Introduction
- R installation
- Working directory
- Getting help
- Install packages
Data structures
Data Wrangling
- Sort and order
- Merge data frames
Programming
- Creating functions
- If else statement
- apply function
- sapply function
- tapply function
Import & export
- Read TXT files
- Import CSV files
- Read Excel files
- Read SQL databases
- Export data
- plot function
- Scatter plot
- Density plot
- Tutorials Introduction Data wrangling Graphics Statistics See all

Printing values in R
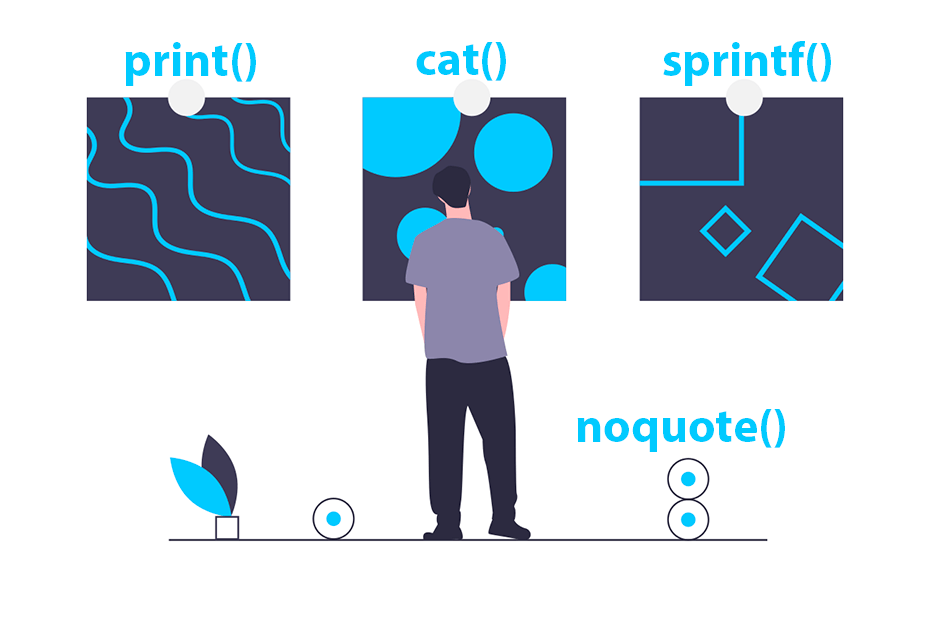
You can print values in R by entering a variable or object on the console or by using the print , sprintf and cat functions. In this tutorial you will learn how to print values in R in different situations.
print function
The print function prints objects on the R console. This function is really useful when you want the code to print some variable, message or object when a code is evaluated, when you want to print some result for debugging purposes or when you to check the current iteration of a for loop printing it on console.
As an example, you can input a string to the print function in order to print a message on console.
You can also print variables and objects and the print function will use the corresponding method to print each type of object. Note that you can also print an object or variable by typing it on the R console.
Nonetheless, the print function allows you to customize the output. For instance, you can use the digits argument to set the desired number of digits.
The print function can be used together with the paste or paste0 functions . In the following example we are printing the current iteration of a for loop.
In order to print a variable or object you don’t really need to use print , as you can just type the name of the variable or object on the console and press enter to print it.
You can print strings, variables, tables, data frames, lists, matrices and any other object with the print function.
Concatenate and print with cat
The cat function concatenates and prints objects without quotes. This function differs from print in many aspects, as it allows you to input several objects but only atomic vectors are allowed . This function also allows you to output values to a file.
Note that by default the function separates the objects with an empty space, but you can customize this specifying a new separator with sep .
If you want the objects to appear on a new line you can use "\n" as separator.
Other interesting difference about print and cat is how each function handles a "\n" inside the string to be printed. While cat adds a new line, the print function prints the result ‘as is’.
You can also use the cat function together with the paste or paste0 functions to print a single object.
Output values into a file with sink
The cat function is useful to write files, as it provides an argument named file to specify the desired output file. You can also append values to the specified file setting append = TRUE .
However, an alternative is to use the sink function to create a file and send all the prints to that file. You will also need to call sink() to close the connection.
Print without quotes with noquote
By default, if you print character values they will be shown with quotes, as in the example below.
However, in R there is a function named noquote that removes the quotes from the output . Note that this function is equivalent to print(x, quote = FALSE) .
sprintf function
The sprintf function is a wrapper of the C function of the same name. It returns a formatted strings based on conversion specifications (see the table below for clarification). For strings you can use %s as shown in the following example.
You can also add more variables to sprintf and each value will be formatted depending on the corresponding conversion specification.
One of the most used conversions is %f to specify the desired number of decimal points (6 by default). In the next example we are setting two decimal points to the value.
The following table summarizes the conversion specifications available in R and its meaning.
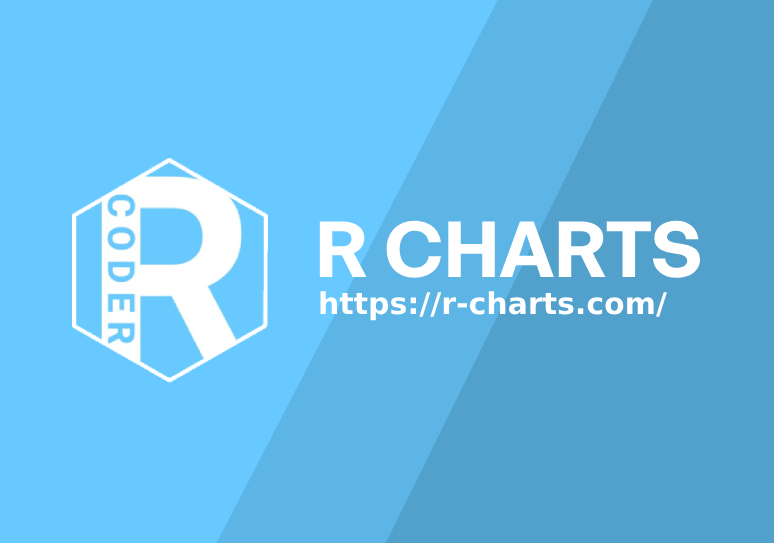
Learn how to plot your data in R with the base package and ggplot2

