

Loops in C: For, While, Do While looping Statements [Examples]

What is Loop in C?
Looping Statements in C execute the sequence of statements many times until the stated condition becomes false. A loop in C consists of two parts, a body of a loop and a control statement. The control statement is a combination of some conditions that direct the body of the loop to execute until the specified condition becomes false. The purpose of the C loop is to repeat the same code a number of times.
Types of Loops in C
Depending upon the position of a control statement in a program, looping statement in C is classified into two types:
1. Entry controlled loop
2. Exit controlled loop
In an entry control loop in C, a condition is checked before executing the body of a loop. It is also called as a pre-checking loop.
In an exit controlled loop , a condition is checked after executing the body of a loop. It is also called as a post-checking loop.
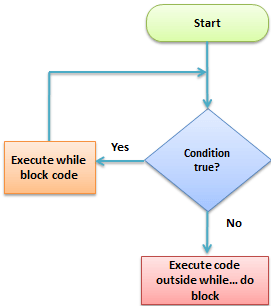
The control conditions must be well defined and specified otherwise the loop will execute an infinite number of times. The loop that does not stop executing and processes the statements number of times is called as an infinite loop . An infinite loop is also called as an “ Endless loop .” Following are some characteristics of an infinite loop:
1. No termination condition is specified.
2. The specified conditions never meet.
The specified condition determines whether to execute the loop body or not.
‘C’ programming language provides us with three types of loop constructs:
1. The while loop
2. The do-while loop
3. The for loop
While Loop in C
A while loop is the most straightforward looping structure. While loop syntax in C programming language is as follows:
Syntax of While Loop in C
It is an entry-controlled loop. In while loop, a condition is evaluated before processing a body of the loop. If a condition is true then and only then the body of a loop is executed. After the body of a loop is executed then control again goes back at the beginning, and the condition is checked if it is true, the same process is executed until the condition becomes false. Once the condition becomes false, the control goes out of the loop.
After exiting the loop, the control goes to the statements which are immediately after the loop. The body of a loop can contain more than one statement. If it contains only one statement, then the curly braces are not compulsory. It is a good practice though to use the curly braces even we have a single statement in the body.
In while loop, if the condition is not true, then the body of a loop will not be executed, not even once. It is different in do while loop which we will see shortly.
Following program illustrates while loop in C programming example:
The above program illustrates the use of while loop. In the above program, we have printed series of numbers from 1 to 10 using a while loop.
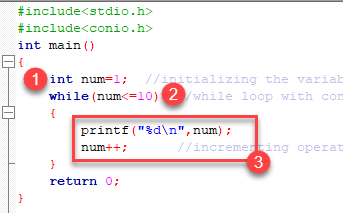
- We have initialized a variable called num with value 1. We are going to print from 1 to 10 hence the variable is initialized with value 1. If you want to print from 0, then assign the value 0 during initialization.
- In a while loop, we have provided a condition (num<=10), which means the loop will execute the body until the value of num becomes 10. After that, the loop will be terminated, and control will fall outside the loop.
- In the body of a loop, we have a print function to print our number and an increment operation to increment the value per execution of a loop. An initial value of num is 1, after the execution, it will become 2, and during the next execution, it will become 3. This process will continue until the value becomes 10 and then it will print the series on console and terminate the loop.
Do-While loop in C
A do…while loop in C is similar to the while loop except that the condition is always executed after the body of a loop. It is also called an exit-controlled loop.
Syntax of do while loop in C programming language is as follows:
Syntax of Do-While Loop in C
As we saw in a while loop, the body is executed if and only if the condition is true. In some cases, we have to execute a body of the loop at least once even if the condition is false. This type of operation can be achieved by using a do-while loop.
In the do-while loop, the body of a loop is always executed at least once. After the body is executed, then it checks the condition. If the condition is true, then it will again execute the body of a loop otherwise control is transferred out of the loop.
Similar to the while loop, once the control goes out of the loop the statements which are immediately after the loop is executed.
The critical difference between the while and do-while loop is that in while loop the while is written at the beginning. In do-while loop, the while condition is written at the end and terminates with a semi-colon (;)
The following loop program in C illustrates the working of a do-while loop:
Below is a do-while loop in C example to print a table of number 2:
In the above example, we have printed multiplication table of 2 using a do-while loop. Let’s see how the program was able to print the series.
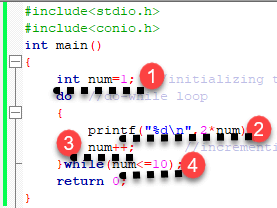
- First, we have initialized a variable ‘num’ with value 1. Then we have written a do-while loop.
- In a loop, we have a print function that will print the series by multiplying the value of num with 2.
- After each increment, the value of num will increase by 1, and it will be printed on the screen.
- Initially, the value of num is 1. In a body of a loop, the print function will be executed in this way: 2*num where num=1, then 2*1=2 hence the value two will be printed. This will go on until the value of num becomes 10. After that loop will be terminated and a statement which is immediately after the loop will be executed. In this case return 0.
For loop in C
A for loop is a more efficient loop structure in ‘C’ programming. The general structure of for loop syntax in C is as follows:
Syntax of For Loop in C
- The initial value of the for loop is performed only once.
- The condition is a Boolean expression that tests and compares the counter to a fixed value after each iteration, stopping the for loop when false is returned.
