

1.7 Java | Assignment Statements & Expressions
An assignment statement designates a value for a variable. An assignment statement can be used as an expression in Java.
After a variable is declared, you can assign a value to it by using an assignment statement . In Java, the equal sign = is used as the assignment operator . The syntax for assignment statements is as follows:
An expression represents a computation involving values, variables, and operators that, when taking them together, evaluates to a value. For example, consider the following code:
You can use a variable in an expression. A variable can also be used on both sides of the = operator. For example:
In the above assignment statement, the result of x + 1 is assigned to the variable x . Let’s say that x is 1 before the statement is executed, and so becomes 2 after the statement execution.
To assign a value to a variable, you must place the variable name to the left of the assignment operator. Thus the following statement is wrong:
Note that the math equation x = 2 * x + 1 ≠ the Java expression x = 2 * x + 1
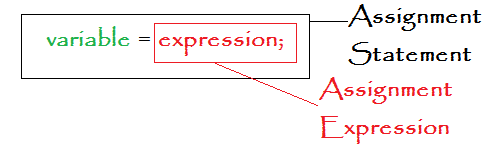
Which is equivalent to:
And this statement
is equivalent to:
Note: The data type of a variable on the left must be compatible with the data type of a value on the right. For example, int x = 1.0 would be illegal, because the data type of x is int (integer) and does not accept the double value 1.0 without Type Casting .
◄◄◄BACK | NEXT►►►
What's Your Opinion? Cancel reply
Enhance your Brain
Subscribe to Receive Free Bio Hacking, Nootropic, and Health Information
HTML for Simple Website Customization My Personal Web Customization Personal Insights
DISCLAIMER | Sitemap | ◘
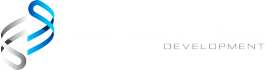
HTML for Simple Website Customization My Personal Web Customization Personal Insights SEO Checklist Publishing Checklist My Tools
Top Posts & Pages

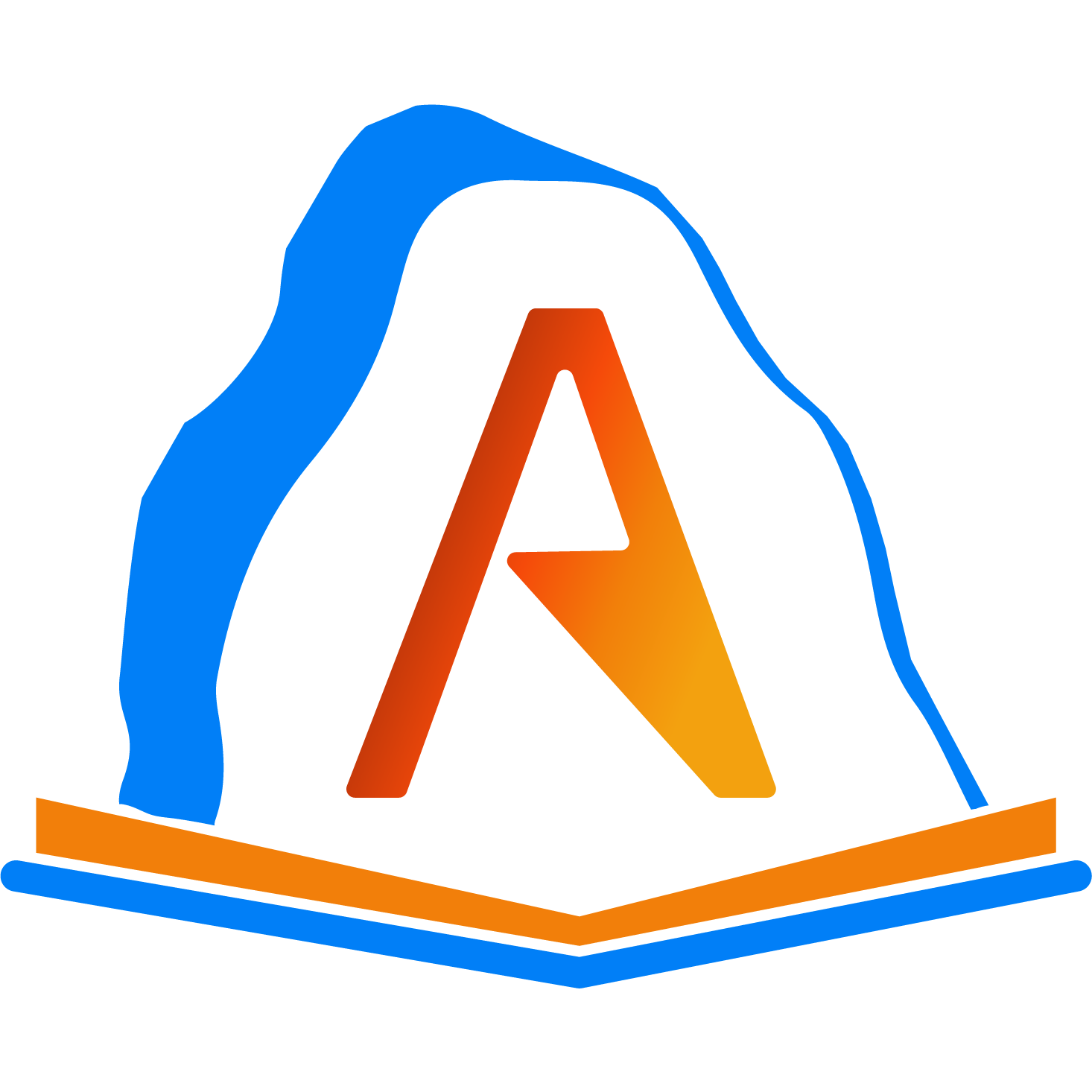
- Table of Contents
- Course Home
- Assignments
- Peer Instruction (Instructor)
- Peer Instruction (Student)
- Change Course
- Instructor's Page
- Progress Page
- Edit Profile
- Change Password
- Scratch ActiveCode
- Scratch Activecode
- Instructors Guide
- About Runestone
- Report A Problem
- 1.1 Preface
- 1.2 Why Programming? Why Java?
- 1.3 Variables and Data Types
- 1.4 Expressions and Assignment Statements
- 1.5 Compound Assignment Operators
- 1.6 Casting and Ranges of Variables
- 1.7 Java Development Environments (optional)
- 1.8 Unit 1 Summary
- 1.9 Unit 1 Mixed Up Code Practice
- 1.10 Unit 1 Coding Practice
- 1.11 Multiple Choice Exercises
- 1.12 Lesson Workspace
- 1.3. Variables and Data Types" data-toggle="tooltip">
- 1.5. Compound Assignment Operators' data-toggle="tooltip" >
Before you keep reading...
Runestone Academy can only continue if we get support from individuals like you. As a student you are well aware of the high cost of textbooks. Our mission is to provide great books to you for free, but we ask that you consider a $10 donation, more if you can or less if $10 is a burden.
Making great stuff takes time and $$. If you appreciate the book you are reading now and want to keep quality materials free for other students please consider a donation to Runestone Academy. We ask that you consider a $10 donation, but if you can give more thats great, if $10 is too much for your budget we would be happy with whatever you can afford as a show of support.
1.4. Expressions and Assignment Statements ¶
In this lesson, you will learn about assignment statements and expressions that contain math operators and variables.
1.4.1. Assignment Statements ¶
Remember that a variable holds a value that can change or vary. Assignment statements initialize or change the value stored in a variable using the assignment operator = . An assignment statement always has a single variable on the left hand side of the = sign. The value of the expression on the right hand side of the = sign (which can contain math operators and other variables) is copied into the memory location of the variable on the left hand side.
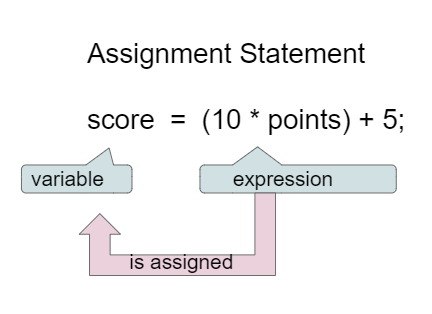
Figure 1: Assignment Statement (variable = expression) ¶
Instead of saying equals for the = operator in an assignment statement, say “gets” or “is assigned” to remember that the variable on the left hand side gets or is assigned the value on the right. In the figure above, score is assigned the value of 10 times points (which is another variable) plus 5.
The following video by Dr. Colleen Lewis shows how variables can change values in memory using assignment statements.
As we saw in the video, we can set one variable to a copy of the value of another variable like y = x;. This won’t change the value of the variable that you are copying from.

Click on the Show CodeLens button to step through the code and see how the values of the variables change.
The program is supposed to figure out the total money value given the number of dimes, quarters and nickels. There is an error in the calculation of the total. Fix the error to compute the correct amount.
Calculate and print the total pay given the weekly salary and the number of weeks worked. Use string concatenation with the totalPay variable to produce the output Total Pay = $3000 . Don’t hardcode the number 3000 in your print statement.