Free resource
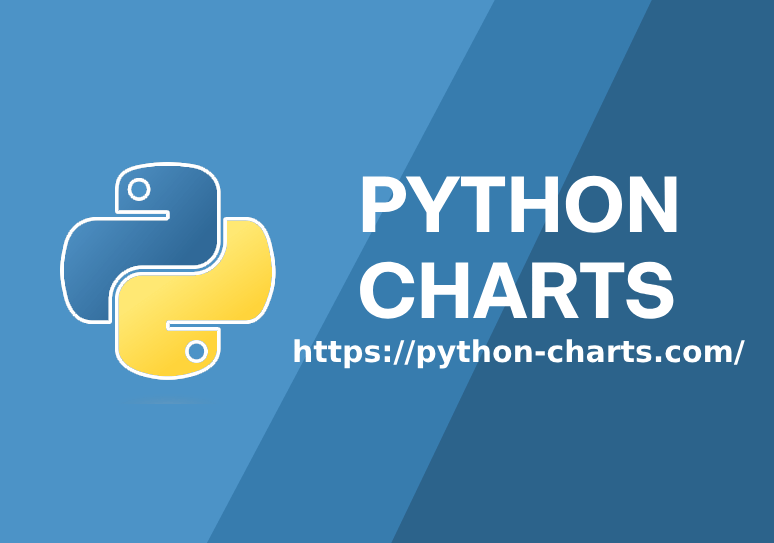
PYTHON CHARTS
Learn how to create plots in Python with matplotlib, seaborn, plotly and folium
Related content
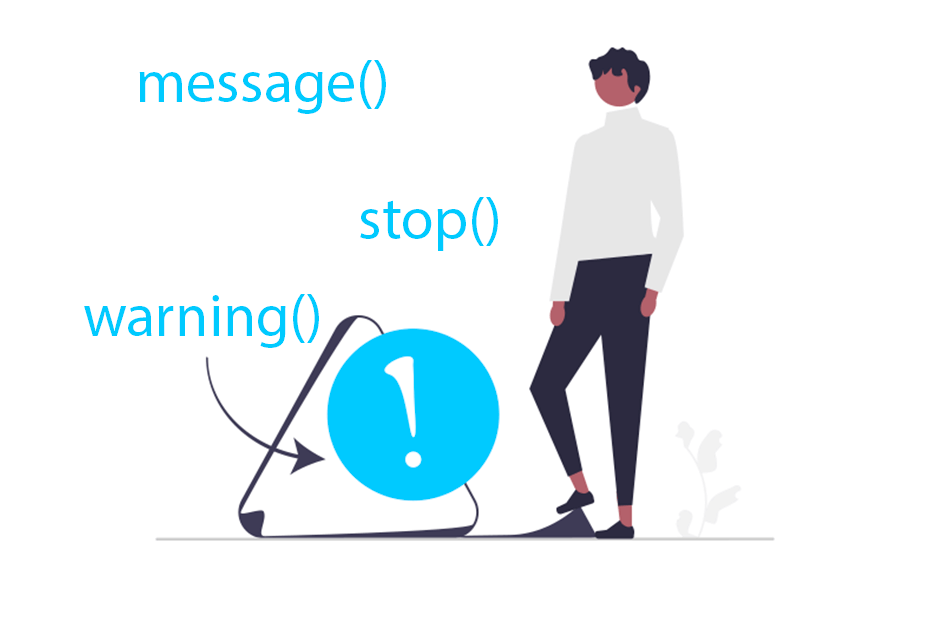
Warning and error messages in R
R introduction
Learn how to add messages in R with the message, warning and stop functions to inform the user and learn how to suppress or list warnings and messages
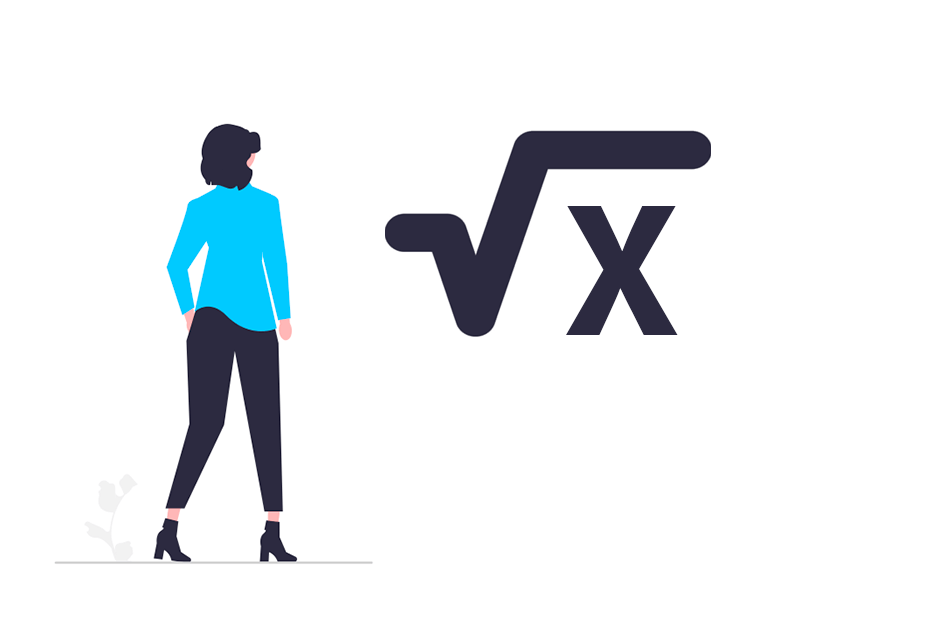
Square root in R
Use the sqrt function to compute the square root of any positive number and learn how to calculate the nth root for any positive number, such as the cube root
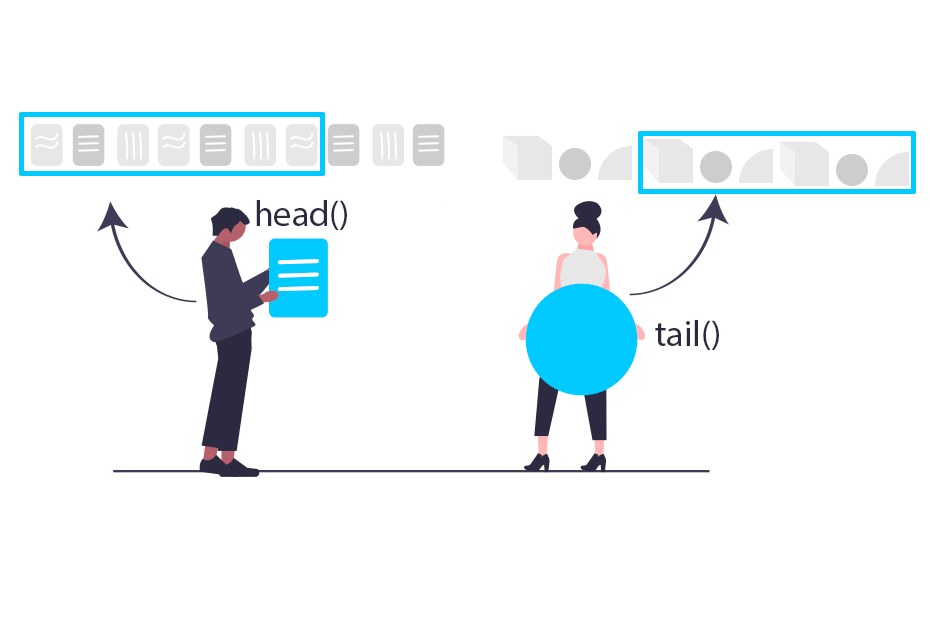
head and tail functions in R
The head and tail functions in R are useful to show the first or last elements or rows of an R object
Try adjusting your search query
👉 If you haven’t found what you’re looking for, consider clicking the checkbox to activate the extended search on R CHARTS for additional graphs tutorials, try searching a synonym of your query if possible (e.g., ‘bar plot’ -> ‘bar chart’), search for a more generic query or if you are searching for a specific function activate the functions search or use the functions search bar .
print: Print Values
Description.
print prints its argument and returns it invisibly (via invisible (x) ). It is a generic function which means that new printing methods can be easily added for new class es.
# S3 method for factor print(x, quote = FALSE, max.levels = NULL, width = getOption("width"), …)
# S3 method for table print(x, digits = getOption("digits"), quote = FALSE, na.print = "", zero.print = "0", right = is.numeric(x) || is.complex(x), justify = "none", …)
# S3 method for function print(x, useSource = TRUE, …)
an object used to select a method.
further arguments passed to or from other methods.
logical, indicating whether or not strings should be printed with surrounding quotes.
integer, indicating how many levels should be printed for a factor; if 0 , no extra "Levels" line will be printed. The default, NULL , entails choosing max.levels such that the levels print on one line of width width .
only used when max.levels is NULL, see above.
minimal number of significant digits, see print.default .
character string (or NULL ) indicating NA values in printed output, see print.default .
character specifying how zeros ( 0 ) should be printed; for sparse tables, using "." can produce more readable results, similar to printing sparse matrices in Matrix.
logical, indicating whether or not strings should be right aligned.
character indicating if strings should left- or right-justified or left alone, passed to format .
logical indicating if internally stored source should be used for printing when present, e.g., if options (keep.source = TRUE) has been in use.
The default method, print.default has its own help page. Use methods ("print") to get all the methods for the print generic.
print.factor allows some customization and is used for printing ordered factors as well.
print.table for printing table s allows other customization. As of R 3.0.0, it only prints a description in case of a table with 0-extents (this can happen if a classifier has no valid data).
See noquote as an example of a class whose main purpose is a specific print method.
Chambers, J. M. and Hastie, T. J. (1992) Statistical Models in S. Wadsworth & Brooks/Cole.
The default method print.default , and help for the methods above; further options , noquote .
For more customizable (but cumbersome) printing, see cat , format or also write . For a simple prototypical print method, see .print.via.format in package tools .
Run the code above in your browser using DataCamp Workspace
Popular Tutorials
Popular examples, learn python interactively, r introduction.
- R Getting Started
- R Variables and Constants
- R Data Types
R Print Output
R flow control.
- R Boolean Expression
- R if...else
- R ifelse() Function
- R while Loop
- R break and next
- R repeat Loop
R Data Structure
- R Data Frame
R Data Visualization
- R Histogram
- R Pie Chart
- R Strip Chart
- R Plot Function
- R Save Plot
- Colors in R
R Data Manipulation
- R Read and Write CSV
- R Read and Write xlsx
- R min() and max()
- R mean, median and mode
- R Percentile
R Additional Topics
- R Objects and Classes
R Tutorials
- Go Print Statement
- Go Variable Scope
- R Functions
- R print() Function
In R, we use the print() function to print values and variables. For example,
In the above example, we have used the print() function to print a string and a variable. When we use a variable inside print() , it prints the value stored inside the variable.
- paste() Function in R
You can also print a string and variable together using the print() function. For this, you have to use the paste() function inside print() . For example,
Notice the use of the paste() function inside print() . The paste() function takes two arguments:
- string - "Welcome to"
- variable - company
By default, you can see there is a space between string Welcome to and the value Programiz .