- The incrementation/decrementation increases (or decreases) the counter by a set value.
Following program illustrates the for loop in C programming example:
The above program prints the number series from 1-10 using for loop.
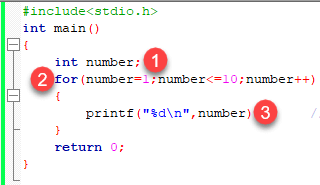
- We have declared a variable of an int data type to store values.
- In for loop, in the initialization part, we have assigned value 1 to the variable number. In the condition part, we have specified our condition and then the increment part.
- In the body of a loop, we have a print function to print the numbers on a new line in the console. We have the value one stored in number, after the first iteration the value will be incremented, and it will become 2. Now the variable number has the value 2. The condition will be rechecked and since the condition is true loop will be executed, and it will print two on the screen. This loop will keep on executing until the value of the variable becomes 10. After that, the loop will be terminated, and a series of 1-10 will be printed on the screen.
In C, the for loop can have multiple expressions separated by commas in each part.
For example:
Also, we can skip the initial value expression, condition and/or increment by adding a semicolon.
Notice that loops can also be nested where there is an outer loop and an inner loop. For each iteration of the outer loop, the inner loop repeats its entire cycle.
Consider the following example with multiple conditions in for loop, that uses nested for loop in C programming to output a multiplication table:
The nesting of for loops can be done up-to any level. The nested loops should be adequately indented to make code readable. In some versions of ‘C,’ the nesting is limited up to 15 loops, but some provide more.
The nested loops are mostly used in array applications which we will see in further tutorials.
Break Statement in C
The break statement is used mainly in the switch statement . It is also useful for immediately stopping a loop.
We consider the following program which introduces a break to exit a while loop:
Continue Statement in C
When you want to skip to the next iteration but remain in the loop, you should use the continue statement.
So, the value 5 is skipped.
Which loop to Select?
Selection of a loop is always a tough task for a programmer, to select a loop do the following steps:
- Analyze the problem and check whether it requires a pre-test or a post-test loop.
- If pre-test is required, use a while or for a loop.
- If post-test is required, use a do-while loop.
- Define loop in C: A Loop is one of the key concepts on any Programming language . Loops in C language are implemented using conditional statements.
- A block of loop control statements in C are executed for number of times until the condition becomes false.
- Loops in C programming are of 2 types: entry-controlled and exit-controlled.
- List various loop control instructions in C: C programming provides us 1) while 2) do-while and 3) for loop control instructions.
- For and while loop C programming are entry-controlled loops in C language.
- Do-while is an exit control loop in C.
- Dynamic Memory Allocation in C using malloc(), calloc() Functions
- Type Casting in C: Type Conversion, Implicit, Explicit with Example
- C Programming Tutorial PDF for Beginners
- 13 BEST C Programming Books for Beginners (2024 Update)
- Difference Between C and Java
- Difference Between Structure and Union in C
- Top 100 C Programming Interview Questions and Answers (PDF)
- calloc() Function in C Library with Program EXAMPLE
Next: Execution Control Expressions , Previous: Arithmetic , Up: Top [ Contents ][ Index ]
7 Assignment Expressions
As a general concept in programming, an assignment is a construct that stores a new value into a place where values can be stored—for instance, in a variable. Such places are called lvalues (see Lvalues ) because they are locations that hold a value.
An assignment in C is an expression because it has a value; we call it an assignment expression . A simple assignment looks like
We say it assigns the value of the expression value-to-store to the location lvalue , or that it stores value-to-store there. You can think of the “l” in “lvalue” as standing for “left,” since that’s what you put on the left side of the assignment operator.
However, that’s not the only way to use an lvalue, and not all lvalues can be assigned to. To use the lvalue in the left side of an assignment, it has to be modifiable . In C, that means it was not declared with the type qualifier const (see const ).
The value of the assignment expression is that of lvalue after the new value is stored in it. This means you can use an assignment inside other expressions. Assignment operators are right-associative so that
is equivalent to
This is the only useful way for them to associate; the other way,
would be invalid since an assignment expression such as x = y is not valid as an lvalue.
Warning: Write parentheses around an assignment if you nest it inside another expression, unless that is a conditional expression, or comma-separated series, or another assignment.
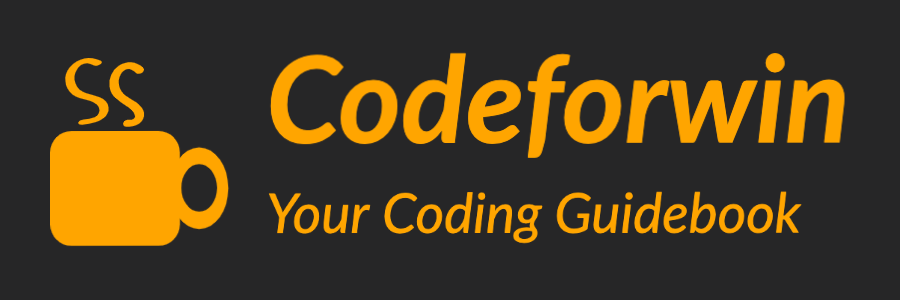
Loop programming exercises and solutions in C
In programming, there exists situations when you need to repeat single or a group of statements till some condition is met. Such as – read all files of a directory, send mail to all employees one after another etc. These task in C programming is handled by looping statements .