Assume you have a package with a given height 3 inches and width 5 inches. If the package is rotated 90 degrees, you should swap the values for the height and width. The code below makes an attempt to swap the values stored in two variables h and w, which represent height and width. Variable h should end up with w’s initial value of 5 and w should get h’s initial value of 3. Unfortunately this code has an error and does not work. Use the CodeLens to step through the code to understand why it fails to swap the values in h and w.
1-4-7: Explain in your own words why the ErrorSwap program code does not swap the values stored in h and w.
Swapping two variables requires a third variable. Before assigning h = w , you need to store the original value of h in the temporary variable. In the mixed up programs below, drag the blocks to the right to put them in the right order.
The following has the correct code that uses a third variable named “temp” to swap the values in h and w.
The code is mixed up and contains one extra block which is not needed in a correct solution. Drag the needed blocks from the left into the correct order on the right, then check your solution. You will be told if any of the blocks are in the wrong order or if you need to remove one or more blocks.
After three incorrect attempts you will be able to use the Help Me button to make the problem easier.
Fix the code below to perform a correct swap of h and w. You need to add a new variable named temp to use for the swap.
1.4.2. Incrementing the value of a variable ¶
If you use a variable to keep score you would probably increment it (add one to the current value) whenever score should go up. You can do this by setting the variable to the current value of the variable plus one (score = score + 1) as shown below. The formula looks a little crazy in math class, but it makes sense in coding because the variable on the left is set to the value of the arithmetic expression on the right. So, the score variable is set to the previous value of score + 1.
Click on the Show CodeLens button to step through the code and see how the score value changes.
1-4-11: What is the value of b after the following code executes?
- It sets the value for the variable on the left to the value from evaluating the right side. What is 5 * 2?
- Correct. 5 * 2 is 10.
1-4-12: What are the values of x, y, and z after the following code executes?
- x = 0, y = 1, z = 2
- These are the initial values in the variable, but the values are changed.
- x = 1, y = 2, z = 3
- x changes to y's initial value, y's value is doubled, and z is set to 3
- x = 2, y = 2, z = 3
- Remember that the equal sign doesn't mean that the two sides are equal. It sets the value for the variable on the left to the value from evaluating the right side.
- x = 1, y = 0, z = 3
1.4.3. Operators ¶
Java uses the standard mathematical operators for addition ( + ), subtraction ( - ), multiplication ( * ), and division ( / ). Arithmetic expressions can be of type int or double. An arithmetic operation that uses two int values will evaluate to an int value. An arithmetic operation that uses at least one double value will evaluate to a double value. (You may have noticed that + was also used to put text together in the input program above – more on this when we talk about strings.)
Java uses the operator == to test if the value on the left is equal to the value on the right and != to test if two items are not equal. Don’t get one equal sign = confused with two equal signs == ! They mean different things in Java. One equal sign is used to assign a value to a variable. Two equal signs are used to test a variable to see if it is a certain value and that returns true or false as you’ll see below. Use == and != only with int values and not doubles because double values are an approximation and 3.3333 will not equal 3.3334 even though they are very close.
Run the code below to see all the operators in action. Do all of those operators do what you expected? What about 2 / 3 ? Isn’t surprising that it prints 0 ? See the note below.
When Java sees you doing integer division (or any operation with integers) it assumes you want an integer result so it throws away anything after the decimal point in the answer, essentially rounding down the answer to a whole number. If you need a double answer, you should make at least one of the values in the expression a double like 2.0.
With division, another thing to watch out for is dividing by 0. An attempt to divide an integer by zero will result in an ArithmeticException error message. Try it in one of the active code windows above.
Operators can be used to create compound expressions with more than one operator. You can either use a literal value which is a fixed value like 2, or variables in them. When compound expressions are evaluated, operator precedence rules are used, so that *, /, and % are done before + and -. However, anything in parentheses is done first. It doesn’t hurt to put in extra parentheses if you are unsure as to what will be done first.
In the example below, try to guess what it will print out and then run it to see if you are right. Remember to consider operator precedence .
1-4-15: Consider the following code segment. Be careful about integer division.
What is printed when the code segment is executed?
- 0.666666666666667
- Don't forget that division and multiplication will be done first due to operator precedence.
- Yes, this is equivalent to (5 + ((a/b)*c) - 1).
- Don't forget that division and multiplication will be done first due to operator precedence, and that an int/int gives an int result where it is rounded down to the nearest int.
1-4-16: Consider the following code segment.
What is the value of the expression?
- Dividing an integer by an integer results in an integer
- Correct. Dividing an integer by an integer results in an integer
- The value 5.5 will be rounded down to 5
1-4-17: Consider the following code segment.
- Correct. Dividing a double by an integer results in a double
- Dividing a double by an integer results in a double
1-4-18: Consider the following code segment.
- Correct. Dividing an integer by an double results in a double
- Dividing an integer by an double results in a double
1.4.4. The Modulo Operator ¶
The percent sign operator ( % ) is the mod (modulo) or remainder operator. The mod operator ( x % y ) returns the remainder after you divide x (first number) by y (second number) so 5 % 2 will return 1 since 2 goes into 5 two times with a remainder of 1. Remember long division when you had to specify how many times one number went into another evenly and the remainder? That remainder is what is returned by the modulo operator.
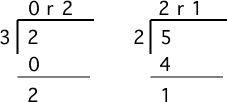
Figure 2: Long division showing the whole number result and the remainder ¶
In the example below, try to guess what it will print out and then run it to see if you are right.
The result of x % y when x is smaller than y is always x . The value y can’t go into x at all (goes in 0 times), since x is smaller than y , so the result is just x . So if you see 2 % 3 the result is 2 .
1-4-21: What is the result of 158 % 10?
- This would be the result of 158 divided by 10. modulo gives you the remainder.
- modulo gives you the remainder after the division.
- When you divide 158 by 10 you get a remainder of 8.
1-4-22: What is the result of 3 % 8?
- 8 goes into 3 no times so the remainder is 3. The remainder of a smaller number divided by a larger number is always the smaller number!
- This would be the remainder if the question was 8 % 3 but here we are asking for the reminder after we divide 3 by 8.
- What is the remainder after you divide 3 by 8?
1.4.5. FlowCharting ¶
Assume you have 16 pieces of pizza and 5 people. If everyone gets the same number of slices, how many slices does each person get? Are there any leftover pieces?
In industry, a flowchart is used to describe a process through symbols and text. A flowchart usually does not show variable declarations, but it can show assignment statements (drawn as rectangle) and output statements (drawn as rhomboid).
The flowchart in figure 3 shows a process to compute the fair distribution of pizza slices among a number of people. The process relies on integer division to determine slices per person, and the mod operator to determine remaining slices.
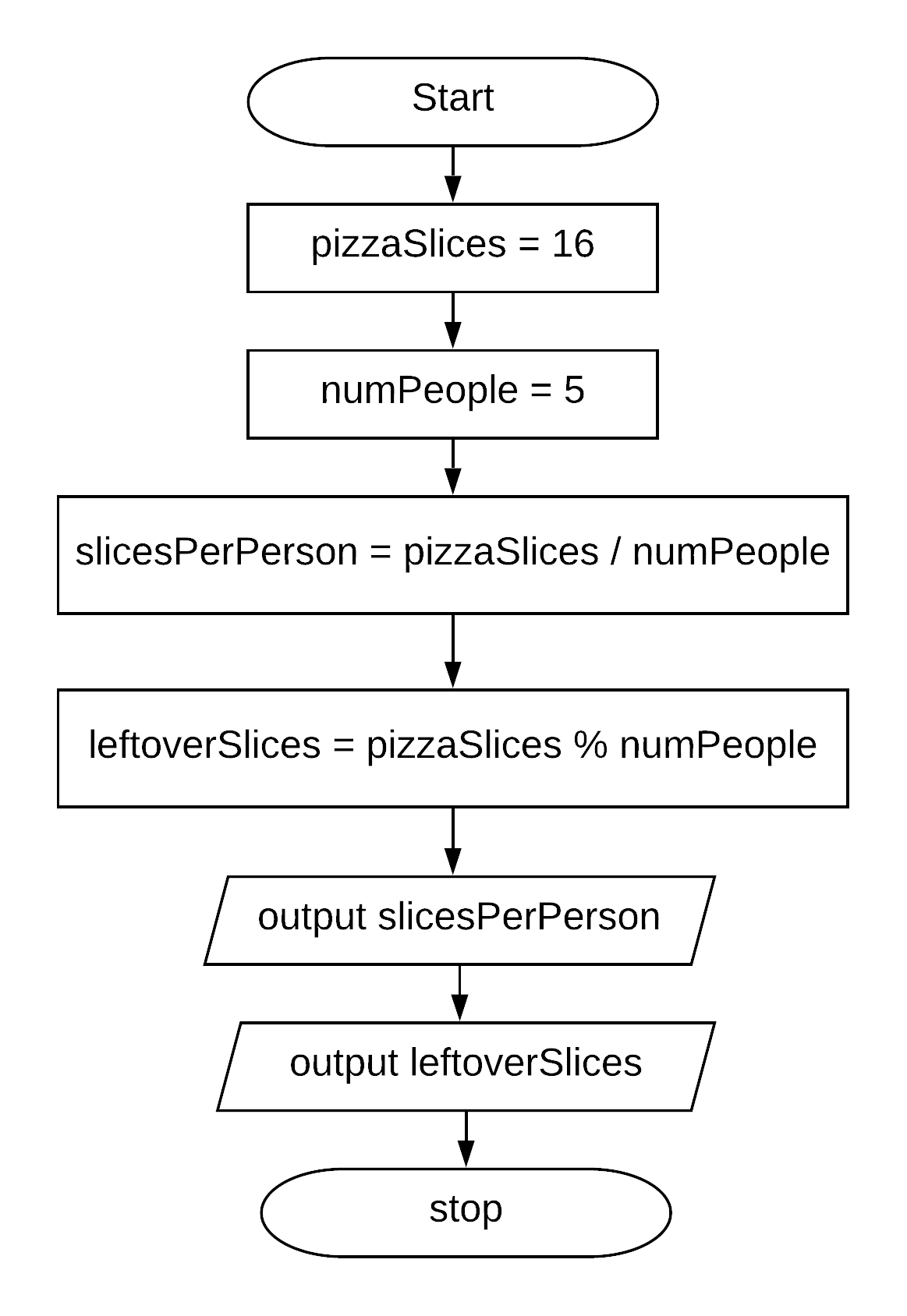
Figure 3: Example Flow Chart ¶
A flowchart shows pseudo-code, which is like Java but not exactly the same. Syntactic details like semi-colons are omitted, and input and output is described in abstract terms.
Complete the program based on the process shown in the Figure 3 flowchart. Note the first line of code declares all 4 variables as type int. Add assignment statements and print statements to compute and print the slices per person and leftover slices. Use System.out.println for output.
1.4.6. Storing User Input in Variables ¶
Variables are a powerful abstraction in programming because the same algorithm can be used with different input values saved in variables.

Figure 4: Program input and output ¶
A Java program can ask the user to type in one or more values. The Java class Scanner is used to read from the keyboard input stream, which is referenced by System.in . Normally the keyboard input is typed into a console window, but since this is running in a browser you will type in a small textbox window displayed below the code. The code below shows an example of prompting the user to enter a name and then printing a greeting. The code String name = scan.nextLine() gets the string value you enter as program input and then stores the value in a variable.
Run the program a few times, typing in a different name. The code works for any name: behold, the power of variables!
Run this program to read in a name from the input stream. You can type a different name in the input window shown below the code.
Try stepping through the code with the CodeLens tool to see how the name variable is assigned to the value read by the scanner. You will have to click “Hide CodeLens” and then “Show in CodeLens” to enter a different name for input.
The Scanner class has several useful methods for reading user input. A token is a sequence of characters separated by white space.
Run this program to read in an integer from the input stream. You can type a different integer value in the input window shown below the code.
A rhomboid (slanted rectangle) is used in a flowchart to depict data flowing into and out of a program. The previous flowchart in Figure 3 used a rhomboid to indicate program output. A rhomboid is also used to denote reading a value from the input stream.
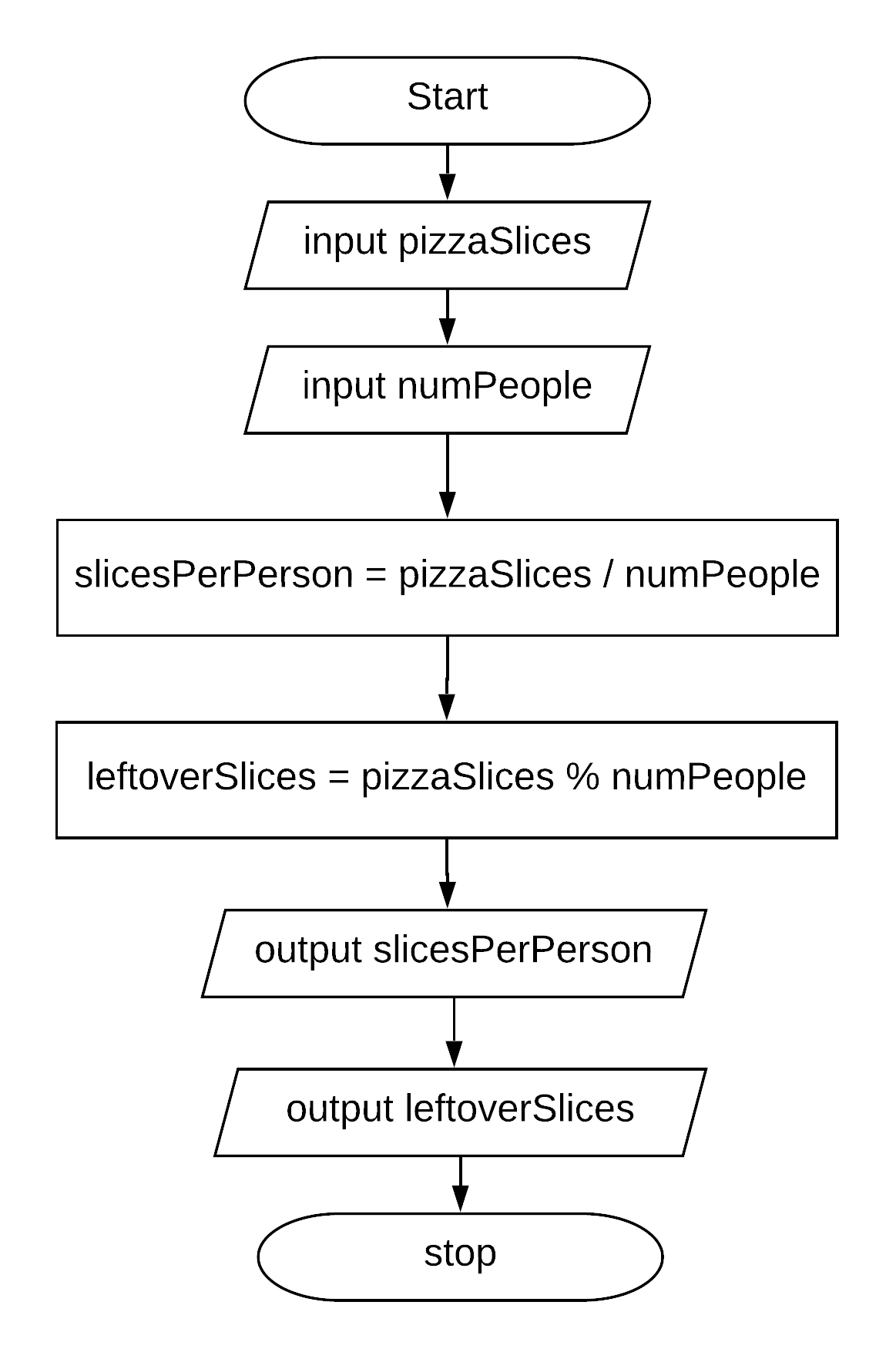
Figure 5: Flow Chart Reading User Input ¶
Figure 5 contains an updated version of the pizza calculator process. The first two steps have been altered to initialize the pizzaSlices and numPeople variables by reading two values from the input stream. In Java this will be done using a Scanner object and reading from System.in.
Complete the program based on the process shown in the Figure 5 flowchart. The program should scan two integer values to initialize pizzaSlices and numPeople. Run the program a few times to experiment with different values for input. What happens if you enter 0 for the number of people? The program will bomb due to division by zero! We will see how to prevent this in a later lesson.
The program below reads two integer values from the input stream and attempts to print the sum. Unfortunately there is a problem with the last line of code that prints the sum.
Run the program and look at the result. When the input is 5 and 7 , the output is Sum is 57 . Both of the + operators in the print statement are performing string concatenation. While the first + operator should perform string concatenation, the second + operator should perform addition. You can force the second + operator to perform addition by putting the arithmetic expression in parentheses ( num1 + num2 ) .
More information on using the Scanner class can be found here https://www.w3schools.com/java/java_user_input.asp
1.4.7. Programming Challenge : Dog Years ¶
In this programming challenge, you will calculate your age, and your pet’s age from your birthdates, and your pet’s age in dog years. In the code below, type in the current year, the year you were born, the year your dog or cat was born (if you don’t have one, make one up!) in the variables below. Then write formulas in assignment statements to calculate how old you are, how old your dog or cat is, and how old they are in dog years which is 7 times a human year. Finally, print it all out.
Calculate your age and your pet’s age from the birthdates, and then your pet’s age in dog years. If you want an extra challenge, try reading the values using a Scanner.
1.4.8. Summary ¶
Arithmetic expressions include expressions of type int and double.
The arithmetic operators consist of +, -, * , /, and % (modulo for the remainder in division).
An arithmetic operation that uses two int values will evaluate to an int value. With integer division, any decimal part in the result will be thrown away, essentially rounding down the answer to a whole number.
An arithmetic operation that uses at least one double value will evaluate to a double value.
Operators can be used to construct compound expressions.
During evaluation, operands are associated with operators according to operator precedence to determine how they are grouped. (*, /, % have precedence over + and -, unless parentheses are used to group those.)
An attempt to divide an integer by zero will result in an ArithmeticException to occur.
The assignment operator (=) allows a program to initialize or change the value stored in a variable. The value of the expression on the right is stored in the variable on the left.
During execution, expressions are evaluated to produce a single value.
The value of an expression has a type based on the evaluation of the expression.
CS101: Introduction to Computer Science I
Variables and Assignment Statements
Read this chapter, which covers variables and arithmetic operations and order precedence in Java.
9. Assignment Statements
No. The incorrect splittings are highlighted in red:
Assignment Statement
So far, the example programs have been using the value initially put into a variable. Programs can change the value in a variable. An assignment statement changes the value that is held in a variable. The program uses an assignment statement.
The assignment statement puts the value 123 into the variable. In other words, while the program is executing there will be a 64 bit section of memory that holds the value 123.
Remember that the word "execute" is often used to mean "run". You speak of "executing a program" or "executing" a line of the program.
Question 10:
Vertex Academy
How to assign a value to a variable in java.

