Destructuring assignment
The two most used data structures in JavaScript are Object and Array .
- Objects allow us to create a single entity that stores data items by key.
- Arrays allow us to gather data items into an ordered list.
However, when we pass these to a function, we may not need all of it. The function might only require certain elements or properties.
Destructuring assignment is a special syntax that allows us to “unpack” arrays or objects into a bunch of variables, as sometimes that’s more convenient.
Destructuring also works well with complex functions that have a lot of parameters, default values, and so on. Soon we’ll see that.

Array destructuring
Here’s an example of how an array is destructured into variables:
Now we can work with variables instead of array members.
It looks great when combined with split or other array-returning methods:
As you can see, the syntax is simple. There are several peculiar details though. Let’s see more examples to understand it better.
It’s called “destructuring assignment,” because it “destructurizes” by copying items into variables. However, the array itself is not modified.
It’s just a shorter way to write:
Unwanted elements of the array can also be thrown away via an extra comma:
In the code above, the second element of the array is skipped, the third one is assigned to title , and the rest of the array items are also skipped (as there are no variables for them).
…Actually, we can use it with any iterable, not only arrays:
That works, because internally a destructuring assignment works by iterating over the right value. It’s a kind of syntax sugar for calling for..of over the value to the right of = and assigning the values.
We can use any “assignables” on the left side.
For instance, an object property:
In the previous chapter, we saw the Object.entries(obj) method.
We can use it with destructuring to loop over the keys-and-values of an object:
The similar code for a Map is simpler, as it’s iterable:
There’s a well-known trick for swapping values of two variables using a destructuring assignment:
Here we create a temporary array of two variables and immediately destructure it in swapped order.
We can swap more than two variables this way.
The rest ‘…’
Usually, if the array is longer than the list at the left, the “extra” items are omitted.
For example, here only two items are taken, and the rest is just ignored:
If we’d like also to gather all that follows – we can add one more parameter that gets “the rest” using three dots "..." :
The value of rest is the array of the remaining array elements.
We can use any other variable name in place of rest , just make sure it has three dots before it and goes last in the destructuring assignment.
Default values
If the array is shorter than the list of variables on the left, there will be no errors. Absent values are considered undefined:
If we want a “default” value to replace the missing one, we can provide it using = :
Default values can be more complex expressions or even function calls. They are evaluated only if the value is not provided.
For instance, here we use the prompt function for two defaults:
Please note: the prompt will run only for the missing value ( surname ).
Object destructuring
The destructuring assignment also works with objects.
The basic syntax is:
We should have an existing object on the right side, that we want to split into variables. The left side contains an object-like “pattern” for corresponding properties. In the simplest case, that’s a list of variable names in {...} .
For instance:
Properties options.title , options.width and options.height are assigned to the corresponding variables.
The order does not matter. This works too:
The pattern on the left side may be more complex and specify the mapping between properties and variables.
If we want to assign a property to a variable with another name, for instance, make options.width go into the variable named w , then we can set the variable name using a colon:
The colon shows “what : goes where”. In the example above the property width goes to w , property height goes to h , and title is assigned to the same name.
For potentially missing properties we can set default values using "=" , like this:
Just like with arrays or function parameters, default values can be any expressions or even function calls. They will be evaluated if the value is not provided.
In the code below prompt asks for width , but not for title :
We also can combine both the colon and equality:
If we have a complex object with many properties, we can extract only what we need:
The rest pattern “…”
What if the object has more properties than we have variables? Can we take some and then assign the “rest” somewhere?
We can use the rest pattern, just like we did with arrays. It’s not supported by some older browsers (IE, use Babel to polyfill it), but works in modern ones.
It looks like this:
In the examples above variables were declared right in the assignment: let {…} = {…} . Of course, we could use existing variables too, without let . But there’s a catch.
This won’t work:
The problem is that JavaScript treats {...} in the main code flow (not inside another expression) as a code block. Such code blocks can be used to group statements, like this:
So here JavaScript assumes that we have a code block, that’s why there’s an error. We want destructuring instead.
To show JavaScript that it’s not a code block, we can wrap the expression in parentheses (...) :
Nested destructuring
If an object or an array contains other nested objects and arrays, we can use more complex left-side patterns to extract deeper portions.
In the code below options has another object in the property size and an array in the property items . The pattern on the left side of the assignment has the same structure to extract values from them:
All properties of options object except extra that is absent in the left part, are assigned to corresponding variables:
Finally, we have width , height , item1 , item2 and title from the default value.
Note that there are no variables for size and items , as we take their content instead.
Smart function parameters
There are times when a function has many parameters, most of which are optional. That’s especially true for user interfaces. Imagine a function that creates a menu. It may have a width, a height, a title, items list and so on.
Here’s a bad way to write such a function:
In real-life, the problem is how to remember the order of arguments. Usually IDEs try to help us, especially if the code is well-documented, but still… Another problem is how to call a function when most parameters are ok by default.
That’s ugly. And becomes unreadable when we deal with more parameters.
Destructuring comes to the rescue!
We can pass parameters as an object, and the function immediately destructurizes them into variables:
We can also use more complex destructuring with nested objects and colon mappings:
The full syntax is the same as for a destructuring assignment:
Then, for an object of parameters, there will be a variable varName for property incomingProperty , with defaultValue by default.
Please note that such destructuring assumes that showMenu() does have an argument. If we want all values by default, then we should specify an empty object:
We can fix this by making {} the default value for the whole object of parameters:
In the code above, the whole arguments object is {} by default, so there’s always something to destructurize.
Destructuring assignment allows for instantly mapping an object or array onto many variables.
