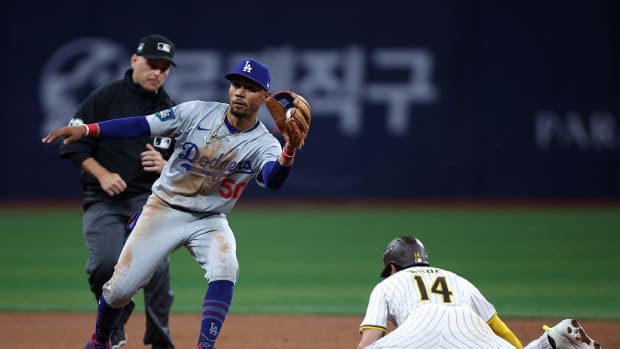
Los Angeles Dodgers' Star Does Something Nearly Never Done in Last 100 Years of Team History
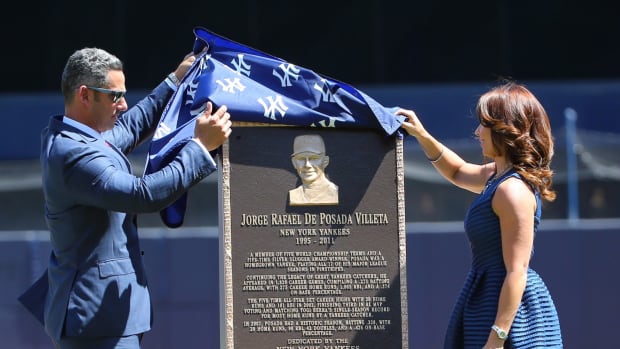
New York Yankees' Legend Jorge Posada Dishes on Career, Baseball and Acting in a Miller Lite Commercial
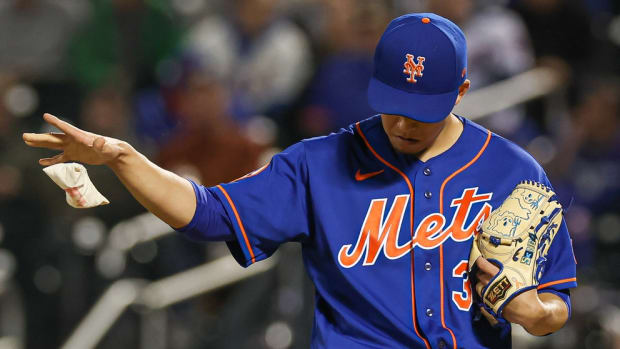
New York Mets Provide Important Information Regarding Ace Pitcher's Health
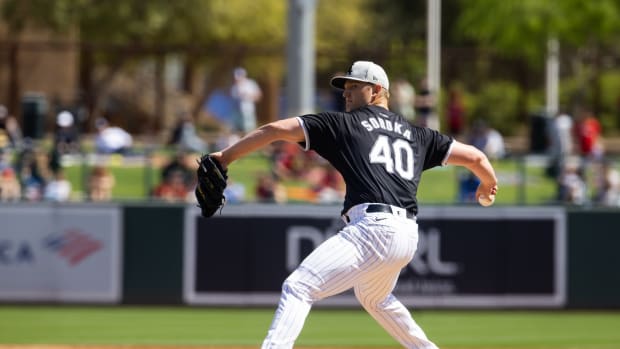
Chicago White Sox to Put Former Atlanta Braves Standout Near Top of Rotation
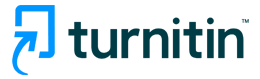
Get Started
Setting up your Turnitin classes is easy when you know how. In just four quick steps, learn more about Turnitin's class management tools and how to get your students started. At the end of this tutorial, you can put these simple steps into practice.
1. Create Your Password
You'll need your email address and last name to create your Turnitin account password and set your security information; this information can be found in your welcome email. You can then log into Turnitin and begin customizing your account.
2. Create a Class
The creation of a class is the first step towards using the Turnitin services available to your institution. A Turnitin class groups assignments, helping you to organize student submissions. Once your classes have been created, you can start creating assignments.