The assigning of a value to a variable is carried out with the help of the "=" symbol. Consider the following examples:
Example No.1
Let’s say we want to assign the value of "10" to the variable "k" of the "int" type. It’s very easy and can be done in two ways:
If you try to run this code on your computer, you will see the following:
In this example, we first declared the variable to be "k" of the "int" type:
Then in another line we assigned the value "10" to the variable "k":
As you you may have understood from the example, the "=" symbol is an assignment operation. It always works from right to left:

This assigns the value "10" to the variable "k."
As you can see, in this example we declared the variable as "k" of the int type and assigned the value to it in a single line:
int k = 10;
So, now you know that:
- The "=" symbol is responsible for assignment operation and we assign values to variables with the help of this symbol.
- There are two ways to assign a value to variables: in one line or in two lines.
What is variable initialization?
Actually, you already know what it is. Initialization is the assignment of an initial value to a variable. In other words, if you just created a variable and didn’t assign any value to it, then this variable is uninitialized. So, if you ever hear:
- “We need to initialize the variable,” it simply means that “we need to assign the initial value to the variable.”
- “The variable has been initialized,” it simply means that “we have assigned the initial value to the variable.”
Here's another example of variable initialization:
Example No.2
15 100 100000000 I love Java M 145.34567 3.14 true
In this line, we declared variable number1 of the byte type and, with the help of the "=" symbol, assigned the value 15 to it.
In this line, we declared variable number2 of the short type and, with the help of the "=" symbol, assigned the value 100 to it.
In this line, we declared variable number3 of the long type and, with the help of the "=" symbol, assigned the value 100000000 to it.
In this line, we declared the variable title of the string type and, with the help of the "=" symbol, assigned the value “I love Java” to it. Since this variable belongs to the string type, we wrote the value of the variable in double quotes .
In this line, we declared the variable letter of the char type and, with the help of the "=" symbol, assigned the value “M” to it. Note that since the variable belongs to the char type, we wrote the value of the variable in single quotes .
In this line, we declared the variable sum of the double type and, with the help of the "=" symbol, assigned the value "145.34567" to it.
In this line, we declared the variable pi of the float type and, with the help of the "=" symbol, assigned the value “3.14f” to it. Note that we added f to the number 3.14. This is a small detail that you'll need to remember: it's necessary to add f to the values of float variables. However, when you see it in the console, it will show up as just 3.14 (without the "f").
In this line, we declared the variable result of the boolean type and, with the help of the "=" symbol, assigned the value "true" to it.
Then we display the values of all the variables in the console with the help of
LET’S SUMMARIZE:
- We assign a value to a variable with the help of the assignment operator "=." It always works from right to left.

2. There are two ways to assign a value to a variable:
- in two lines
- or in one line
- You also need to remember:
If we assign a value to a variable of the string type, we need to put it in double quotes :
If we assign a value to a variable of the char type, we need to put it in single quotes :
If we assign a value to a variable of the float type, we need to add the letter "f" :
- "Initialization" means “to assign an initial value to a variable.”

- ← How do you add comments to your code?
- Operators in Java →
You May Also Like
Java - variable types. how to create a variable in java, why is java so popular, how do you add comments to your code.
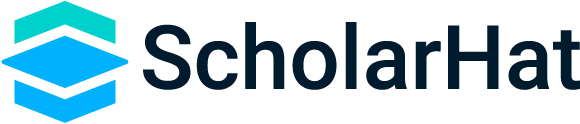
01 Career Opportunities
- Top 50 Java Interview Questions and Answers
- Java Developer Salary Guide in India – For Freshers & Experienced
02 Beginner
- Best Java Developer Roadmap 2024
- Hierarchical Inheritance in Java
- Arithmetic operators in Java
- Unary operator in Java
- Relational operators in Java
Assignment operator in Java
- Logical operators in Java
- Single Inheritance in Java
- Primitive Data Types in Java
- Multiple Inheritance in Java
- Hybrid Inheritance in Java
- Parameterized Constructor in Java
- Constructor Chaining in Java
- What are Copy Constructors In Java? Explore Types,Examples & Use
- What is a Bitwise Operator in Java? Type, Example and More
- Constructor Overloading in Java
- Ternary Operator in Java
- For Loop in Java: Its Types and Examples
- Top 10 Reasons to know why Java is Important?
- What is Java? A Beginners Guide to Java
- Differences between JDK, JRE, and JVM: Java Toolkit
- Variables in Java: Local, Instance and Static Variables
- Data Types in Java - Primitive and Non-Primitive Data Types
- Conditional Statements in Java: If, If-Else and Switch Statement
- What are Operators in Java - Types of Operators in Java ( With Examples )
- Looping Statements in Java - For, While, Do-While Loop in Java
- Java VS Python
- Jump Statements in JAVA - Types of Statements in JAVA (With Examples)
- Java Arrays: Single Dimensional and Multi-Dimensional Arrays
- What is String in Java - Java String Types and Methods (With Examples)
03 Intermediate
- OOPs Concepts in Java: Encapsulation, Abstraction, Inheritance, Polymorphism
- What is Class in Java? - Objects and Classes in Java {Explained}
- Access Modifiers in Java: Default, Private, Public, Protected
- Constructors in Java: Types of Constructors with Examples
- Polymorphism in Java: Compile time and Runtime Polymorphism
- Abstract Class in Java: Concepts, Examples, and Usage
- What is Inheritance in Java: Types of Inheritance in Java
- Exception handling in Java: Try, Catch, Finally, Throw and Throws
04 Training Programs
- Java Programming Course
- C++ Programming Course
- MERN: Full-Stack Web Developer Certification Training
- Data Structures and Algorithms Training
- Assignment Operator In Ja..
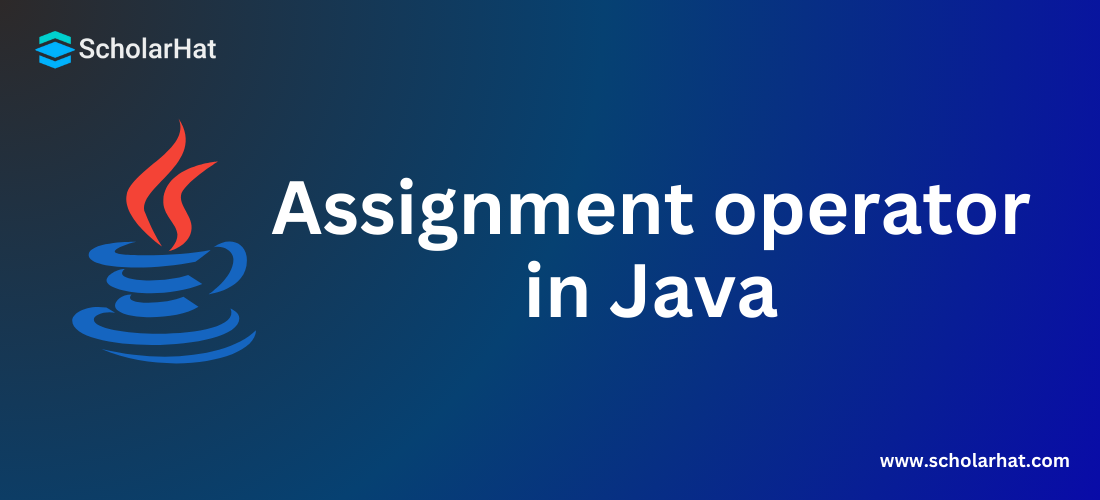
Java Programming For Beginners Free Course
Assignment operators in java: an overview.
We already discussed the Types of Operators in the previous tutorial Java. In this Java tutorial , we will delve into the different types of assignment operators in Java, and their syntax, and provide examples for better understanding. Because Java is a flexible and widely used programming language. Assignment operators play a crucial role in manipulating and assigning values to variables. To further enhance your understanding and application of Java assignment operator's concepts, consider enrolling in the best Java Certification Course .
What are the Assignment Operators in Java?
Assignment operators in Java are used to assign values to variables . They are classified into two main types: simple assignment operator and compound assignment operator.
The general syntax for a simple assignment statement is:
And for a compound assignment statement:
Read More - Advanced Java Interview Questions
Types of Assignment Operators in Java
- Simple Assignment Operator: The Simple Assignment Operator is used with the "=" sign, where the operand is on the left side and the value is on the right. The right-side value must be of the same data type as that defined on the left side.
- Compound Assignment Operator: Compound assignment operators combine arithmetic operations with assignments. They provide a concise way to perform an operation and assign the result to the variable in one step. The Compound Operator is utilized when +,-,*, and / are used in conjunction with the = operator.
1. Simple Assignment Operator (=):
The equal sign (=) is the basic assignment operator in Java. It is used to assign the value on the right-hand side to the variable on the left-hand side.
Explanation
2. addition assignment operator (+=) :, 3. subtraction operator (-=):, 4. multiplication operator (*=):.
Read More - Java Developer Salary
5. Division Operator (/=):
6. modulus assignment operator (%=):, example of assignment operator in java.
Let's look at a few examples in our Java Playground to illustrate the usage of assignment operators in Java:
- Unary Operator in Java
- Arithmetic Operators in Java
- Relational Operators in Java
- Logical Operators in Java
Q1. Can I use multiple assignment operators in a single statement?
Q2. are there any other compound assignment operators in java, q3. how many types of assignment operators.
- 1. (=) operator
- 1. (+=) operator
- 2. (-=) operator
- 3. (*=) operator
- 4. (/=) operator
- 5. (%=) operator
About Author
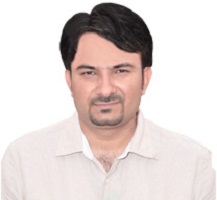
We use cookies to make interactions with our websites and services easy and meaningful. Please read our Privacy Policy for more details.
- Watch & Listen
- Oracle University
Previous in the Series: Summary of Operators
Next in the Series: Control Flow Statements
Expressions, Statements and Blocks
Expressions.
An expression is a construct made up of variables, operators, and method invocations, which are constructed according to the syntax of the language, that evaluates to a single value. You have already seen examples of expressions, illustrated in code below:
The data type of the value returned by an expression depends on the elements used in the expression. The expression cadence = 0 returns an int because the assignment operator returns a value of the same data type as its left-hand operand; in this case, cadence is an int . As you can see from the other expressions, an expression can return other types of values as well, such as boolean or String .
The Java programming language allows you to construct compound expressions from various smaller expressions as long as the data type required by one part of the expression matches the data type of the other. Here is an example of a compound expression:
In this particular example, the order in which the expression is evaluated is unimportant because the result of multiplication is independent of order; the outcome is always the same, no matter in which order you apply the multiplications. However, this is not true of all expressions. For example, the following expression gives different results, depending on whether you perform the addition or the division operation first:
You can specify exactly how an expression will be evaluated using balanced parenthesis: ( and ) . For example, to make the previous expression unambiguous, you could write the following:
If you don't explicitly indicate the order for the operations to be performed, the order is determined by the precedence assigned to the operators in use within the expression. Operators that have a higher precedence get evaluated first. For example, the division operator has a higher precedence than does the addition operator. Therefore, the following two statements are equivalent:
When writing compound expressions, be explicit and indicate with parentheses which operators should be evaluated first. This practice makes code easier to read and to maintain.
Floating Point Arithmetic
Floating point arithmetic is a special world in which common operations may behave unexpectedly. Consider the following code.
You would probably expect that it prints true . Due to the way floating point addition is conducted and rounded, it prints false .
Presenting how floating point arithmetic is implemented in Java is beyond the scope of this tutorial. If you need to learn more on this topic, you may watch the following vide.
Statements are roughly equivalent to sentences in natural languages. A statement forms a complete unit of execution. The following types of expressions can be made into a statement by terminating the expression with a semicolon ( ; ).
- Assignment expressions
- Any use of ++ or --
- Method invocations
- Object creation expressions
- Such statements are called expression statements. Here are some examples of expression statements.
In addition to expression statements, there are two other kinds of statements: declaration statements and control flow statements. A declaration statement declares a variable. You have seen many examples of declaration statements already:
Finally, control flow statements regulate the order in which statements get executed. You will learn about control flow statements in the next section, Control Flow Statements.
A block is a group of zero or more statements between balanced braces and can be used anywhere a single statement is allowed. The following example, BlockDemo , illustrates the use of blocks:
In this tutorial
Last update: September 22, 2021
Java Tutorial
Java methods, java classes, java file handling, java how to, java reference, java examples, java variables.
Variables are containers for storing data values.
In Java, there are different types of variables, for example:
- String - stores text, such as "Hello". String values are surrounded by double quotes
- int - stores integers (whole numbers), without decimals, such as 123 or -123
- float - stores floating point numbers, with decimals, such as 19.99 or -19.99
- char - stores single characters, such as 'a' or 'B'. Char values are surrounded by single quotes
- boolean - stores values with two states: true or false
Declaring (Creating) Variables
To create a variable, you must specify the type and assign it a value:
Where type is one of Java's types (such as int or String ), and variableName is the name of the variable (such as x or name ). The equal sign is used to assign values to the variable.
To create a variable that should store text, look at the following example:
Create a variable called name of type String and assign it the value " John ":
Try it Yourself »
To create a variable that should store a number, look at the following example:
Create a variable called myNum of type int and assign it the value 15 :
You can also declare a variable without assigning the value, and assign the value later:
Note that if you assign a new value to an existing variable, it will overwrite the previous value:
Change the value of myNum from 15 to 20 :