The full object syntax:
This means that property prop should go into the variable varName and, if no such property exists, then the default value should be used.
Object properties that have no mapping are copied to the rest object.
The full array syntax:
The first item goes to item1 ; the second goes into item2 , all the rest makes the array rest .
It’s possible to extract data from nested arrays/objects, for that the left side must have the same structure as the right one.
We have an object:
Write the destructuring assignment that reads:
- name property into the variable name .
- years property into the variable age .
- isAdmin property into the variable isAdmin (false, if no such property)
Here’s an example of the values after your assignment:
The maximal salary
There is a salaries object:
Create the function topSalary(salaries) that returns the name of the top-paid person.
- If salaries is empty, it should return null .
- If there are multiple top-paid persons, return any of them.
P.S. Use Object.entries and destructuring to iterate over key/value pairs.
Open a sandbox with tests.
Open the solution with tests in a sandbox.
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy

JAVASCRIPT ASSIGNMENT OPERATORS
In this tutorial, you will learn about all the different assignment operators in javascript and how to use them in javascript.
Assignment Operators
In javascript, there are 16 different assignment operators that are used to assign value to the variable. It is shorthand of other operators which is recommended to use.
The assignment operators are used to assign value based on the right operand to its left operand.
The left operand must be a variable while the right operand may be a variable, number, boolean, string, expression, object, or combination of any other.
One of the most basic assignment operators is equal = , which is used to directly assign a value.
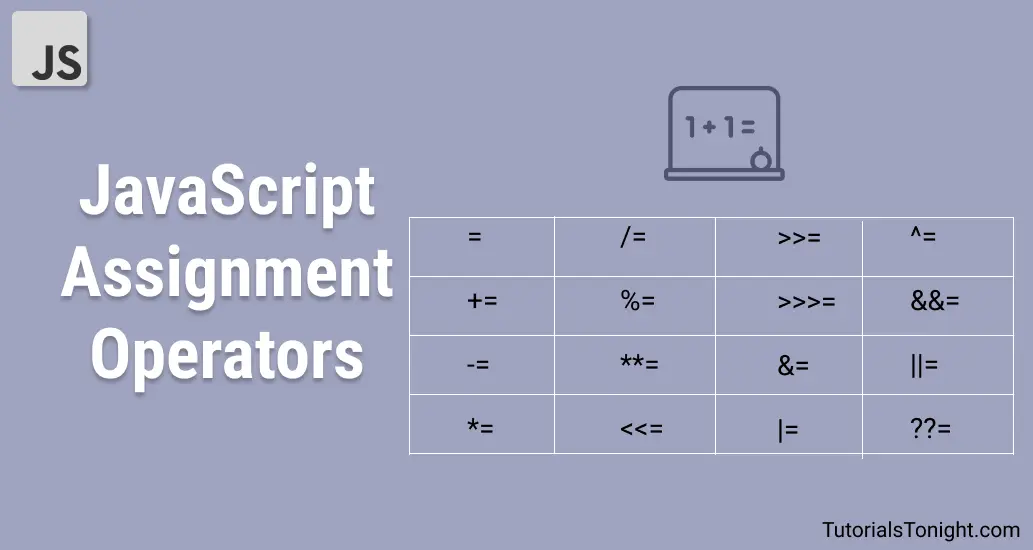
Assignment Operators List
Here is the list of all assignment operators in JavaScript:
In the following table if variable a is not defined then assume it to be 10.
Assignment operator
The assignment operator = is the simplest value assigning operator which assigns a given value to a variable.
The assignment operators support chaining, which means you can assign a single value in multiple variables in a single line.
Addition assignment operator
The addition assignment operator += is used to add the value of the right operand to the value of the left operand and assigns the result to the left operand.
On the basis of the data type of variable, the addition assignment operator may add or concatenate the variables.
Subtraction assignment operator
The subtraction assignment operator -= subtracts the value of the right operand from the value of the left operand and assigns the result to the left operand.
If the value can not be subtracted then it results in a NaN .
Multiplication assignment operator
The multiplication assignment operator *= assigns the result to the left operand after multiplying values of the left and right operand.
Division assignment operator
The division assignment operator /= divides the value of the left operand by the value of the right operand and assigns the result to the left operand.
Remainder assignment operator
The remainder assignment operator %= assigns the remainder to the left operand after dividing the value of the left operand by the value of the right operand.
Exponentiation assignment operator
The exponential assignment operator **= assigns the result of exponentiation to the left operand after exponentiating the value of the left operand by the value of the right operand.
Left shift assignment
The left shift assignment operator <<= assigns the result of the left shift to the left operand after shifting the value of the left operand by the value of the right operand.
Right shift assignment
The right shift assignment operator >>= assigns the result of the right shift to the left operand after shifting the value of the left operand by the value of the right operand.
Unsigned right shift assignment
The unsigned right shift assignment operator >>>= assigns the result of the unsigned right shift to the left operand after shifting the value of the left operand by the value of the right operand.
Bitwise AND assignment
The bitwise AND assignment operator &= assigns the result of bitwise AND to the left operand after ANDing the value of the left operand by the value of the right operand.
Bitwise OR assignment
The bitwise OR assignment operator |= assigns the result of bitwise OR to the left operand after ORing the value of left operand by the value of the right operand.
Bitwise XOR assignment
The bitwise XOR assignment operator ^= assigns the result of bitwise XOR to the left operand after XORing the value of the left operand by the value of the right operand.
Logical AND assignment
The logical AND assignment operator &&= assigns value to left operand only when it is truthy .