If you don't want any default separator between the string and variable, you can use another variant of paste() called paste0() . For example,
Now, you can see there is no space between the string and the variable.
- R sprintf() Function
The sprintf() function of C Programming can also be used in R. It is used to print formatted strings. For example,
- String: %s - a formatted string
- %s - format specifier that represents string values
- myString - variable that replaces the format specifier %s
Besides %s , there are many other format specifiers that can be used for different types values.
Let's use some of them in examples.
- R cat() Function
R programming also provides the cat() function to print variables. However, unlike print() , the cat() function is only used with basic types like logical, integer, character, etc.
In the example above, we have used the cat() function to display a string along with a variable. The \n is used as a newline character.
Note : As mentioned earlier, you cannot use cat() with list or any other object.
- Print Variables in R Terminal
You can also print variables inside the R terminal by simply typing the variable name. For example,
Table of Contents
Sorry about that.
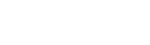
R Programming
- Overview of R
- Installing R on Windows
- Download and Install RStudio on Windows
- Setting Your Working Directory (Windows)
- Getting Help with R
- Installing R Packages
Loading R Packages
Take input and print in r, r objects and attributes.
- Mixing Objects
- Explicit Coercion
- Data Frames
- Missing Values
- R – Operators
- Vectorization
- Dates and Times
- Data Summary
- CSV Files in R
- JSON Files in R
- If-else condition
- While loops
- Repeat, Next, Break
- Generating Random Numbers
- Random Number Seed in R
- Random Sampling
- Data Visualization Using R
The <- symbol is the assignment operator. Assign some value to a variable then you can do either auto-printing for the variables just typing its name or you can use explicit printing.
msg <- "hello R!!!" msg #auto-printing occurs print(msg) #explicit printing
msg [1] "hello R!!!" > print(msg) [1] "hello R!!!"
Try it Yourself
Take input from user:.
When we are working with R in an interactive session, we can use readline() function to take input from the user.
name <- readline(prompt="Enter your name: ")
name <- readline(prompt="Enter your name: ") Enter your name: Kalyan
print(name)
[1] "Kalyan"
Multiple inputs from user using R:
You can combine the statements into a clause like below to take multiple input from user.
{name <- readline(prompt="Enter your name: "); age <- readline(prompt="Enter your age: ")} #OR {name <- readline("Enter your name: "); age <- readline("Enter your age: ")}
{name <- readline(prompt="Enter your name: "); + age <- readline(prompt="Enter your age: ")} Enter your name: Kalyan Enter your age: '26'
print(name) print(age)
print(name) [1] "Kalyan" > print(age) [1] "26"
OR you can make them into a function like below. Both the above and below codes serve the same purpose. First define the function. The call the function.
readlines <- function(…) { lapply(list(…), readline) }
readlines("Enter your name: ","Enter your age: ")
[[1]] [1] "Kalyan" [[2]] [1] “26”
How to create and print variables in R
Get Started With Machine Learning
Learn the fundamentals of Machine Learning with this free course. Future-proof your career by adding ML skills to your toolkit — or prepare to land a job in AI or Data Science.
In this shot, we will learn to create and print variables in the R programming language. A variable is a container for storing data values.
How to create a variable
Unlike many other programming languages, R does not have a command that is used for declaring variables. However, variables are assigned a value using the <- sign. We type the variable name to return the output of the variable that we created.
Code example
Note: In the R language, we can use the normal assignment operators, = and <- , like in any other programming language. However, the use of the = operator is limited to certain contexts.
How to print a variable
The R language is unique. It does not require the print() function to return an output. However, this does not mean that we cannot use the print() function in R. This function is only used when dealing with for loops. In R, we type the name of the variable in order to print it.
Multiple variables
In the R language, we can create multiple variables with the same value in only one line of code.
RELATED TAGS
CONTRIBUTOR
Learn in-demand tech skills in half the time
Mock Interview
Skill Paths
Assessments
Learn to Code
Tech Interview Prep
Generative AI
Data Science
Machine Learning
GitHub Students Scholarship
Early Access Courses
For Individuals
Try for Free
Gift a Subscription
Become an Author
Become an Affiliate
Earn Referral Credits
Cheatsheets
Frequently Asked Questions
Privacy Policy
Cookie Policy
Terms of Service
Business Terms of Service
Data Processing Agreement
Copyright © 2024 Educative, Inc. All rights reserved.

