- Data Types in Go
- Go Keywords
- Go Control Flow
- Go Functions
- GoLang Structures
- GoLang Arrays
- GoLang Strings
- GoLang Pointers
- GoLang Interface
- GoLang Concurrency
- fmt.Fprint() Function in Golang With Examples
- fmt.Sscanln() Function in Golang With Examples
- fmt.Sscan() Function in Golang With Examples
- fmt.Fscanln() Function in Golang With Examples
- fmt.Fscan() Function in Golang With Examples
- fmt.Sprintln() Function in Golang With Examples
- fmt.Sprintf() Function in Golang With Examples
- fmt.Sprint() Function in Golang With Examples
- fmt.Println() Function in Golang With Examples
- fmt.Printf() Function in Golang With Examples
- fmt.print() Function in Golang With Examples
- fmt.Fprintln() Function in Golang With Examples
- fmt.Fprintf() Function in Golang With Examples
- fmt.Errorf() Function in Golang With Examples
- fmt.Scanf() Function in Golang With Examples
- fmt.Scanln() Function in Golang With Examples
- fmt.Sscanf() Function in Golang With Examples
- fmt.Fscanf() Function in Golang With Examples
- Compare Println vs Printf in Golang with Examples

fmt.Scan() Function in Golang With Examples
In Go language, fmt package implements formatted I/O with functions analogous to C’s printf() and scanf() function. The fmt.Scan() function in Go language scans the input texts which is given in the standard input, reads from there and stores the successive space-separated values into successive arguments. Moreover, this function is defined under the fmt package. Here, you need to import the “fmt” package in order to use these functions.
Syntax:
Here, “a …interface{}” receives each type of the given texts. Returns: It returns the number of items successfully scanned.
Example 1:
Input:
Output:
Example 2:
Please Login to comment...
Similar reads.
- Go Language
- 5 Reasons to Start Using Claude 3 Instead of ChatGPT
- 6 Ways to Identify Who an Unknown Caller
- 10 Best Lavender AI Alternatives and Competitors 2024
- The 7 Best AI Tools for Programmers to Streamline Development in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Go by Example : Multiple Return Values
Next example: Variadic Functions .
by Mark McGranaghan and Eli Bendersky | source | license
Functions in Golang
Gurleen Sethi on 01 May 2022

Getting Started #
Functions are at the core of Golang , they are easy to understand, fun to work with and surprisingly provide a decent amount of flexibility. In this article I aim to teach you the main aspects of functions.
As always, I recommend you to try out all the examples by hand 👨🏻💻 the more you practice, the more you understand, and the more you retain. Go Playground and GoPlay are good online websites to quickly write and run some go code.
Declaring Functions #
First thing you do when you write GO code is to declare the main function.
We declare functions using the func keyword followed by the name of the function ( main in this case).
A custom hello world function would look something like this.
Function Arguments #
Let's add an argument to our SayHello function.
Just like that you can keep adding as many arguments as required.
If there are two arguments of the same type declared back to back, you can omit the type declaration of the arguments except the last one, so here firstName and lastName are declared back to back and have the same type string , we can omit the type for firstName .
Variadic Functions #
Go allows you to create functions where you can pass any number of arguments when calling the function. Let's declare a function that will take any number of string s and print them separately on each line.
You can make an argument a variadic argument using the ... syntax, here ...string will accept any number of string s when PrintLines is being called.
An important thing to note here is that inside PrintLines function the type of argument lines is []string , so it is a slice of strings. Whenever you use variadic arguments it will be a slice of a type.
⚠ Note : Variadic argument can only be the final argument of the function, once you have a variadic argument declared you cannot declare anything else after it, so the below code will not compile.
Function Returns #
Let's take our SayHello function, but this time rather than printing lets return the string.
Multiple Return Values #
In golang you can return as many values as you want, lets change the SayHello function to return the line as well as its length.
Ignoring values in multiple return #
When a function returns multiple values, you have to capture all of the variables, you don't get a choice to store just one value in a variable.
To solve this problem go provides a way to ignore a value by using _ . Whatever value you want to ignore, rather than storing it in a variable, use _ .
Basically, you are telling go, "Hey GO! I acknowledge that this function returns two variables, but I don't care about the second one" . Some people find this annoying, but trust me this is a very powerful concept which makes you think about everything that the function returns.