Looping statement defines a set of repetitive statements. These statements are repeated with same or different parameters for a number of times. Looping statement is also known as iterative or repetitive statement .
C supports three looping statements.
- do…while loop
In this exercise we will practice lots of looping problems to get a strong grip on loop. This is most recommended C programming exercise for beginners.
Always feel free to drop your queries, suggestions, hugs or bugs down below in the comments section . I always look forward to hear from you.
Required knowledge
Basic C programming , Relational operators , Logical operators , If else , For loop
List of loop programming exercises
- Write a C program to print all natural numbers from 1 to n. – using while loop
- Write a C program to print all natural numbers in reverse (from n to 1) . – using while loop
- Write a C program to print all alphabets from a to z. – using while loop
- Write a C program to print all even numbers between 1 to 100. – using while loop
- Write a C program to print all odd number between 1 to 100.
- Write a C program to find sum of all natural numbers between 1 to n.
- Write a C program to find sum of all even numbers between 1 to n .
- Write a C program to find sum of all odd numbers between 1 to n .
- Write a C program to print multiplication table of any number .
- Write a C program to count number of digits in a number .
- Write a C program to find first and last digit of a number .
- Write a C program to find sum of first and last digit of a number.
- Write a C program to swap first and last digits of a number .
- Write a C program to calculate sum of digits of a number .
- Write a C program to calculate product of digits of a number .
- Write a C program to enter a number and print its reverse .
- Write a C program to check whether a number is palindrome or not.
- Write a C program to find frequency of each digit in a given integer .
- Write a C program to enter a number and print it in words.
- Write a C program to print all ASCII character with their values .
- Write a C program to find power of a number using for loop .
- Write a C program to find all factors of a number .
- Write a C program to calculate factorial of a number .
- Write a C program to find HCF (GCD) of two numbers .
- Write a C program to find LCM of two numbers .
- Write a C program to check whether a number is Prime number or not.
- Write a C program to print all Prime numbers between 1 to n.
- Write a C program to find sum of all prime numbers between 1 to n .
- Write a C program to find all prime factors of a number .
- Write a C program to check whether a number is Armstrong number or not.
- Write a C program to print all Armstrong numbers between 1 to n.
- Write a C program to check whether a number is Perfect number or not .
- Write a C program to print all Perfect numbers between 1 to n .
- Write a C program to check whether a number is Strong number or not .
- Write a C program to print all Strong numbers between 1 to n .
- Write a C program to print Fibonacci series up to n terms .
- Write a C program to find one’s complement of a binary number .
- Write a C program to find two’s complement of a binary number .
- Write a C program to convert Binary to Octal number system .
- Write a C program to convert Binary to Decimal number system .
- Write a C program to convert Binary to Hexadecimal number system .
- Write a C program to convert Octal to Binary number system .
- Write a C program to convert Octal to Decimal number system .
- Write a C program to convert Octal to Hexadecimal number system .
- Write a C program to convert Decimal to Binary number system .
- Write a C program to convert Decimal to Octal number system .
- Write a C program to convert Decimal to Hexadecimal number system .
- Write a C program to convert Hexadecimal to Binary number system .
- Write a C program to convert Hexadecimal to Octal number system .
- Write a C program to convert Hexadecimal to Decimal number system .
- Write a C program to print Pascal triangle upto n rows .
- Star pattern programs – Write a C program to print the given star patterns.
- Number pattern programs – Write a C program to print the given number patterns .

- C Programming Tutorial
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Passing Pointers to Functions
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Pointers to Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Assignment Operators in C
In C language, the assignment operator stores a certain value in an already declared variable. A variable in C can be assigned the value in the form of a literal, another variable, or an expression.
The value to be assigned forms the right-hand operand, whereas the variable to be assigned should be the operand to the left of the " = " symbol, which is defined as a simple assignment operator in C.
In addition, C has several augmented assignment operators.
The following table lists the assignment operators supported by the C language −
Simple Assignment Operator (=)
The = operator is one of the most frequently used operators in C. As per the ANSI C standard, all the variables must be declared in the beginning. Variable declaration after the first processing statement is not allowed.
You can declare a variable to be assigned a value later in the code, or you can initialize it at the time of declaration.
You can use a literal, another variable, or an expression in the assignment statement.
Once a variable of a certain type is declared, it cannot be assigned a value of any other type. In such a case the C compiler reports a type mismatch error.
In C, the expressions that refer to a memory location are called "lvalue" expressions. A lvalue may appear as either the left-hand or right-hand side of an assignment.
On the other hand, the term rvalue refers to a data value that is stored at some address in memory. A rvalue is an expression that cannot have a value assigned to it which means an rvalue may appear on the right-hand side but not on the left-hand side of an assignment.
Variables are lvalues and so they may appear on the left-hand side of an assignment. Numeric literals are rvalues and so they may not be assigned and cannot appear on the left-hand side. Take a look at the following valid and invalid statements −
Augmented Assignment Operators
In addition to the = operator, C allows you to combine arithmetic and bitwise operators with the = symbol to form augmented or compound assignment operator. The augmented operators offer a convenient shortcut for combining arithmetic or bitwise operation with assignment.
For example, the expression "a += b" has the same effect of performing "a + b" first and then assigning the result back to the variable "a".