Note : A truthy value is a value that is considered true when encountered in a boolean context.
Logical OR assignment
The logical OR assignment operator ||= assigns value to left operand only when it is falsy .
Note : A falsy value is a value that is considered false when encountered in a boolean context.
Logical nullish assignment
The logical nullish assignment operator ??= assigns value to left operand only when it is nullish ( null or undefined ).
Array vs Object Destructuring in JavaScript – What’s the Difference?

The destructuring assignment in JavaScript provides a neat and DRY way to extract values from your arrays and objects.
This article aims to show you exactly how array and object destructuring assignments work in JavaScript.
So, without any further ado, let’s get started with array destructuring.
What Is Array Destructuring?
Array destructuring is a unique technique that allows you to neatly extract an array’s value into new variables.
For instance, without using the array destructuring assignment technique, you would copy an array’s value into a new variable like so:
Try it on StackBlitz
Notice that the snippet above has a lot of repeated code which is not a DRY ( D on’t R epeat Y ourself) way of coding.
Let’s now see how array destructuring makes things neater and DRYer.
You see, like magic, we’ve cleaned up our code by placing the three new variables (that is, firstName , lastName , and website ) into an array object ( [...] ). Then, we assigned them the profile array's values.
In other words, we instructed the computer to extract the profile array’s values into the variables on the left-hand side of the assignment operator .
Therefore, JavaScript will parse the profile array and copy its first value ( "Oluwatobi" ) into the destructuring array’s first variable ( firstName ).
Likewise, the computer will extract the profile array’s second value ( "Sofela" ) into the destructuring array’s second variable ( lastName ).
Lastly, JavaScript will copy the profile array’s third value ( "codesweetly.com" ) into the destructuring array’s third variable ( website ).
Notice that the snippet above destructured the profile array by referencing it. However, you can also do direct destructuring of an array. Let’s see how.
How to Do Direct Array Destructuring
JavaScript lets you destructure an array directly like so:
Suppose you prefer to separate your variable declarations from their assignments. In that case, JavaScript has you covered. Let’s see how.
How to Use Array Destructuring While Separating Variable Declarations from Their Assignments
Whenever you use array destructuring, JavaScript allows you to separate your variable declarations from their assignments.
Here’s an example:
What if you want "Oluwatobi" assigned to the firstName variable—and the rest of the array items to another variable? How you do that? Let’s find out below.
How to Use Array Destructuring to Assign the Rest of an Array Literal to a Variable
JavaScript allows you to use the rest operator within a destructuring array to assign the rest of a regular array to a variable.
Note: Always use the rest operator as the last item of your destructuring array to avoid getting a SyntaxError .
Now, what if you only want to extract "codesweetly.com" ? Let's discuss the technique you can use below.
How to Use Array Destructuring to Extract Values at Any Index
Here’s how you can use array destructuring to extract values at any index position of a regular array:
In the snippet above, we used commas to skip variables at the destructuring array's first and second index positions.
By so doing, we were able to link the website variable to the third index value of the regular array on the right side of the assignment operator (that is, "codesweetly.com" ).
At times, however, the value you wish to extract from an array is undefined . In that case, JavaScript provides a way to set default values in the destructuring array. Let’s learn more about this below.
How Default Values Work in an Array Destructuring Assignment
Setting a default value can be handy when the value you wish to extract from an array does not exist (or is set to undefined ).
Here’s how you can set one inside a destructuring array:
In the snippet above, we set "Tobi" and "CodeSweetly" as the default values of the firstName and website variables.
Therefore, in our attempt to extract the first index value from the right-hand side array, the computer defaulted to "CodeSweetly" —because only a zeroth index value exists in ["Oluwatobi"] .
So, what if you need to swap firstName ’s value with that of website ? Again, you can use array destructuring to get the job done. Let’s see how.
How to Use Array Destructuring to Swap Variables’ Values
You can use the array destructuring assignment to swap the values of two or more different variables.
Here's an example:
In the snippet above, we used direct array destructuring to reassign the firstName and website variables with the values of the array literal on the right-hand side of the assignment operator.
As such, firstName ’s value will change from "Oluwatobi" to "CodeSweetly" . While website ’s content will change from "CodeSweetly" to "Oluwatobi" .
Keep in mind that you can also use array destructuring to extract values from a regular array to a function’s parameters. Let’s talk more about this below.
How to Use Array Destructuring to Extract Values from an Array to a Function’s Parameters
Here’s how you can use array destructuring to extract an array’s value to a function’s parameter:
In the snippet above, we used an array destructuring parameter to extract the profile array’s values into the getUserBio ’s firstName and lastName parameters.
Note: An array destructuring parameter is typically called a destructuring parameter .
Here’s another example:
In the snippet above, we used two array destructuring parameters to extract the profile array’s values into the getUserBio ’s website and userName parameters.
There are times you may need to invoke a function containing a destructuring parameter without passing any argument to it. In that case, you will need to use a technique that prevents the browser from throwing a TypeError .
Let’s learn about the technique below.
How to Invoke a Function Containing Array Destructuring Parameters Without Supplying Any Argument
Consider the function below:
Now, let’s invoke the getUserBio function without passing any argument to its destructuring parameter:
Try it on CodeSandBox
After invoking the getUserBio function above, the browser will throw an error similar to TypeError: undefined is not iterable .
The TypeError message happens because functions containing a destructuring parameter expect you to supply at least one argument.
So, you can avoid such error messages by assigning a default argument to the destructuring parameter.
Notice in the snippet above that we assigned an empty array as the destructuring parameter’s default argument.