The error Pattern #
I will cover error handling in golang in another article but it is worth touching this topic briefly because it shows up everywhere, in golang there is no try/catch , the way we handle errors is by returning them from functions. Let's see an example.
Read the above example 3 times, understand it properly because if you plan to use go for serious development this pattern of error handling is everywhere, literally everywhere.
It is nothing compliated, a function returns an error type, you store the error in a variable, if the error is not nil there is an error, else you proceed with normal execution 🚗.

Named returns #
Go allows you to pre-declare return variables when declaring the return types of a function, let's see how this works.
Look at the return types of SayHello function, not only do we specify the type but also the name, what GO does is it makes the line and length variable available inside the function body as variables , you can change the value of these variables inside the function body. When writing the return statement, you can just use the return keyword and go will automatically return these variables, this is called naked return .
⚠️ While this feature of GO might look appealing and it has its benefits, please try to avoid using it, using named return can make your code hard to read and understand. Even the official "A Tour of Go" says it:
Naked return statements should be used only in short functions, as with the example shown here. They can harm readability in longer functions.
Be a good gopher .
The defer Keyword #
When calling a function (B) from within a function (A), GO allows you to defer the execution of the function (B) until the calling function (A) finishes its execution. This can be achieved using the defer keyword.
In the above example SayHelloA calls the SayHelloB function using the defer keyword, what this will do is defer the execution of SayHelloB until the complete body of SayHelloA is executed, so it will first print Hello A and then it prints Hello B . If you remove the defer keyword SayHelloB is executed first and then the print statement in SayHelloA is executed.
Notice closely that it seems like you are calling SayHelloB function because there are () after the function, but that is not the case, when using defer keyword you have to use the braces () , but the function is not called immediately it will be deferred.
Here is another example where we are calculating the execution time of a function.
In the above example we are using the time package to store the start and end time of the function, we could have calculated the execution time by just placing the end time calulcate statement after the for loop, but what this pattern allows us to do is create a general function that we can use everywhere, let's see how.
In case you have multiple defer s in a function, the execution order is last in first out, so the last defer will be executed first.
Deferring a function execution is very helpful functionality and you will be using it often when writing software using GO.
This article has been on the lengthier side but now you have the ability to write effective functions in GO 🔨. There is much more to functions that we haven't covered in this article (such as methods), I will cover those topics in another article so keep an eye 👀 on the blog for updates.
Get notified once/twice per month when new articles are published.

Related Articles
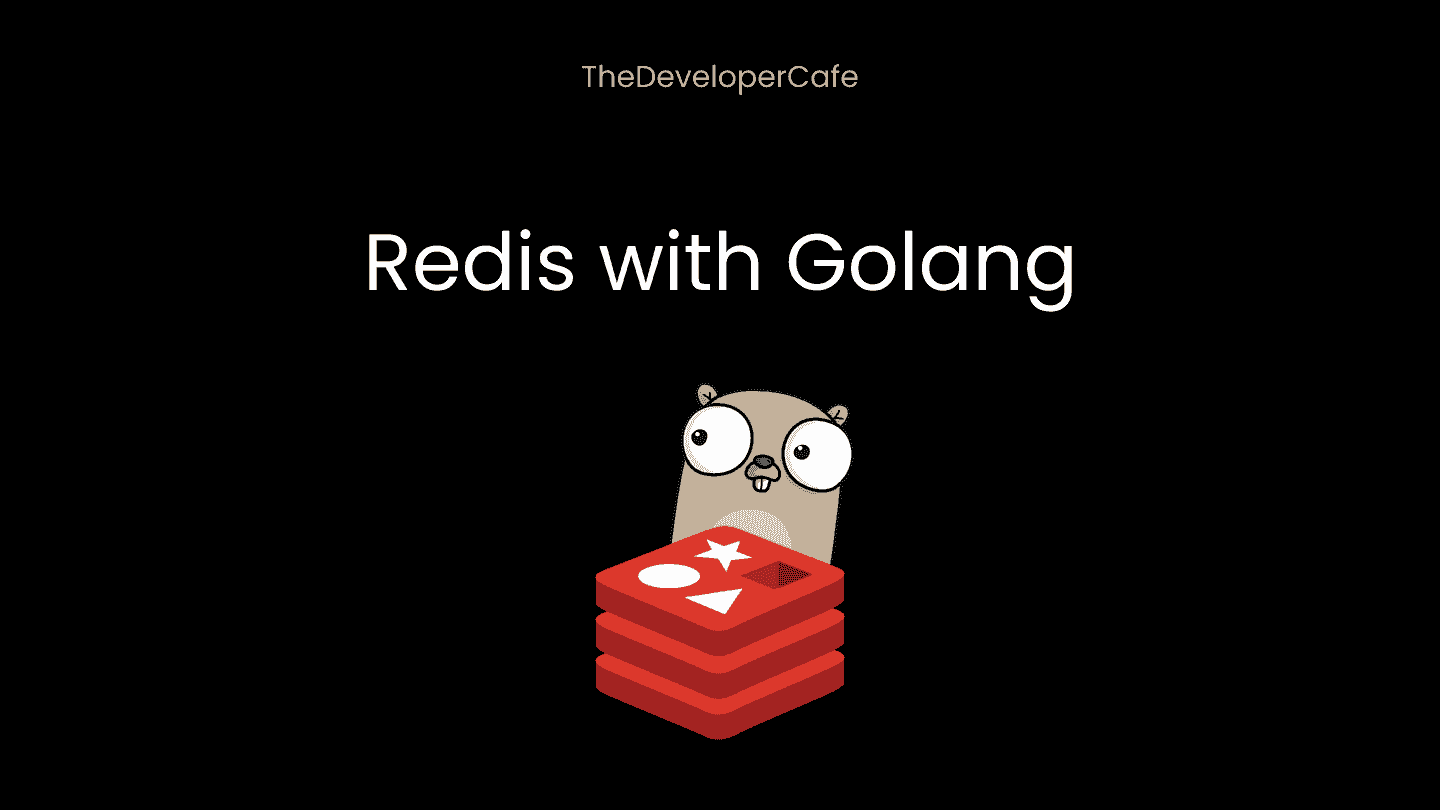
Redis with Golang
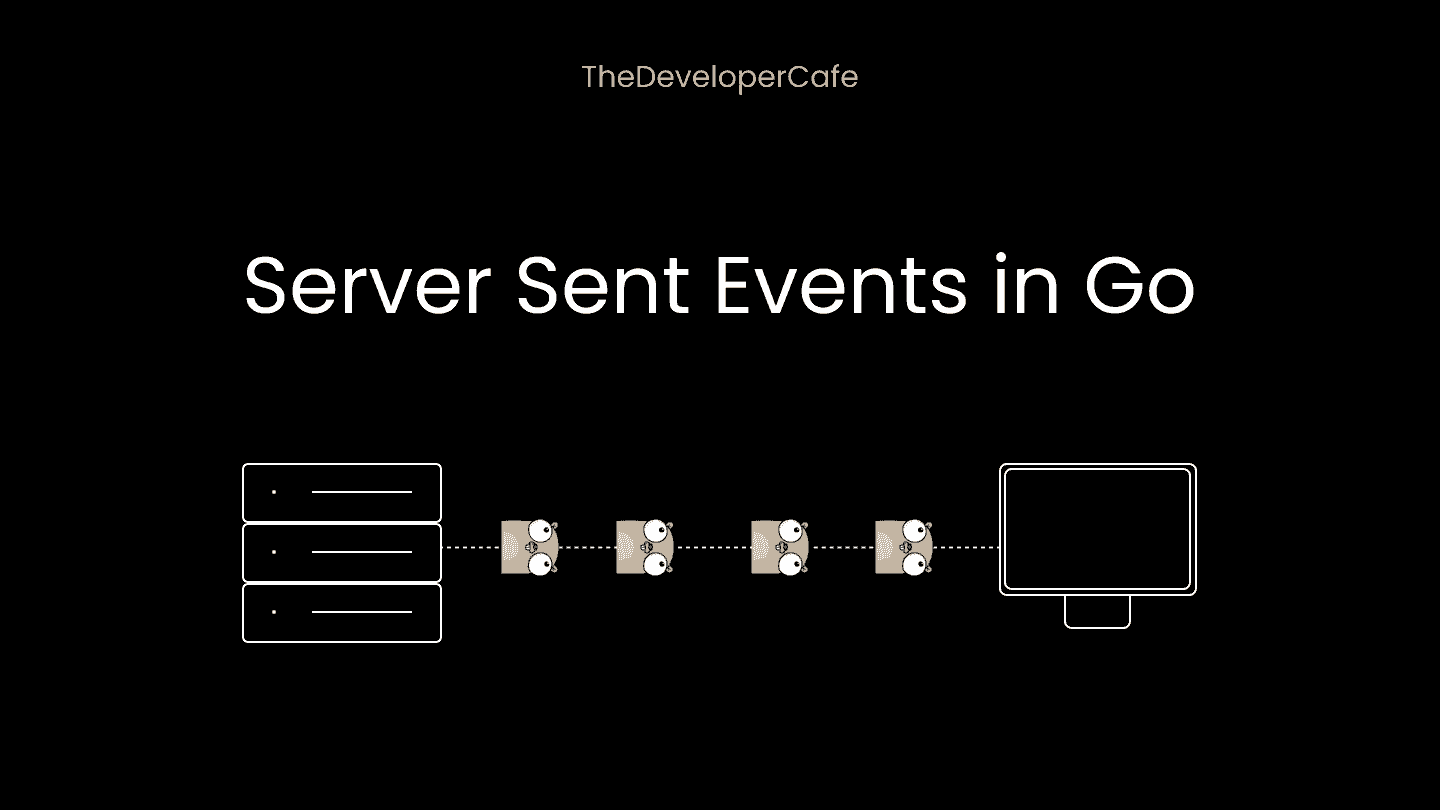
Server Sent Events in Go