Run the code and check its output −
Similarly, the expression "a <<= b" has the same effect of performing "a << b" first and then assigning the result back to the variable "a".
Here is a C program that demonstrates the use of assignment operators in C −
When you compile and execute the above program, it will produce the following result −
To Continue Learning Please Login
C Language Tutorial
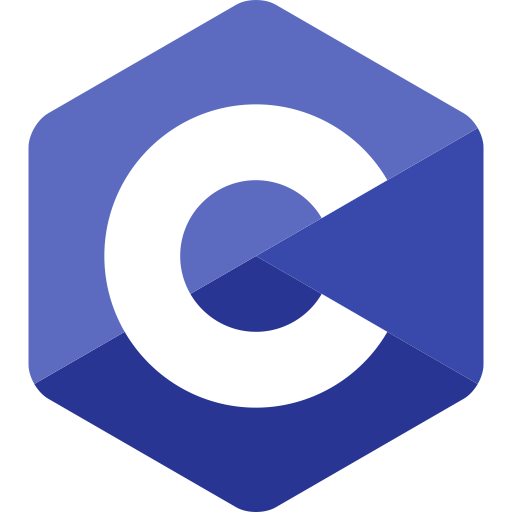
C Operators
C control structure, branch & jump stmt, loop in c programming.
- Examples 2 Program for while loop :-
- Examples 9 Factorial:-
- Examples 10 Check weather a number is prime or not:-
Before understanding the concept of loops, let's understand the problem without them. In the following program, we repeatedly use the same line of code n times, causing the length of the code to increase.
Now before to solve this problem using loop. lets understand the concept of loop.
A loop is a fundamental control structure in programming that allows a set of instructions or a block of code to be executed repeatedly as long as a specific condition is true or for a predetermined number of iterations. Loops are used to automate repetitive tasks and make code more efficient.
Loops are an essential part of programming and are used to perform tasks like iterating over data structures (e.g., arrays), performing calculations, reading data from external sources, and many other tasks where repetition is required to accomplish a specific goal. They help reduce redundancy and make programs more concise and maintainable.
Type of Loops :
In C programming, there are three main types of loops that are commonly used to control the flow of a program and execute a block of code repeatedly until a certain condition is met. These loop types are:
- For loop in c programming
- While loop in c programming
- Do-while loop in c programming
- Learn C Language
- Learn C++ Language
- Learn python Lang
C Data Types
C operators.
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
C File Handling
- C Cheatsheet
C Interview Questions
- C Programming Language Tutorial
- C Language Introduction
- Features of C Programming Language
- C Programming Language Standard
- C Hello World Program
- Compiling a C Program: Behind the Scenes
- Tokens in C
- Keywords in C
C Variables and Constants
- C Variables
- Constants in C
- Const Qualifier in C
- Different ways to declare variable as constant in C
- Scope rules in C
- Internal Linkage and External Linkage in C
- Global Variables in C
- Data Types in C
- Literals in C
- Escape Sequence in C
- Integer Promotions in C
- Character Arithmetic in C
- Type Conversion in C
C Input/Output
- Basic Input and Output in C
- Format Specifiers in C
- printf in C
- Scansets in C
- Formatted and Unformatted Input/Output functions in C with Examples
- Operators in C
- Arithmetic Operators in C
- Unary operators in C
- Relational Operators in C
- Bitwise Operators in C
- C Logical Operators
- Assignment Operators in C
- Increment and Decrement Operators in C
- Conditional or Ternary Operator (?:) in C
- sizeof operator in C
- Operator Precedence and Associativity in C
C Control Statements Decision-Making
- Decision Making in C (if , if..else, Nested if, if-else-if )
- C - if Statement
- C if...else Statement
- C if else if ladder
- Switch Statement in C
- Using Range in switch Case in C
while loop in C
- do...while Loop in C
- For Versus While
- Continue Statement in C
- Break Statement in C
- goto Statement in C
- User-Defined Function in C
- Parameter Passing Techniques in C
- Function Prototype in C
- How can I return multiple values from a function?
- main Function in C
- Implicit return type int in C
- Callbacks in C
- Nested functions in C
- Variadic functions in C
- _Noreturn function specifier in C
- Predefined Identifier __func__ in C
- C Library math.h Functions
C Arrays & Strings
- Properties of Array in C
- Multidimensional Arrays in C
- Initialization of Multidimensional Array in C
- Pass Array to Functions in C
- How to pass a 2D array as a parameter in C?
- What are the data types for which it is not possible to create an array?
- How to pass an array by value in C ?
- Strings in C
- Array of Strings in C
- What is the difference between single quoted and double quoted declaration of char array?
- C String Functions
- Pointer Arithmetics in C with Examples
- C - Pointer to Pointer (Double Pointer)
- Function Pointer in C
- How to declare a pointer to a function?
- Pointer to an Array | Array Pointer
- Difference between constant pointer, pointers to constant, and constant pointers to constants
- Pointer vs Array in C
- Dangling, Void , Null and Wild Pointers in C
- Near, Far and Huge Pointers in C
- restrict keyword in C
C User-Defined Data Types
- C Structures
- dot (.) Operator in C
- Structure Member Alignment, Padding and Data Packing
- Flexible Array Members in a structure in C
- Bit Fields in C
- Difference Between Structure and Union in C
- Anonymous Union and Structure in C
- Enumeration (or enum) in C
C Storage Classes
- Storage Classes in C
- extern Keyword in C
- Static Variables in C
- Initialization of static variables in C
- Static functions in C
- Understanding "volatile" qualifier in C | Set 2 (Examples)
- Understanding "register" keyword in C
C Memory Management
- Memory Layout of C Programs
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Difference Between malloc() and calloc() with Examples
- What is Memory Leak? How can we avoid?