So, let’s now invoke the getUserBio function without passing any argument to its destructuring parameter:
The function will output:
Keep in mind that you do not have to use an empty array as the destructuring parameter’s default argument. You can use any other value that is not null or undefined .
So, now that we know how array destructuring works, let's discuss object destructuring so we can see the differences.
What Is Object Destructuring in JavaScript?
Object destructuring is a unique technique that allows you to neatly extract an object’s value into new variables.
For instance, without using the object destructuring assignment technique, we would extract an object’s value into a new variable like so:
Let’s now see how the object destructuring assignment makes things neater and DRY.
You see, like magic, we’ve cleaned up our code by placing the three new variables into a properties object ( {...} ) and assigning them the profile object’s values.
In other words, we instructed the computer to extract the profile object’s values into the variables on the left-hand side of the assignment operator .
Therefore, JavaScript will parse the profile object and copy its first value ( "Oluwatobi" ) into the destructuring object’s first variable ( firstName ).
Likewise, the computer will extract the profile object’s second value ( "Sofela" ) into the destructuring object’s second variable ( lastName ).
Lastly, JavaScript will copy the profile object’s third value ( "codesweetly.com" ) into the destructuring object’s third variable ( website ).
Keep in mind that in { firstName: firstName, lastName: lastName, website: website } , the keys are references to the profile object’s properties – while the keys’ values represent the new variables.

Alternatively, you can use shorthand syntax to make your code easier to read.
In the snippet above, we shortened { firstName: firstName, age: age, gender: gender } to { firstName, age, gender } . You can learn more about the shorthand technique here .
Observe that the snippets above illustrated how to assign an object’s value to a variable when both the object’s property and the variable have the same name.
However, you can also assign a property’s value to a variable of a different name. Let’s see how.
How to Use Object Destructuring When the Property’s Name Differs from That of the Variable
JavaScript permits you to use object destructuring to extract a property’s value into a variable even if both the property and the variable’s names are different.
In the snippet above, the computer successfully extracted the profile object’s values into the variables named forename , surname , and onlineSite —even though the properties and variables are of different names.
Note: const { firstName: forename } = profile is equivalent to const forename = profile.firstName .
In the snippet above, the computer successfully extracted the profile object’s value into the surname variable—even though the property and variable are of different names.
Note: const { lastName: { familyName: surname } } = profile is equivalent to const surname = profile.lastName.familyName .
Notice that so far, we’ve destructured the profile object by referencing it. However, you can also do direct destructuring of an object. Let’s see how.
How to Do Direct Object Destructuring
JavaScript permits direct destructuring of a properties object like so:
Suppose you prefer to separate your variable declarations from their assignments. In that case, JavaScript has you covered. Let see how.
How to Use Object Destructuring While Separating Variable Declarations from Their Assignments
Whenever you use object destructuring, JavaScript allows you to separate your variable declarations from their assignments.
- Make sure that you encase the object destructuring assignment in parentheses. By so doing, the computer will know that the object destructuring is an object literal, not a block.
- Place a semicolon ( ; ) after the parentheses of an object destructuring assignment. By doing so, you will prevent the computer from interpreting the parentheses as an invocator of a function that may be on the previous line.
What if you want "Oluwatobi" assigned to the firstName variable—and the rest of the object’s values to another variable? How can you do this? Let’s find out below.

How to Use Object Destructuring to Assign the Rest of an Object to a Variable
JavaScript allows you to use the rest operator within a destructuring object to assign the rest of an object literal to a variable.
Note: Always use the rest operator as the last item of your destructuring object to avoid getting a SyntaxError .
At times, the value you wish to extract from a properties object is undefined . In that case, JavaScript provides a way to set default values in the destructuring object. Let’s learn more about this below.
How Default Values Work in an Object Destructuring Assignment
Setting a default value can be handy when the value you wish to extract from an object does not exist (or is set to undefined ).
Here’s how you can set one inside a destructuring properties object:
Therefore, in our attempt to extract the second property’s value from the right-hand side object, the computer defaulted to "CodeSweetly" —because only a single property exists in {firstName: "Oluwatobi"} .
So, what if you need to swap firstName ’s value with that of website ? Again, you can use object destructuring to get the job done. Let’s see how below.
How to Use Object Destructuring to Swap Values
You can use the object destructuring assignment to swap the values of two or more different variables.
The snippet above used direct object destructuring to reassign the firstName and website variables with the values of the object literal on the right-hand side of the assignment operator.
Keep in mind that you can also use object destructuring to extract values from properties to a function’s parameters. Let’s talk more about this below.
How to Use Object Destructuring to Extract Values from Properties to a Function’s Parameters
Here’s how you can use object destructuring to copy a property’s value to a function’s parameter:
In the snippet above, we used an object destructuring parameter to copy the profile object’s values into getUserBio ’s firstName and lastName parameters.
Note: An object destructuring parameter is typically called a destructuring parameter .
In the snippet above, we used two destructuring parameters to copy the profile object’s values into getUserBio ’s website and userName parameters.
Note: If you are unclear about the destructuring parameter above, you may grasp it better by reviewing this section .
How to Invoke a Function Containing Destructured Parameters Without Supplying Any Argument
After invoking the getUserBio function above, the browser will throw an error similar to TypeError: (destructured parameter) is undefined .
Notice that in the snippet above, we assigned an empty object as the destructuring parameter’s default argument.
Keep in mind that you do not have to use an empty object as the destructuring parameter’s default argument. You can use any other value that is not null or undefined .
Wrapping It Up
Array and object destructuring work similarly. The main difference between the two destructuring assignments is this:
- Array destructuring extracts values from an array. But object destructuring extracts values from a JavaScript object.