Statistics Made Easy
How to Use the assign() Function in R (3 Examples)
The assign() function in R can be used to assign values to variables.
This function uses the following basic syntax:
assign(x, value)
- x : A variable name, given as a character string.
- value : The value(s) to be assigned to x.
The following examples show how to use this function in practice.
Example 1: Assign One Value to One Variable
The following code shows how to use the assign() function to assign the value of 5 to a variable called new_variable:
When we print the variable called new_variable , we can see that a value of 5 appears.
Example 2: Assign Vector of Values to One Variable
The following code shows how to use the assign() function to assign a vector of values to a variable called new_variable:
When we print the variable called new_variable , we can see that a vector of values appears.
Example 3: Assign Values to Several Variables
The following code shows how to use the assign() function within a for loop to assign specific values to several new variables:
By using the assign() function with a for loop, we were able to create four new variables.
Additional Resources
The following tutorials explain how to use other common functions in R:
How to Use the dim() Function in R How to Use the table() Function in R How to Use sign() Function in R

Published by Zach
Leave a reply cancel reply.
Your email address will not be published. Required fields are marked *

Secure Your Spot in Our PCA Online Course Starting on April 02 (Click for More Info)

assign Function in R (2 Examples)
In this tutorial, I’ll illustrate how to assign values to a variable name using the assign() function in R .
Table of contents:
Sound good? Great, here’s how to do it…
Definition & Basic R Syntax of assign Function
Definition: The assign R function assigns values to a variable name.
Basic R Syntax: Please find the basic R programming syntax of the assign function below.
In the remaining article, I’ll show you two examples for the application of the assign function in the R programming language.
Example 1: Using assign Function to Create New Vector
In Example 1, I’ll show how to use the assign() command to assign numeric values to a new vector object.
Within the assign function, we have to specify the name of the new vector (i.e. “x”) and the values we want to store in this vector object (i.e. five numeric values ranging from 1 to 5):
Let’s have a look at our new data object x:
As you can see based on the previous output of the RStudio console, we have saved a numeric sequence from 1 to 5 in a new variable called x.
Example 2: Using assign & paste0 Functions Dynamically in for-Loop
In Example 2, I’ll show how to use the assign function in combination with the paste0 function to create new variable names within a for-loop dynamically.
Within the assign function, we are using paste0 to create new variable names combining the prefix “x_” with the running index i:
Let’s have a look at the output variables:
Video, Further Resources & Summary
Have a look at the following video of my YouTube channel. I explain the contents of this article in the video:
The YouTube video will be added soon.
In addition, you might have a look at the related tutorials on this homepage .
- for-Loop in R
- paste & paste0 R Functions
- R Functions List (+ Examples)
- The R Programming Language
In this R tutorial you learned how to create new data objects using assign() . In case you have further questions, let me know in the comments section.
Subscribe to the Statistics Globe Newsletter
Get regular updates on the latest tutorials, offers & news at Statistics Globe. I hate spam & you may opt out anytime: Privacy Policy .
2 Comments . Leave new
You are very welcome Hong!
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Post Comment

I’m Joachim Schork. On this website, I provide statistics tutorials as well as code in Python and R programming.
Statistics Globe Newsletter
Get regular updates on the latest tutorials, offers & news at Statistics Globe. I hate spam & you may opt out anytime: Privacy Policy .
Related Tutorials
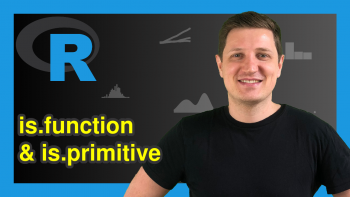
is.function & is.primitive Functions in R (2 Examples)
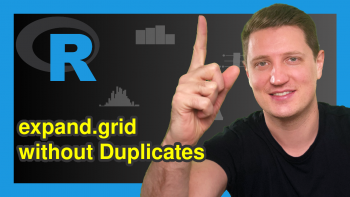
Non-Redundant Version of expand.grid in R (Example)
Popular Tutorials
Popular examples, learn python interactively, r introduction.
- R Reserved Words
- R Variables and Constants
R Operators
- R Operator Precedence and Associativitys
R Flow Control
- R if…else Statement
- R ifelse() Function
- R while Loop
- R break and next Statement
- R repeat loop
- R Functions
- R Return Value from Function
R Environment and Scope
- R Recursive Function
- R Infix Operator
- R switch() Function
R Data Structures
- R Data Frame
R Object & Class
R Classes and Objects
R Reference Class
R Graphs & Charts
- R Histograms
- R Pie Chart
- R Strip Chart
R Advanced Topics
- R Plot Function
- R Multiple Plots
- Saving a Plot in R
- R Plot Color

Related Topics
In this tutorial, you will learn everything about environment and scope in R programming with the help of examples.
In order to write functions in a proper way and avoid unusual errors, we need to know the concept of environment and scope in R.
- R Programming Environment
Environment can be thought of as a collection of objects (functions, variables etc.). An environment is created when we first fire up the R interpreter. Any variable we define, is now in this environment.
The top level environment available to us at the R command prompt is the global environment called R_GlobalEnv . Global environment can be referred to as . GlobalEnv in R codes as well.
We can use the ls() function to show what variables and functions are defined in the current environment. Moreover, we can use the environment() function to get the current environment.
- Example of environment() function
In the above example, we can see that a , b and f are in the R_GlobalEnv environment.
Notice that x (in the argument of the function) is not in this global environment. When we define a function, a new environment is created.
Here, the function f() creates a new environment inside the global environment.
Actually an environment has a frame, which has all the objects defined, and a pointer to the enclosing (parent) environment.
Hence, x is in the frame of the new environment created by the function f . This environment will also have a pointer to R_GlobalEnv .
- Example: Cascading of environments
In the above example, we have defined two nested functions: f and g .
The g() function is defined inside the f() function. When the f() function is called, it creates a local variable g and defines the g() function within its own environment.
The g() function prints "Inside g" , displays its own environment using environment() , and lists the objects in its environment using ls() .
After that, the f() function prints "Inside f" , displays its own environment using environment() , and lists the objects in its environment using ls() .
- R Programming Scope
In R programming, scope refers to the accessibility or visibility of objects (variables, functions, etc.) within different parts of your code.
In R, there are two main types of variables: global variables and local variables.
Let's consider an example:
Global Variables
Global variables are those variables which exist throughout the execution of a program. It can be changed and accessed from any part of the program.
However, global variables also depend upon the perspective of a function.
For example, in the above example, from the perspective of inner_func() , both a and b are global variables .
However, from the perspective of outer_func() , b is a local variable and only a is a global variable. The variable c is completely invisible to outer_func() .
Local Variables
On the other hand, local variables are those variables which exist only within a certain part of a program like a function, and are released when the function call ends.
In the above program the variable c is called a local variable .
If we assign a value to a variable with the function inner_func() , the change will only be local and cannot be accessed outside the function.
This is also the same even if names of both global variables and local variables match.
For example, if we have a function as below.
Here, the outer_func() function is defined, and within it, a local variable a is assigned the value 20 .
Inside outer_func() , there is an inner_func() function defined. The inner_func() function also has its own local variable a , which is assigned the value 30 .
When inner_func() is called within outer_func() , it prints the value of its local variable a (30) . Then, outer_func() continues executing and prints the value of its local variable a (20) .
Outside the functions, a global variable a is assigned the value 10 . This code then prints the value of the global variable a (10) .
- Accessing global variables
Global variables can be read but when we try to assign to it, a new local variable is created instead.
To make assignments to global variables, super assignment operator, <<- , is used.
When using this operator within a function, it searches for the variable in the parent environment frame, if not found it keeps on searching the next level until it reaches the global environment.
If the variable is still not found, it is created and assigned at the global level.
When the statement a <<- 30 is encountered within inner_func() , it looks for the variable a in outer_func() environment.
When the search fails, it searches in R_GlobalEnv .
Since, a is not defined in this global environment as well, it is created and assigned there which is now referenced and printed from within inner_func() as well as outer_func() .
Table of Contents
- Introduction
Sorry about that.
R Tutorials
Programming

R news and tutorials contributed by hundreds of R bloggers
Assignment in r: slings and arrows.
Posted on July 30, 2018 by Chris in R bloggers | 0 Comments
[social4i size="small" align="align-left"] --> [This article was first published on R – Doodling in Data , and kindly contributed to R-bloggers ]. (You can report issue about the content on this page here ) Want to share your content on R-bloggers? click here if you have a blog, or here if you don't.
Having recently shared my post about defensive programming in R on the r/rstats subreddit , I was blown away by the sheer number of comments as much as I was blown away by the insight many displayed. One particular comment by u/guepier struck my attention. In my previous post , I came out quite vehemently against using the = operator to effect assignment in R. u/guepier ‘s made a great point, however:
But point 9 is where you’re quite simply wrong, sorry: never, ever, ever use = to assign. Every time you do it, a kitten dies of sadness.
This is FUD, please don’t spread it. There’s nothing wrong with =. It’s purely a question of personal preference. In fact, if anything <- is more error-prone (granted, this is a very slight chance but it’s still higher than the chance of making an error when using =).
Now, assignment is no doubt a hot topic – a related issue, assignment expressions, has recently led to Python’s BDFL to be forced to resign –, so I’ll have to tread carefully. A surprising number of people have surprisingly strong feelings about assignment and assignment expressions. In R, this is complicated by its unusual assignment structure, involving two assignment operators that are just different enough to be trouble.
A brief history of <-
There are many ways in which <- in R is anomalous. For starters, it is rare to find a binary operator that consists of two characters – which is an interesting window on the R <- operator’s history.
The <- operator, apparently, stems from a day long gone by, when keyboards existed for the programming language eldritch horror that is APL . When R’s precursor, S, was conceived, APL keyboards and printing heads existed, and these could print a single ← character. It was only after most standard keyboard assignments ended up eschewing this all-important symbol that R and S accepted the digraphic <- as a substitute.
OK, but what does it do ?