- Click the All Classes tab from any Turnitin page to direct you to the homepage
- Click the green Add Class button
- From the Create a new class page, select the class type, and complete the fields marked with an asterisk
- Select the class end date
- Click Submit to add your class to Turnitin
- Once your class has been created, you will be provided with the Class ID and enrollment password, which will allow your students self enroll
3. Create an Assignment
Once your class is ready, it's time to set up your first assignment. A Turnitin assignment forms the basis of accepting student submissions. Once your assignments are set up, you start adding students to your class.
- Click the relevant class name
- From your class, click the green Add Assignment button
- Enter an assignment title
- Opt to only allow students to submit file types that generate Originality Reports or to allow any file type
- Next, select your assignment's start date, end date, and post date; the assignment post date is the date from which your students can view your feedback
- To customize your assignment further, click the optional settings button to reveal an array of options; each option will be accompanied with contextual help icons
- Click Submit to add your assignment to your Turnitin class
4. Add Students
There are three routes available for adding students. You may find it convenient to add students one by one, or add a large portion of students at once by uploading a list. Alternatively, why not allow your students to enroll themselves at their own pace?
Add Students One by One
You may prefer to use this method when adding fewer than ten students.
- Click Home from any Turnitin page to direct you to the homepage
- From the Class homepage, click the Students tab at the top of the page
- Click the Add Student button to the right
- Enter the student's first name, last name, and email address
- Click Submit to add the student
Upload a List of Students
For adding ten students or more, you may find it quicker and easier to upload a list.
- In a Word™ or plain text file, each student should be written as: first name, last name, email address format with one student per line. In Excel™, separate the first name, last name, and email address into different cells in a column.
- From the student list, click the Upload List button
- Click the Choose file button and browse for the plain text, Word™, or Excel™ file that you wish to upload
- Once the file has uploaded, click the Submit button to upload
- Check the student details displayed on screen, then click yes, submit to add the students, or no, go back to amend the file
Allow Students to Self-Enroll
Allowing students to self-enroll can save you time.
- Make a note of the seven-digit Class ID for the class you would like your students to join
- Next, select the cog icon under Edit
- From the Edit Class page, make a note of the enrollment password
- Pass the Class ID and enrollment password to your students
- Ensure this information is kept safe at all times
Ready to Start Using Turnitin?
Or why not download this page as a PDF for later reading? This information and more is available at guides.turnitin.com !
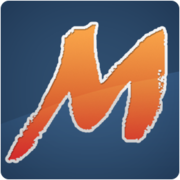
Breaking News Alerts
Press "allow" to activate.
- Food & Dining
- Arts & Entertainment
- Real Estate
- Crime Statistics
- Local Sports
- Weather Forecast
- Surf Report
- Maui Arts & Entertainment
- Food and Dining
- On the Menu
- Visitors’ Guide
- Maui Discussion
- Reader Survey
- Upcoming Maui Events
- Map of Events
- Post an Event
- Recent Job Listings
- IMUA Discovery Garden
- Medical Minute
- Latest Maui Videos
- About Maui Now
- Contact Information
- Get the App
- Advertising
- Meet the Team
Privacy Policy | About Our Ads

- Wildfires & Recovery
- Entertainment
Angels on Assignment, a new support program for Maui cancer patients, takes flight
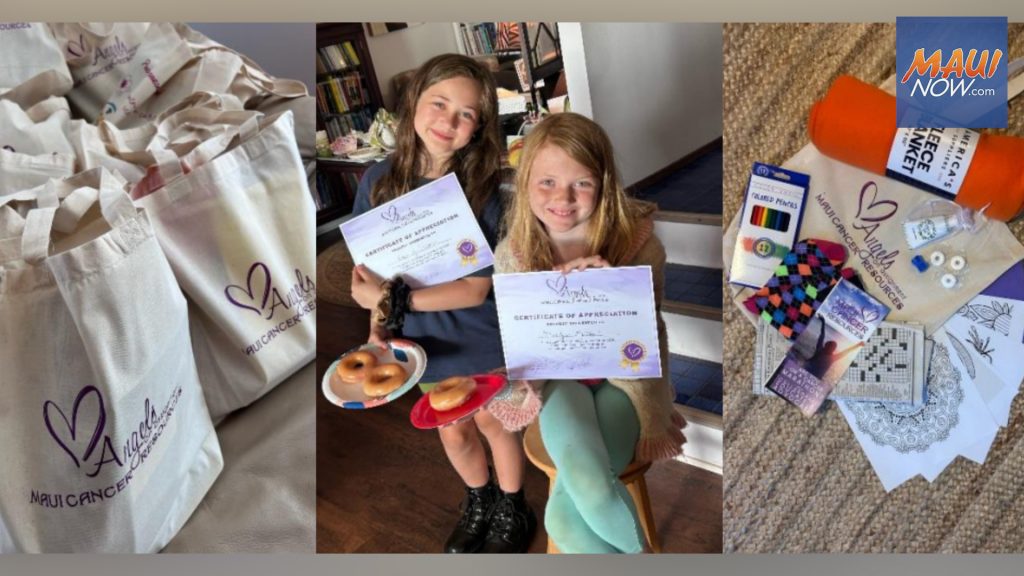
“Angels on Assignment” is a new, volunteer-based program launched by Maui Cancer Resources to support Maui cancer patients as they get through chemotherapy or radiation treatment.