- Dynamic Array in C
- How to dynamically allocate a 2D array in C?
- Dynamically Growing Array in C
C Preprocessor
- C Preprocessor Directives
- How a Preprocessor works in C?
- Header Files in C
- What’s difference between header files "stdio.h" and "stdlib.h" ?
- How to write your own header file in C?
- Macros and its types in C
- Interesting Facts about Macros and Preprocessors in C
- # and ## Operators in C
- How to print a variable name in C?
- Multiline macros in C
- Variable length arguments for Macros
- Branch prediction macros in GCC
- typedef versus #define in C
- Difference between #define and const in C?
- Basics of File Handling in C
- C fopen() function with Examples
- EOF, getc() and feof() in C
- fgets() and gets() in C language
- fseek() vs rewind() in C
- What is return type of getchar(), fgetc() and getc() ?
- Read/Write Structure From/to a File in C
- C Program to print contents of file
- C program to delete a file
- C Program to merge contents of two files into a third file
- What is the difference between printf, sprintf and fprintf?
- Difference between getc(), getchar(), getch() and getche()
Miscellaneous
- time.h header file in C with Examples
- Input-output system calls in C | Create, Open, Close, Read, Write
- Signals in C language
- Program error signals
- Socket Programming in C
- _Generics Keyword in C
- Multithreading in C
- C Programming Interview Questions (2024)
- Commonly Asked C Programming Interview Questions | Set 1
- Commonly Asked C Programming Interview Questions | Set 2
- Commonly Asked C Programming Interview Questions | Set 3
The while Loop is an entry-controlled loop in C programming language. This loop can be used to iterate a part of code while the given condition remains true.
The while loop syntax is as follows:
The below example shows how to use a while loop in a C program
while Loop Structure
The while loop works by following a very structured top-down approach that can be divided into the following parts:
- Initialization: In this step, we initialize the loop variable to some initial value. Initialization is not part of while loop syntax but it is essential when we are using some variable in the test expression
- Conditional Statement: This is one of the most crucial steps as it decides whether the block in the while loop code will execute. The while loop body will be executed if and only the test condition defined in the conditional statement is true.
- Body: It is the actual set of statements that will be executed till the specified condition is true. It is generally enclosed inside { } braces.
- Updation: It is an expression that updates the value of the loop variable in each iteration. It is also not part of the syntax but we have to define it explicitly in the body of the loop.
Flowchart of while loop in C
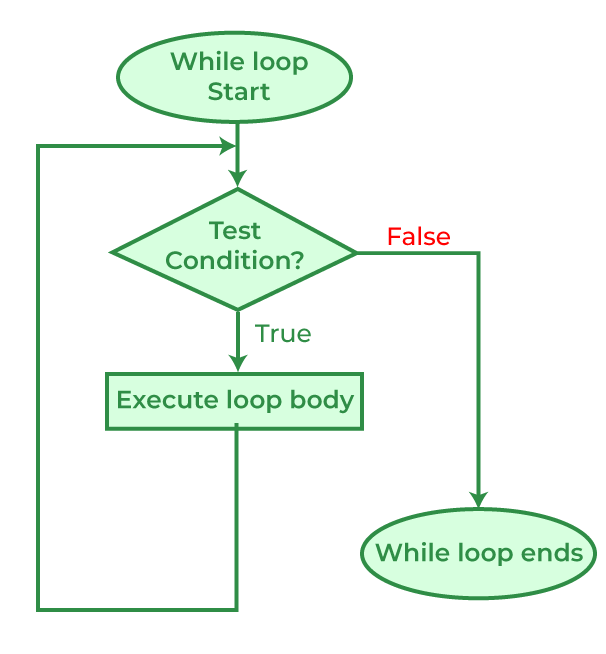
Working of while Loop
We can understand the working of the while loop by looking at the above flowchart:
- STEP 1: When the program first comes to the loop, the test condition will be evaluated.
- STEP 2A: If the test condition is false, the body of the loop will be skipped program will continue.
- STEP 2B: If the expression evaluates to true, the body of the loop will be executed.
- STEP 3: After executing the body, the program control will go to STEP 1. This process will continue till the test expression is true.
Infinite w hile loop
An infinite while loop is created when the given condition is always true. It is encountered by programmers in when:
- The test condition is incorrect.
- Updation statement not present.
As seen in the above example, the loop will continue till infinite because the loop variable will always remain the same resulting in the condition that is always true.
Important Points
- It is an entry-controlled loop.
- It runs the block of statements till the conditions are satiated, once the conditions are not satisfied it will terminate.
- Its workflow is firstly it checks the condition and then executes the body. Hence, a type of pre-tested loop.
- This loop is generally preferred over for loop when the number of iterations is unknown.
Please Login to comment...
Similar reads.

Improve your Coding Skills with Practice
What kind of Experience do you want to share?
cppreference.com
Executes a statement repeatedly, until the value of condition becomes false . The test takes place before each iteration.