Because quite peculiarly, there is another way to accomplish a simple assignment in R: the equality sign ( = ). And because on the top level, a <- b and a = b are equivalent, people have sometimes treated the two as being quintessentially identical. Which is not the case . Or maybe it is. It’s all very confusing. Let’s see if we can unconfuse it.
The Holy Writ
The Holy Writ, known to uninitiated as the R Manual , has this to say about assignment operators and their differences:
The operators – R Documentation: {base} assignOps
If this sounds like absolute gibberish, or you cannot think of what would qualify as not being on the top level or a subexpression in a braced list of expressions, welcome to the squad – I’ve had R experts scratch their head about this for an embarrassingly long time until they realised what the R documentation, in its neutron starlike denseness, actually meant.
If it’s in (parentheses) rather than {braces} , = and <- are going to behave weird
To translate the scriptural words above quoted to human speak, this means = cannot be used in the conditional part (the part enclosed by (parentheses) as opposed to {curly braces} ) of control structures, among others. This is less an issue between <- and = , and rather an issue between = and == . Consider the following example:
So far so good: you should not use a single equality sign as an equality test operator. The right way to do it is:
But what about arrow assignment?
Oh, look, it works! Or does it?
The problem is that an assignment will always yield true if successful. So instead of comparing x to 4, it assigned 4 to x , then happily informed us that it is indeed true.
The bottom line is not to use = as comparison operator, and <- as anything at all in a control flow expression’s conditional part. Or as John Chambers notes,
Disallowing the new assignment form in control expressions avoids programming errors (such as the example above) that are more likely with the equal operator than with other S assignments. – John Chambers, Assignments with the = Operator
Chain assignments
One example of where <- and = behave differently (or rather, one behaves and the other throws an error) is a chain assignment. In a chain assignment, we exploit the fact that R assigns from right to left. The sole criterion is that all except the rightmost members of the chain must be capable of being assigned to.
So we’ve seen that as long as the chain assignment is logically valid, it’ll work fine, whether it’s using <- or = . But what if we mix them up?
The bottom line from the example above is that where <- and = are mixed, the leftmost assignment has to be carried out using = , and cannot be by <- . In that one particular context, = and <- are not interchangeable.
A small note on chain assignments: many people dislike chain assignments because they’re ‘invisible’ – they literally return nothing at all. If that is an issue, you can surround your chain assignment with parentheses – regardless of whether it uses <- , = or a (valid) mixture thereof:
Assignment and initialisation in functions
This is the big whammy – one of the most important differences between <- and = , and a great way to break your code. If you have paid attention until now, you’ll be rewarded by, hopefully, some interesting knowledge.
= is a pure assignment operator. It does not necessary initialise a variable in the global namespace. <- , on the other hand, always creates a variable, with the lhs value as its name, in the global namespace. This becomes quite prominent when using it in functions.
Traditionally, when invoking a function, we are supposed to bind its arguments in the format parameter = argument. 1 And as we know from what we know about functions, the keyword’s scope is restricted to the function block. To demonstrate this:
This is expected: a (as well as b , but that didn’t even make it far enough to get checked!) doesn’t exist in the global scope, it exists only in the local scope of the function add_up_numbers . But what happens if we use <- assignment?
Now, a and b still only exist in the local scope of the function add_up_numbers . However, using the assignment operator, we have also created new variables called a and b in the global scope. It’s important not to confuse it with accessing the local scope, as the following example demonstrates:
In other words, a + b gave us the sum of the values a and b had in the global scope. When we invoked add_up_numbers(c <- 5, d <- 6) , the following happened, in order:
- A variable called c was initialised in the global scope. The value 5 was assigned to it.
- A variable called d was initialised in the global scope. The value 6 was assigned to it.
- The function add_up_numbers() was called on positional arguments c and d.
- c was assigned to the variable a in the function’s local scope.
- d was assigned to the variable b in the function’s local scope.
- The function returned the sum of the variables a and b in the local scope.
It may sound more than a little tedious to think about this function in this way, but it highlights three important things about <- assignment:
- In a function call, <- assignment to a keyword name is not the same as using = , which simply binds a value to the keyword.
- <- assignment in a function call affects the global scope, using = to provide an argument does not.
- Outside this context, <- and = have the same effect, i.e. they assign, or initialise and assign, in the current scope.
Phew. If that sounds like absolute confusing gibberish, give it another read and try playing around with it a little. I promise, it makes some sense. Eventually.
So… should you or shouldn’t you?
Which raises the question that launched this whole post: should you use = for assignment at all? Quite a few style guides, such as Google’s R style guide, have outright banned the use of = as assignment operator , while others have encouraged the use of -> . Personally, I’m inclined to agree with them , for three reasons.
- Because of the existence of -> , assignment by definition is best when it’s structured in a way that shows what is assigned to which side. a -> b and b <- a have a formal clarity that a = b does not have.
- Good code is unambiguous even if the language isn’t. This way, -> and <- always mean assignment, = always means argument binding and == always means comparison.
- Many argue that <- is ambiguous, as x<-3 may be mistyped as x<3 or x-3 , or alternatively may be (visually) parsed as x < -3 , i.e. compare x to -3. In reality, this is a non-issue. RStudio has a built-in shortcut ( Alt/⎇ + – ) for
Like with all coding standards, consistency is key. Consistently used suboptimal solutions are superior, from a coding perspective, to an inconsistent mixture of right and wrong solutions.
References [ + ]
The post Assignment in R: slings and arrows appeared first on Doodling in Data .
To leave a comment for the author, please follow the link and comment on their blog: R – Doodling in Data . R-bloggers.com offers daily e-mail updates about R news and tutorials about learning R and many other topics. Click here if you're looking to post or find an R/data-science job . Want to share your content on R-bloggers? click here if you have a blog, or here if you don't.
Copyright © 2022 | MH Corporate basic by MH Themes
Never miss an update! Subscribe to R-bloggers to receive e-mails with the latest R posts. (You will not see this message again.)
Data Visualization
- Statistics in R
- Machine Learning in R
- Data Science in R
Packages in R
- R Tutorial | Learn R Programming Language
Introduction
- R Programming Language - Introduction
- Interesting Facts about R Programming Language
- R vs Python
- Environments in R Programming
- Introduction to R Studio
- How to Install R and R Studio?
- Creation and Execution of R File in R Studio
- Clear the Console and the Environment in R Studio
- Hello World in R Programming
Fundamentals of R
- Basic Syntax in R Programming
- Comments in R
- R Operators
- R - Keywords
- R Data Types
- R Variables - Creating, Naming and Using Variables in R
- Scope of Variable in R
- Dynamic Scoping in R Programming
- Lexical Scoping in R Programming
Input/Output
- Taking Input from User in R Programming
Printing Output of an R Program
- Print the Argument to the Screen in R Programming - print() Function
Control Flow
- Control Statements in R Programming
- Decision Making in R Programming - if, if-else, if-else-if ladder, nested if-else, and switch
- Switch case in R
- For loop in R
- R - while loop
- R - Repeat loop
- goto statement in R Programming
- Break and Next statements in R
- Functions in R Programming
- Function Arguments in R Programming
- Types of Functions in R Programming
- Recursive Functions in R Programming
- Conversion Functions in R Programming
Data Structures
- Data Structures in R Programming
- R - Matrices
- R - Data Frames
Object Oriented Programming
- R - Object Oriented Programming
- Classes in R Programming
- R - Objects
- Encapsulation in R Programming
- Polymorphism in R Programming
- R - Inheritance
- Abstraction in R Programming
- Looping over Objects in R Programming
- S3 class in R Programming
- Explicit Coercion in R Programming
Error Handling
- Handling Errors in R Programming
- Condition Handling in R Programming
- Debugging in R Programming
File Handling
- File Handling in R Programming
- Reading Files in R Programming
- Writing to Files in R Programming