“Although Angels has been in existence a little over a month, we are finding ways to help and support those with cancer,” said Dr. Bridget Bongaard, medical director.
The program is funded through a generous donation from 100 Women Who Care Maui.
On March 28, Angel volunteers made 20 yarn lei that were delivered to the Maui Health Oncology Department to present to cancer patients when they complete their chemotherapy or radiation treatment. Each lei carried a special uplifting card to let patients know they are supported and loved. Angels has committed to provide 20 lei each month.
The Angels group is also creating Cancer Care Bags for patients in treatment. In each bag, a cancer patient will find a blanket, socks, lotion, mints, a journal, colored pencils with a sharpener and mandalas coloring pages plus crossword puzzles.
On April 6, Makawao Montessori School students and another child and their mothers joined Dr. Bongaard and other Angel volunteers at her house to put together the Angel’s first 25 Cancer Care Bags as part of their school project.
Adults and youths are welcomed and appreciated for volunteering. Anyone interested in volunteer opportunities as an Angel, or to donate funds for needed supplies on the Amazon Gift Registry, contact Cassie Pali at [email protected] .
Sponsored Content
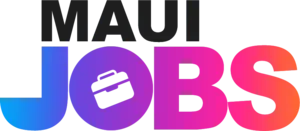
Subscribe to our Newsletter
- Send Me Daily Updates
- Send Me Weekly Updates
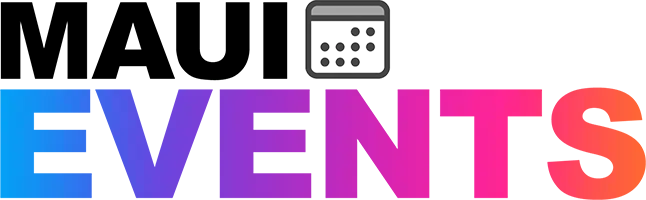
- Maui Business
- Maui Sports
- Letters to the Editor
- Maui Activities
- Official Visitors’ Guide
- About Our Ads
- Terms of Service

Facebook YouTube Twitter Instagram
- Robert Stephenson To Miss 2024 Season With Elbow Injury
- Astros To Activate Justin Verlander On Friday
- Whitey Herzog Passes Away
- Rangers To Promote Jack Leiter
- Dodgers To Promote Andy Pages
- 2024-25 MLB Free Agent Power Rankings
- Hoops Rumors
- Pro Football Rumors
- Pro Hockey Rumors
MLB Trade Rumors
Blue Jays Designate Mitch White For Assignment
By Darragh McDonald | April 16, 2024 at 3:20pm CDT
The Blue Jays announced today that right-handers Jordan Romano and Erik Swanson have been activated off the injured list. In corresponding moves, they optioned righty Nate Pearson to Triple-A and designated righty Mitch White for assignment.
Romano and Swanson were key pieces of the Toronto bullpen last year but they haven’t been able to contribute to the club thus far in 2024. During the spring, Romano had some inflammation in his elbow while Swanson had some tightness in his forearm, leading both to start the season on the injured list.
With those two unavailable, the club has turned to Yimi García and Chad Green for most of the high-leverage work, with those two filling in admirably. García has a 1.17 earned run average through seven appearances, with 11 strikeouts to go with just one walk. Green, meanwhile, has a 2.35 ERA in his seven appearances, with seven punchies and two walks.