This article discussed how array and object destructuring works in JavaScript. We also looked at the main difference between the two destructuring assignments.
Thanks for reading!
And here's a useful ReactJS resource:
I wrote a book about React!
- It's beginner’s friendly ✔
- It has live code snippets ✔
- It contains scalable projects ✔
- It has plenty of easy-to-grasp examples ✔
The React Explained Clearly book is all you need to understand ReactJS.
Click Here to Get Your Copy

O-sweet-programming, my interest is to make you sweeter for all.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
- Skip to main content
- Select language
- Skip to search
- Expressions and operators
- Operator precedence
Left-hand-side expressions
« Previous Next »
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more.
A complete and detailed list of operators and expressions is also available in the reference .
JavaScript has the following types of operators. This section describes the operators and contains information about operator precedence.
- Assignment operators
- Comparison operators
- Arithmetic operators
- Bitwise operators
Logical operators
String operators, conditional (ternary) operator.
- Comma operator
Unary operators
- Relational operator
JavaScript has both binary and unary operators, and one special ternary operator, the conditional operator. A binary operator requires two operands, one before the operator and one after the operator:
For example, 3+4 or x*y .
A unary operator requires a single operand, either before or after the operator:
For example, x++ or ++x .
An assignment operator assigns a value to its left operand based on the value of its right operand. The simple assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x .
There are also compound assignment operators that are shorthand for the operations listed in the following table:
Destructuring
For more complex assignments, the destructuring assignment syntax is a JavaScript expression that makes it possible to extract data from arrays or objects using a syntax that mirrors the construction of array and object literals.
A comparison operator compares its operands and returns a logical value based on whether the comparison is true. The operands can be numerical, string, logical, or object values. Strings are compared based on standard lexicographical ordering, using Unicode values. In most cases, if the two operands are not of the same type, JavaScript attempts to convert them to an appropriate type for the comparison. This behavior generally results in comparing the operands numerically. The sole exceptions to type conversion within comparisons involve the === and !== operators, which perform strict equality and inequality comparisons. These operators do not attempt to convert the operands to compatible types before checking equality. The following table describes the comparison operators in terms of this sample code:
Note: ( => ) is not an operator, but the notation for Arrow functions .
An arithmetic operator takes numerical values (either literals or variables) as their operands and returns a single numerical value. The standard arithmetic operators are addition ( + ), subtraction ( - ), multiplication ( * ), and division ( / ). These operators work as they do in most other programming languages when used with floating point numbers (in particular, note that division by zero produces Infinity ). For example:
In addition to the standard arithmetic operations (+, -, * /), JavaScript provides the arithmetic operators listed in the following table:
A bitwise operator treats their operands as a set of 32 bits (zeros and ones), rather than as decimal, hexadecimal, or octal numbers. For example, the decimal number nine has a binary representation of 1001. Bitwise operators perform their operations on such binary representations, but they return standard JavaScript numerical values.
The following table summarizes JavaScript's bitwise operators.
Bitwise logical operators
Conceptually, the bitwise logical operators work as follows:
- The operands are converted to thirty-two-bit integers and expressed by a series of bits (zeros and ones). Numbers with more than 32 bits get their most significant bits discarded. For example, the following integer with more than 32 bits will be converted to a 32 bit integer: Before: 11100110111110100000000000000110000000000001 After: 10100000000000000110000000000001
- Each bit in the first operand is paired with the corresponding bit in the second operand: first bit to first bit, second bit to second bit, and so on.
- The operator is applied to each pair of bits, and the result is constructed bitwise.
For example, the binary representation of nine is 1001, and the binary representation of fifteen is 1111. So, when the bitwise operators are applied to these values, the results are as follows:
Note that all 32 bits are inverted using the Bitwise NOT operator, and that values with the most significant (left-most) bit set to 1 represent negative numbers (two's-complement representation).
Bitwise shift operators
The bitwise shift operators take two operands: the first is a quantity to be shifted, and the second specifies the number of bit positions by which the first operand is to be shifted. The direction of the shift operation is controlled by the operator used.
Shift operators convert their operands to thirty-two-bit integers and return a result of the same type as the left operand.
The shift operators are listed in the following table.
Logical operators are typically used with Boolean (logical) values; when they are, they return a Boolean value. However, the && and || operators actually return the value of one of the specified operands, so if these operators are used with non-Boolean values, they may return a non-Boolean value. The logical operators are described in the following table.
Examples of expressions that can be converted to false are those that evaluate to null, 0, NaN, the empty string (""), or undefined.
The following code shows examples of the && (logical AND) operator.
The following code shows examples of the || (logical OR) operator.
The following code shows examples of the ! (logical NOT) operator.
Short-circuit evaluation
As logical expressions are evaluated left to right, they are tested for possible "short-circuit" evaluation using the following rules:
- false && anything is short-circuit evaluated to false.
- true || anything is short-circuit evaluated to true.
The rules of logic guarantee that these evaluations are always correct. Note that the anything part of the above expressions is not evaluated, so any side effects of doing so do not take effect.
In addition to the comparison operators, which can be used on string values, the concatenation operator (+) concatenates two string values together, returning another string that is the union of the two operand strings.
For example,
The shorthand assignment operator += can also be used to concatenate strings.
The conditional operator is the only JavaScript operator that takes three operands. The operator can have one of two values based on a condition. The syntax is:
If condition is true, the operator has the value of val1 . Otherwise it has the value of val2 . You can use the conditional operator anywhere you would use a standard operator.