- Working with Binary Files in R Programming
- Packages in R Programming
- Data visualization with R and ggplot2
- dplyr Package in R Programming
- Grid and Lattice Packages in R Programming
- Shiny Package in R Programming
- tidyr Package in R Programming
- What Are the Tidyverse Packages in R Language?
- Data Munging in R Programming
Data Interfaces
- Data Handling in R Programming
- Importing Data in R Script
- Exporting Data from scripts in R Programming
- Working with CSV files in R Programming
- Working with XML Files in R Programming
- Working with Excel Files in R Programming
- Working with JSON Files in R Programming
- Working with Databases in R Programming
- Data Visualization in R
- R - Line Graphs
- R - Bar Charts
- Histograms in R language
- Scatter plots in R Language
- R - Pie Charts
- Boxplots in R Language
- R - Statistics
- Mean, Median and Mode in R Programming
- Calculate the Average, Variance and Standard Deviation in R Programming
- Descriptive Analysis in R Programming
- Normal Distribution in R
- Binomial Distribution in R Programming
- ANOVA (Analysis of Variance) Test in R Programming
- Covariance and Correlation in R Programming
- Skewness and Kurtosis in R Programming
- Hypothesis Testing in R Programming
- Bootstrapping in R Programming
- Time Series Analysis in R
Machine Learning
- Introduction to Machine Learning in R
- Setting up Environment for Machine Learning with R Programming
- Supervised and Unsupervised Learning in R Programming
- Regression and its Types in R Programming
- Classification in R Programming
- Naive Bayes Classifier in R Programming
- KNN Classifier in R Programming
- Clustering in R Programming
- Decision Tree in R Programming
- Random Forest Approach in R Programming
- Hierarchical Clustering in R Programming
- DBScan Clustering in R Programming
- Deep Learning in R Programming
In R there are various methods to print the output. Most common method to print output in R program, there is a function called print() is used. Also if the program of R is written over the console line by line then the output is printed normally, no need to use any function for print that output. To do this just select the output variable and press run button. Example:
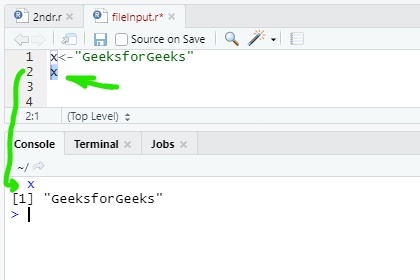
Print output using print() function
Using print() function to print output is the most common method in R. Implementation of this method is very simple.
Syntax: print(“any string”) or, print(variable)
Example:
Print output using paste() function inside print() function
R provides a method paste() to print output with string and variable together. This method defined inside the print() function. paste() converts its arguments to character strings. One can also use paste0() method.
Note: The difference between paste() and paste0() is that the argument sep by default is ” “(paste) and “”(paste0).
Syntax: print(paste(“any string”, variable)) or, print(paste0(variable, “any string”))
Print output using sprintf() function
sprintf() is basically a C library function. This function is use to print string as C language . This is working as a wrapper function to print values and strings together like C language. This function returns a character vector containing a formatted combination of string and variable to be printed.
Syntax: sprintf(“any string %d”, variable) or, sprintf(“any string %s”, variable) or, sprintf(“any string %f”, variable)) etc.
Print output using cat() function
Another way to print output in R is using of cat() function. It’s same as print() function. cat() converts its arguments to character strings. This is useful for printing output in user defined functions.
Syntax: cat(“any string”) or, cat(“any string”, variable)
Print output using message() function
Another way to print something in R by using message() function. This is not used for print output but its use for showing simple diagnostic messages which are no warnings or errors in the program. But it can be used for normal uses for printing output.
Syntax: message(“any string”) or, message(“any string”, variable)
Write output to a file
To print or write a file with a value of a variable there is a function called write() . This function is used a option called table to write a file.
Syntax: write.table(variable, file = “file1.txt”) or, write.table(“any string”, file = “file1.txt”)
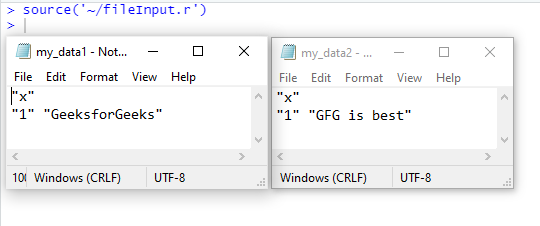
Please Login to comment...
- 10 Best HuggingChat Alternatives and Competitors
- Best Free Android Apps for Podcast Listening
- Google AI Model: Predicts Floods 7 Days in Advance
- Who is Devika AI? India's 'AI coder', an alternative to Devin AI
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- FanNation FanNation FanNation
- SI.COM SI.COM SI.COM
- SI Swimsuit SI Swimsuit SI Swimsuit
- SI Sportsbook SI Sportsbook SI Sportsbook
- SI Tickets SI Tickets SI Tickets
- SI Showcase SI Showcase SI Showcase
- SI Resorts SI Resorts SI Resorts
- TRANSACTIONS
© Isaiah J. Downing-USA TODAY Sports
B/R Offers Interesting Trade Proposal to Get Giants into Bottom of Round 1
B/R has the right idea, but the wrong value. Here is what we would do.
- Author: Ezekiel Trezevant IV , Patricia Traina
- Publish date: Apr 03, 2024
In this story:
The New York Giants have expressed belief in starting quarterback Daniel Jones, but that doesn't mean they won't attempt to draft a quarterback at some point later this month.
But with the top four quarterbacks—Caleb Williams, Drake Maye, Jayden Daniels, and J.J. McCarthy—likely to be off the board by the time the Giants go on the clock at No. 6, New York might very well have to trade back into the bottom of the first round if it wants one of those top guys.
What would such a trade entail? Bleacher Report’s Kristopher Knox proposed this scenario involving the Kansas City Chiefs, who hold Pick No. 32 in the first round:
- Giants Get: 2024 Round 1 Pick (No. 32), 2024 Round 4 Pick (No. 131)
- Chiefs Get: 2024 Round 2 Pick (No. 47), 2024 Round 3 Pick (No. 70)
A scenario involving the Giants trading back into the bottom of the first round for a quarterback (assuming they draft a receiver at No. 6, which is still very much the most realistic scenario at this point, not only makes sense, but it would be less taxing on the Giants draft haul, both this year and next.
To trade up from six likely means the Giants will have to give up a top premium pick in next year's class, something general manager Joe Schoen should approach with caution, especially given this team's remaining needs (some of which may not get addressed this year).
Trading back into the bottom of the first round to have a chance at ORegon's Bo Nix or South Carolina's Spencer Rattler, though, makes more sense as not only would the Giants be able to get a quarterback on the five-year plan (thanks to the option year that first-round picks have in their contracts), but the cost would be far less than the alternative.
So, let's look at this proposal. Knox notes that "the 2024 draft class has an impressive second tier of talent, so teams like the Kansas City Chiefs—who should be eager to augment their roster with rookie contracts—should be plenty interested in trading out of Round 1."
The proposed trade structure, though, seems a little too unrealistic. It's unlikely that Schoen will want to sit out Day 2 of the draft, which he would have to do in this scenario. According to the DraftTek trade value chart , the 32nd pick is worth 590 points, and the compensation proposed here would be worth 670.
Instead, we would propose sending the second-round pick (No. 47) and a 2025 fourth-rounder to the Chiefs because the Giants, per Over the Cap , are currently projected to get a compensatory fourth-round selection next year.
With two such picks, the Giants might be more agreeable to parting with one of those picks, the same as what they did this year when they gave up one of their second-round picks to acquire edge rusher Brian Burns in a trade with the Carolina Panthers.
- Follow and like us on Facebook .
- Submit your questions for our mailbag .
- Follow Patricia Traina on Instagram .
- Check out the Giants Country YouTube Channel .
- Subscribe and like the LockedOn Giants YouTube Channel
Latest Giants News
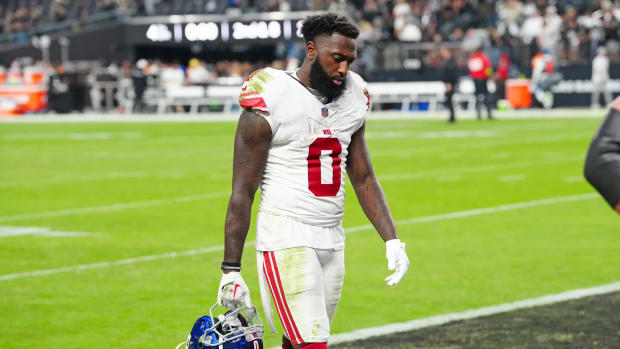
Parris Campbell Signs with Eagles
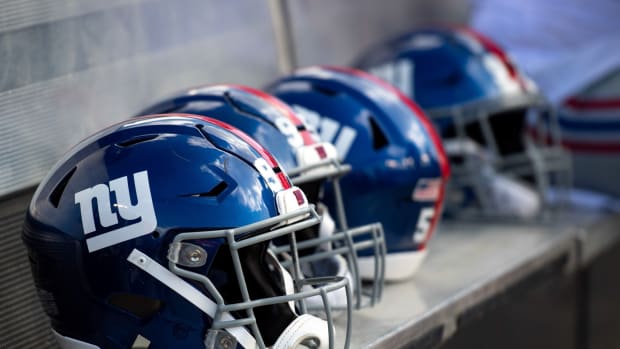
Grading New York Giants’ Most Significant Off-Season Moves
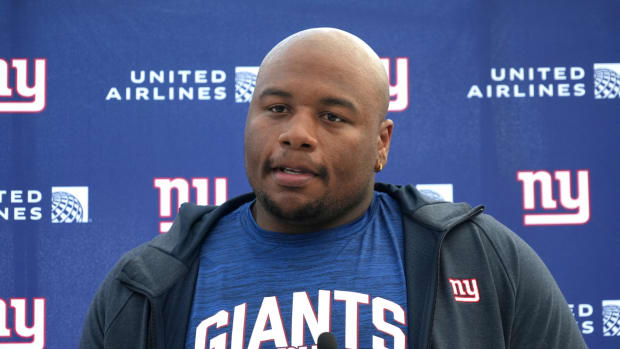
Giants Restructure IDL Dexter Lawrence's Contract
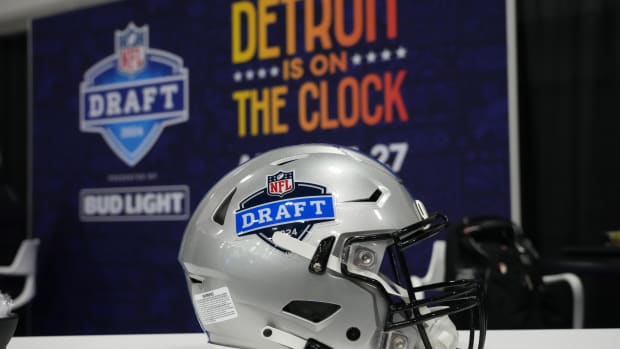
Arizona Cardinals "Open for Business" Regarding Fourth Overall Pick
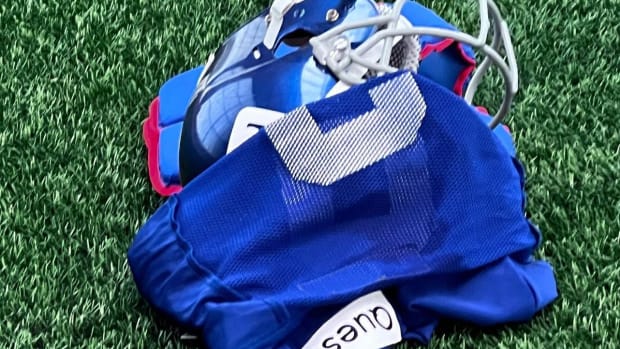
A Look Behind Some of the Giants New Jersey Number Assignments
Joint resolution relating to weekend adjournment on March 22, 2024
Sponsors: Sen. Philip Baruth
Last Recorded Action: 3/22/2024 - As adopted by Senate and House
Committee Activity - Meeting Full List
Regular session 2023-2024, no meeting history found for j.r.s.49 in the regular session 2023-2024..
View or print an assignment or item
Updated 11 May 2023
Important: Printed answers should NEVER be distributed to students or posted on the web. Printed answers are protected under copyright laws. Answers printed using the instructions below are for instructor convenience only.
This procedure prints all items in the assignment. Each item may or may not be identical to the latest published version available in the Item Library .