Despite that strong work from those two, the Jays are surely glad to get Romano and Swanson back. Romano has emerged as the club’s closer over the past three years, which included saving 36 games in each of the past two campaigns while keeping his ERA under 3.00 in each. Swanson had 29 holds and four saves last year while posting a 2.97 ERA on the season. Those two, along with García and Green, give the Jays a strong four-headed bullpen mix to finish out games.
The health of that group has nudged White off the roster. Now 29, White was a second-round pick of the Dodgers back in 2016 and was considered by Baseball America to be the #69 prospect in the league in 2018. The Jays acquired him in a 2022 deadline deal alongside Alex De Jesus , with prospects Nick Frasso and Moises Brito going the other way.
At the time of that deal, White had thrown 105 2/3 big league innings with a 3.58 ERA, 22% strikeout rate and 8.3% walk rate. Unfortunately, the jersey swap corresponded with an immediate downturn in his results. White tossed 43 innings for the Jays in 2022 with a 7.74 ERA and 15.3% strikeout rate. There was a bit of bad luck in there, as his .368 batting average on balls in play and 54.3% strand rate were both on the unfortunate side, which is why his FIP was 3.76 in that time and his SIERA 4.70.
Luck or not, the poor results meant the Jays couldn’t guarantee a rotation spot to White going into 2023. At that time, four rotation spots were taken by Alek Manoah , Kevin Gausman , José Berríos and Chris Bassitt . White went into Spring Training battling Yusei Kikuchi for the final spot but dealt with some shoulder and elbow injuries and had to start the season on the IL. By the time he got back, there was no rotation spot for him and he worked a long relief role in the bullpen.
He didn’t take to that move, posting a 7.11 ERA in 10 outings before being designated for assignment. The 29 other clubs passed on the chance to grab him off waivers and he was sent outright to Triple-A. He got stretched out in Buffalo and finished the season in good form, with a 1.89 ERA over his last 33 1/3 innings, pairing a 31.4% strikeout rate in that time with a 10.2% walk rate.
The Jays added him back to the 40-man in November to prevent him from reaching minor league free agency, which put him in a similar spot to where he was a year prior, coming into spring out of options and battling for a spot. The Jays had to put Manoah on the IL this spring, which opened a rotation spot, but Bowden Francis beat White for that gig. Now that Yariel Rodríguez has also been stretched out and has seemingly bumped Francis from the rotation, White has been moved even further back. He has only made four long relief appearances this year but his uninspiring 5.40 ERA in those surely didn’t help him.
White has now been bumped off the roster yet again and the Jays will have one week to work out a trade or pass him through waivers. Since he cleared waivers last year, doing so again would give him the right to elect free agency. It’s possible he may garner interest based on his past results and strong finish at Triple-A last year. The fact that he’s out of options means that he needs an active roster spot somewhere, but he has less than three years of service time, meaning he can be controlled for three more seasons beyond this one.
A number of teams around the league are dealing with significant pitching injuries and it was less than a week ago that the Jays managed to flip Wes Parsons to the Guardians for international bonus pool space. Parsons is optionable but is more than two years older than White and doesn’t have the same past prospect pedigree.
35 Comments
I guess maybe a team like the A’s or Marlins could put in a claim for white, but I assume he’ll remain in the jays system
Name correlates well to the story about Mitch White.
White is another overrated prospect from LAD, even the A’s should take a pass on him.
I got to hand it to LAD they surely make out when trading these overhyped prospects and getting good return so far Frasso may have higher upside in the end.
Although they have lost out with Busch but they had no where to place him.
Like they did with Yordan Alvarez
White was a lot better with LA than Toronto. I wonder why…
Why the hell would they option Pearson. He’s been nothing short of outstanding. The guy is finally putting it together and they ship him back to AAA
It’s called options. He’ll be back as soon as another injury happens
All other right handers in their pen have looked good, only the two lefties look horrible but they probably don’t want to go with just one lefty in the pen
6.1 innings of awesome after years of not so awesome. Who would you have lost out of the bullpen instead?

Two Jays starters aren’t fully stretched out. They need a long man in the bullpen. Pearson is not it.

Damn the curse of having options for Pearson.
I recommended the Jays release Mitch White in Aug 2022 when he was acquired because with the BlueJays he is not a MLB level pitcher. What a waste of time and money. Let Mitch White go for good.
The Mitch White deal reminds me of trading star in the making Moreno for Varsho, which was also called a bad deal at that time too.