Final Variables
If you don't want others (or yourself) to overwrite existing values, use the final keyword (this will declare the variable as "final" or "constant", which means unchangeable and read-only):
Other Types
A demonstration of how to declare variables of other types:
You will learn more about data types in the next section.
Test Yourself With Exercises
Create a variable named carName and assign the value Volvo to it.
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
What Is an Assignment Statement in Java?
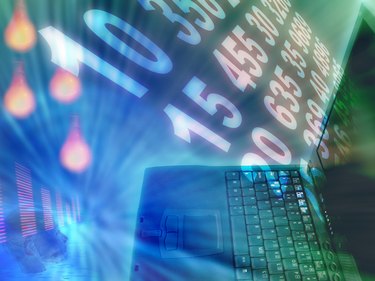
Java programs store data values in variables. When a programmer creates a variable in a Java application, he declares the type and name of the variable, then assigns a value to it. The value of a variable can be altered at subsequent points in execution using further assignment operations. The assignment statement in Java involves using the assignment operator to set the value of a variable. The exact syntax depends on the type of variable receiving a value.
Advertisement
Video of the Day
In Java, variables are strongly typed. This means that when you declare a variable in a Java program, you must declare its type, followed by its name. The following sample Java code demonstrates declaring two variables, one of primitive-type integer and one of an object type for a class within the application: int num; ApplicationHelper myHelp;
Once a program contains a variable declaration, the kind of value assigned to the variable must be suited to the type declared. These variable declarations could be followed by assignment statements on subsequent lines. However, the assignment operation could also take place on the same line as the declaration.
Assignment in Java is the process of giving a value to a primitive-type variable or giving an object reference to an object-type variable. The equals sign acts as assignment operator in Java, followed by the value to assign. The following sample Java code demonstrates assigning a value to a primitive-type integer variable, which has already been declared: num = 5;
The assignment operation could alternatively appear within the same line of code as the declaration of the variable, as follows: int num = 5;
The value of the variable can be altered again in subsequent processing as in this example: num++;
This code increments the variable value, adding a value of one to it.
Instantiation
When the assignment statement appears with object references, the assignment operation may also involve object instantiation. When Java code creates a new object instance of a Java class in an application, the "new" keyword causes the constructor method of the class to execute, instantiating the object. The following sample code demonstrates instantiating an object variable: myHelp = new ApplicationHelper();
This could also appear within the same line as the variable declaration as follows: ApplicationHelper myHelp = new ApplicationHelper();
When this line of code executes, the class constructor method executes, returning an instance of the class, a reference to which is stored by the variable.
Referencing
Once a variable has been declared and assigned a value, a Java program can refer to the variable in subsequent processing. For primitive-type variables, the variable name refers to a stored value. For object types, the variable refers to the location of the object instance in memory. This means that two object variables can point to the same instance, as in the following sample code: ApplicationHelper myHelp = new ApplicationHelper(); ApplicationHelper sameHelp = myHelp;
This syntax appears commonly when programs pass object references as parameters to class methods.
- Oracle: The Java Tutorials - Variables
- Oracle: The Java Tutorials - Assignment, Arithmetic, and Unary Operators
- Oracle: The Java Tutorials - Primitive Data Types
- Oracle: The Java Tutorials - Creating Objects
- Oracle: The Java Tutorials - What Is an Object?
- Oracle: The Java Tutorials - Summary of Variables
- Java Language Specification; Types, Values, and Variables; 2000
- Oracle: The Java Tutorials - Understanding Instance and Class Members
Report an Issue
Screenshot loading...

Java Tutorial
Control statements, java object class, java inheritance, java polymorphism, java abstraction, java encapsulation, java oops misc.
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

Java Tutorials
Java assignment statement.
In this tutorial, we will learn about Java Assignment Statement. We can assign or give value to a variable using the assignment statement.
The general syntax for assigning a value to a variable is as follows:
variable_name = value;
We can also assign value to a variable as part of the declaration. This is known as variable initialization. The syntax for the variable initialization is:
Datatype variable_name = value;
To assign a value of 72 to a variable named length :
// assign a value to the variable
length = 72;
For example, to initialize a variable width to 6.2:
//declare and initialize the variable
float width = 6.2;
Java Tutorial on this website:
https://www.testingdocs.com/java-tutorial/
For more information on Java, visit the official website :
https://www.oracle.com/java/
Related Posts