[ edit ] Syntax
[ edit ] explanation.
Whether statement is a compound statement or not, it always introduces a block scope . Variables declared in it are only visible in the loop body, in other words,
is the same as
If condition is a declaration such as T t = x , the declared variable is only in scope in the body of the loop, and is destroyed and recreated on every iteration, in other words, such while loop is equivalent to
If the execution of the loop needs to be terminated at some point, break statement can be used as terminating statement.
If the execution of the loop needs to be continued at the end of the loop body, continue statement can be used as shortcut.
[ edit ] Notes
As part of the C++ forward progress guarantee, the behavior is undefined if a loop , unless it is a trivial infinite loop, (since C++26) has no observable behavior (does not make calls to I/O functions, access volatile objects, or perform atomic or synchronization operations) does not terminate. Compilers are permitted to remove such loops.
and the true-condition is a constant expression that evaluates to true , then its statement is replaced with std:: this_thread :: yield ( ) ; ; this replacement is implementation-defined in the freestanding implementation .
[ edit ] Keywords
[ edit ] example, [ edit ] see also.
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 25 February 2023, at 03:31.
- This page has been accessed 246,849 times.
- Privacy policy
- About cppreference.com
- Disclaimers


- C All Exercises & Assignments
Write a C program to check whether a number is even or odd
Description:
Write a C program to check whether a number is even or odd.
Note: Even number is divided by 2 and give the remainder 0 but odd number is not divisible by 2 for eg. 4 is divisible by 2 and 9 is not divisible by 2.
Conditions:
- Create a variable with name of number.
- Take value from user for number variable.
Enter the Number=9 Number is Odd.
Write a C program to swap value of two variables using the third variable.
You need to create a C program to swap values of two variables using the third variable.
You can use a temp variable as a blank variable to swap the value of x and y.
- Take three variables for eg. x, y and temp.
- Swap the value of x and y variable.
Write a C program to check whether a user is eligible to vote or not.
You need to create a C program to check whether a user is eligible to vote or not.
- Minimum age required for voting is 18.
- You can use decision making statement.
Enter your age=28 User is eligible to vote
Write a C program to check whether an alphabet is Vowel or Consonant
You need to create a C program to check whether an alphabet is Vowel or Consonant.
- Create a character type variable with name of alphabet and take the value from the user.
- You can use conditional statements.
Enter an alphabet: O O is a vowel.
Write a C program to find the maximum number between three numbers
You need to write a C program to find the maximum number between three numbers.
- Create three variables in c with name of number1, number2 and number3
- Find out the maximum number using the nested if-else statement
Enter three numbers: 10 20 30 Number3 is max with value of 30
Write a C program to check whether number is positive, negative or zero
You need to write a C program to check whether number is positive, negative or zero
- Create variable with name of number and the value will taken by user or console
- Create this c program code using else if ladder statement
Enter a number : 10 10 is positive
Write a C program to calculate Electricity bill.
You need to write a C program to calculate electricity bill using if-else statements.
- For first 50 units – Rs. 3.50/unit
- For next 100 units – Rs. 4.00/unit
- For next 100 units – Rs. 5.20/unit
- For units above 250 – Rs. 6.50/unit
- You can use conditional statements.
Enter the units consumed=278.90 Electricity Bill=1282.84 Rupees
Write a C program to print 1 to 10 numbers using the while loop
You need to create a C program to print 1 to 10 numbers using the while loop
- Create a variable for the loop iteration
- Use increment operator in while loop
1 2 3 4 5 6 7 8 9 10
- C Exercises Categories
- C Top Exercises
- C Decision Making
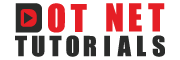
Assignment Solution of Loops
Back to: C++ Tutorials For Beginners and Professionals
Assignment Solution of Loops in C++
In this article, I am going to give you the solutions that we give you as an assignment in our Loops in C++ article. So, please read our Loops in C++ article, if you have not read it yet. First, try to solve the problem by yourself and then only look at the below solutions.
Program to find the sum of first N natural numbers.
Testcase1 : input: N=20 Expected output =210 Note : Also select which loop is better to find the first N natural numbers. Solution : To make a choice of which loop? Let’s understand the question. Here we need to find the sum of N natural numbers.
Let’s say we want to find some of the first 5 numbers. 1,2,3,4,5 sum of these numbers is 1+2+3+4+5. Here I know how many times I need to run the loops. Yes. You guessed it right 5 times as we have 5 numbers. As discussed in our previous article, if we know how many times to execute then the best choice is counter loops (for-loop). However; you can implement it using other loops as well.

Program to find factorial of a number.
Testcase1 : input N=5; Output =120;
Solution : Algorithm for finding factorial of a number in C++ 1. Declare variables i (for loop) and fact (for storing final answer). 2. Initialize fact with value 1 3. Take input from the user whose factorial u want to find (suppose n here) 4. Run a loop from i=n to i>0 for(i=n;i>0;i – -) fact=fact*i; 5. Print fact on the console window

Program to find a palindrome or not.
Testcase1 : input:1212121 Output : palindrome Solution :
Program to find GCD between two numbers
Testcase1 : input: 81 153 Output : 9 Solution :
Program to Perform banking operation.
Solution: Don’t try this exercise, for now, it is good if we try after discussing class, object, and methods.