This statement assigns the value "adult" to the variable status if age is eighteen or more. Otherwise, it assigns the value "minor" to status .
The comma operator ( , ) simply evaluates both of its operands and returns the value of the last operand. This operator is primarily used inside a for loop, to allow multiple variables to be updated each time through the loop.
For example, if a is a 2-dimensional array with 10 elements on a side, the following code uses the comma operator to update two variables at once. The code prints the values of the diagonal elements in the array:
A unary operation is an operation with only one operand.
The delete operator deletes an object, an object's property, or an element at a specified index in an array. The syntax is:
where objectName is the name of an object, property is an existing property, and index is an integer representing the location of an element in an array.
The fourth form is legal only within a with statement, to delete a property from an object.
You can use the delete operator to delete variables declared implicitly but not those declared with the var statement.
If the delete operator succeeds, it sets the property or element to undefined . The delete operator returns true if the operation is possible; it returns false if the operation is not possible.
Deleting array elements
When you delete an array element, the array length is not affected. For example, if you delete a[3] , a[4] is still a[4] and a[3] is undefined.
When the delete operator removes an array element, that element is no longer in the array. In the following example, trees[3] is removed with delete . However, trees[3] is still addressable and returns undefined .
If you want an array element to exist but have an undefined value, use the undefined keyword instead of the delete operator. In the following example, trees[3] is assigned the value undefined , but the array element still exists:
The typeof operator is used in either of the following ways:
The typeof operator returns a string indicating the type of the unevaluated operand. operand is the string, variable, keyword, or object for which the type is to be returned. The parentheses are optional.
Suppose you define the following variables:
The typeof operator returns the following results for these variables:
For the keywords true and null , the typeof operator returns the following results:
For a number or string, the typeof operator returns the following results:
For property values, the typeof operator returns the type of value the property contains:
For methods and functions, the typeof operator returns results as follows:
For predefined objects, the typeof operator returns results as follows:
The void operator is used in either of the following ways:
The void operator specifies an expression to be evaluated without returning a value. expression is a JavaScript expression to evaluate. The parentheses surrounding the expression are optional, but it is good style to use them.
You can use the void operator to specify an expression as a hypertext link. The expression is evaluated but is not loaded in place of the current document.
The following code creates a hypertext link that does nothing when the user clicks it. When the user clicks the link, void(0) evaluates to undefined , which has no effect in JavaScript.
The following code creates a hypertext link that submits a form when the user clicks it.
Relational operators
A relational operator compares its operands and returns a Boolean value based on whether the comparison is true.
The in operator returns true if the specified property is in the specified object. The syntax is:
where propNameOrNumber is a string or numeric expression representing a property name or array index, and objectName is the name of an object.
The following examples show some uses of the in operator.
The instanceof operator returns true if the specified object is of the specified object type. The syntax is:
where objectName is the name of the object to compare to objectType , and objectType is an object type, such as Date or Array .
Use instanceof when you need to confirm the type of an object at runtime. For example, when catching exceptions, you can branch to different exception-handling code depending on the type of exception thrown.
For example, the following code uses instanceof to determine whether theDay is a Date object. Because theDay is a Date object, the statements in the if statement execute.
The precedence of operators determines the order they are applied when evaluating an expression. You can override operator precedence by using parentheses.
The following table describes the precedence of operators, from highest to lowest.
A more detailed version of this table, complete with links to additional details about each operator, may be found in JavaScript Reference .
- Expressions
An expression is any valid unit of code that resolves to a value.
Every syntactically valid expression resolves to some value but conceptually, there are two types of expressions: with side effects (for example: those that assign value to a variable) and those that in some sense evaluates and therefore resolves to value.
The expression x = 7 is an example of the first type. This expression uses the = operator to assign the value seven to the variable x . The expression itself evaluates to seven.
The code 3 + 4 is an example of the second expression type. This expression uses the + operator to add three and four together without assigning the result, seven, to a variable. JavaScript has the following expression categories:
- Arithmetic: evaluates to a number, for example 3.14159. (Generally uses arithmetic operators .)
- String: evaluates to a character string, for example, "Fred" or "234". (Generally uses string operators .)
- Logical: evaluates to true or false. (Often involves logical operators .)
- Primary expressions: Basic keywords and general expressions in JavaScript.
- Left-hand-side expressions: Left values are the destination of an assignment.
Primary expressions
Basic keywords and general expressions in JavaScript.
Use the this keyword to refer to the current object. In general, this refers to the calling object in a method. Use this either with the dot or the bracket notation:
Suppose a function called validate validates an object's value property, given the object and the high and low values:
You could call validate in each form element's onChange event handler, using this to pass it the form element, as in the following example:
- Grouping operator
The grouping operator ( ) controls the precedence of evaluation in expressions. For example, you can override multiplication and division first, then addition and subtraction to evaluate addition first.
Comprehensions
Comprehensions are an experimental JavaScript feature, targeted to be included in a future ECMAScript version. There are two versions of comprehensions:
Comprehensions exist in many programming languages and allow you to quickly assemble a new array based on an existing one, for example.
Left values are the destination of an assignment.
You can use the new operator to create an instance of a user-defined object type or of one of the built-in object types. Use new as follows:
The super keyword is used to call functions on an object's parent. It is useful with classes to call the parent constructor, for example.
Spread operator
The spread operator allows an expression to be expanded in places where multiple arguments (for function calls) or multiple elements (for array literals) are expected.