Java Tutorials /
Improving Java Performance with Multithreading

Download & Install Greenfoot on Windows
Java static code analysis, java testing tools, handle multiple exceptions in java.
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
- Java Ternary Operator with Examples
- Java Arithmetic Operators with Examples
- Java Assignment Operators with Examples
- Equality (==) operator in Java with Examples
- Java Logical Operators with Examples
- Java Relational Operators with Examples
- Short Circuit Logical Operators in Java with Examples
- Java Unary Operator with Examples
- Left Shift Operator in Java
- Java Ternary Operator Puzzle
- Difference between Simple and Compound Assignment in Java
- Shift Operator in Java
- & Operator in Java with Examples
- || operator in Java
- && operator in Java with Examples
- What is Java Ring?
- Types of References in Java
- Java Debugger (JDB)
- Remote Method Invocation in Java
Compound assignment operators in Java
Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand. The following are all possible assignment operator in java:
Implementation of all compound assignment operator
Rules for resolving the Compound assignment operators
At run time, the expression is evaluated in one of two ways.Depending upon the programming conditions:
- First, the left-hand operand is evaluated to produce a variable. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason; the right-hand operand is not evaluated and no assignment occurs.
- Otherwise, the value of the left-hand operand is saved and then the right-hand operand is evaluated. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason and no assignment occurs.
- Otherwise, the saved value of the left-hand variable and the value of the right-hand operand are used to perform the binary operation indicated by the compound assignment operator. If this operation completes abruptly, then the assignment expression completes abruptly for the same reason and no assignment occurs.
- Otherwise, the result of the binary operation is converted to the type of the left-hand variable, subjected to value set conversion to the appropriate standard value set, and the result of the conversion is stored into the variable.
- First, the array reference sub-expression of the left-hand operand array access expression is evaluated. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason; the index sub-expression (of the left-hand operand array access expression) and the right-hand operand are not evaluated and no assignment occurs.
- Otherwise, the index sub-expression of the left-hand operand array access expression is evaluated. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason and the right-hand operand is not evaluated and no assignment occurs.
- Otherwise, if the value of the array reference sub-expression is null, then no assignment occurs and a NullPointerException is thrown.
- Otherwise, the value of the array reference sub-expression indeed refers to an array. If the value of the index sub-expression is less than zero, or greater than or equal to the length of the array, then no assignment occurs and an ArrayIndexOutOfBoundsException is thrown.
- Otherwise, the value of the index sub-expression is used to select a component of the array referred to by the value of the array reference sub-expression. The value of this component is saved and then the right-hand operand is evaluated. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason and no assignment occurs.
Examples : Resolving the statements with Compound assignment operators
We all know that whenever we are assigning a bigger value to a smaller data type variable then we have to perform explicit type casting to get the result without any compile-time error. If we did not perform explicit type-casting then we will get compile time error. But in the case of compound assignment operators internally type-casting will be performed automatically, even we are assigning a bigger value to a smaller data-type variable but there may be a chance of loss of data information. The programmer will not responsible to perform explicit type-casting. Let’s see the below example to find the difference between normal assignment operator and compound assignment operator. A compound assignment expression of the form E1 op= E2 is equivalent to E1 = (T) ((E1) op (E2)), where T is the type of E1, except that E1 is evaluated only once.
For example, the following code is correct:
and results in x having the value 7 because it is equivalent to:
Because here 6.6 which is double is automatically converted to short type without explicit type-casting.
Refer: When is the Type-conversion required?
Explanation: In the above example, we are using normal assignment operator. Here we are assigning an int (b+1=20) value to byte variable (i.e. b) that’s results in compile time error. Here we have to do type-casting to get the result.
Explanation: In the above example, we are using compound assignment operator. Here we are assigning an int (b+1=20) value to byte variable (i.e. b) apart from that we get the result as 20 because In compound assignment operator type-casting is automatically done by compile. Here we don’t have to do type-casting to get the result.
Reference: http://docs.oracle.com/javase/specs/jls/se7/html/jls-15.html#jls-15.26.2
Please Login to comment...
Similar reads.
- Java-Operators
- 5 Reasons to Start Using Claude 3 Instead of ChatGPT
- 6 Ways to Identify Who an Unknown Caller
- 10 Best Lavender AI Alternatives and Competitors 2024
- The 7 Best AI Tools for Programmers to Streamline Development in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Blogs by Topic
The IntelliJ IDEA Blog
IntelliJ IDEA – the Leading Java and Kotlin IDE, by JetBrains
- Twitter Twitter
- Facebook Facebook
- Youtube Youtube
Java 22 and IntelliJ IDEA
Java 22 is here, fully supported by IntelliJ IDEA 2024.1 , allowing you to use these features now!
Java 22 has something for all – from new developers to Java experts, features related to performance and security for big organizations to those who like working with bleeding edge technology, from additions to the Java language to improvements in the JVM platform, and more.
It is also great to see how all these Java features, release after release, work together to create more possibilities and have a bigger impact on how developers create their applications that address existing pain points, perform better and are more secure.
This blog post doesn’t include a comprehensive coverage of all the Java 22 features. If you are interested in that, I’d recommend you to check out this link to know everything about what’s new and changing in Java 22, including the bugs.
In this blog post, I’ll cover how IntelliJ IDEA helps you get started, up and running with some of the Java 22 features, such as, String Templates , Implicitly Declared Classes and Instance Main Methods , Statements before super() , and Unnamed variables and patterns .
Over the past month, I published separate blog posts to cover each of these topics in detail. If you are new to these topics, I’d highly recommend you check out those detailed blog posts (I’ve included their links in the relevant subsections in this blog post). In this blog post, I’ll cover some sections from those blog posts, especially how IntelliJ IDEA supports them. Let’s start by configuring IntelliJ IDEA to work with the Java 22 features.
IntelliJ IDEA Configuration
Java 22 support is available in IntelliJ IDEA 2024.1 Beta . The final version will release soon in March 2024.
In your Project Settings, set the SDK to Java 22. For the language level, select ‘22 (Preview) – Statements before super(), string templates (2nd preview etc.)’ on both the Project and Modules tab, as shown in the below settings screenshot:
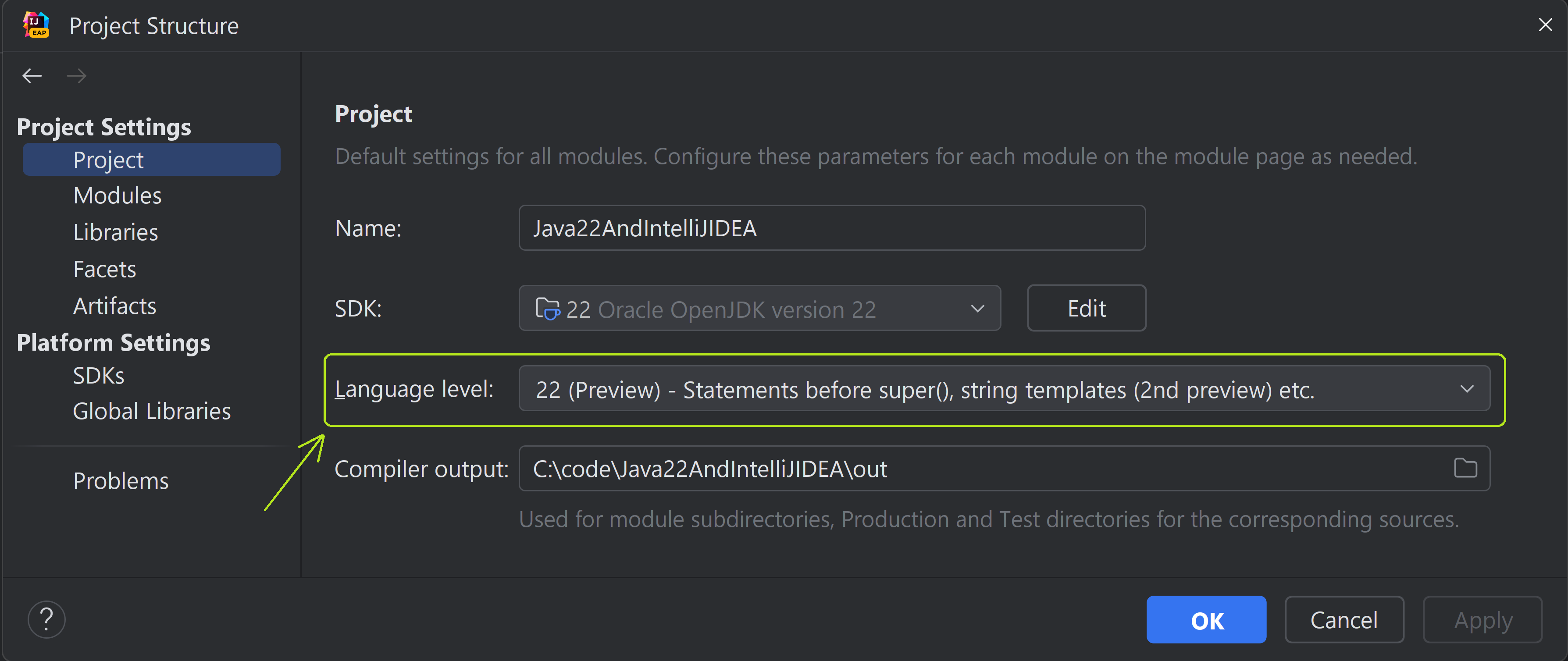
If you do not have Java 22 downloaded to your system yet, don’t worry; IntelliJ IDEA has your back! You could use the same Project settings window, select ‘Download JDK’, after you click on the drop down next to SDK. You’ll see the below popup that would enable you to choose from a list of vendors (such as Oracle OpenJDK, GraalVM, Azul Zulu and others):
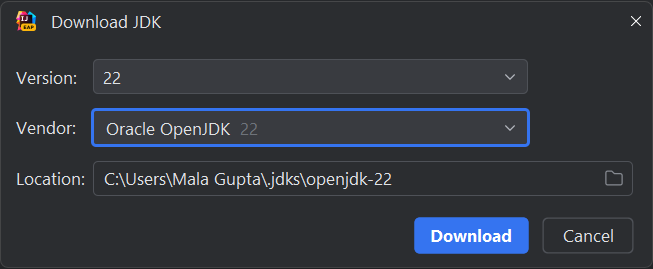
With the configuration under our belt, let’s get started with covering one of my favorite new features, that is, String Templates.
String Templates (Preview Language feature) The existing String concatenation options are difficult to work with and could be error prone; String templates offer a better alternative, that is, String interpolation with additional benefits such as validation, security and transformations via template processors.
Please check out my detailed blog post on this topic: String Templates in Java – why should you care? if you are new to this topic. It covers all the basics, including why you need String Templates, with multiple hands-on examples on built-in and user defined String processors.
IntelliJ IDEA can highlight code that could be replaced with String Templates Let’s assume you defined the following code to log a message that combines string literals and variable values using the concatenation operator:
The output from the preceding code could be an issue if you miss adding spaces in the String literals. The code isn’t quite easy to read or understand due to multiple opening and closing double quotes, that is, " and the + operator, and it would get worse if you add more literals, or variable values to it.
You could replace the preceding code with either StringBuilder.append() , String.format() or String.formatted() method or by using the class MessageFormat (as shown in my detailed blog post on this topic), but each of these methods have their own issues.
Don’t worry; IntelliJ IDEA could detect such code, suggest that you could replace it with String template, and do that for you, as shown below. It doesn’t matter if you are not even aware of the syntax of the String templates, IntelliJ IDEA has your back 🙂
Embedded expressions in String Templates and IntelliJ IDEA The syntax to embed a remplate expression (variable, expressible or a method call) is still new to what Java developers are used to and could be challenging to use without help. Don’t worry, IntelliJ IDEA has your back!
Each embedded expression must be enclosed within \{}. When you type \{, IntelliJ IDEA adds the closing ‘}’ for you. It also offers code completion to help you select a variable in scope, or any methods on it. If the code that you insert doesn’t compile, IntelliJ IDEA will highlight that too (as a compilation error), as shown in the following gif:
Using String Templates with Text Blocks Text blocks are quite helpful when working with string values that span multiple lines, such as, JSON, XML, HTML, SQL or other values that are usually processed by external environments. It is common for us Java developers to create such string values using a combination of string literals and variable values (variables, expressions or method calls).