That’s it for today. We have given the solutions that we give you as an assignment in our Loops in C++ article. If you have a better solution, then please post your solution in the comment box so that other guys will get benefits.
About the Author: Pranaya Rout
Pranaya Rout has published more than 3,000 articles in his 11-year career. Pranaya Rout has very good experience with Microsoft Technologies, Including C#, VB, ASP.NET MVC, ASP.NET Web API, EF, EF Core, ADO.NET, LINQ, SQL Server, MYSQL, Oracle, ASP.NET Core, Cloud Computing, Microservices, Design Patterns and still learning new technologies.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively, introduction.
- Getting Started with C
- Your First C Program
- C Variables, Constants and Literals
- C Data Types
- C Input Output (I/O)
- C Programming Operators
Flow Control
- C if...else Statement
- C while and do...while Loop
- C break and continue
- C switch Statement
- C goto Statement
- C Functions
- C User-defined functions
- Types of User-defined Functions in C Programming
- C Recursion
- C Storage Class
Programming Arrays
C Multidimensional Arrays
Pass arrays to a function in C
Programming Pointers
Relationship Between Arrays and Pointers
- C Pass Addresses and Pointers
- C Dynamic Memory Allocation
- C Array and Pointer Examples
Programming Strings
- C Programming Strings
- String Manipulations In C Programming Using Library Functions
- String Examples in C Programming
Structure and Union
- C structs and Pointers
- C Structure and Function
Programming Files
- C File Handling
C Files Examples
Additional Topics
- C Keywords and Identifiers
- C Precedence And Associativity Of Operators
- C Bitwise Operators
- C Preprocessor and Macros
- C Standard Library Functions
C Tutorials
- Find Largest Element in an Array
- Calculate Average Using Arrays
- Access Array Elements Using Pointer
- Add Two Matrices Using Multi-dimensional Arrays
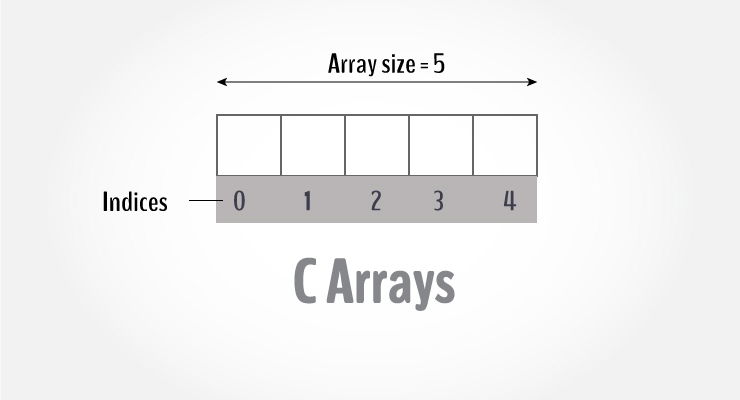
An array is a variable that can store multiple values. For example, if you want to store 100 integers, you can create an array for it.
How to declare an array?
For example,
Here, we declared an array, mark , of floating-point type. And its size is 5. Meaning, it can hold 5 floating-point values.
It's important to note that the size and type of an array cannot be changed once it is declared.
Access Array Elements
You can access elements of an array by indices.
Suppose you declared an array mark as above. The first element is mark[0] , the second element is mark[1] and so on.
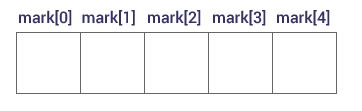
Few keynotes :
- Arrays have 0 as the first index, not 1. In this example, mark[0] is the first element.
- If the size of an array is n , to access the last element, the n-1 index is used. In this example, mark[4]
- Suppose the starting address of mark[0] is 2120d . Then, the address of the mark[1] will be 2124d . Similarly, the address of mark[2] will be 2128d and so on. This is because the size of a float is 4 bytes.
How to initialize an array?
It is possible to initialize an array during declaration. For example,
You can also initialize an array like this.
Here, we haven't specified the size. However, the compiler knows its size is 5 as we are initializing it with 5 elements.
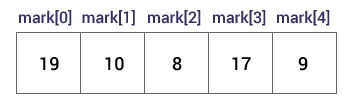
Change Value of Array elements
Input and output array elements.
Here's how you can take input from the user and store it in an array element.
Here's how you can print an individual element of an array.
Example 1: Array Input/Output
Here, we have used a for loop to take 5 inputs from the user and store them in an array. Then, using another for loop, these elements are displayed on the screen.
Example 2: Calculate Average
Here, we have computed the average of n numbers entered by the user.
Access elements out of its bound!
Suppose you declared an array of 10 elements. Let's say,
You can access the array elements from testArray[0] to testArray[9] .
Now let's say if you try to access testArray[12] . The element is not available. This may cause unexpected output (undefined behavior). Sometimes you might get an error and some other time your program may run correctly.
Hence, you should never access elements of an array outside of its bound.
Multidimensional arrays
In this tutorial, you learned about arrays. These arrays are called one-dimensional arrays.
In the next tutorial, you will learn about multidimensional arrays (array of an array) .
Table of Contents
- C Arrays (Introduction)
- Declaring an Array
- Access array elements
- Initializing an array
- Change Value of Array Elements
- Array Input/Output
- Example: Calculate Average
- Array Elements Out of its Bound
Video: C Arrays
Sorry about that.