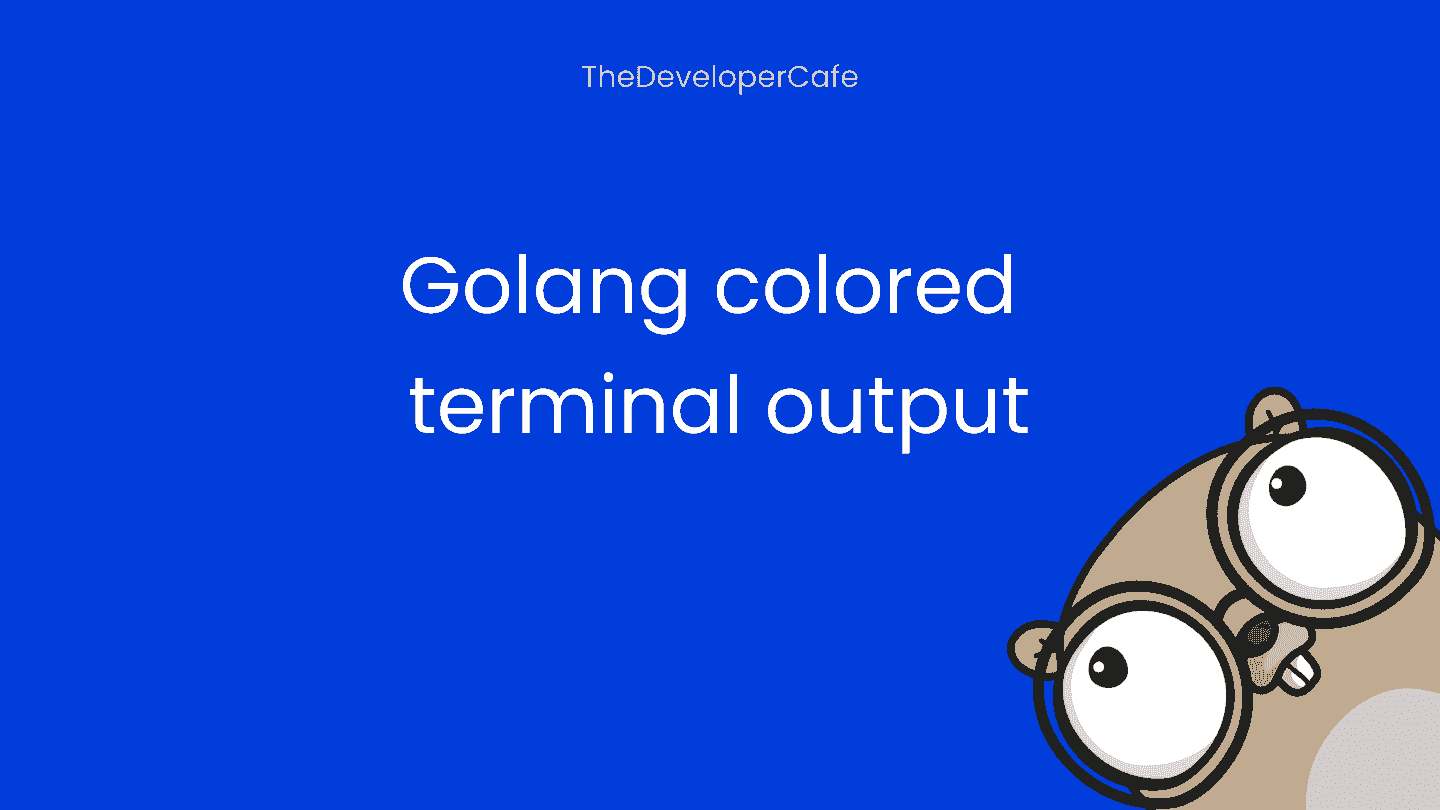
Golang color terminal output with fatih/color

- Data Structure
- Coding Problems
- C Interview Programs
- C++ Aptitude
- Java Aptitude
- C# Aptitude
- PHP Aptitude
- Linux Aptitude
- DBMS Aptitude
- Networking Aptitude
- AI Aptitude
- MIS Executive
- Web Technologie MCQs
- CS Subjects MCQs
- Databases MCQs
- Programming MCQs
- Testing Software MCQs
- Digital Mktg Subjects MCQs
- Cloud Computing S/W MCQs
- Engineering Subjects MCQs
- Commerce MCQs
- More MCQs...
- Machine Learning/AI
- Operating System
- Computer Network
- Software Engineering
- Discrete Mathematics
- Digital Electronics
- Data Mining
- Embedded Systems
- Cryptography
- CS Fundamental
- More Tutorials...
- Tech Articles
- Code Examples
- Programmer's Calculator
- XML Sitemap Generator
- Tools & Generators
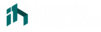
Home » Golang » Golang Find Output Programs
Golang Basics | Find Output Programs | Set 1
This section contains the Golang basics find output programs (set 1) with their output and explanations. Submitted by Nidhi , on August 06, 2021
Explanation:
In the above program, we used fmt.Print() , fmt.Println() , and fmt.Printf() functions to print the value of var1 variable.
There is no such printf() function in fmt package, the correct function is Printf() .
The above program will generate a syntax error because we created the variable var1 but it was not used.
While declaring the variable var1 , we used data type " Int " which is not defined in Golang. The correct data type for integer is " int ".
The above program will generate a syntax error, because the function fmt.Printf() returns two values but we used only one value as an lvalue.
Golang Find Output Programs »
Comments and Discussions!
Load comments ↻
- Marketing MCQs
- Blockchain MCQs