The example below shows how IntelliJ IDEA could detect and create a text block using String templates for multiline string values that concatenates string literals with variable values. It also shows how IntelliJ IDEA provides code completion for variable names within such blocks. When you type in \{ , IntelliJ IDEA adds } . As you start typing the variable name countryName , it shows the available variables in that context:
Language injection and String Templates You could also inject a language or a reference in string values that spans single line or multiple lines, such as, a text block. By doing so, you get comprehensive coding assistance to edit the literal value. You could avail of this feature temporarily or permanently by using the @Language annotation, as shown below:
You can check out this link for detailed information on the benefits and usage of injecting language or reference in IntelliJ IDEA.
Predefined Template Processors With the String templates, you get access to predefined processors like the STR , FMT and RAW . I’d highly recommend you to check out my detailed blog post on String templates for multiple hands-on examples on it.
Custom Template Processor
Let’s work with a custom String template that isn’t covered in my previous blog post.
Imagine you’d like to create an instance of a record, say, WeatherData , that stores the details of the JSON we used in the previous section. Assume you define the following records to store this weather data represented by the JSON in the previous section:
You could create a method to return a custom String template that would process interpolated string, accept a class name ( WeatherData for this example) and return its instance:
Depending on the logic of your application, you might want to escape, delete or throw errors for the special characters that you encounter in the the JSON values interpolated via template expressions, as follows (the following method chooses to escape the special characters and include them as part of the JSON value):
You could initialize and use this custom JSON template processor as below. Note how elegant and concise the solution is with a combination of textblocks and String templates. The JSON is easy to read, write and understand (thanks to text blocks). The template expressions make it clear and obvious about the sections that are not constants and would be injected by the variables. At the end, the custom template processor WEATHER_JSON would ensure the resultant JSON is validated according to the logic you defined and returns an instance of WeatherData (doesn’t it sound magical?) :
Do not miss to check out my detailed blog post on this topic: String Templates in Java – why should you care? to discover how you could use the predefined String templates like FMT , to generate properly formatted receipts for, say, your neighborhood stationery store, or, say encode and decode combinations like :) or :( to emojis like 🙂 or ☹️. Does that sound fun to you?
Implicitly Declared Classes and Instance Main Methods (Preview language feature)
Introduced as a preview language feature in Java 21, this feature is in its second preview in Java 22.
It would revolutionize how new Java developers would get started learning Java. It simplifies the initial steps for students when they start learning basics, such as variable assignment, sequence, conditions and iteration. Students no longer need to declare an explicit class to develop their code, or write their main() method using this signature – public static void main(String []) . With this feature, classes could be declared implicitly and the main() method can be created with a shorter list of keywords.
If you are new to this feature, I’d highly recommend you to check out my detailed blog post: ‘HelloWorld’ and ‘main()’ meet minimalistic on this feature. In this blog post, I’ll include a few of the sections from it.
Class ‘HelloWorld’ before and after Java 21
Before Java 21, you would need to define a class, say, HelloWorld , that defined a main() method with a specific list of keywords, to print any text, say, ‘Hello World’ to the console, as follows:
With Java 21, this initial step has been shortened. You can define a source code file, say, HelloWorld.java, with the following code, to print a message to the console (it doesn’t need to define a class; it has a shorter signature for method main() ):
The preceding code is simpler than what was required earlier. Let’s see how this change could help you focus on what you need, rather than what you don’t.
Compiling and executing your code
Once you are done writing your code, the next step is to execute it.
On the command prompt, you could use the javac and java commands to compile and execute your code. Assuming you have defined your code in a source code file HelloWorld.java, you could use the following commands to run and execute it:
Since Java 11, it is possible to skip the compilation process for code defined in a single source code file, so you could use just the second command (by specifying the name of the source code file, as follows):
However, since instance main methods and implicit classes is a preview language feature, you should add the flag --enable-preview with --source 22 with these commands, as follows:
Sooner or later, you might switch to using an IDE to write your code. If you wish to use IntelliJ IDEA for creating instance main methods, here’s a quick list of steps to follow. Create a new Java project, select the build system as IntelliJ (so you could use Java compiler and runtime tools), create a new file, say, HelloWorld.java with your instance main method and set the properties to use Java 22, before you run your code, as shown in the following gif (It could save you from typing out the compilation/ execution commands on the command prompt each time you want to execute your code):
Are you wondering if it would be better to create a ‘Java class’ instead of a ‘File’ in the ‘src’ folder? The option of selecting a Java class would generate the body of a bare minimum class, say, public class HelloWorld { } . Since we are trying to avoid unnecessary keywords in the beginning, I recommended creating a new ‘File’ which wouldn’t include any code.
What else can main() do apart from printing messages to the console?
As included in my detailed post on this topic , I included multiple hand-on examples to show what you could achieve via just the main() method:
- Example 1. Variable declarations, assignments and simple calculations
- Example 2. Print patterns, such as, big letters using a specified character
- Example 3. Animating multiline text – one word at a time
- Example 4. Data structure problems
- Example 5. Text based Hangman game
The idea to include multiple examples as listed above is to demonstrate the power of sequence, condition and iteration all of which can be included in the main() method, to build good programming foundations with problem solving skills. By using the run command or the icon to run and execute their code in IntelliJ IDEA, new programmers reduce another step when getting started.
Changing an implicit class to a regular class
When you are ready to level up and work with other concepts like user defined classes, you could also covert the implicit classes and code that we used in the previous examples, to regular classes, as shown below:
What happens when you create a source code file with method main(), but no class declaration?
Behind the scenes, the Java compiler creates an implicit top level class, with a no-argument constructor, so that these classes don’t need to be treated in a way that is different to the regular classes.
Here’s a gif that shows a decompiled class for you for the source code file AnimateText.java:
Variations of the main method in the implicit class
As we are aware, a method can be overloaded. Does that imply an implicit class can define multiple main methods? If yes, which one of them qualifies as the ‘main’ method? This is an interesting question. First of all, know that you can’t define a static and non-static main method with the same signature, that is, with the same method parameters. The following method are considered valid main() methods in an implicit class:
If there is no valid main method detected, IntelliJ IDEA could add one for you, as shown in the following gif:
Educators could use this feature to introduce other concepts to the students in an incremental way
If you are an educator, you could introduce your students to other commonly used programming practices, such as creating methods- that is delegating part of your code to another method and calling it from the main method. You could also talk about passing values vs. variables to these methods.
The following gif shows how to do it:
Statements before super() – a preview language feature
Typically, we create alternative solutions for tasks that are necessary, but not officially permitted. For instance, executing statements before super() in a derived class constructor was not officially allowed, even though it was important for, say, validating values being passed to the base class constructor. A popular workaround involved creating static methods to validate values and then calling these methods on the arguments of super() . Though this approach worked well, it could make the code look complicated. This is changing with Statements before super() , a preview language feature in Java 22.
By using this feature, you can opt for a more direct approach, that is, drop the workaround of creating static methods, and execute code that validates arguments, just before calling super() . Terms and conditions still apply, such as, not accessing instance members of a derived class before execution of super() completes.
An example – Validating values passed to super() in a derived class constructor Imagine you need to create a class, say, IndustryElement , that extends class Element , which is defined as follows:
The constructor of the class Element misses checking if the atomicNumber is in the range of 1-118 (all known elements have atomic numbers between 1 to 118). Often the source code of a base class is not accessible or open for modification. How would you validate the values passed to atomicNumber in the constructor of class IndustryElement ?
Until Java 21, no statements were allowed to execute before super() . Here’s one of the ways we developers found a workaround by defining and calling static methods (static methods belong to a class and not to instances and can be executed before any instance of a class exists):
Starting Java 22, you could inline the contents of your static method in the constructor for your derived class, as shown in the following gif:
Here’s the resultant code for your reference:
Where else would you use this feature? If you are new to this feature, I’d recommend that you check out my detailed blog post, Constructor Makeover in Java 22 , in which I’ve covered this feature in detail using the following examples:
- Example 2 – base class constructor parameters that use annotations for validations
- Example 3 – Transforming variable values received in a derived class constructor, before calling a base class constructor.
- Example 4 – Executing statements before this() in constructor of Records
- Example 5 – Executing statements before this() in Enum constructors
- Example 6 – Executing statements before this() in classes
How does it work behind the scenes? The language syntax has been relaxed but it doesn’t change or impact the internal JVM instructions. There are no changes to the JVM instructions for this new feature because the order of execution of the constructors still remains unchanged – from base class to a derived class. Also, this feature still doesn’t allow you to use members of a derived class instance, until super() executes.
Let’s access and compare the instruction set of the constructor of class IndustryElement , before and after its modification – one that can execute statements before super() and the one that doesn’t. To do so, use the following command:
Here’s the instruction set for the constructor that doesn’t explicitly execute statements before super() and calls static methods to validate range of atomic number:
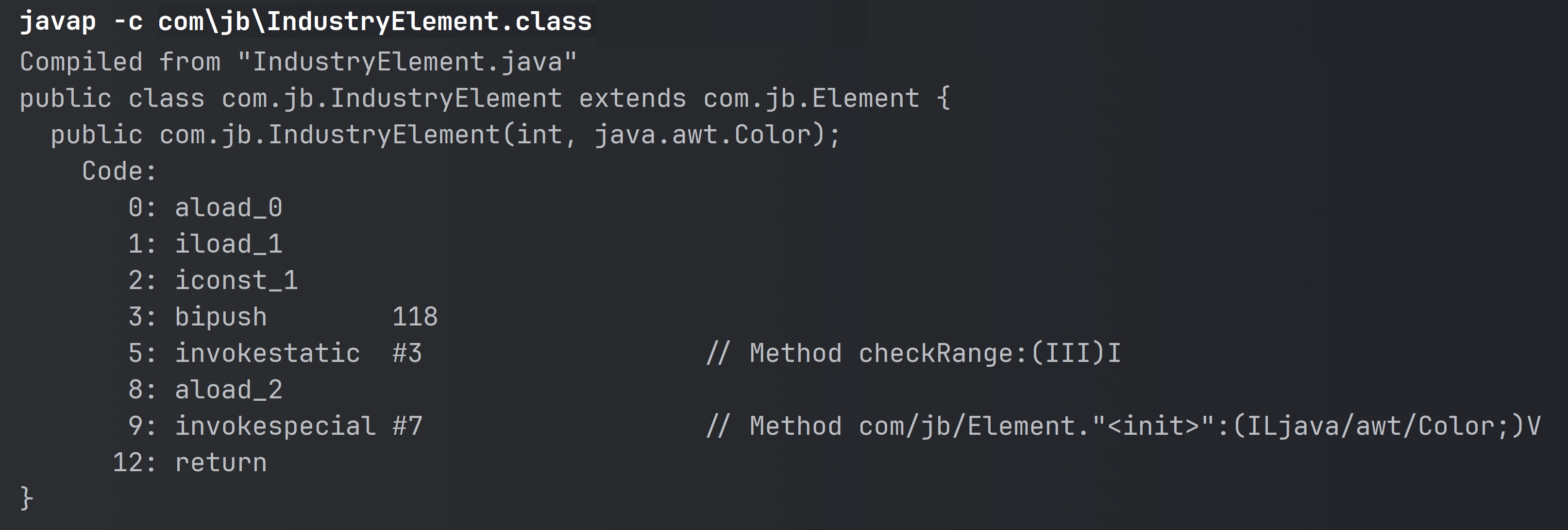
Here’s instruction set for the constructor that explicitly executes statements before super() to validate range of atomic number:
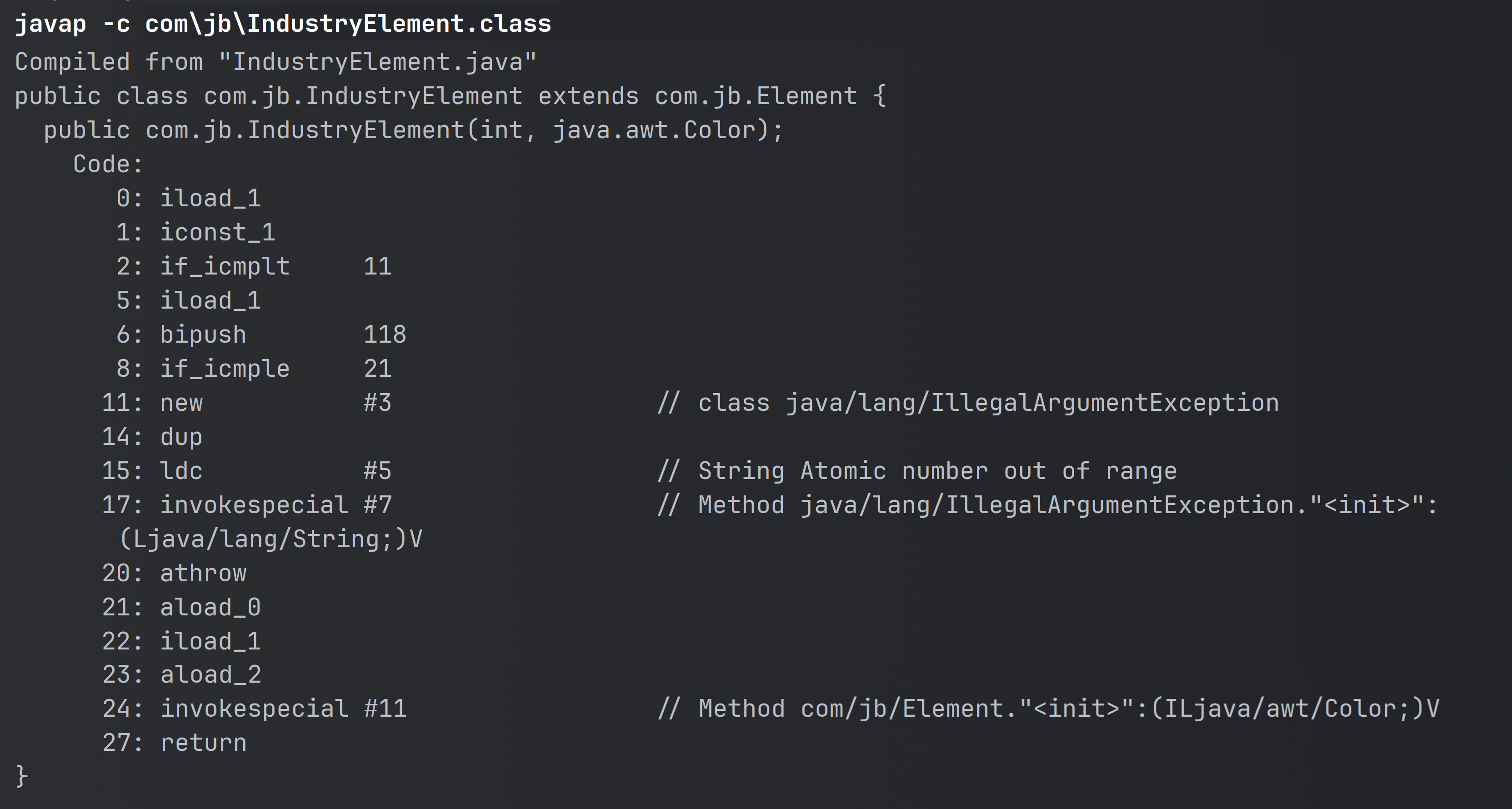
The most important point to note here is that in both the cases, the constructor of the base class, that is, Element is called, after the execution of all other statements. Essentially, it means, you are still doing the same thing, it is just packaged in a way that makes things easier for you.
I understand it is difficult to remember what each of these instruction codes means. Access the following link and search for the instruction code and following the above instructions set would be a breeze:
https://docs.oracle.com/javase/specs/jvms/se21/html/jvms-6.html#jvms-6.5.aload_n
Can you execute ‘any’ statements before calling super()?
No. If the statements before super() try to access instance variables or execute methods of your derived class, your code won’t compile. For example, if you change the static checkRange() method to an instance method, your code won’t compile, as shown below:
Unnamed Variables and Patterns
Starting Java 22, using Unnamed Variables & Patterns you can mark unused local variables, patterns and pattern variables to be ignored, by replacing their names (or their types and names) with an underscore, that is, _ . Since such variables and patterns no longer have a name, they are referred to as Unnamed variables and patterns. Ignoring unused variables would reduce the time and energy anyone would need to understand a code snippet. In the future, this could prevent errors :-). This language feature doesn’t apply to instance or class variables.
Are you wondering if replacing unused variables with _ is always a good idea, or do they imply code smells and should you consider refactoring your codebase to remove them? Those are good questions to ask. If you are new to this topic, I’d recommend you to check out my detailed blog post: Drop the Baggage: Use ‘_’ for Unnamed Local Variables and Patterns in Java 22 to find out answer to this question.
Since this is not a preview language feature, set Language Level in your Project Settings to ‘22 – Unnamed variables and patterns’ on both the Project and Modules tab, as shown in the below settings screenshot:
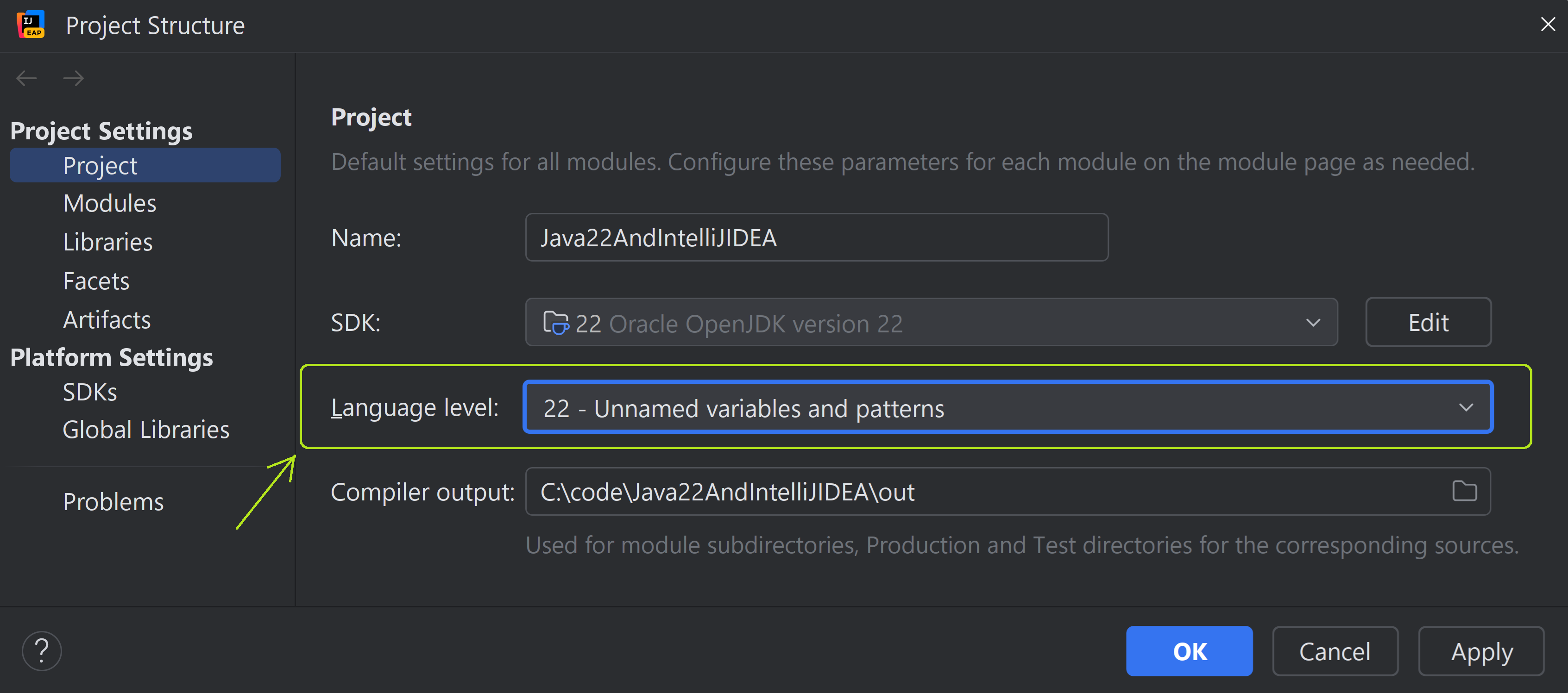
A quick example
The following gif gives a sneak peek into how an unused local variable, connection, is detected by IntelliJ IDEA, and could be replaced with an underscore, that is, _ .
The modified code shown in the preceding gif makes it clear that the local variable defined in the try-with-resources statement is unused, making it concise and easier to understand.
Unused Patterns and Pattern variables in switch constructs
Imagine you defined a sealed interface, say, GeometricShape , and records to represent shapes, such as, Point , Line , Triangle , Square , as shown in the following code:
Now assume you need a method that accepts an instance of GeometricShape and returns its area. Since Point and a Line are considered one-dimensional shapes, they wouldn’t have an area. Following is one of the ways to define such method that calculates and returns area:
In the previous example, the patterns int x , int y , Point a and Point B (for case label Line) remain unused as detected by IntelliJ IDEA. These could be replaced by an _ . Also, since all the record components of the case Point remain unused, it could be replaced as Point _ . This could also allow us to merge the first and second case labels. All of these steps are shown in the following gif:
Here’s the modified code for your reference:
In the preceding example, you can’t delete the pattern variables even if they are unused. The code must include the cases when the instance passed to the method calcArea() is of type Point and Line , so that it could return 0 for them.
Unused Patterns or variables with nested records
This feature also comes in quite handy for nested records with multiple unused patterns or pattern variables, as demonstrated using the following example code:
In the preceding code, since the if condition in the method checkFirstNameAndCountryCodeAgain uses only two pattern variables, others could be replaced using _ ; it reduced the noise in the code too.
Where else can you use this feature? Checkout my detailed detailed blog post: Drop the Baggage: Use ‘_’ for Unnamed Local Variables and Patterns in Java 22 to learn more about other use cases where this feature can be used:
- Requirements change, but you need side effects of constructs like an enhanced for-loop
- Unused parameters in exception handlers; whose signature can’t be modified
- Unused auto-closeable resources in try-with-resources statements
It isn’t advisable to use this feature without realising if an unused variable or pattern is a code smell or not. I used these examples to show that at times it might be better to refactor your code to get rid of the unused variable instead of just replacing it with an underscore, that is, _ .
- Unused lambda parameter
- Methods with multiple responsibilities
Preview Features
‘String Templates’, ‘Implicitly Declared Classes and Instance Main Methods’ and ‘Statements before super()’ are preview language features in Java 22. With Java’s new release cadence of six months, new language features are released as preview features. They may be reintroduced in later Java versions in the second or more preview, with or without changes. Once they are stable enough, they may be added to Java as a standard language feature.
Preview language features are complete but not permanent, which essentially means that these features are ready to be used by developers, although their finer details could change in future Java releases depending on developer feedback. Unlike an API, language features can’t be deprecated in the future. So, if you have feedback about any of the preview language features, feel free to share it on the JDK mailing list (free registration required).
Because of how these features work, IntelliJ IDEA is committed to only supporting preview features for the current JDK. Preview language features can change across Java versions, until they are dropped or added as a standard language feature. Code that uses a preview language feature from an older release of the Java SE Platform might not compile or run on a newer release.
In this blog post, I covered four Java 22 features – String Templates , Implicitly Declared Classes and Instance Main Methods , Statements before super() , and Unnamed variable and patterns .
String Templates is a great addition to Java. Apart from helping developers to work with strings that combine string constants and variables, they provide a layer of security. Custom String templates can be created with ease to accomplish multiple tasks, such as, to decipher letter combinations, either ignoring them or replacing them for added security.
Java language designers are reducing the ceremony that is required to write the first HelloWorld code for Java students, by introducing implicitly declared classes and instance main methods. New students can start with bare minimum main() method, such as, void main() and build strong programming foundation by polishing their skills with basics like sequence, selection and iteration.
In Java 22, the feature Statements before super() lets you execute code before calling super() in your derived class constructors, this() in your records or enums, so that you could validate the method parameters, or transform values, as required. This avoids creating workarounds like creating static methods and makes your code easier to read and understand. This feature doesn’t change how constructors would operate now vs. how they operated earlier – the JVM instructions remain the same.
Unnamed variables are local to a code construct, they don’t have a name, and they are represented by using an underscore, that is, _ . They can’t be passed to methods, or used in expressions. By replacing unused local variables in a code base with _ their intention is conveyed very clearly. It clearly communicates to anyone reading a code snippet that the variable is not used elsewhere. Until now, this intention could only be communicated via comments, which, unfortunately, all developers don’t write.
Happy Coding!
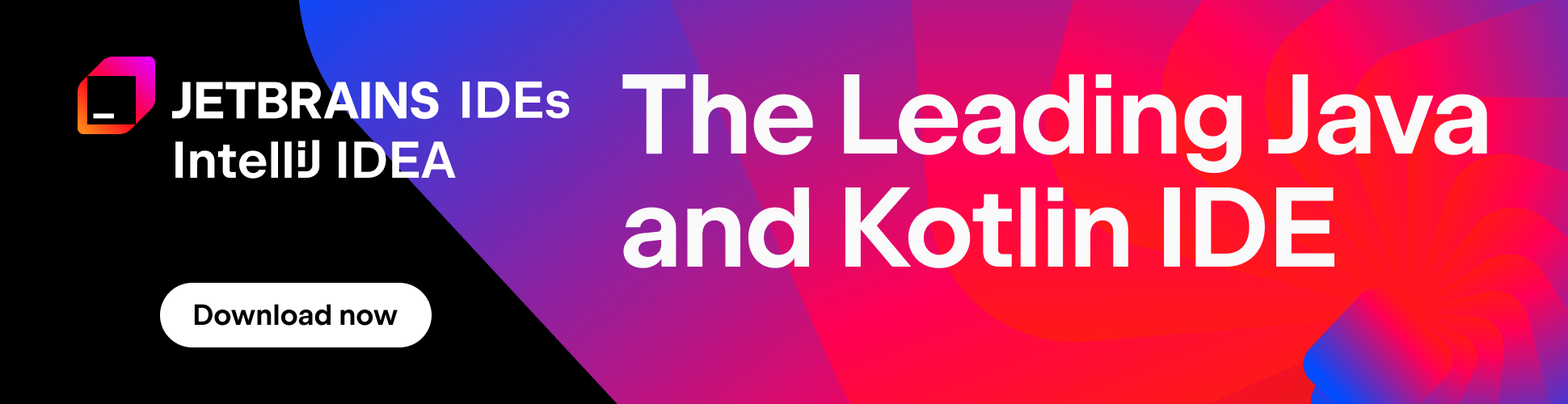
Subscribe to IntelliJ IDEA Blog updates
By submitting this form, I agree that JetBrains s.r.o. ("JetBrains") may use my name, email address, and location data to send me newsletters, including commercial communications, and to process my personal data for this purpose. I agree that JetBrains may process said data using third-party services for this purpose in accordance with the JetBrains Privacy Policy . I understand that I can revoke this consent at any time in my profile . In addition, an unsubscribe link is included in each email.
Thanks, we've got you!
Discover more
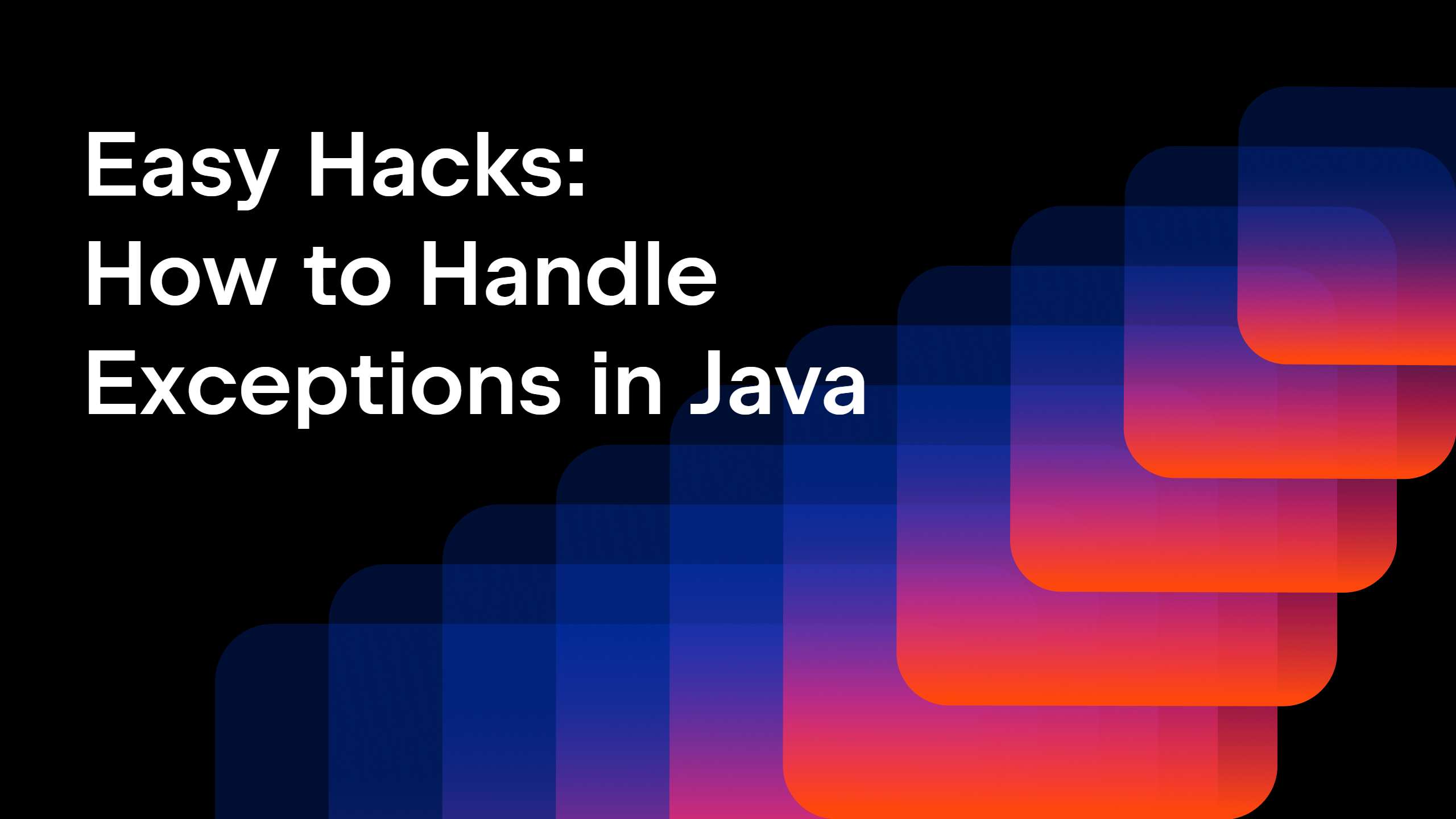
Easy Hacks: How to Handle Exceptions in Java
Previously, we explored how to throw exceptions in Java to signal error conditions in our code. But throwing exceptions is only half the story. To build robust and reliable applications, we also need to know how to handle them effectively. In this post, we’ll explore how you can handle exceptions…