Related Tutorials

COMMENTS
C Programming While Loop Assignment. 2. Variable assignment in conditional statement. 0. While Loop Variable Initialization and Variable Types(C) 3. Using the assignment operator in a while loop condition in C. 0. While loop doesn't execute statements at each iteration. 0.
As you may know, the original loop is a common C idiom for copying a zero-terminated string. Any C programmer should recognize it at a glance. So in that sense it it already quite readable. The revised version is harder to understand, with the repeated assignment outside and inside the loop.
for Loop. for loop in C programming is a repetition control structure that allows programmers to write a loop that will be executed a specific number of times. for loop enables programmers to perform n number of steps together in a single line. Syntax: for (initialize expression; test expression; update expression) {.
In do-while loop, the while condition is written at the end and terminates with a semi-colon (;) The following loop program in C illustrates the working of a do-while loop: Below is a do-while loop in C example to print a table of number 2: #include<stdio.h>. #include<conio.h>.
The do..while loop is similar to the while loop with one important difference. The body of do...while loop is executed at least once. Only then, the test expression is evaluated. The syntax of the do...while loop is: do {. // the body of the loop.
7 Assignment Expressions. As a general concept in programming, an assignment is a construct that stores a new value into a place where values can be stored—for instance, in a variable. Such places are called lvalues (see Lvalues) because they are locations that hold a value. An assignment in C is an expression because it has a value; we call it an assignment expression.
The program is an example of infinite while loop. Since the value of the variable var is same (there is no ++ or - operator used on this variable, inside the body of loop) the condition var<=2 will be true forever and the loop would never terminate. Examples of infinite while loop. Example 1:
In programming, a loop is used to repeat a block of code until the specified condition is met. C programming has three types of loops: for loop; while loop; do...while loop; We will learn about for loop in this tutorial. In the next tutorial, we will learn about while and do...while loop.
Reverse a number. Calculate the power of a number. Check whether a number is a palindrome or not. Check whether an integer is prime or Not. Display prime numbers between two intervals. Check Armstrong number. Display Armstrong numbers between two intervals. Display factors of a number. Print pyramids and triangles.
List of loop programming exercises. Write a C program to print all natural numbers from 1 to n. - using while loop. Write a C program to print all natural numbers in reverse (from n to 1). - using while loop. Write a C program to print all alphabets from a to z. - using while loop.
Simple assignment operator. Assigns values from right side operands to left side operand. C = A + B will assign the value of A + B to C. +=. Add AND assignment operator. It adds the right operand to the left operand and assign the result to the left operand. C += A is equivalent to C = C + A. -=.
A loop is a fundamental control structure in programming that allows a set of instructions or a block of code to be executed repeatedly as long as a specific condition is true or for a predetermined number of iterations. Loops are used to automate repetitive tasks and make code more efficient. Loops are an essential part of programming and are ...
Working of while Loop. We can understand the working of the while loop by looking at the above flowchart: STEP 1: When the program first comes to the loop, the test condition will be evaluated. STEP 2A: If the test condition is false, the body of the loop will be skipped program will continue. STEP 2B: If the expression evaluates to true, the ...
For one thing, it limits the scope of loop variables to the loop body itself. For another, it provides an unambiguous place to look for those elements, which is a big readability boost in a large loop. Loops with fixed iteration counts happen to fit the for loop criteria, but that doesn't mean they're the only kind of loops that do. -
attr - (since C++11) any number of attributes condition - any expression which is contextually convertible to bool or a declaration of a single variable with a brace-or-equals initializer.This expression is evaluated before each iteration, and if it yields false, the loop is exited.If this is a declaration, the initializer is evaluated before each iteration, and if the value of the declared ...
Write a C program to print 1 to 10 numbers using the while loop . Description: You need to create a C program to print 1 to 10 numbers using the while loop. Conditions: Create a variable for the loop iteration; Use increment operator in while loop
Solution: Algorithm for finding factorial of a number in C++. 1. Declare variables i (for loop) and fact (for storing final answer). 2. Initialize fact with value 1. 3. Take input from the user whose factorial u want to find (suppose n here) 4.
Getting Started with C; Your First C Program; C Comments; C Variables, Constants and Literals; C Data Types; C Input Output (I/O) C Programming Operators; Flow Control. C if...else Statement; C for Loop; C while and do...while Loop; C break and continue; C switch Statement; C goto Statement; Functions. C Functions; C User-defined functions
A three-vehicle accident occurred this late afternoon, west of the eastbound exit at Loop 250 and A Street causing traffic to be backed up for miles since 5:50 p.m. Credit: City of Midland.
The answer is it doesn't work as an increment. The for loop will have the same result as the while loop below: string st; while (getline(is, st, ' ')) {. v.push_back(st); } One could argue if the for loop example you gave is a desirable coding style. answered Nov 3, 2015 at 11:46.
519K likes, 1,925 comments - nasa on May 9, 2024: "You make a loop-de-loop and pull, and your Sun is looking cool! Late at night on May 7 and in the wee hours of May 8, ...
2. (std::cin >> userIn) will be != 0 if the input succeeded, not if the input was 0. To check both, you can do while ( (std::cint >> userIn) && userIn ). This will first make sure that the input succeeded and then that the number is actually non-zero. answered May 23, 2013 at 18:04.