Pearson belongs in the bigs. He’ll be back. If they use him as a one inning guy Pearson can be a very good pitcher. Pearson needs a one inning per use managed and reduced workload to maintain performance excellence.
Atkins is bad, no one denies it.

Get creative and trade excess 2b to AA , for something we need like a good bat for the of Got clement ikf Biggio Schneider… atl could use a bat at 2b w Albies out for a while
Finally ,another atkins mistake dfa’d . Shakin’ My Head …..
Hasn’t been all bad with Atkins on the pitching side. Brought in guys like Matz Robbie ray stripling Kikuchi etc and got the most out of them. They let em go and they stink or hurt. Good call to go to Gausman instead of ray for the dough . Got Romano Jimmy Garcia for nothing as afterthoughts Built good pens last few years. It’s the offence he’s bad , both drafting and at the mlb level.
And where has that got us ??
They were in on Ray as well. He wanted too much though
Im not a shatkins guy and doo want them both gon but you can deny that have made great decisions alomost with all there signing and every trade but 2. Even the trad for Grichuk for example sure he never lived up to want they hoped but thay nothing for him. Drafting bad or very inconveniently draft good player is this jays groups down fall
@Murphy NFLD
The Grichuks trade wasn’t all that good. The 5 year, 50M deal was nothing short of awful.
This is a premature move. With Gausman not Gausman’ing, Rodriguez having less than 5 IP of pro ball (no innings pitched last year at any league level) and Kikuchi pitching more than 5 innings only 10 times since the start of ’23, the Jays needed someone to give them innings. Even comparing Francis to White, the numbers are similar, but Francis has an option.
To put this in perspective, if the FO options Francis instead, then a week later a starter hits the IL, Francis can come back up to fill the spot. With this decision, Francis fills the spot and who becomes the long man?
Just poor roster management.
Perhaps they felt like it would not be beneficial for Bowden Francis to go down despite his numbers right now. Mitch White hasn’t had a good track record in the majors for quite some time.
I think they should trade Mitch White (if they can) for more international international signing bonus money…
The need is to chuck in a guy to cover Rodriguez, Gausman, and maybe Kikuchi. Francis can come in for one of them for multiple innings. Richards can take one of the remaining 2. After that, you’re looking at leveraged arms coming in non leveraged spots. DFA’ing White was a move that was coming but it shouldn’t have been this early. In tennis terms, this is an unforced error.
I have a feeling that they’ll be able to nab a similar arm off waivers in short order.
20 hours ago
I agree with this. I’m not comfortable about the innings that need covering. I’d have optioned Francis and kept White. Jays fans hate White. But there are a few things he does well enough and a number 6 or 7 starter isn’t really going to perform like a 3 or 4. Does he perform well enough compared to other depth starters? I think there’s reason to think he can. But I also think Rodriguez looks like he can leave the game with the team in good shape and Francis seems to do a lot better in that shorter stint bridge to the leverage arms role than White and they are probably projecting a very tight race for those wild card spots and playing for the games immediately in front of them more than having the luxury of thinking about the longer term and preserving depth. With the benefit of hindsight, I would have just DFA’d White instead of Parsons at the time. Then with this move they could have just optioned Peason and Parsons and the active roster would look the same with a full 40 man roster that still had Parsons available as depth. But they just signed this year’s version of Parsons in Mike Mayers, so maybe they see traits there they think they can tap into.
Too bad about Pearson but this made some sense. Neither LH bullpen arm is going anywhere, they need Bowden to eat innings, and the rest firmly have a place in the bullpen.
They could have gotten creative by sending Francis down and putting White in his spot in the rotation. Would he have been worse? I’d argue that, based on Francis’ early season numbers, White may have been slightly better. But probably not enough to warrant such a move. Also, I don’t know if Francis has options left. If not, the Blue Jays probably value him much more than they do White.
@Baseball77
Francis has one option left.
White is better than nobody. His presence has been unnecessary since the horrendous trade happened.
What specific factors led to Mitch White’s designation for assignment, considering his past prospect pedigree and recent strong finish at Triple-A, and how does this decision align with the Blue Jays’ long-term pitching strategy?
I suspect the decision was made by throwing darts at the wall or playing beer pong. Applying logic, reason, or common sense fails.