Java Frameworks You Must Know in 2024
Many developers trust Java worldwide because it is adaptable, secure, and versatile, making it perfect for developing various applications across multiple platforms. It also stands out for its readability and scalability. What are frameworks? Why do you need them? Since Java has a long history…

Java Annotated Monthly – April 2024
Welcome to this month’s Java Annotated Monthly, where we’ll cover the most prominent updates, news, and releases in March. This edition is truly special because it introduces a new section – Featured content, where we invite influential people from the industry to share a personal selection of inter…
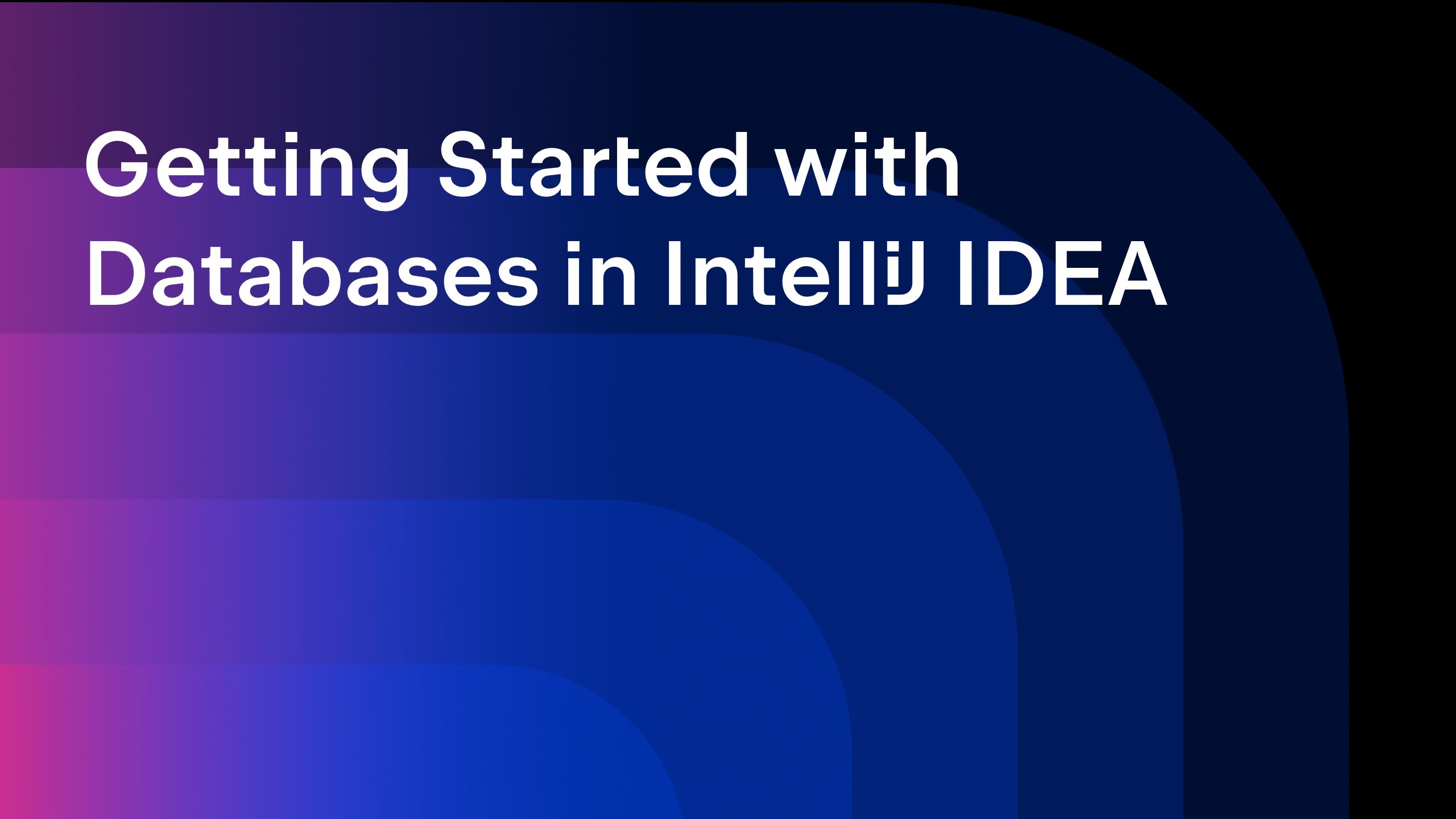
Getting Started with Databases in IntelliJ IDEA
Have you ever wondered how IntelliJ IDEA handles databases? Well, today is the perfect opportunity to find out in our database tutorial on initial setups and first steps. All of the features you’ll need when working with databases are available out of the box in IntelliJ IDEA Ultimate…

IMAGES
VIDEO
COMMENTS
Even if what you tried were to work, what would be the point? Assuming you could define the variable scope inside the test, your variable v would not exist outside that scope. Hence, creating the variable and assigning the value would be pointless, for you would not be able to use it. Variables exist only in the scope they were created.
An assignment statement designates a value for a variable. An assignment statement can be used as an expression in Java. After a variable is declared, you can assign a value to it by using an assignment statement. In Java, the equal sign = is used as the assignment operator. The syntax for assignment statements is as follows: variable ...
In this lesson, you will learn about assignment statements and expressions that contain math operators and variables. 1.4.1. Assignment Statements ¶. Remember that a variable holds a value that can change or vary. Assignment statements initialize or change the value stored in a variable using the assignment operator =.
So far, the example programs have been using the value initially put into a variable. Programs can change the value in a variable. An assignment statement changes the value that is held in a variable. The program uses an assignment statement. The assignment statement puts the value 123 into the variable.
1. String title = "I love Java"; If we assign a value to a variable of the char type, we need to put it in single quotes: 1. char letter = 'M'; If we assign a value to a variable of the float type, we need to add the letter "f": "Initialization" means "to assign an initial value to a variable.".
The data type of the value returned by an expression depends on the elements used in the expression. The expression cadence = 0 returns an int because the assignment operator returns a value of the same data type as its left-hand operand; in this case, cadence is an int.As you can see from the other expressions, an expression can return other types of values as well, such as boolean or String.
This operator can also be used on objects to assign object references, as discussed in Creating Objects. The Arithmetic Operators. The Java programming language provides operators that perform addition, subtraction, multiplication, and division. There's a good chance you'll recognize them by their counterparts in basic mathematics.
They provide a concise way to perform an operation and assign the result to the variable in one step. The Compound Operator is utilized when +,-,*, and / are used in conjunction with the = operator. 1. Simple Assignment Operator (=): The equal sign (=) is the basic assignment operator in Java. It is used to assign the value on the right-hand ...
Compound Assignment Operators. Sometime we need to modify the same variable value and reassigned it to a same reference variable. Java allows you to combine assignment and addition operators using a shorthand operator. For example, the preceding statement can be written as: i +=8; //This is same as i = i+8; The += is called the addition ...
variable operator value; Types of Assignment Operators in Java. The Assignment Operator is generally of two types. They are: 1. Simple Assignment Operator: The Simple Assignment Operator is used with the "=" sign where the left side consists of the operand and the right side consists of a value. The value of the right side must be of the same data type that has been defined on the left side.
An expression is a construct made up of variables, operators, and method invocations, which are constructed according to the syntax of the language, that evaluates to a single value. You have already seen examples of expressions, illustrated in code below: int cadence = 0; anArray[0] = 100; System.out.println("Element 1 at index 0: " + anArray ...
In Java, there are different types of variables, for example: String - stores text, such as "Hello". String values are surrounded by double quotes. int - stores integers (whole numbers), without decimals, such as 123 or -123. float - stores floating point numbers, with decimals, such as 19.99 or -19.99. char - stores single characters, such as ...
Assignment. Assignment in Java is the process of giving a value to a primitive-type variable or giving an object reference to an object-type variable. The equals sign acts as assignment operator in Java, followed by the value to assign. The following sample Java code demonstrates assigning a value to a primitive-type integer variable, which has ...
The definite assignment will consider the structure of expressions and statements. The Java compiler will decide that "k" is assigned before its access, like an argument with the method invocation in the code. It is because the access will occur if the value of the expression is accurate.
In this tutorial, we will learn about Java Assignment Statement. We can assign or give value to a variable using the assignment statement. Syntax. The general syntax for assigning a value to a variable is as follows: variable_name = value; We can also assign value to a variable as part of the declaration. This is known as variable initialization.
Array Variable Assignment in Java. An array is a collection of similar types of data in a contiguous location in memory. After Declaring an array we create and assign it a value or variable. During the assignment variable of the array things, we have to remember and have to check the below condition. 1.
The following are all possible assignment operator in java: 1. += (compound addition assignment operator) 2. -= (compound subtraction assignment operator) 3. *= (compound multiplication assignment operator) 4. /= (compound division assignment operator) 5. %= (compound modulo assignment operator)
However, with a JDK 1.4 on Solaris I think that that the value is null (like if the assignment was performed even though the exception is thrown). This is obviously linked to a bug and we will deploy a version without the assignment just to make sure but we would like to know if one of you also noticed this or have some kind of explanation to ...
It simplifies the initial steps for students when they start learning basics, such as variable assignment, sequence, conditions and iteration. ... In Java 22, the feature Statements before super() lets you execute code before calling super() in your derived class constructors, this() in your records or enums, so that you could validate the ...
The scope of a label of a labeled statement is the immediately contained Statement. In other words, case 1, case 2 are labels within the switch statement. break and continue statements can be applied to labels. Because labels share the scope of the statement, all variables defined within labels share the scope of the switch statement.