- Artificial Intelligence MCQs
- Data Analytics & Visualization MCQs
- Python MCQs
- C++ Programs
- Python Programs
- Java Programs
- D.S. Programs
- Golang Programs
- C# Programs
- JavaScript Examples
- jQuery Examples
- CSS Examples
- C++ Tutorial
- Python Tutorial
- ML/AI Tutorial
- MIS Tutorial
- Software Engineering Tutorial
- Scala Tutorial
- Privacy policy
- Certificates
- Content Writers of the Month
Copyright © 2024 www.includehelp.com. All rights reserved.
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
assignment mismatch: 2 variables but uuid.NewV4 returns 1 values #34280
chingiz2387 commented Sep 13, 2019
ianlancetaylor commented Sep 13, 2019
Sorry, something went wrong.
agnivade commented Sep 13, 2019
Fercho120 commented Jan 13, 2020
No branches or pull requests

IMAGES
VIDEO
COMMENTS
This function is returning 2 values, thus we have to use 2 variables. So you have to use. config := mollie.NewConfig(true, mollie.OrgTokenEnv) mollieClient, err := mollie.NewClient(client, config) assignment mismatch: 1 variable but mollie.NewClient returns 2 values will be resolved with this change.
./main.go:11:5 assignment mismatch: 2 variables but uuid.NewV4() returns 1 values In the environment where I encounter this problem, in Visual Studio Code when I hover with mouse over the call to uuid.NewV4() I see: func uuid.NewV4() (uuid.UUID, error) uuid.NewV4 on pkg.go.dev NewV4 returns random generated UUID. and hover over uuid shows:
The fmt.Scan () function in Go language scans the input texts which is given in the standard input, reads from there and stores the successive space-separated values into successive arguments. Moreover, this function is defined under the fmt package. Here, you need to import the "fmt" package in order to use these functions. Syntax: Here ...