21 hours ago
The long term pitching strategy is the interesting part of the question. I find it surprising that they DFA’d Parsons who still had an option only to turn around and DFA White a couple weeks later. They didn’t need the 40 man spot. Only the active one. Moving on from both Parsons and White when Rodriguez isn’t expected to be a big source of innings and Francis lost his his starting gig for now seems like a move you make when you are confident you have someone claiming a stake to a depth role. I’m not sure who that is. The prospects in AAA are having a rough transition so they aren’t particularly close and the veteran AAA arms are less inspiring than White or Parsons. Manoah is a very big part of the conversation but also a very big mystery. In terms of the long term, they don’t seem to have a lot of guys who fit into the traditional starter mold. What they do have is kind of hybridized guys who can move fast up the system. Most of them will back down to single inning types but I wonder if they might be comfortable with the idea they can be more competitive with bullpen games featuring multi inning types than throwing out lousy depth starters .
Management basically looked at the roster of pitchers and said to themselves ״who’s the worst “ came up with Mitch.
The white flag has been dfa’d
At this point, White is a quadruple-A player. Good enough to be fill in for an injury, but not good enough to stick. Out of options means he’ll be on the dfa carousel and shuttling among the many mlb franchises if he’s lucky. Otherwise, it’s Asia, Mexico, or some other line of work….
Leave a Reply Cancel reply
Please login to leave a reply.
Log in Register

- Feeds by Team
- Commenting Policy
- Privacy Policy
MLB Trade Rumors is not affiliated with Major League Baseball, MLB or MLB.com
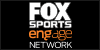
Username or Email Address
Remember Me

IMAGES
VIDEO
COMMENTS
Assigning to lst[lst.index(row)] results in O(n²) performance instead of O(n), and may cause errors if the list contains multiple identical items. Instead, assign a new list, constructed with a list comprehension or map: lst = [1,2,3,4] doubled = [n*2 for n in lst] Alternatively, you can use enumerate if you really want to modify the original ...
The list class is a fundamental built-in data type in Python. It has an impressive and useful set of features, allowing you to efficiently organize and manipulate heterogeneous data. Knowing how to use lists is a must-have skill for you as a Python developer. ... In this slice assignment, you assign an empty list to a slice that grabs the whole ...
Python 3.8 will introduce Assignment Expressions. It is a new symbol: := that allows assignment in (among other things) comprehensions. This new operator is also known as the walrus operator. It will introduce a lot of potential savings w.r.t. computation/memory, as can be seen from the following snippet of the above linked PEP (formatting ...
Python lists store multiple data together in a single variable. In this tutorial, we will learn about Python lists (creating lists, changing list items, removing items, and other list operations) with the help of examples.
The ultimate study app. The MyStudyLife student planner helps you keep track of all your classes, tasks, assignments and exams - anywhere, on any device. Whether you're in middle school, high school or college MyStudyLife's online school agenda will organize your school life for you for less stress, more productivity, and ultimately ...
The list is the first mutable data type you have encountered. Once a list has been created, elements can be added, deleted, shifted, and moved around at will. Python provides a wide range of ways to modify lists. Modifying a Single List Value. A single value in a list can be replaced by indexing and simple assignment:
To create a new variable or to update the value of an existing one in Python, you'll use an assignment statement. This statement has the following three components: A left operand, which must be a variable. The assignment operator ( =) A right operand, which can be a concrete value, an object, or an expression.
List. Lists are used to store multiple items in a single variable. Lists are one of 4 built-in data types in Python used to store collections of data, the other 3 are Tuple, Set, and Dictionary, all with different qualities and usage. Lists are created using square brackets:
Organize your work and life, finally. Become focused, organized, and calm with Todoist. The world's #1 task manager and to-do list app. Start for free. 42+ million people and teams trust their sanity and productivity to Todoist. Clear your mind.
Homework Checklist. For a plain and simple homework checklist, this template from TeacherVision is great for younger students, but can work for any age. Each subject is in its own spot with days of the week and check boxes to mark off as you complete assignments. 2. Printable Homework Planner.
What this handout is about. The first step in any successful college writing venture is reading the assignment. While this sounds like a simple task, it can be a tough one. This handout will help you unravel your assignment and begin to craft an effective response. Much of the following advice will involve translating typical assignment terms ...