Example: Today if you have an array and want to create a new array with the existing one being part of it, the array literal syntax is no longer sufficient and you have to fall back to imperative code, using a combination of push , splice , concat , etc. With spread syntax this becomes much more succinct:
Similarly, the spread operator works with function calls:
Document Tags and Contributors
- l10n:priority
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Legacy generator function expression
- Logical Operators
- Object initializer
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration`X' before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
How TO - The Spread Operator (...)
Learn how to use the three dots operator (...) a.k.a the spread operator in JavaScript.
The Spread Operator
The JavaScript spread operator ( ... ) expands an iterable (like an array) into more elements.
This allows us to quickly copy all or parts of an existing array into another array:
Assign the first and second items from numbers to variables and put the rest in an array:
The spread operator is often used to extract only what's needed from an array:
We can use the spread operator with objects too:
Notice the properties that did not match were combined, but the property that did match, color , was overwritten by the last object that was passed, updateMyVehicle . The resulting color is now yellow.
See also : JavaScript ES6 Tutorial .

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.
Home » JavaScript Tutorial » JavaScript Logical Assignment Operators
JavaScript Logical Assignment Operators
Summary : in this tutorial, you’ll learn about JavaScript logical assignment operators, including the logical OR assignment operator ( ||= ), the logical AND assignment operator ( &&= ), and the nullish assignment operator ( ??= ).
ES2021 introduces three logical assignment operators including:
- Logical OR assignment operator ( ||= )
- Logical AND assignment operator ( &&= )
- Nullish coalescing assignment operator ( ??= )
The following table shows the equivalent of the logical assignments operator:
The Logical OR assignment operator
The logical OR assignment operator ( ||= ) accepts two operands and assigns the right operand to the left operand if the left operand is falsy:
In this syntax, the ||= operator only assigns y to x if x is falsy. For example:
In this example, the title variable is undefined , therefore, it’s falsy. Since the title is falsy, the operator ||= assigns the 'untitled' to the title . The output shows the untitled as expected.
See another example:
In this example, the title is 'JavaScript Awesome' so it is truthy. Therefore, the logical OR assignment operator ( ||= ) doesn’t assign the string 'untitled' to the title variable.
The logical OR assignment operator:
is equivalent to the following statement that uses the logical OR operator :
Like the logical OR operator, the logical OR assignment also short-circuits. It means that the logical OR assignment operator only performs an assignment when the x is falsy.
The following example uses the logical assignment operator to display a default message if the search result element is empty:
The Logical AND assignment operator
The logical AND assignment operator only assigns y to x if x is truthy:
The logical AND assignment operator also short-circuits. It means that
is equivalent to:
The following example uses the logical AND assignment operator to change the last name of a person object if the last name is truthy:
The nullish coalescing assignment operator
The nullish coalescing assignment operator only assigns y to x if x is null or undefined :
It’s equivalent to the following statement that uses the nullish coalescing operator :
The following example uses the nullish coalescing assignment operator to add a missing property to an object:
In this example, the user.nickname is undefined , therefore, it’s nullish. The nullish coalescing assignment operator assigns the string 'anonymous' to the user.nickname property.
The following table illustrates how the logical assignment operators work:
- The logical OR assignment ( x ||= y ) operator only assigns y to x if x is falsy.
- The logical AND assignment ( x &&= y ) operator only assigns y to x if x is truthy.
- The nullish coalescing assignment ( x ??= y ) operator only assigns y to x if x is nullish.
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
- NCERT Solutions for Class 10 History Chapter 4 : The Age of Industrialisation
- Check if given path between two nodes of a graph represents a shortest paths
- 5 Tips On Learning How to Code - General Advice For Programmers
- Introduction to React-Redux
- Set value of unsigned char array in C during runtime
- How to perform a real time search and filter on a HTML table?
- CSS will-change property
- HTTP headers | Range
- Sirion Labs Interview Experience (Off-Campus FTE)
- Amazon Interview Experience for SDE-2
- Standard Chartered Interview Experience | On-Campus Internship
- BlackRock Interview Experience | On-Campus Internship 2019
- DE-Shaw Interview Experience (On-Campus)
- Amazon Interview Experience for SDE 1 (1.5 years Experienced)
- Cvent Interview Experience(Pool Campus for fulltime)
- Check if any K ranges overlap at any point
- How Software Is Made?
- IIT Madras M.S Admission Experience
- Public vs Protected in C++ with Examples
Assignment Operators in Programming
Assignment operators in programming are symbols used to assign values to variables. They offer shorthand notations for performing arithmetic operations and updating variable values in a single step. These operators are fundamental in most programming languages and help streamline code while improving readability.
Table of Content
What are Assignment Operators?
- Types of Assignment Operators
- Assignment Operators in C
- Assignment Operators in C++
- Assignment Operators in Java
- Assignment Operators in Python
- Assignment Operators in C#
- Assignment Operators in Javascript
- Application of Assignment Operators
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign ( = ), which assigns the value on the right side of the operator to the variable on the left side.
Types of Assignment Operators:
- Simple Assignment Operator ( = )
- Addition Assignment Operator ( += )
- Subtraction Assignment Operator ( -= )
- Multiplication Assignment Operator ( *= )
- Division Assignment Operator ( /= )
- Modulus Assignment Operator ( %= )
Below is a table summarizing common assignment operators along with their symbols, description, and examples:
Assignment Operators in C:
Here are the implementation of Assignment Operator in C language:
Assignment Operators in C++:
Here are the implementation of Assignment Operator in C++ language:
Assignment Operators in Java:
Here are the implementation of Assignment Operator in java language:
Assignment Operators in Python:
Here are the implementation of Assignment Operator in python language:
Assignment Operators in C#:
Here are the implementation of Assignment Operator in C# language:
Assignment Operators in Javascript:
Here are the implementation of Assignment Operator in javascript language:
Application of Assignment Operators:
- Variable Initialization : Setting initial values to variables during declaration.