From the course menu: Select Assignments .
- Select an assignment title to open its Overview page.
- Choose Print View with Answers (top right).
- Select Print (top right) to print the entire assignment with all solutions, as well as hints and follow-up comments that students see.
This procedure prints the latest published version of the selected item in the Item Library .
- Open the item either from the Item Library or from an assignment .
To show any hidden parts in this view, select View all hidden parts . This option is available only to instructors and section instructors .

- Select the Print link (top right).
You can control whether students can print assignments from their Assignments list by using the security setting , Allow Students to Print .
- Homework category: By default, the Homework category allows students to print a homework assignment (with correct answers for the parts they have completed ).
- Quiz and Test categories: By default, these categories don't allow students to print a quiz or test assignment.

Copyright protection: The same copyright protection applies when students print assignments either before or after they complete them. These questions or answers should not be shared or distributed in any way. They are provided strictly for students' personal use to study later.
See also: Replace items in an assignment | Print assignment items (Student Help topic)
- Study Guides
- Homework Questions
Assignment 01-ENSC204-1
College of Liberal Arts
In Print: Agriculture in the Midwest
- CLA Marketing & Communications
- April 02, 2024

Publication Title
Agriculture in the Midwest, 1815-1900
R. Douglas Hurt
University of Nebraska Press
Publication Date
About the Book (from the publisher)
After the War of 1812 and the removal of the region’s Indigenous peoples, the American Midwest became a paradoxical land for settlers. Even as many settlers found that the region provided the bountiful life of their dreams, others found disappointment, even failure—and still others suffered social and racial prejudice.
In this broad and authoritative survey of midwestern agriculture from the War of 1812 to the turn of the twentieth century, R. Douglas Hurt contends that this region proved to be the country’s garden spot and the nation’s heart of agricultural production. During these eighty-five years the region transformed from a sparsely settled area to the home of large industrial and commercial cities, including Chicago, Milwaukee, Cleveland, and Detroit. Still, it remained primarily an agricultural region that promised a better life for many of the people who acquired land, raised crops and livestock, provided for their families, adopted new technologies, and sought political reform to benefit their economic interests. Focusing on the history of midwestern agriculture during wartime, utopian isolation, and colonization as well as political unrest, Hurt contextualizes myriad facets of the region’s past to show how agricultural life developed for midwestern farmers—and to reflect on what that meant for the region and nation.
About the Author
Professor Hurt received his Ph.D. from Kansas State University. He came to Purdue in 2003 as Department Head for the Department of History. Prior service included Director of the Graduate Program in Agricultural History and Rural Studies at Iowa State University for fourteen years and Associate Director for the State Historical Society of Missouri. He has served as editor for Agricultural History from 1994-2003 and editor for Ohio History from 2006-2009.
College News Home