The issue is this: Christophe_Meessen: func2() returns three values, and you assign the returned values of func2 to two variables. func2 expects three variables. If you post the definition of func2 we can point out the specific location where this is happening, but it might be more useful for the long term to go through the Go tour as Jakob ...
proposal: spec: require return values to be explicitly used or ignored (Go 2) Today, if a Go function returns both a value and an error, the user must either …. Some creative solutions were put forward but it didn't get much traction and at this point it would break the compatibility promise. Ah!
If I use fmt.Println it all works fine. But if I use fmt.Printf I get the error: #command-line-arguments ./main.go:10:31: multiple-value addNavg() in single-value context I read the existing threads and the first one I came across, the OP of that thread had noticed issue with fmt.Println. But I'm getting it for fmt.Printf. Where am I going ...
import "fmt" The (int, int) in this function signature shows that the function returns 2 ints. func vals (int, int) {return 3, 7} func main {Here we use the 2 different return values from the call with multiple assignment. a, b:= vals fmt. Println (a) fmt. Println (b) If you only want a subset of the returned values, use the blank identifier ...
% cat /tmp/x.go package p func f() string func _() { x := f(), 1, 2 } % go tool compile /tmp/x.go /tmp/x.go:6:4: assignment mismatch: 1 variables but f returns 3 values % Narrator: f does not return 3 values. Been this way back to Go 1.1...
When a function returns many values, Go requires us to assign each to a variable. In the last example, we do this by providing names for the two values returned from the capitalize function. These names should be separated by commas and appear on the left-hand side of the := operator. The first value returned from capitalize will be assigned to ...
assignment mismatch: 1 variable but select_user.Exec returns 2 values However we can see from the client it returns a single string... sqlite> SELECT username FROM users WHERE username='ANDY';
./prog.go:17:11: assignment mismatch: 1 variable but reader.ReadString returns 2 values Reason for the failure is ReadString method returned two values, but we assigned only one value. If you check the ReadString function implementation, Reference — bufio package — bufio — Go Packages , you will find that it returns a string message if ...
Mastering Advanced Error Handling in Golang: Build Robust Applications with Graceful Error Management, Custom Types, and More!
When a function returns multiple values, you have to capture all of the variables, you don't get a choice to store just one value in a variable. // `SayHello` same as above func main {line := SayHello ("First") // this will not compile fmt. Println (line)} // compilation error: "assignment mismatch: 1 variable but SayHello returns 2 values"
What version of SQLBoiler are you using (sqlboiler --version)? v3.0.0-rc9 If this happened at runtime what code produced the issue? Tried: sqlboiler psql --add-global-variants --no-context and sqlboiler psql both generates models but go ...
Saved searches Use saved searches to filter your results more quickly
./prog.go:8:7: assignment mismatch: 1 variable but fmt.Printf returns 2 values ./prog.go:9:2: cannot refer to unexported name fmt.println Explanation: The above program will generate a syntax error, because the function fmt.Printf() returns two values but we used only one value as an lvalue. Golang Find Output Programs »
The function signature for split.String is. func Split(s, sep string) []string. So it returns a slice of strings and only returns one type of value. It doesn't return any errors. I haven't tried but I would assume if the original value is equal to the only item in return value, that means the original string doesn't contain the delimiter.
assignment mismatch: 2 variables but uuid.NewV4 returns 1 values #34280. Closed chingiz2387 opened this issue Sep 13, 2019 · 3 comments Closed assignment mismatch: 2 variables but uuid.NewV4 returns 1 values #34280. chingiz2387 opened this issue Sep 13, 2019 · 3 ... "cannot initialize 2 variables with 1 values", "source": "LSP ...
As detailed above (with fmt.Println()), we can pass the return values of any function that has at least 1 return value to wrap() as the values of its input parameters. Now using this wrap() function, see the following example:
It's less of a "design decision" than just how the language works. fmt.Printf is a function like any other, written in Go, and not a special language construct. There's no special handling, the compiler doesn't parse the input printf string specially because it's just a function call like any other as far as it cares.