- Mathematical Operations : Combining arithmetic operations with assignment to update variable values.
- Loop Control : Updating loop variables to control loop iterations.
- Conditional Statements : Assigning different values based on conditions in conditional statements.
- Function Return Values : Storing the return values of functions in variables.
- Data Manipulation : Assigning values received from user input or retrieved from databases to variables.
Conclusion:
In conclusion, assignment operators in programming are essential tools for assigning values to variables and performing operations in a concise and efficient manner. They allow programmers to manipulate data and control the flow of their programs effectively. Understanding and using assignment operators correctly is fundamental to writing clear, efficient, and maintainable code in various programming languages.
Please Login to comment...
- Programming
- How to Delete Whatsapp Business Account?
- Discord vs Zoom: Select The Efficienct One for Virtual Meetings?
- Otter AI vs Dragon Speech Recognition: Which is the best AI Transcription Tool?
- Google Messages To Let You Send Multiple Photos
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

IMAGES
VIDEO
COMMENTS
Unpacking values from a regular expression match. When the regular expression exec() method finds a match, it returns an array containing first the entire matched portion of the string and then the portions of the string that matched each parenthesized group in the regular expression. Destructuring assignment allows you to unpack the parts out of this array easily, ignoring the full match if ...
If an object or an array contains other nested objects and arrays, we can use more complex left-side patterns to extract deeper portions. In the code below options has another object in the property size and an array in the property items. The pattern on the left side of the assignment has the same structure to extract values from them:
When your "test" function returns, you're correct that "names" is "gone". However, its value is not, because it's been assigned to a global variable. The value of the "names" local variable was a reference to an array object. That reference was copied into the global variable, so now that global variable also contains a reference to the array object.
An assignment operator assigns a value to its left operand based on the value of its right operand.. Overview. The basic assignment operator is equal (=), which assigns the value of its right operand to its left operand.That is, x = y assigns the value of y to x.The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
An assignment operator ( =) assigns a value to a variable. The syntax of the assignment operator is as follows: let a = b; Code language: JavaScript (javascript) In this syntax, JavaScript evaluates the expression b first and assigns the result to the variable a. The following example declares the counter variable and initializes its value to zero:
The ( ..) around the assignment statement is required syntax when using object literal destructuring assignment without a declaration. {a, b} = {a: 1, b: 2} is not valid stand-alone syntax, as the {a, b} on the left-hand side is considered a block and not an object literal. However, ({a, b} = {a: 1, b: 2}) is valid, as is var {a, b} = {a: 1, b: 2} NOTE: Your ( ..) expression needs to be ...
In JavaScript, an array is an object constituted by a group of items having a specific order. Arrays can hold values of mixed data types and their size is not fixed. How to Create an Array in JavaScript. You can create an array using a literal syntax - specifying its content inside square brackets, with each item separated by a comma.
In this tutorial, you will learn about all the different assignment operators in javascript and how to use them in javascript. Assignment Operators. In javascript, there are 16 different assignment operators that are used to assign value to the variable. It is shorthand of other operators which is recommended to use.
The destructuring assignment is a special operator that allows you to "unpack" or "extract" the values of JavaScript arrays and objects. It has become one of the most useful features of JavaScript language for two reasons: It helps you to avoid code repetition. It keeps your code clean and easy to understand.
Get started. The destructuring assignment in JavaScript provides a neat and DRY way to extract values from your arrays and objects. This article aims to show you exactly how array and object destructuring assignments work in JavaScript. So, without any further ado, let's get started with array destructuring.
This example shows three ways to create new array: first using array literal notation, then using the Array () constructor, and finally using String.prototype.split () to build the array from a string. js. // 'fruits' array created using array literal notation. const fruits = ["Apple", "Banana"];
The shorthand assignment operator += can also be used to concatenate strings. For example, var mystring = 'alpha'; mystring += 'bet'; // evaluates to "alphabet" and assigns this value to mystring. Conditional (ternary) operator. The conditional operator is the only JavaScript operator that takes three operands. The operator can have one of two ...
Supported Browsers: The browsers supported by all JavaScript Assignment operators are listed below: Google Chrome. Firefox. Opera. Safari. Microsoft Edge. Internet Explorer. "This course was packed with amazing and well-organized content! The project-based approach of this course made it even better to understand concepts faster.
Example. Assign the first and second items from numbers to variables and put the rest in an array: const numbersOne = [1, 2, 3]; const numbersTwo = [4, 5, 6]; const numbersCombined = [...numbersOne, ...numbersTwo]; Try it Yourself ». The spread operator is often used to extract only what's needed from an array:
The logical OR assignment operator ( ||=) accepts two operands and assigns the right operand to the left operand if the left operand is falsy: In this syntax, the ||= operator only assigns y to x if x is falsy. For example: console .log(title); Code language: JavaScript (javascript) In this example, the title variable is undefined, therefore ...
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
2 Answers. Sorted by: 1. You can assign something to an array or and object, but the way you do it is different. If you're assigning something to an array, you should be using an index (though @tehhowch's answer is correct, but we never assign values to an array in the same way we do to an object).
0. In Vue.js, array changes detection is based on JavaScript's built-in array mutation methods like push, pop, shift, unshift, splice, sort, and reverse. Vue.js uses these methods to detect changes in arrays and trigger reactive updates in the DOM. However, there are some caveats and considerations to keep in mind when working with arrays in ...