The available colors are the following: blue, gray, and white. You should also use a colon to introduce a list if semicolons separate the items in the list: The available colors are: blue and gray; black and white; and red and pink. Later I'll explain whether to choose commas or semicolons to separate the items in your list.
Our free homework planner printable will keep you organized and on top of your homework assignments. We also offer a digital version if you prefer. Both are free. Contents hide. 1 Homework Planner Template. 1.1 Homework Calendar. 1.2 Daily Homework Planner. 1.3 Weekly Homework Planner. 1.4 Homework Checklist.
Find free task list and checklist templates for Word, Excel, and PDF, including basic task and to-do list templates, as well as specific use case templates for business and personal projects. ... Create a list of tasks and assign each item to an individual for any day of the week. With a simple format, this template is easy to use, so you can ...
2. Fill in the title of the subjects you would like to track assignments for in each header row in the Assignments tab. 3. Fill in the title of each of your assignments and all the required tasks underneath each assignment. 4. List the title of the assignment for each subject and color code the week that the assignment is due in the Study Schedule.
Assignment list with details like class, due date, and status; Weekly schedule with time slots to map out days; Due date alerts to never miss a deadline; With its intuitive layout, useful visual features, and thorough assignment tracking, the Convenient Homework Planner has all you need to master organization and time management as a student ...
Transform your productivity with Notion's To-Do Lists templates. Designed for daily tasks, project milestones, and everything in between, our lists keep you organized and on track. Perfect for individuals and teams looking to streamline their priorities and boost efficiency. Get Notion free. Notion + creators. Free + paid. Popular. 309 Templates.
General to-do items always display below all courses [2]. To-do items can be edited at any time by clicking the Edit icon [3]. To add a To-Do item, click the Add Item icon [4]. Note: You can also use the Calendar to add to-do items, which will also display in the List View Dashboard.
THAT list is built based on what assignments have sync turned on. If you have older assignments, maybe from a prior quarter or semester, I suggest turning of the sync for those assignments. You can do that on the Assignments page by just clicking on the sync symbol on the far right for each assignment you want to turn off. So go from green to ...
We predict the assignment of one modality from the representation of another modality data, enforcing consistent assignments for paired multimodal data. This allows vision and language representations to contain the same information, grounding the frozen LLMs' word embedding space in visual data. Moreover, a robust semantic taxonomy of LLMs can ...
Twins right fielder Max Kepler is set to start a rehab assignment. Kepler was placed on the injured list on April 9 with a right knee contusion, but according to multiple reports from Twins beat ...
By The New York Times. Published April 11, 2024 Updated April 16, 2024. The New York Times is providing comprehensive live coverage of the first criminal trial of former President Donald J. Trump ...
vladimir gutierrez dfa. In a series of roster moves on Wednesday, the Milwaukee Brewers designated former highly-regarded prospect Vladimir Gutierrez for assignment. They'll now have a week to ...
MIAMI -- Hours after the Marlins announced left-hander Braxton Garrett sustained a setback in his rehab assignment, No. 3 prospect Max Meyer was optioned to Triple-A Jacksonville and slugger Jake Burger was headed to the injured list, the club needed others to step up. Right-hander Edward Cabrera was up to
Once your classes have been created, you can start creating assignments. 3. Create an Assignment. Once your class is ready, it's time to set up your first assignment. A Turnitin assignment forms the basis of accepting student submissions. Once your assignments are set up, you start adding students to your class. 4.
"Angels on Assignment" is a new, volunteer-based program launched by Maui Cancer Resources to support Maui cancer patients as they get through chemotherapy or radiation treatment.
3. a is a pointer to the list [1,2]. When you do the assignment b = a the value of b is the address of the list [1,2]. So when you do a.append(3) you are not actually changing a, you are changing the list that a points to. Since a and b both point to the same list, they both appear to change when you modify the other.
Manager Kevin Cash said Lowe, the team's most productive left-handed hitter last season, is slated to begin a rehab assignment Thursday with Triple-A Durham. And he might not need too much time ...
He didn't take to that move, posting a 7.11 ERA in 10 outings before being designated for assignment. The 29 other clubs passed on the chance to grab him off waivers and he was sent outright to ...
NEW YORK — Ke'Bryan Hayes was scratched from the Pirates lineup Tuesday evening just two hours before first pitch against the Mets. Pirates...