IMAGES
VIDEO
COMMENTS
1 Answer. If you put the expression in parentheses the result will be printed: This works because the assignment operator <- returns the value (invisibly, which strangely is not in the documentation). Putting it in parentheses (or in print or c, or show, or cat (without newline)) makes it visible.
You can also print variables and objects and the print function will use the corresponding method to print each type of object. Note that you can also print an object or variable by typing it on the R console. # Print a variable x <- sqrt(18) print(x) # Note that this is equivalent to: # x [1] 4.242641. Nonetheless, the print function allows ...
On this page you'll learn how to apply the different assignment operators in the R programming language. The content of the article is structured as follows: 1) Example 1: Why You Should Use <- Instead of = in R. 2) Example 2: When <- is Really Different Compared to =. 3) Example 3: The Difference Between <- and <<-. 4) Video ...
How to Print Output in R. Last Updated On March 12, 2024 by Krunal Lathiya. Here are six primary methods used to print output in R: Using print () Using implicit printing. Using cat () Using message (), warning (), and stop () Using sprintf () to format string. Writing to a file.
We can do this by using the if statement. We first assign the variable x, and then write the if condition. In this case, assign -3 to x, and set the if condition to be true if x is smaller than 0 ( x < 0 ). If we run the example code, we indeed see that the string "x is a negative number" gets printed out.
an object used to select a method. further arguments passed to or from other methods. logical, indicating whether or not strings should be printed with surrounding quotes. integer, indicating how many levels should be printed for a factor; if 0, no extra "Levels" line will be printed.
You can also print variables inside the R terminal by simply typing the variable name. For example, # inside R terminal. x = "Welcome to Programiz!" # print value of x in console. x. // Output: [1] "Welcome to Programiz". You can use several functions such as print (), sprintf (), cat (), etc. to print output in R.
The <- symbol is the assignment operator. Assign some value to a variable then you can do either auto-printing for the variables just typing its name or you can use explicit printing. ... Output: msg [1] "hello R!!!" > print(msg) [1] "hello R!!!" Try it Yourself. Take Input From User: When we are working with R in an interactive session, we can ...
Note: In the R language, we can use the normal assignment operators, = and <-, like in any other programming language.However, the use of the = operator is limited to certain contexts.. How to print a variable. The R language is unique. It does not require the print() function to return an output. However, this does not mean that we cannot use the print() function in R.
The assign() function in R can be used to assign values to variables.. This function uses the following basic syntax: assign(x, value) where: x: A variable name, given as a character string.; value: The value(s) to be assigned to x.; The following examples show how to use this function in practice.
The <<-operator is used for assigning to variables in the parent environments (more like global assignments). The rightward assignments, although available, are rarely used. x <- 5 x x <- 9 x 10 -> x x. Output [1] 5 [1] 9 [1] 10. Check out these examples to learn more: Add Two Vectors; Take Input From User; R Multiplication Table
In this tutorial, I'll illustrate how to assign values to a variable name using the assign () function in R. Table of contents: 1) Definition & Basic R Syntax of assign Function. 2) Example 1: Using assign Function to Create New Vector. 3) Example 2: Using assign & paste0 Functions Dynamically in for-Loop. 4) Video, Further Resources & Summary.
Output. [1] "a" "b" "f". <environment: R_GlobalEnv>. In the above example, we can see that a, b and f are in the R_GlobalEnv environment. Notice that x (in the argument of the function) is not in this global environment. When we define a function, a new environment is created. Here, the function f() creates a new environment inside the global ...
Arguments are the actual values you put in. So add_up_numbers(a,b) has the parameters a and b, and add_up_numbers(a = 3, b = 5) has the arguments 3 and 5. The post Assignment in R: slings and arrows appeared first on Doodling in Data. To leave a comment for the author, please follow the link and comment on their blog: R - Doodling in Data.
In R there are various methods to print the output. Most common method to print output in R program, there is a function called print() is used. Also if the program of R is written over the console line by line then the output is printed normally, no need to use any function for print that output. To do this just select the output variable and press run button.
Open the assignment.; Select Print Assignment (bottom right) to open the print preview, which shows which items or parts of items will print. The assignment is reformatted so you can more easily scroll through it. If you haven't worked on the assignment yet: Any item Parts (A, B, etc.) not visible online until you complete the previous Part are also not visible in the print preview.
For coding, we recommend you improve your skills with Python, R, and/or Julia. The (evil) Deliverator uses (evil) Matlab. Graduate students are requested to use LATEX (or related TEX variant). If you are new to LATEX, please endeavor to submit at least n questions per assignment in LATEX, where n is the assignment number. Assignment submission:
According to the manual page here, . The operators <<-and ->> cause a search to made through the environment for an existing definition of the variable being assigned.. I've never had to do this in practice, but to my mind, assign wins a lot of points for specifying the environment exactly, without even having to think about R's scoping rules. The <<-performs a search through environments and ...
Bleacher Report's Kristopher Knox proposed this scenario involving the Kansas City Chiefs, who hold Pick No. 32 in the first round: Giants Get: 2024 Round 1 Pick (No. 32), 2024 Round 4 Pick (No ...
J.R.S.49 Joint resolution relating to weekend adjournment on March 22, 2024. Sponsors: Sen. Philip Baruth Last Recorded Action: 3/22/2024 - As adopted by Senate and House. Committee Activity - Meeting Full List Regular Session 2023-2024.
View or print an assignment or item. Updated 11 May 2023. Important: Printed answers should NEVER be distributed to students or posted on the web. Printed answers are protected under copyright laws. Answers printed using the instructions below are for instructor convenience only. Watch a video: See assignment as instructor or student and print ...
Assignment #1 - Due September 14 th, 3:30 PM ENSC 204 | Fall 2022 Engineering Graphics Page 1 of 4 Student Name: SFU ID#: Notes: • Print out this assignment then sketch your works on the allocated spaces. • The assignment has three parts (10 points), part 1 (3 points) has been delivered in the class. • Part two should be delivered BEFORE the start of the session on September 14 th.
How can I write into a function a way to detect if the output is being assigned (<-) to something?The reasoning is I'd like to print a message if it is not being assigned and just goes to the console but if it is being assigned I'd like it not to print the message.
Dr. R. Douglas Hurt, professor of history, and his new book, "Agriculture in the Midwest, 1815-1900." University of Nebraska Press. July 2023. After the War of 1812 and the removal of the region's Indigenous peoples, the American Midwest became a paradoxical land for settlers. Even as many settlers found that the region provided the bountiful ...
Prior to tonight's game against the Cincinnati Reds, the Phillies selected the contract of right-handed pitcher Ricardo Pinto from triple-A Lehigh Valley. To make room for him on the 26-man roster, right-handed pitcher Connor Brogdon was designated for assignment. Pinto will wear No. 51. Phillies President of Baseball Operations David.
A custom print() method will only run when an object is actually print()ed.When you type a value at the console, the final expression is implicitly print()ed.That printing does not happen when you do an assignment. So unless you explicitly call print() or wrap the assignment in (), nothing triggers the print() function. If you need it to print no matter what, you might consider adding code to ...